JS JavaScript is one of the most significant languages for web development. Yet, even seasoned developers sometimes struggle with its nuances. Understanding JS JavaScript functions and scope is crucial for writing efficient, maintainable code. This comprehensive guide aims to demystify these concepts, making them accessible for everyone, from programming beginners to seasoned web developers.
Introduction to JS JavaScript Functions
Functions are the building blocks of JS JavaScript. They allow you to encapsulate code for reuse, making your scripts more modular and easier to manage. But what exactly are JS JavaScript functions, and why are they so important?
Functions are reusable chunks of code that accomplish a specified purpose. They can take inputs in the form of parameters, process those inputs, and return an output. Functions assist in breaking down large problems into smaller, more manageable bits.
In this blog post, we will explore different types of functions—such as function declarations, function expressions, and arrow functions—and how they interact with various scopes in JS JavaScript.
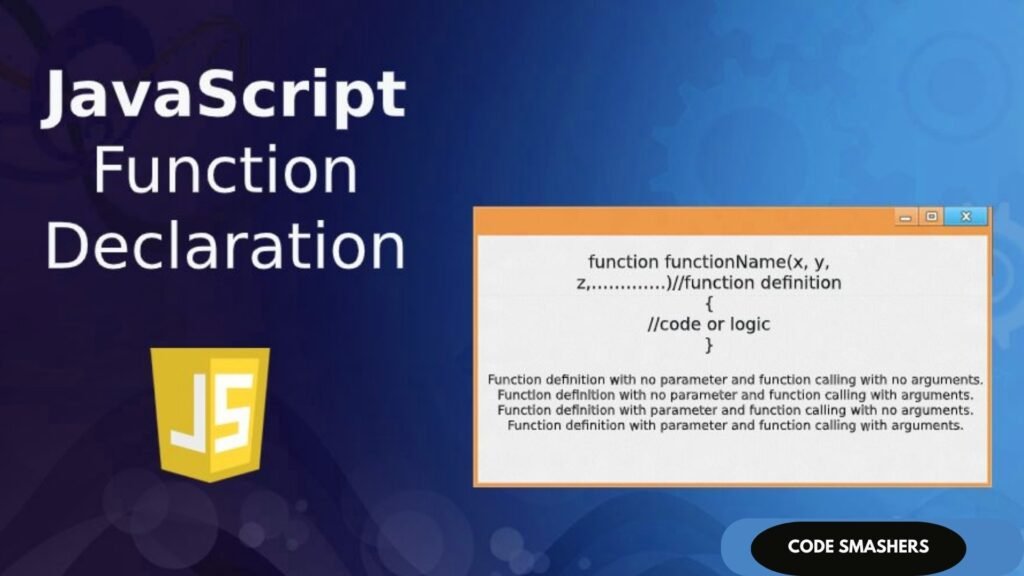
Understanding Function Declarations
Function declarations are one of the simplest ways to define a function in JS JavaScript. The syntax is straightforward:
function greet() {
console.log(“Hello, World!”);
}
ReaThis type of function is hoisted to the top of its scope, meaning it can be called before it is defined. Function declarations are ideal when you need to ensure that a function is available throughout its scope.
Use Cases:
- Utility functions that need to be accessible throughout your script.
- Functions that do not need to be redefined.
Key Points:
- Hoisted to the top of their scope.
- Must have a name.
Exploring Function Expressions
Function expressions provide additional flexibility when defining functions. You may declare a function as part of an expression by assigning it to a variable.
const greet = function() {
console.log(“Hello, World!”);
};
Unlike function declarations, function expressions are not hoisted. They can be anonymous or named, but the name is usually omitted unless it’s for debugging purposes.
Use Cases:
- Functions that need to be defined conditionally.
- Event handlers and callbacks.
Key Points:
- Not hoisted.
- Can be anonymous or named.
The Rise of Arrow Functions
Arrow functions are a more concise way to write function expressions and were introduced in ES6. They provide a shorter syntax and do not bind their own `this`.
const greet = () => {
console.log(“Hello, World!”);
};
Arrow functions are especially useful for writing short functions and are commonly used in array methods like `map`, `filter`, and `reduce`.
Benefits:
- Shorter syntax.
- Lexically binds `this`.
Key Points:
- No binding of `this`.
- Cannot be used as constructors.
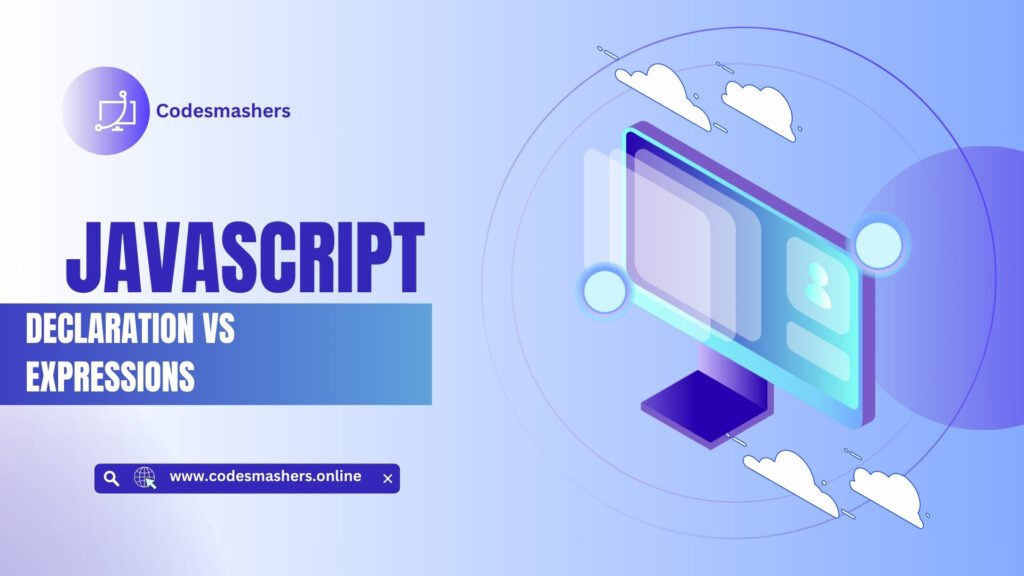
Comparing Function Declarations and Expressions
While both function declarations and expressions serve the same basic purpose, they have some key differences that can affect how and where you use them.
Performance Considerations:
- Function declarations are hoisted, making them more versatile.
- Function expressions offer more flexibility and are excellent for callback functions.
Best Practices:
- Use function declarations for utility functions.
- Use function expressions for inline functions, event handlers, and callbacks.
Local Scope in JS JavaScript
Local scope refers to variables that can only be accessed within a given function. This is crucial for preventing variable name conflicts and ensuring that your variables aren’t accidentally modified by other parts of your code.
function greet() {
const message = “Hello, World!”;
console.log(message); // Accessible here
}
console.log(message); // Error: message is not defined
Benefits:
- Encapsulates variables.
- Prevents name conflicts.
Considerations:
- Local variables can only be accessed within their respective function.
- Function declarations create their own scope, whereas arrow functions share the scope of their surrounding code.
Global Scope in JS JavaScript
Global scope refers to variables that are accessible from anywhere in your code. Variables declared outside of any function have global scope and can be accessed by any other part of your code.
const message = “Hello, World!”;
function greet() {
console.log(message); // Accessible here
}
console.log(message); // Also accessible here
While global variables offer convenience, they also pose a risk of accidental modification and name conflicts.
Benefits:
- Convenient accessibility
Key Points:
- Defined within a function.
- Not accessible outside the function.
Global Scope and Its Implications
Global scope, on the other hand, refers to variables that are accessible throughout your entire script. While this can be convenient, it also comes with risks, such as variable name conflicts and accidental modifications.
var globalMessage = “Hello, World!”;
function greet() {
console.log(globalMessage); // Accessible here
}
console.log(globalMessage); // Also accessible here
Implications:
- Risk of conflicts.
- Harder to manage in large scripts.
Best Practices:
- Minimize the use of global variables.
- Use `const` and `let` for local scope.
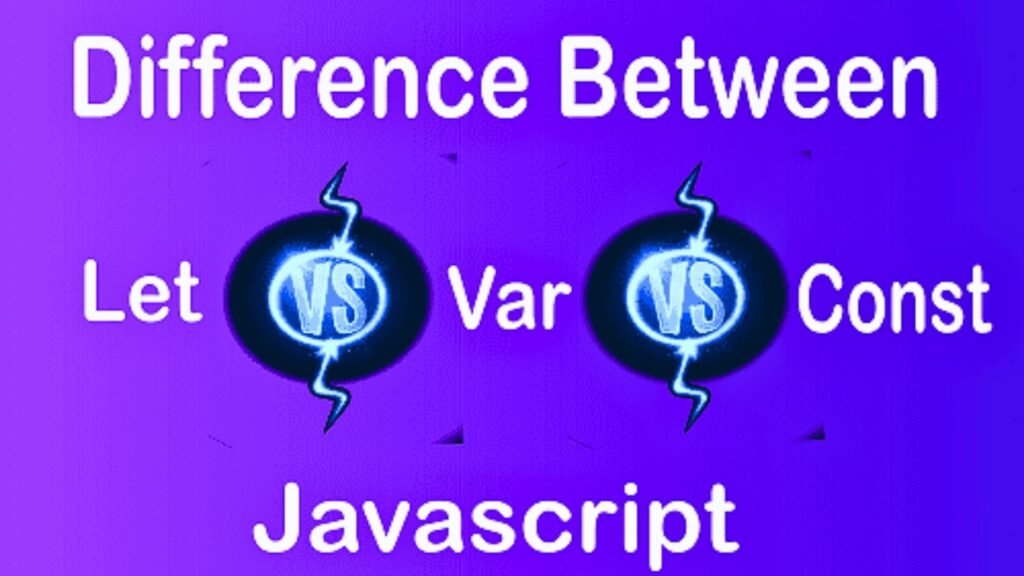
Block Scope and the Let/Const Keywords
ES6 introduces block scope with the ‘let’ and ‘const’ keywords. These keywords allow you to define variables that are restricted to the block in which they are defined, such as within loops or conditional statements.
if (true) {
let message = “Hello, World!”;
console.log(message); // Accessible here
}
console.log(message); // Error: message is not defined
Advantages:
- Reduces the risk of conflicts.
- Makes the code easier to comprehend and maintain.
Key Points:
- Use `let` for variables that change.
- Use `const` for constants.
Understanding Lexical Scope
The lexical scope specifies that variables’ accessibility is determined by their placement inside the nested function scopes. In other words, inner functions have access to variables defined in their outer scope.
function outer() {
const outerMessage = “Hello from outer!”;
function inner() {
console.log(outerMessage); // Accessible here
}
inner();
}
outer();
Benefits:
- Predictable variable access.
- Supports closures.
Key Points:
- Variables are accessible within nested scopes.
- Supports modular code design.
Function Scope vs. Block Scope
Function scope and block scope are two different levels of scoping in JS JavaScript. Function scope is limited to the function in which it is defined, while block scope is limited to a block of code, such as inside loops or conditional statements.
Differences:
- Function scope is more permissive.
- Block scope is more restrictive and safer.
Use Cases:
- Use function scope for larger, more complex functions.
- Use block scope for tight control over variable access.
Closures in JS JavaScript
Closures are a powerful feature in JS JavaScript. They allow a function to retain access to variables from its containing scope even after that scope has finished executing.
function outer() {
const outerMessage = “Hello from outer!”;
return function inner() {
console.log(outerMessage); // Still accessible here
};
}
const innerFunction = outer();
innerFunction(); // Logs “Hello from outer!”
Benefits:
- Enables data privacy.
- Supports functional programming.
Key Points:
- Functions retain access to their containing scope.
- Useful for creating private variables.
The Role of the `this` Keyword in Functions
In JS JavaScript, the value of the ‘this’ keyword varies depending on how a function is invoked. the `this` keyword is a bit tricky in JavaScript, because its value depends on how a function is called. In the context of regular functions, `this` refers to the object from which the function was called. In arrow functions, however, `this` retains the value from its enclosing context.
const object = {
message: “Hello, World!”,
greet() {
console.log(this.message); // Refers to obj
}
};
obj.greet(); // Logs “Hello, World!”
Context and Binding:
- Regular functions have dynamic `this`.
- Arrow functions have lexical `this`.
Best Practices:
- Use arrow functions for callbacks to avoid losing context.
- Use regular functions when you need dynamic `this`.
Managing Scope with Immediately Invoked Function Expressions (IIFEs)
Immediate Invoked Function Expressions (IIFEs) are important for controlling scope and avoiding polluting the global namespace. An IIFE is a function that is run immediately after being declared.
(function() {
const message = “Hello, World!”;
console.log(message); // Accessible here
})();
console.log(message); // Error: message is not defined
Use Cases:
- Encapsulating code.
- Avoiding global variable pollution.
Understanding and Using Arrow Functions
Arrow functions, introduced in ECMAScript 6 (ES6), provide a concise and modern way to write function expressions. They enable developers to write more understandable code with a shorter syntax than typical function expressions. One of the most important aspects of arrow functions is that they do not have their own `this` context; instead, they inherit `this` from the surrounding lexical context. This characteristic makes arrow functions particularly useful for certain cases, like array methods and event handlers, where dealing with `this` can be cumbersome.
javascript
const add = (a, b) => a + b;
console.log(add(2, 3)); // Outputs: 5
const object = {
name: “Arrow”,
sayHello: function() {
setTimeout(() => {
console.log(`Hello, my name is ${this.name}`); // `this` refers to `obj`
}, 1000);
}
};
obj.sayHello(); // Outputs: Hello, my name is Arrow
Advantages:
- Simpler syntax: Makes the code less verbose.
- Lexical `this`: Avoids common pitfalls of the traditional `this` behavior.
Best Practices:
- Use arrow functions for callbacks and simple operations.
- Avoid using arrow functions for methods that need a dynamic `this`.
Key Points:
- Executes immediately.
- Encapsulates variables.
Best Practices for Scope Management
Managing scope effectively is crucial for writing maintainable and bug-free code. Here are some excellent practices to consider:
Minimize Global Variables:
- Avoid using global variables whenever possible. They can lead to conflicts and are harder to maintain.
- Use `const` and `let`:
- Always prefer `const` for variables that don’t change and `let` for those that do. This helps prevent accidental modifications.
- Leverage Closures:
- Use closures to construct private variables and functions, which improves data privacy and security.
Advanced Function Patterns
Once you’re comfortable with the basics, you can explore more advanced function patterns to enhance your code even further. Some of these include currying, partial application, and higher-order functions.
Currying:
- Transforming a function with numerous parameters into a succession of functions, each with a single argument.
Partial Application:
- Creating a new function by pre-filling part of the arguments of an existing function.
Higher-Order Functions:
- Functions that accept other functions as parameters and return functions as results.
Conclusion
Understanding JS JavaScript functions and scope is essential for any web developer. These concepts form the backbone of efficient, maintainable, and scalable code. By mastering function declarations, function expressions, arrow functions, and various scopes, you can write cleaner and more effectiveJS JavaScript.
JS JavaScript is a powerful language, and with the right knowledge, you can leverage its full potential to build amazing applications. Whether you’re a beginner or an experienced developer, there’s always something new to learn JS JavaScript.
Are you ready to take your JS JavaScript abilities to the next level? Explore our additional resources and tutorials to deepen your understanding and stay ahead in your programming journey.