Introduction to Loops and Conditionals in JS Javascript
JS Javascript is fundamental to web development, providing dynamic functionality that enhances user experience. Two core concepts integral to mastering JS Javascript are loops and conditionals. Understanding these control flow mechanisms will empower you to write more efficient, readable, and maintainable code. This guide will walk you through the basics and best practices of loops and conditionals, ensuring you have a solid foundation to build upon. By the end, you’ll know how to apply these principles to real-world scenarios, optimizing your code’s performance and readability.
Overview of Control Flow Mechanisms
Control flow mechanisms determine the order in which statements are executed in a program. In JS Javascript, loops and conditionals are pivotal control structures. Loops allow you to repeat a block of code multiple times, while conditionals enable you to execute code based on specific conditions. Together, they offer robust tools for handling various programming tasks, from simple iterations to complex decision-making processes.
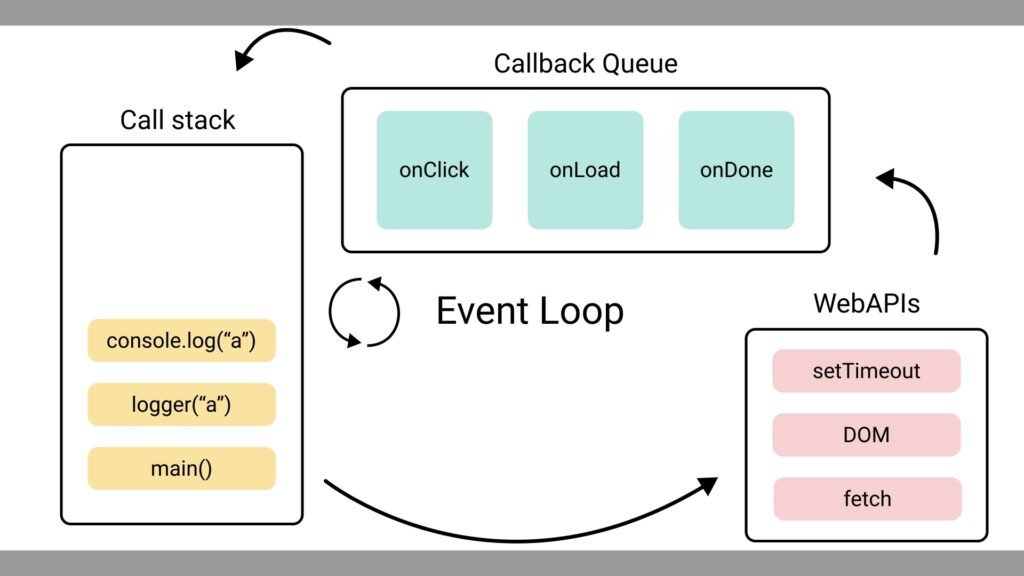
Understanding the for Loop
The `for` loop is one of the most versatile looping constructs in JS Javascript. JS Javascript syntax is straightforward:
for (initialization; condition; increment) {
// Code to be executed
}
The `for` loop is ideal for scenarios where you know in advance how many times you need to execute a statement or block of statements. One frequent use case is to process each element of an array by iterating over it.
Syntax, Use Cases, and Variations
The basic syntax of a `for` loop includes initialization, a condition, and an increment expression. You can also create variations like nested `for` loops for multidimensional array processing. Here’s a simple example:
for (let i = 0; i < 5; i++) {
console.log(i);
}
This loop logs numbers 0 to 4 to the console. Understanding these variations will help you tackle more complex problems efficiently.
Using the while Loop
The `while` loop continues executing its block as long as the specified condition evaluates to true. Its syntax is:
while (condition) {
// Code to be executed
}
Syntax, Common Pitfalls, and Best Practices
When there is no set amount of iterations, the `while` loop comes in handy. However, it comes with pitfalls like infinite loops if the condition never becomes false. Make sure there is always a termination scenario in your loop. As an illustration, consider this:
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
This loop also logs numbers 0 to 4 to the console. Employing best practices, such as clear and concise termination conditions, will help you avoid common pitfalls.
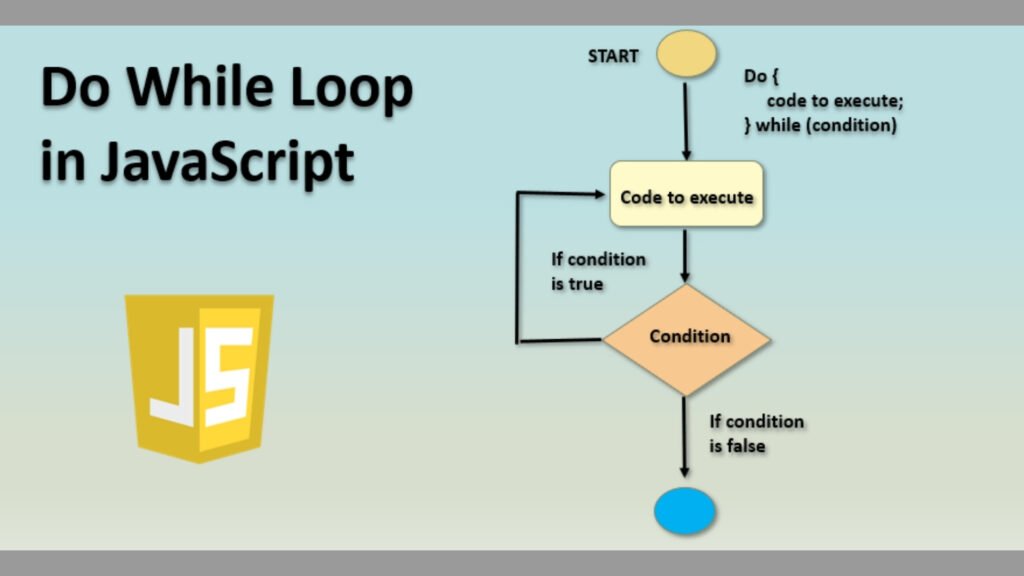
The do-while Loop A Unique Approach
Similar to the `while` loop, the `do-while` loop runs its block at least once before verifying the condition. Its syntax is:
do {
// Code to be executed
} while (condition);
Syntax and Practical Applications
The `do-while` loop is beneficial when you need to ensure the block is executed at least once, regardless of the condition. For example:
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
This loop logs numbers 0 to 4 to the console, demonstrating its unique capability.
Comparing Loop Types for while and do-while
Choosing the right loop type depends on your specific requirements. The `for` loop is perfect for known iteration counts, while `while` and `do-while` loops are better for scenarios where the number of iterations is uncertain.
When to Use Each Type
Understanding when to use each loop type is crucial for writing efficient code. Use `for` loops for definite iteration counts, `while` loops for indefinite ones, and `do-while` loops when the block must execute at least once.
Nested Loops Techniques and Use Cases
Nested loops involve placing one loop inside another, enabling you to handle more complex data structures like multidimensional arrays.
How to Work with Multiple Loops
Working with nested loops requires careful consideration to avoid performance issues. For example:
for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
console.log(`i = ${i}, j = ${j}`);
}
}
This loop logs all combinations of `i` and `j` from 0 to 2. Understanding proper nesting techniques will help you manage complex data efficiently.
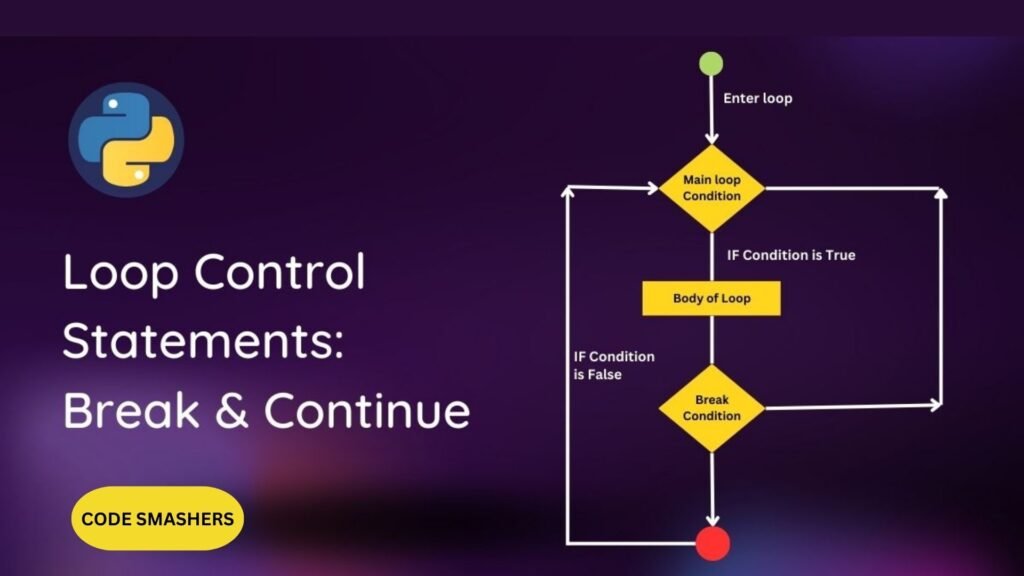
Loop Control Statements break and continue
Control statements like `break` and `continue` allow you to manage your loop’s execution flow. While `continue` skips this iteration and moves on to the next, the `break` statement ends the loop completely.
Managing Loop Execution Flow
Using these statements effectively can optimize your loop’s performance. For example:
for (let i = 0; i < 5; i++) {
if (i === 3) break;
console.log(i);
}
This loop logs numbers 0 to 2, demonstrating the use of `break`.
Iterating Over Arrays with Loops
Loops are essential for processing array data. Whether you’re using `for`, `while`, or `do-while` loops, iterating over arrays allows you to manipulate data efficiently.
Using Loops to Process Array Data
Processing array data is a common task in web development. For example:
const array = [1, 2, 3, 4, 5];
for (let i = 0; i < array.length; i++) {
console.log(array[i]);
}
This loop demonstrates the use of loops in data processing by logging each element of the array.
Introduction to Conditional Statements
The `if`, {else if{, and `else} statements are essential for managing the flow of your program. Conditional statements allow you to run code based on predetermined circumstances.
The Role of if else if and else
The `if` statement checks a condition and executes its block if the condition is true. The `else if` and `else` statements provide additional layers of condition checking.
Using switch Statements for Multiple Conditions
The `switch` statement is a handy tool for managing numerous circumstances. After evaluating an expression, the associated case block is carried out.
Syntax, Examples, and Best Practices
The `switch` statement’s syntax is:
switch (expression) {
case value1:
// Code to be executed
break;
case value2:
// Code to be executed
break;
default:
// Code to be executed
}
Using `switch` statements improves code readability and efficiency when dealing with multiple conditions.
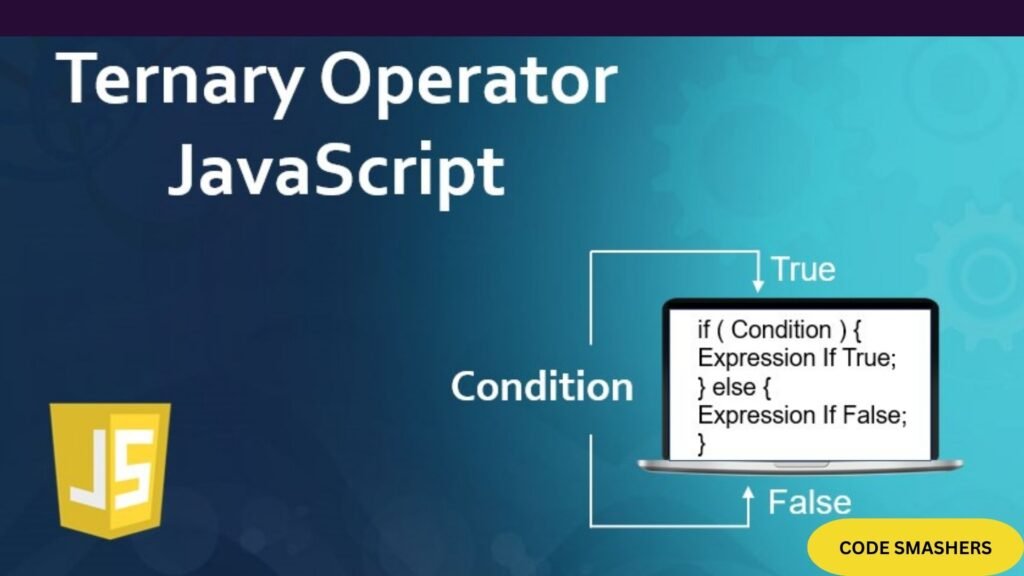
Understanding the Ternary Operator
Using the ternary operator, you may quickly write `if-else` sentences. Its syntax is:
condition ? expression1 : expression2;
Simplifying Conditional Logic
The ternary operator simplifies conditional logic, making your code more concise. For example:
const result = (a > b) ? ‘a is greater’ : ‘b is greater’;
This statement assigns ‘a is greater’ to `result` if `a` is greater than `b`, otherwise ‘b is greater’.
Conditional Statements and Boolean Logic
Combining conditions with logical operators (`&&`, `||`, `!`) enhances your code’s decision-making capabilities. You are able to construct intricate conditional statements with these operators.
Combining Conditions with Logical Operators
Logical operators enable you to combine multiple conditions efficiently. For example:
if (a > b && a > c) {
console.log(‘a is the greatest’);
}
This statement checks if `a` is greater than both `b` and `c`, enhancing your conditional logic.
Advanced Conditional Patterns
Advanced conditional patterns involve using `if` statements with complex conditions, such as nested conditions or combining multiple logical operators.
Using if Statements with Complex Conditions
Handling complex conditions requires careful structuring of your `if` statements. For example:
if ((a > b && b > c) || (a === b && b === c)) {
console.log(‘conditions met’);
}
This statement checks multiple conditions, demonstrating advanced conditional logic.
Looping with Objects and Arrays
Looping through objects and arrays allows you to manipulate their properties and elements efficiently. Techniques like `for…in` and `for…of` loops are essential for this task.
Iterating Over Properties and Elements
Iterating over object properties and array elements is crucial for data manipulation. For example:
const obj = {a: 1, b: 2, c: 3};
for (const key in obj) {
console.log(`${key}: ${obj[key]}`);
}
This loop demonstrates efficient iteration techniques by logging every property and value of the object.
Performance Considerations for Loops
Optimizing loop performance is crucial for efficient code execution. Factors like loop complexity and the number of iterations impact performance.
Optimizing Loop Efficiency
Optimizing loop efficiency involves minimizing unnecessary iterations and reducing computational complexity. For example:
const array = [1, 2, 3, 4, 5];
for (const value of array) {
console.log(value);
}
This loop uses the `for…of` statement, which is more efficient for iterating over array elements.
Error Handling in Loops and Conditionals
Managing unexpected scenarios in loops and conditionals ensures your code runs smoothly. Techniques like try-catch blocks help handle errors gracefully.
Managing Unexpected Scenarios
Handling errors in loops and conditionals involves anticipating potential issues and providing fallback solutions. For example:
try {
for (const value of array) {
if (value === undefined) throw ‘Value is undefined’;
console.log(value);
}
} catch (error) {
console.error(error);
}
This code handles errors in the loop, demonstrating effective error management.
Common Pitfalls and How to Avoid Them
Avoiding common pitfalls in loops and conditionals ensures your code is robust and maintainable. Issues like infinite loops and incorrect condition checks can lead to bugs.
Debugging Issues in Loops and Conditionals
Debugging issues involve identifying and resolving problems in your loops and conditionals. Tools like console logs and debugging environments aid this process.
Real-World Examples of Loops and Conditionals
Applying loops and conditionals to real-world scenarios showcases their practical utility. Examples include data processing, user interactions, and algorithm implementations.
Practical Applications in JS Javascript
Practical applications of loops and conditionals demonstrate their versatility. For example:
const users = [
{name: ‘Alice’, age: 25},
{name: ‘Bob’, age: 30},
{name: ‘Charlie’, age: 35}
];
for (const user of users) {
if (user.age > 30) console.log(`${user.name} is over 30`);
}
This loop processes user data, highlighting practical use cases.
Best Practices for Writing Efficient Loops and Conditionals
Following best practices ensures your loops and conditionals are efficient and maintainable. Concise conditions, little nesting, and obvious variable naming are some tips.
Tips for Clean and Maintainable Code
Following best practices is essential to writing code that is readable and maintainable. For example:
const isAdult = (age) => age >= 18 ? ‘Adult’ : ‘Minor’;
console.log(isAdult(20));
This example demonstrates concise and readable code.
Future Trends and Alternatives to Traditional Loops
Exploring new approaches in modern JS Javascript reveals alternatives to traditional loops, such as higher-order functions like `map`, `filter`, and `reduce`.
Exploring New Approaches in Modern JS Javascript
Modern JS Javascript offers new approaches to looping and conditional logic. For example:
const array = [1, 2, 3, 4, 5];
const doubled = array.map(value => value * 2);
console.log(doubled);
This example demonstrates the use of `map`, highlighting future trends in JS Javascript.
Conclusion
Mastering loops and conditionals in JS Javascript is essential for writing efficient, readable, and maintainable code. By understanding these control flow mechanisms, you can tackle a wide range of programming tasks with confidence. Whether you’re a novice or an intermediate learner, the principles and best practices discussed in this guide will enhance your coding skills, enabling you to create more robust and efficient applications.
Are you prepared to advance your JS Javascript knowledge? Start applying these concepts in your projects today and watch your coding proficiency soar. Happy coding!