Introduction to Python Modules and Packages
Welcome to the world of Python, where clean and organized code reigns supreme. If you’ve just started your coding journey or are a tech enthusiast exploring programming languages, Python’s approach to modules and packages will be a game changer for you. The purpose of this blog post is simple yet powerful – to unravel the mysteries behind Python modules and packages and show you how they can transform your coding experience. By the end of this guide, you’ll understand the difference between modules and packages, know how to create and import them, and appreciate their role in promoting code reusability and organization.
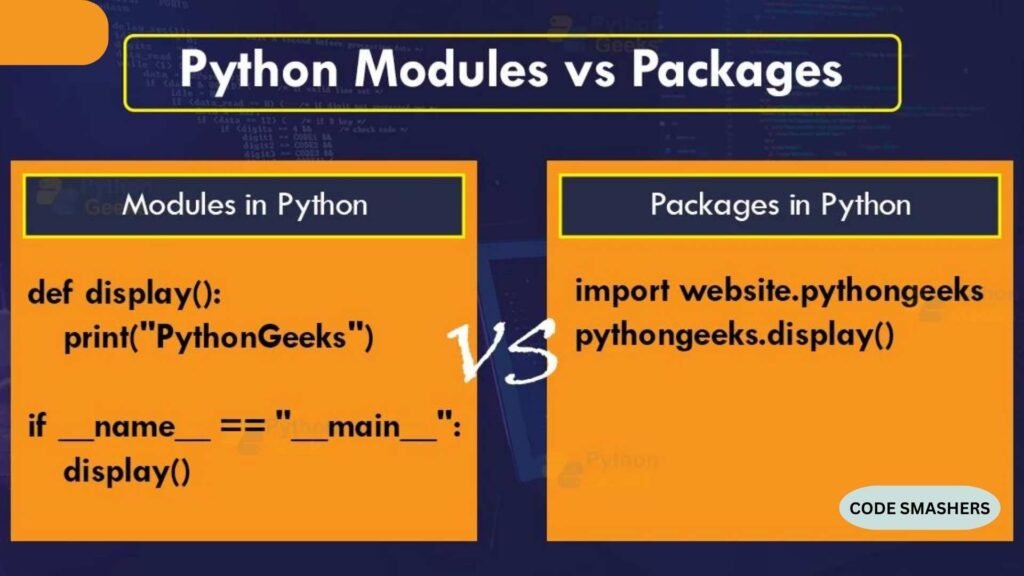
Explaining the Difference Between Modules and Packages
Python modules and packages might seem like jargon at first glance, but they’re fundamental to writing efficient code. Let’s break it down:
- Individual files containing Python code are called modules. They can include functions, classes, and variables. Think of a module as a single script that can be reused across different parts of your project.
- Packages, on the other hand, are directories that bundle multiple modules together. A package typically contains an `init.py` file, signaling to Python that the directory should be treated as a package.
You can reduce redundancy and improve the maintainability of your code by utilizing modules and packages. With the modular approach, you can put together smaller, reusable components to create sophisticated applications.
Importing Modules and Packages in Python
One of the most powerful features of Python is its ability to import and use modules and packages effortlessly. Here’s a step-by-step tutorial to get you going.:
- Importing a Module
import math
print(math.sqrt(16)) # Outputs 4.0
The `math` module is imported in this example, so you can use its functions, such as `sqrt`.
- Importing a Specific Function:
from math import sqrt
print(sqrt(16)) # Outputs 4.0
This method simplifies your code by merely importing the `sqrt` function from the `math` package.
- Importing a Package:
import mypackage.mymodule
mypackage.mymodule.my_function()
You can import and utilize the module named `mymodule} as seen above if it is contained in {mypackage}.
By mastering these import techniques, you can unlock the full potential of Python libraries and make your projects more dynamic.
Creating Your Modules and Packages
Creating your modules and packages can seem daunting, but it’s simpler than you might think. Follow these steps to create a module:
- Create a Python File:
# mymodule.py
def greet(name):
return Hello, {name}!”
This file contains a function `greet` that can be reused in other scripts.
- Create a Package:
plaintext
mypackage/
init.py
mymodule.py
Initialization code may or may not be present in the `init.py` file. The directory structure indicates that `mymodule.py` is part of the `mypackage` package.
By organizing your code into modules and packages, you’ll find it easier to manage and scale your projects.
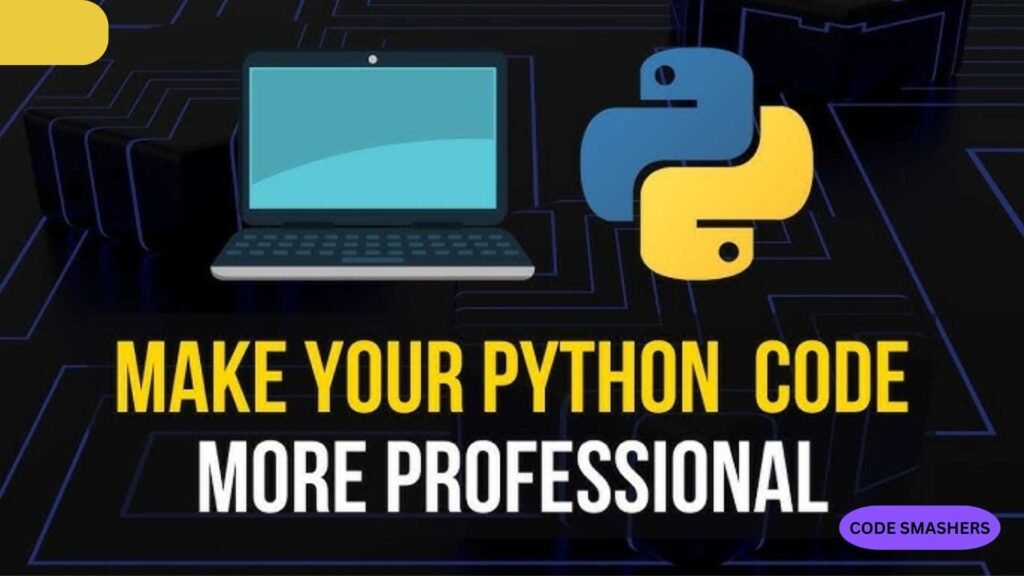
Best Practices for Organizing Your Code with Modules and Packages
To make the most of Python modules and packages, adhere to these best practices:
- Use Meaningful Names:
To make your code easier to read, give your modules and packages meaningful names. For example, instead of naming a module `mod1.py`, name it `data_processing.py`.
- Keep Modules Small and Focused:
Each module should have a single responsibility. This makes your code easier to understand and maintain.
- Document Your Code:
Include docstrings and comments to explain the purpose of your functions, classes, and modules. This helps others (and your future self) understand your code better.
Following these best practices will ensure that your code remains clean, efficient, and easy to maintain.
Advanced Techniques and Real-World Examples
Once you’re comfortable with basics, you can explore advanced techniques for using modules and packages:
- Dynamic Imports:
import importlib
mymodule = importlib.import_module(‘mymodule’)
mymodule.my_function()
This allows you to import modules dynamically, useful for plugin systems or modular applications.
- Alias Imports:
import pandas as pd
df = pd.DataFrame(data)
Using aliases can shorten module names and make your code more concise.
Real-world examples include web frameworks like Django and Flask, which are built using extensive modular structures, demonstrating the power of well-organized code.
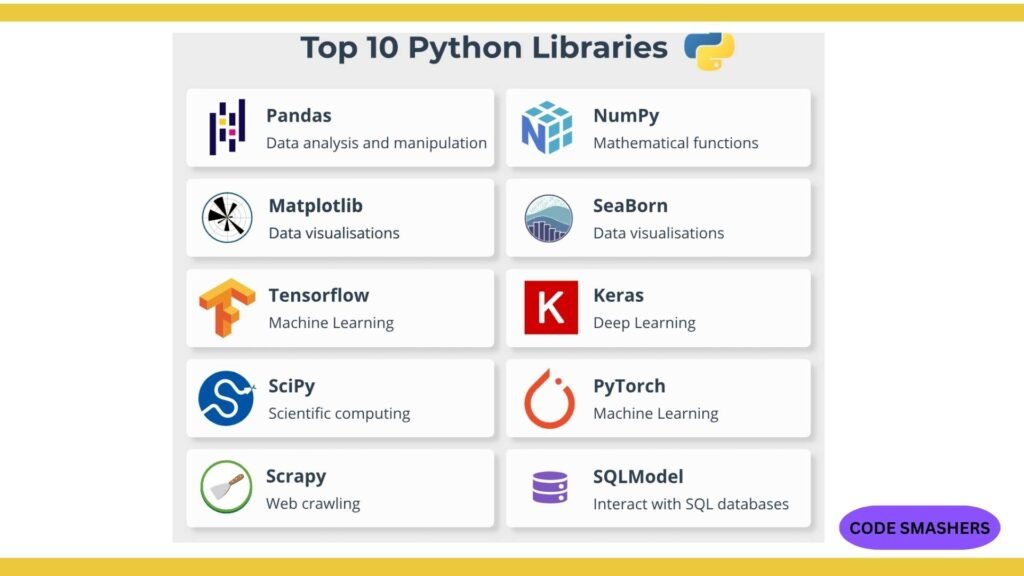
Examples of Python Libraries
Python has a large library with ready-to-use tools and functions for a wide range of tasks.
- NumPy: A fundamental package for numerical computations in Python. It provides support for arrays, matrices, and a broad collection of high-level mathematical functions.
import numpy as np
arr = np.array([1, 2, 3])
print(np.mean(arr)) # Outputs 2.0
2. Pandas: Essential for data manipulation and analysis, especially in handling structured data. It provides data structures like DataFrames that are ideal for working with tabular data.
import pandas as pd
data = {‘Name’: [‘Alice’, ‘Bob’], ‘Age’: [25, 30]}
df = pd.DataFrame(data)
print(df)
3. Matplotlib: a Python plotting package for making static, interactive, and animated visuals.
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 2])
plt.show()
- Requests: A simple and elegant HTTP library for Python, useful for making requests to web services and handling responses.
import requests
response = requests.get(‘https://api.example.com/data’)
print(response.content)
- Scikit-learn: A library for machine learning that provides simple and efficient tools for data mining and data analysis.
from sklearn import datasets
iris = datasets.load_iris()
print(iris.data)
These libraries are just a few examples of how Python’s rich ecosystem can simplify and accelerate development in areas such as data analysis, visualization, web development, and machine learning.
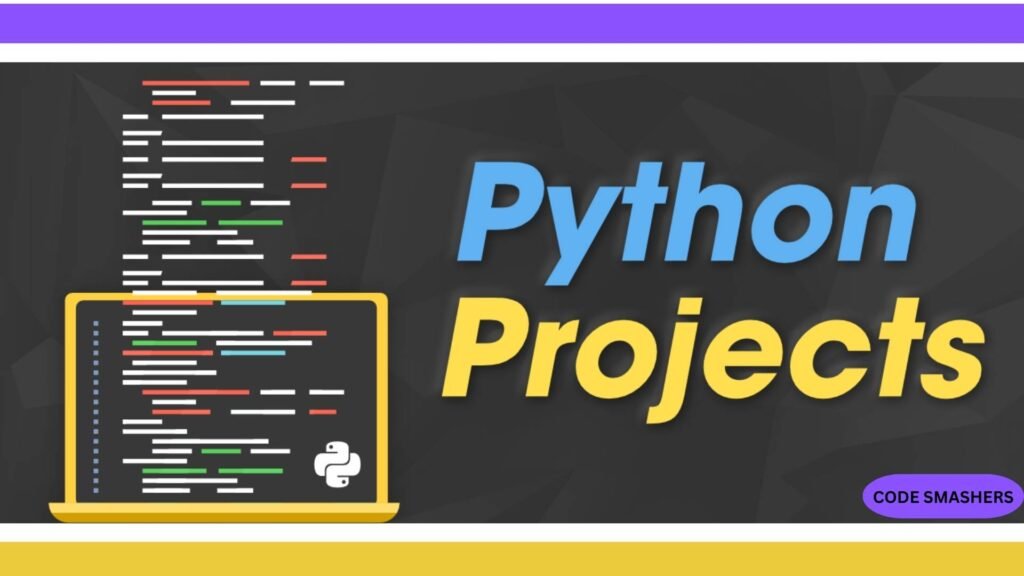
The Importance of Python Libraries in Enhancing Code Reusability
Python libraries are collections of modules and packages designed to solve specific problems. To improve code reusability, they are essential. For instance:
- NumPy and Pandas for data manipulation.
- Requests for handling HTTP requests.
- Matplotlib for data visualization.
Leveraging these libraries can save time and effort and ensure that you’re using well-tested and optimized code.
Conclusion Recap and Next Steps for Python Developers
In this guide, we’ve explored the world of Python modules and packages, from understanding their differences to creating and importing them effectively. We’ve also touched on best practices and advanced techniques to help you organize your code and make it more reusable. Remember, mastering these foundational concepts is the key to becoming a proficient Python developer.
It’s now time for you to apply what you’ve learned. Start by organizing your next project into modules and packages. Examine well-known Python packages to see how they might improve your productivity. If you’re looking for more resources, consider joining Python communities or taking online courses to deepen your understanding.
By following these steps, you’ll be well on your way to writing clean, efficient, and maintainable Python code. Happy coding!