Introduction to JS JavaScript Data Structures
Ever wondered how JS JavaScript handles and organizes data? Comprehending data structures, such as arrays and objects, is essential for effective data management and manipulation in web development. Whether you’re a beginner just starting or seasoned developer looking to polish your skills, this guide will take you through the ins and outs of JS JavaScript arrays and objects. By the end, you’ll have a solid grasp of these fundamental concepts, making your coding more effective and your applications more dynamic.
Arrays and Objects Overview
Arrays and objects are the two main data structures that JS JavaScript provides for arranging and storing data. Both have unique characteristics and are suitable for different use cases. Arrays are ordered collections of items, while objects are collections of key-value pairs. Each offers methods and functionalities, making them versatile tools in your programming toolkit. In this guide, we’ll explore the properties and methods of arrays and objects, and how to use them effectively in your code.
Understanding Arrays
One of the most utilized data structures in JS JavaScript is an array. They allow you to store values under a single variable name. These values can be any type, including strings, numbers, booleans, or other arrays or objects. You can think of arrays as a list that keeps track of items’ order, making it easy to access and manipulate specific elements.
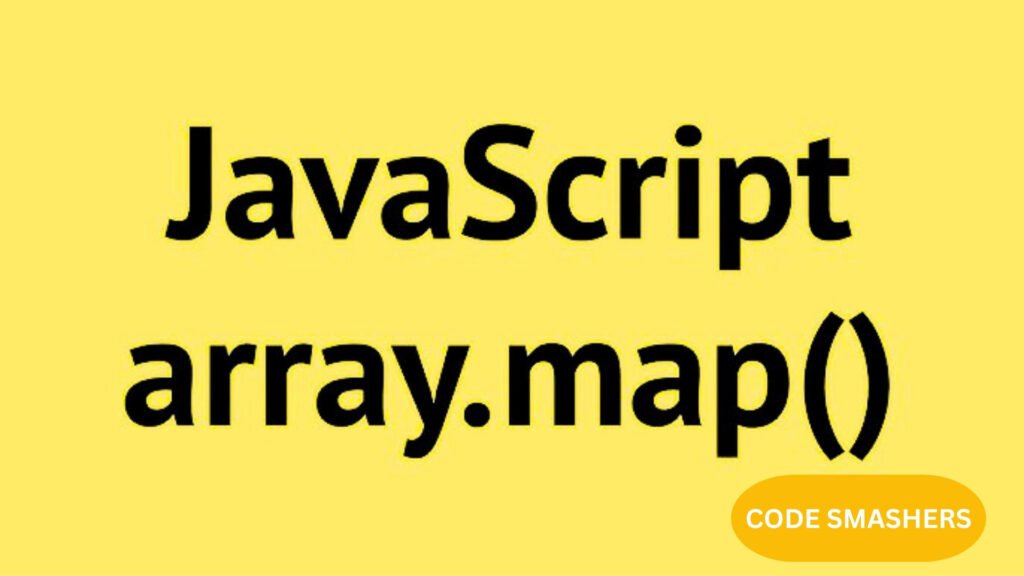
Understanding JS JavaScript Arrays
Basic Syntax and Initialization
Arrays in JS JavaScript are straightforward to create. Square brackets {[]{ can be used to declare and initialize arrays. For example:
const fruits = [‘Apple’, ‘Banana’, ‘Cherry’];
This simple line of code creates an array named `fruits` containing three elements. Arrays can store any data, including numbers, strings, and even other arrays.
Accessing and Modifying Array Elements
Indexing and Assignment
An array’s elements can be accessed via zero-based indexing. The first element has an index of `0`, the second `1`, and so on. This is how you can access and change elements in an array:
console.log(fruits[0]); // Output: Apple
fruits[1] = ‘Blueberry’;
console.log(fruits); // Output: [‘Apple’, ‘Blueberry’, ‘Cherry’]
Indexing allows quick access to items and assignments to enable straightforward updates.
Array Methods: An Introduction
Overview of Commonly Used Methods
JS JavaScript provides various methods to manipulate arrays. Among the most widely utilized ones are the following:
- push(): extends an array’s end by one or more elements.
- pop(): Removes the last element present in an array.
- shift(): Removes the first present in an array.
- unshift(): Adds one or more elements to the start in an array.
These methods allow you to dynamically modify the array, adapting to changing data requirements.
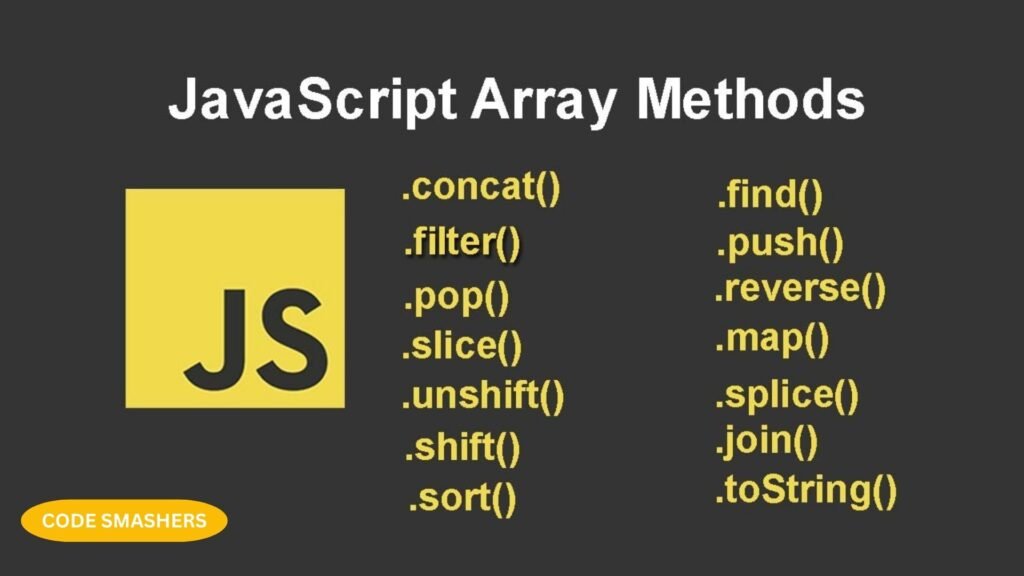
Exploring Array Iteration Methods
forEach, map, filter, and reduce
Iteration methods are powerful tools for processing array elements. Here are four essential iteration methods:
- forEach(): performs a given function once for every element in the array.
fruits.forEach(fruit => console.log(fruit));
- map(): calls a supplied function on each element to create a new array filled with the results.
const upperFruits = fruits.map(fruit => fruit.toUpperCase());
- filter(): builds a new array containing every element that passes the test carried out by the given function.
const berryFruits = fruits.filter(fruit => fruit.includes(‘berry’));
- reduce(): produces a single output value by applying a reducer function to each element of the array.
const totalLength = fruits.reduce((total, fruit) => total + fruit.length, 0);
These methods enable efficient and readable array processing, making your code cleaner and more functional.
Advanced Array Methods
Find, some, every, and sort
Advanced array methods offer even more flexibility:
- find(): gives back the first element that fulfills the testing function that has been supplied.
const foundFruit = fruits.find(fruit => fruit === ‘Cherry’);
- some(): determines if the array’s minimum number of elements pass the test.
const hasBerry = fruits.some(fruit => fruit.includes(‘berry’));
- every(): check to see if each entry in the array passes the condition.
const allHaveFiveLetters = fruits.every(fruit => fruit.length === 5);
- sort(): returns the sorted array after sorting the items of the array in place.
fruits.sort();
With these methods, you can perform complex operations on arrays with minimal code.
The Role of Multidimensional Arrays
Creating and Manipulating Nested Arrays
Multidimensional arrays are arrays of arrays. They are useful for representing more complex data structures, like matrices or grids.
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
You can access nested elements using multiple indices:
console.log(matrix[0][1]); // Output: 2
Manipulating nested arrays involves similar methods to those used with single-dimensional arrays, allowing you to perform intricate data operations.
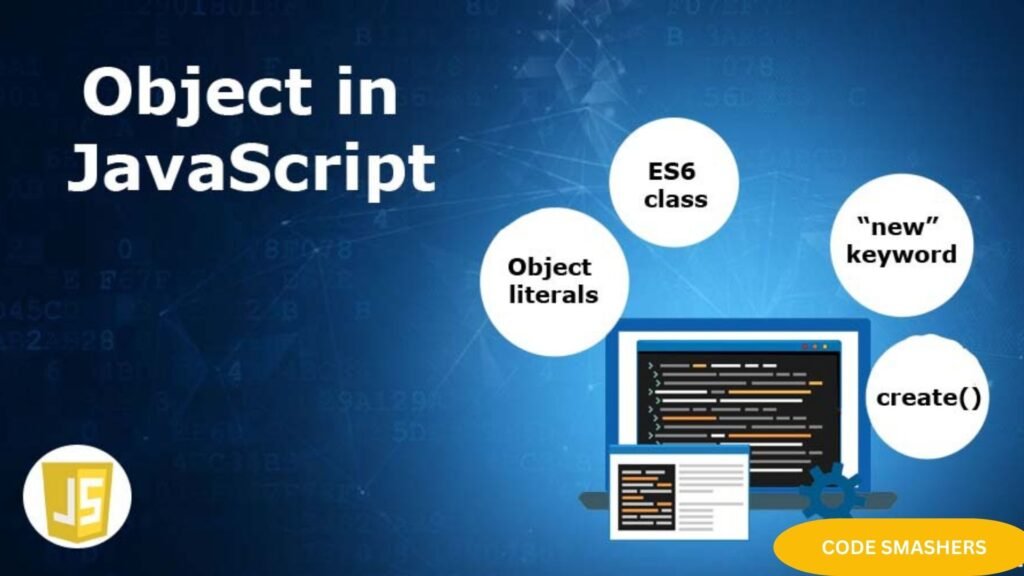
Introduction to JS JavaScript Objects
Object Syntax and Initialization
Objects in JS JavaScript are collections of key-value pairs. You can use curly braces {{}} to create an object:
const person = {
name: ‘John’,
age: 30,
job: ‘Developer’
};
Each property in the object (`name`, `age`, `job`) is a key with an associated value.
Defining and Accessing Object Properties
Dot Notation vs. Bracket Notation
Dot notation or bracket notation can be used to access object properties:
console.log(person.name); // Output: John
console.log(person[‘age’]); // Output: 30
Dot notation is generally preferred for its readability, but bracket notation allows the use of variables as keys.
Adding and Modifying Object Properties
Dynamic Property Assignment
You can add or modify properties dynamically:
person.location = ‘New York’;
console.log(person.location); // Output: New York
This flexibility allows objects to adapt to new information as needed.
Understanding Methods in Objects
Defining and Calling Methods
Methods are functions stored as object properties. They can be defined and called like this:
person.greet = function() {
return `Hello, my name is ${this.name}`;
};
console.log(person.greet()); // Output: Hello, my name is John
Methods encapsulate functionality relevant to the object, making code more modular and reusable.
Key-value Pairs and Property Descriptors
Using Object.keys, Object.values, and Object.entries
JS JavaScript provides methods to interact with object properties:
- Object.keys(): Returns an array of the object’s keys.
- Object.values(): Returns an array of the object’s values.
- Object.entries(): Returns an array of key-value pairs.
console.log(Object.keys(person)); // Output: [‘name’, ‘age’, ‘job’, ‘location’]
console.log(Object.values(person)); // Output: [‘John’, 30, ‘Developer’, ‘New York’]
console.log(Object.entries(person)); // Output: [[‘name’, ‘John’], [‘age’, 30], [‘job’, ‘Developer’], [‘location’, ‘New York’]]
These methods are invaluable for iterating over and manipulating object properties.
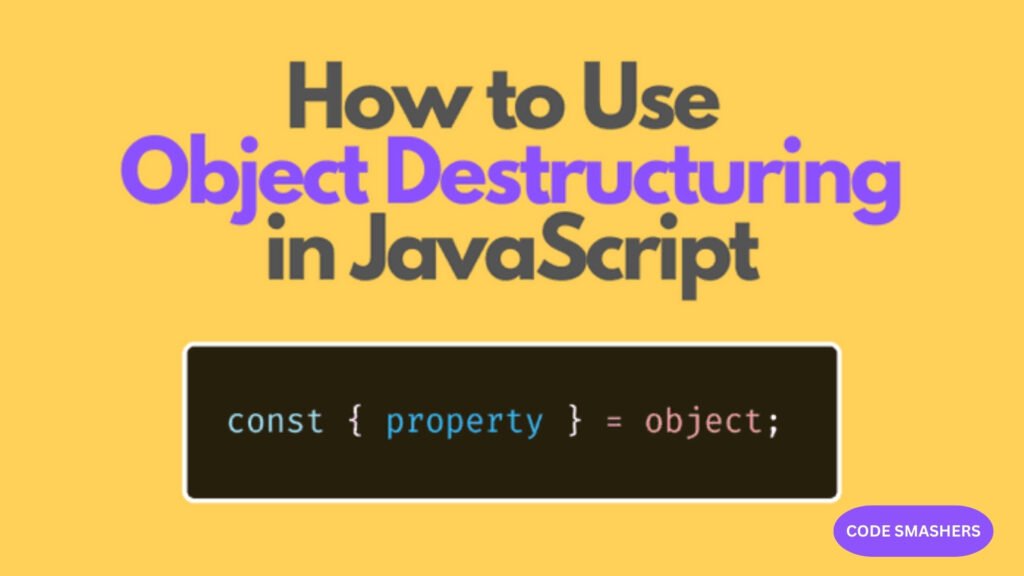
Object Destructuring
Extracting Properties with Ease
Destructuring allows you to extract properties from objects into variables:
const {name, job} = person;
console.log(name); // Output: John
console.log(job); // Output: Developer
This syntax makes it easier to work with object data.
The Role of Prototypes in Objects
Prototype Inheritance and Extending Objects
Prototypes allow objects to inherit properties and methods from other objects.
const animal = {
eats: true
};
const rabbit = Object.create(animal);
rabbit.jumps = true;
console.log(rabbit.eats); // Output: true
Understanding prototypes is crucial for mastering JS JavaScript’s inheritance model.
Comparing Arrays and Objects
When to Use Each Data Structure
Arrays and objects serve different purposes:
- Arrays are ideal for ordered collections of items.
- Objects are better for collections of key-value pairs with unique identifiers.
Choosing the right structure depends on your data and how you plan to manipulate it.
Handling Nested Objects and Arrays
Accessing and Modifying Deep Structures
Nested objects and arrays can be accessed and modified using a combination of dot and bracket notation:
const user = {
name: ‘Jane’,
posts: [
{title: ‘Post 1’, likes: 10},
{title: ‘Post 2’, likes: 20}
]
};
console.log(user.posts[0].title); // Output: Post 1
This approach allows you to manage complex data hierarchies effectively.
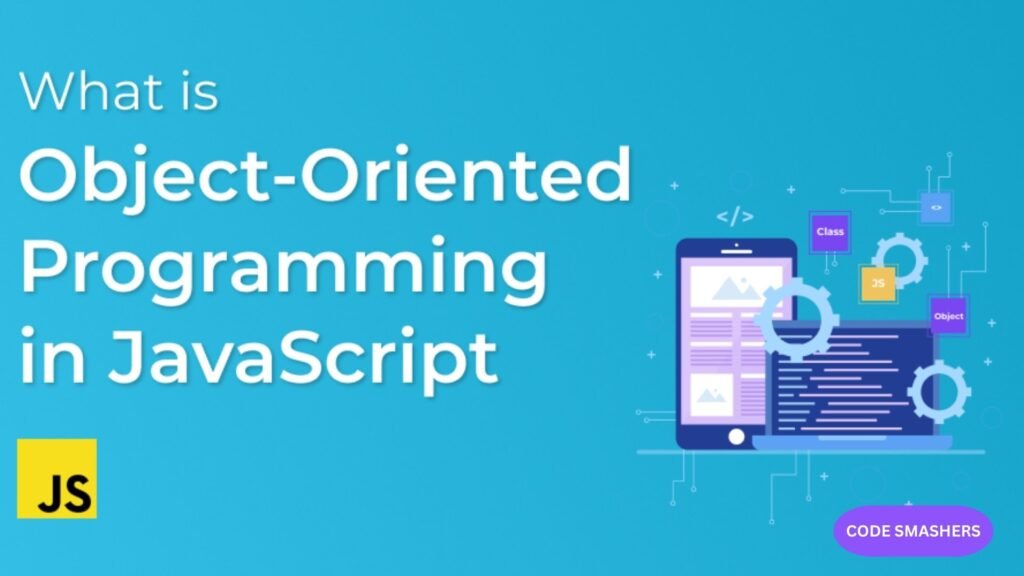
Object-Oriented Programming with Objects
Creating and Using Classes
Classes provide a blueprint for creating objects with shared properties and methods:
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
drive() {
return `${this.make} ${this.model} is driving.`;
}
}
const myCar = new Car(‘Toyota’, ‘Corolla’);
console.log(myCar.drive()); // Output: Toyota Corolla is driving.
Classes simplify object creation and promote code reuse.
Array and Object Performance Considerations
Optimizing Data Access and Manipulation
Performance is crucial in large applications. Here are some tips:
- Use arrays for ordered data and frequent iteration.
- Utilize objects with distinct keys for quicker lookups.
- Optimize large data sets by minimizing unnecessary copying and deep nesting.
Best Practices for Working with Arrays and Objects
Coding Standards and Common Pitfalls
Adhering to best practices ensures clean and maintainable code.
- Use clear, descriptive variables and property names.
- Avoid deep nesting to reduce complexity.
- Regularly refactor code to improve performance and readability.
Summary of Benefits
Arrays and objects are foundational data structures in JS JavaScript, each offering distinct advantages tailored to specific use cases. Arrays are ideal for managing ordered collections of elements, allowing efficient access and manipulation through indexed positions. They are perfect for tasks that require iterations or sequence-based processing. On the other hand, objects excel in handling collections of key-value pairs, enabling rapid data lookup and organization through descriptive keys. This makes objects highly versatile for modeling complex data structures, supporting dynamic property assignments, and encapsulating functionalities within methods. Together, arrays and objects provide robust tools for organizing and processing data, each offering unique strengths that enhance the flexibility and efficiency of JS JavaScript programming.
Real-World Applications of Arrays and Objects
Practical Examples and Use Cases
Arrays and objects shine in real-world scenarios:
- E-commerce sites use arrays to manage product inventories, with each product represented as an object.
- To-do list applications leverage arrays to add, remove, and update tasks dynamically.
- Social media platforms use objects to represent user profiles and interactions, storing properties like names and followers and methods like posting and liking.
Conclusion
Mastering JS JavaScript arrays and objects opens up a world of possibilities for developers. Whether managing data in a to-do app or implementing complex e-commerce functionalities, these structures are invaluable. By understanding their properties, methods, and best practices, you can write more efficient, scalable, and maintainable code. Ready to deepen your knowledge? Explore more JS JavaScript tutorials and start experimenting with your projects today. Happy coding!