Introduction to Error Handling in Python
In the world of programming, errors are inevitable. Whether you’re a novice or an experienced developer, managing these issues politely is essential to keeping programs stable and easy to use. Python, a versatile and widely-used programming language, offers powerful tools for error handling, primarily through the try, accept, and finally blocks. This blog post will guide you through mastering error handling in Python, ensuring your code can handle unexpected situations with ease.
Understanding Exception Types in Python
Errors in Python are typically handled as exceptions. Python raises these exceptions—built-in error types—when something goes wrong. Understanding these exception types is the first step toward effective error handling.
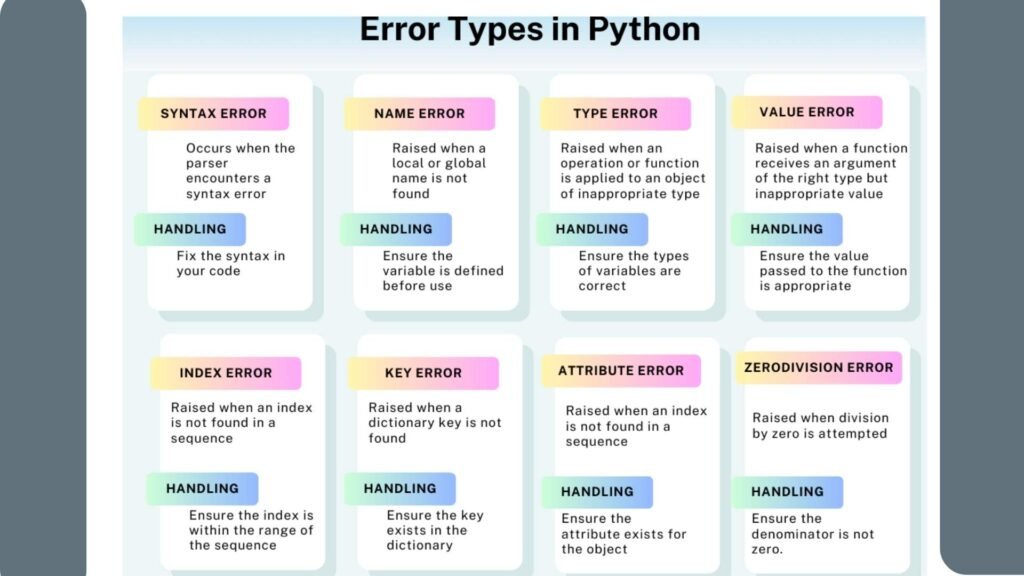
Common Built-in Exception Types
- Syntax Error: Raised when there is an error in the program’s syntax.
- Type Error: This happens when an operation is performed on an incorrectly typed object.
- Value Error: Issued when a function is called with an argument that is the right type but has the wrong value.
- Index Error: This happens when attempting to obtain an element from a list using an index out of range.
- Key Error: raised when a dictionary is accessed with a key that does not exist.
Real-World Scenarios
- Syntax Error: Helps identify erroneous code during the development stage.
- Type Error: Essential when dealing with functions that expect specific data types.
- Value Error: Important for validating user inputs in applications.
- Index Error: Critical when working with lists or arrays to prevent program crashes.
- Key Error: necessary when manipulating dictionaries, especially with dynamic keys.
The Try-Except Block Basic Syntax and Use Cases
Python’s try-except block serves as the cornerstone for managing errors. Python allows you to “try” executing a block of code and “accept” any exceptions that occur, preventing the program from crashing.
Basic Syntax
A basic try-except block example is as follows:
Try:
# Code that may cause an exception
result = 10 / 0
except ZeroDivisionError:
# Code to execute if the exception occurs
print(“Cannot divide by zero.”)
Common Use Cases
- File Operations: Handling file not found errors when attempting to open a file.
- User input validation: managing incorrect data types or invalid input values.
- Database Connections: Catching connection errors or query failures.
Practical Example
Imagine the following situation where you must read a file:
Try:
with open(‘data.txt’, ‘r’) as file:
content = file.read()
except FileNotFoundError:
print(“The file does not exist.”)
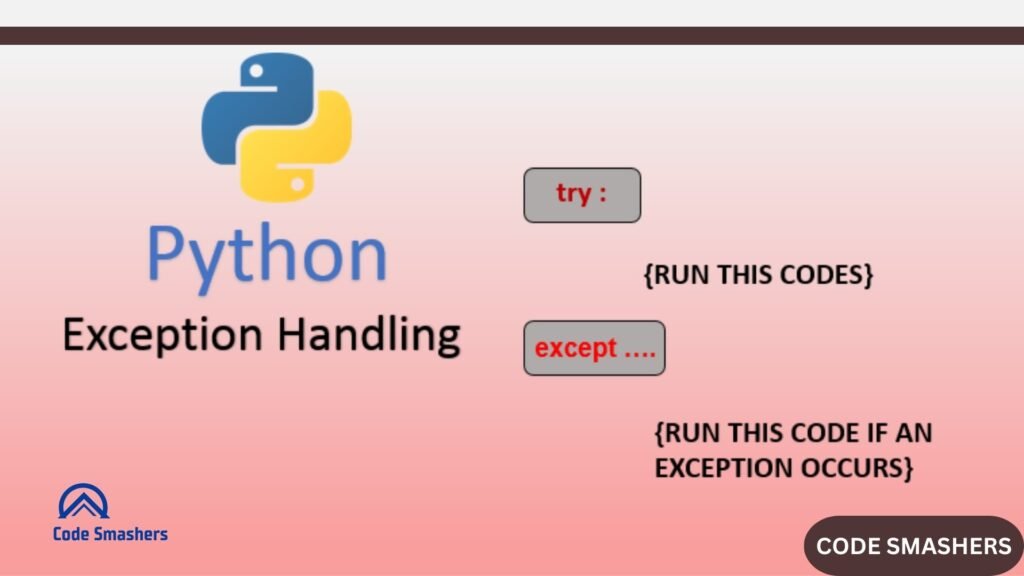
Multiple Except Blocks and Handling Specific Exceptions
Python allows you to handle numerous exceptions using multiple except blocks. This feature in Python provides granular control over different types of errors.
Handling Multiple Exceptions
try:
# Code that may cause multiple exceptions
result = 10 / 0
number = int(“not a number”)
except ZeroDivisionError:
print(“Cannot divide by zero.”)
except ValueError:
print(“Invalid value provided.”)
Best Practices
- Be Specific: Handle specific exceptions rather than a generic exception.
- Order Matters: Place specific exceptions before general exceptions.
- Minimal Code in Try Block: Keep the try block short, focusing only on code that may cause an exception.
Example
try:
with open(‘data.txt’, ‘r’) as file:
content = file.read()
number = int(content)
except FileNotFoundError:
print(“The file does not exist.”)
except ValueError:
print(“The file’s content is not an integer.”)
The Else and Finally Clauses in Error Handling
The else and finally clauses provide additional control over error handling, ensuring certain code runs regardless of exceptions in the Python programming language.
Purpose and Usage
- Else Clause: Executes if no exceptions are raised in the try block.
- Finally, Clause carries out the action whether or not an exception occurred.
Examples
Else Clause Example
try:
number = int(input(“Enter a number: “))
except ValueError:
print(“That’s not a valid number.”)
else:
print(“The number is:”, number)
Finally, Clause Example
try:
file = open(‘data.txt’, ‘r’)
content = file.read()
except FileNotFoundError:
print(“The file does not exist.”)
finally:
file.close()
print(“File closed.”)
Comprehensive Error Handling
Integrating every block can result in reliable error management.
Try:
file = open(‘data.txt’, ‘r’)
content = file.read()
number = int(content)
except FileNotFoundError:
print(“The file does not exist.”)
except ValueError:
print(“The file’s content is not an integer.”)
else:
print(“Successfully read the file and converted content to an integer.”)
finally:
file.close()
print(“File closed.”)
Best Practices for Exception Handling in Python
Effective error handling in Python goes beyond just using try-except blocks. It involves adopting best practices to ensure your code remains clean, readable, and efficient.
Key Best Practices
- Employ Specific Exceptions: Debugging becomes more difficult when broad exceptions, such as `Exception}, are used.
- Log Exceptions: Use logging to keep track of exceptions without exposing them to end-users.
- Avoid silent failures. Always provide meaningful messages when exceptions occur.
Tips for Optimal Code Performance
- Minimal Try Blocks: Limit the scope of try blocks to only the code that might raise an exception.
- Resource Management: Use context managers (`with` statement) to handle resources like files and network connections.
- Custom Exceptions: Create custom exception classes to handle specific error conditions unique to your application.
Example of Custom Exception
class CustomError(Exception):
pass
try:
raise CustomError(“This is a custom error.”)
except for CustomError as
print(e)
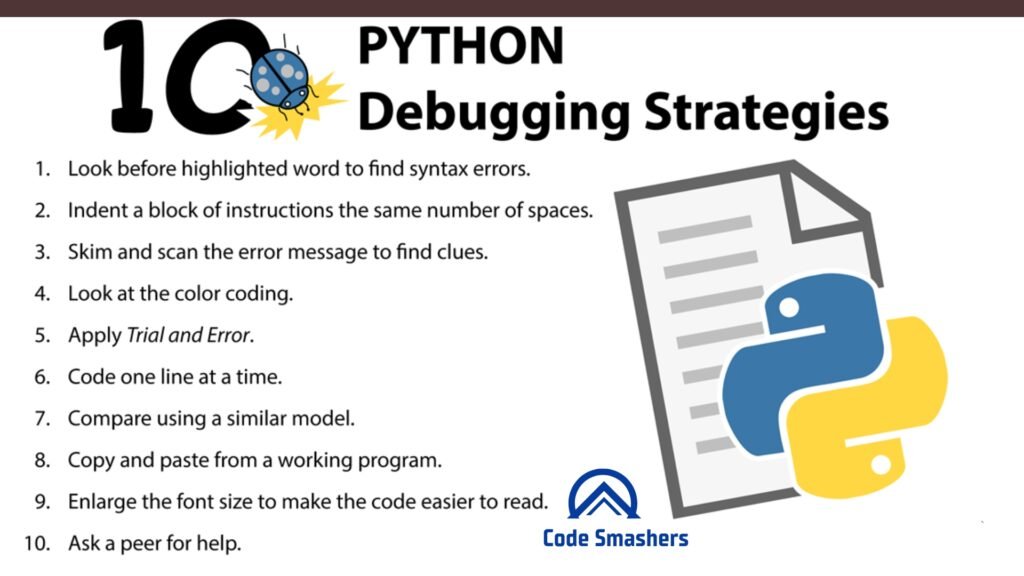
Debugging Techniques and Tips
Effective debugging is an essential skill for any programmer aiming to resolve issues efficiently and improve how code is written. Debugging involves identifying, isolating, and fixing bugs or errors within a program. Here are some techniques and tips that can facilitate the debugging process:
Techniques
Print Statements: One of the simplest and most common debugging techniques is using print statements to display variable values and program flow. This will enable you to comprehend the actions taken by your code at every stage.
- Interactive Debuggers: Utilize built-in debugging tools like Python’s `pdb` or integrated IDE debuggers to set breakpoints, step through code, inspect variables, and evaluate expressions dynamically.
- Logging: Implement logging throughout your application to record various execution states and behaviors. Libraries like `logging` in Python allow configurable logging levels, such as DEBUG, INFO, WARNING, ERROR, and CRITICAL.
- Error Reproduction: Make a consistent effort to reproduce the bug by determining what conditions or inputs lead to it.This can help isolate the cause of the problem.
- Code Review: Sometimes a fresh pair of eyes can identify issues you might have missed. Sharing your code with colleagues for a review can be beneficial.
Tips
- Stay Organized: Document your debugging process and keep track of changes and observations. This can prevent repeating efforts and be a reference for future debugging tasks.
- Understand the Error: Read error messages carefully. They often provide valuable clues about what went wrong and where.
- Break Down Complex Problems: Test each area of your code after dividing it into smaller pieces. Isolating code elements can help locate where an error originates.
- Test Edge Cases: Ensure you consider edge cases or rarely occurring conditions that might cause errors, as they are easy to overlook during development.
- Know Your Tools: Get to know the various debugging tools and fully comprehend their features. Features like conditional breakpoints and watch expressions can save significant time and effort.
Applying these techniques and tips allows you to streamline your debugging process, resulting in quicker resolutions and more robust code. Debugging is as much an art as a science, requiring analytical thinking and a systematic approach.
Real-world examples and Code Snippets
Understanding how error handling is applied in real-world scenarios can significantly enhance your coding skills.
API Integration
When working with external APIs, error handling ensures smooth interaction even when the API response is not what was anticipated.
import requests
try:
response = requests.get(‘https://api.example.com/data’)
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
print(f’HTTP error occurred: {http_err}’)
except Exception as err:
print(f’Other error occurred: {err}’)
else:
print(‘Success:’, response.json())
Web Development
In web development, handling form validation errors prevents server crashes and provides clear feedback to users.
from flask import Flask, request, jsonify
app = Flask(name)
@app.route(‘/submit’, methods=[‘POST’])
def submit():
try:
data = request.json
age = int(data[‘age’])
except ValueError:
return jsonify({“error”: “Invalid age provided”}), 400
except KeyError:
return jsonify({“error”: “Age is required”}), 400
else:
return jsonify({“message”: “Data received successfully”}), 200
if name == ‘main‘:
app.run()
Data Science and Analysis
Data integrity and program stability are ensured by handling errors when processing huge datasets.
import pandas
try:
df = pd.read_csv(‘large_dataset.csv’)
except FileNotFoundError:
print(“The file does not exist.”)
except pd.errors.EmptyDataError:
print(“The file is empty.”)
else:
print(“Data loaded successfully.”)
finally:
print(“Execution complete.”)
File I/O Operations
Managing file operations with proper error handling avoids program interruptions and ensures data integrity.
Try:
with open(‘config.json’, ‘r’) as file:
config = json.load(file)
except FileNotFoundError:
print(“Configuration file not found.”)
except json.JSONDecodeError:
print(“Error decoding JSON from file.”)
else:
print(“Configuration loaded successfully.”)
User Input Validation
Validating and sanitizing user input can prevent unintended program behavior or security vulnerabilities.
def get_user_input():
try:
user_input = input(“Enter a number: “)
return int(user_input)
except ValueError:
print(“Invalid input. Please enter a valid number.”)
return get_user_input()
number = get_user_input()
print(“You entered:”, number)
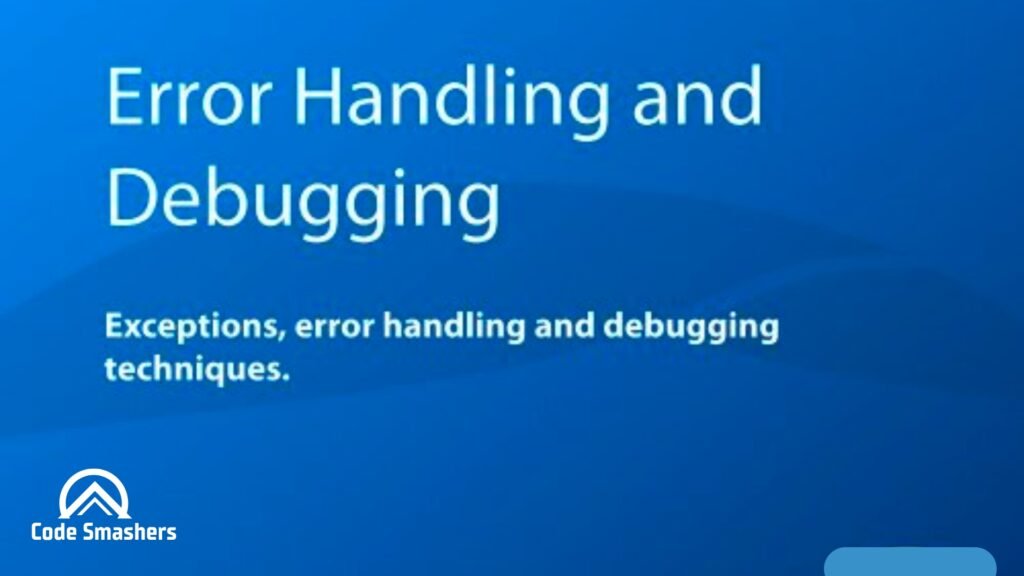
Debugging with Error Handling Tips and Tricks
Integrating error handling within your debugging process can make finding and fixing issues more efficient.
Efficient Debugging Techniques
- Use Breakpoints: Set breakpoints to pause execution and inspect variables.
- Step Through Code: Step through your code to understand its flow and catch exceptions.
- Logging: Add logging statements to track execution flow and capture exception details.
Error Handling in Debugging
- Catch and Log Exceptions: Catch exceptions and log them before re-raising to understand the error context.
- Make Use of Assertions: Assertions can be used to support presumptions and identify mistakes early in the development process.
- Test Thoroughly: Write unit tests to cover different scenarios and verify error handling mechanisms.
Example of Logging Exceptions
import logging
logging.basicConfig(filename=’app.log’, level=logging.ERROR)
try:
result = 10 / 0
except ZeroDivisionError as
logging.error(“Exception occurred”, exc_info=True)
Conclusion and Recap of Key Points
Mastering error handling in Python is essential for developing robust and reliable applications. By understanding exception types, utilizing try-except blocks, and following best practices, you can handle errors effectively and ensure your programs run smoothly.
Call to Action
Ready to elevate your Python skills? Try implementing advanced error handling in your next project. Explore our additional resources for further learning and don’t hesitate to share your experiences or ask questions in the comments section.