JS JavaScript can be a quirky language with unique features that distinguish it from other programming languages. One of the core concepts every JavaScript developer should understand is prototypes and inheritance. If you’ve scratched your head over these topics, this blog post is for you.
Understanding JS JavaScript Prototypes and Inheritance
In this blog post, we’ll demystify JS JavaScript prototypes and inheritance. By the end, you’ll have a clear understanding of how they work, why they’re useful, and how to leverage them in your projects. Let’s get started!
Introduction to JS JavaScript Prototypes
What Are Prototypes?
In JS JavaScript, every object has an internal property called `[[Prototype]]`. This property is either `null` or references another object, which is known as its prototype. Prototypes are fundamental to how JavaScript objects inherit properties and methods from one another.
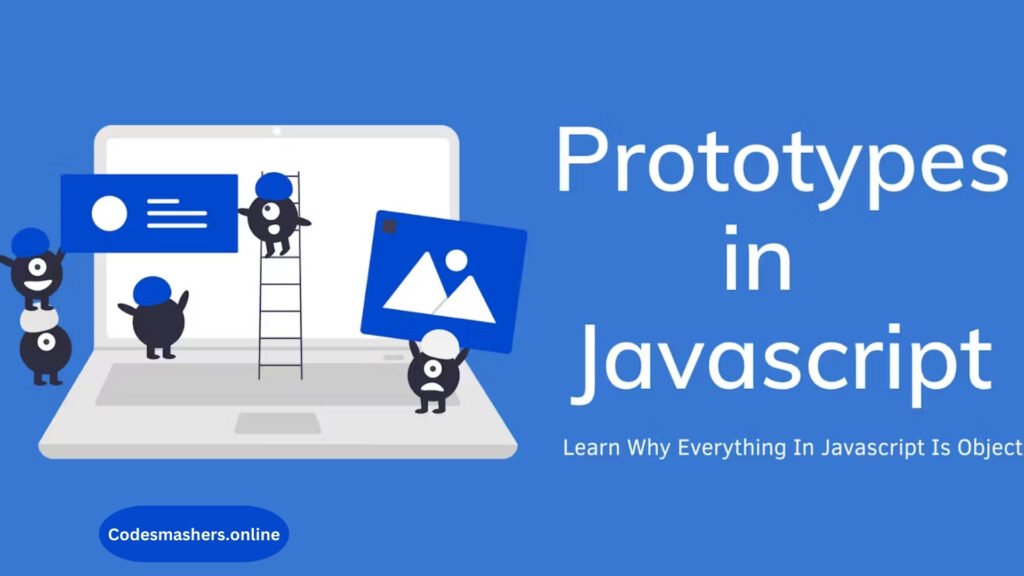
The Role of Prototypes in JS JavaScript
Prototypes enable JS JavaScriptto implement inheritance. When you access a property or method on an object, JS JavaScript looks for it on the object itself. If it’s not found, JavaScript follows the `[[Prototype]]` chain to see it. This mechanism allows objects to share behavior (methods) efficiently.
Understanding Prototype Inheritance
How Inheritance Works in JS JavaScript
In JavaScript, inheritance is prototype-based. This means that objects can inherit from other objects directly. Unlike classical inheritance, where classes inherit from different classes, JavaScript uses prototypes to share properties and methods among objects.
Prototype Chain Basics
The prototype chain is a series of linked objects. When JS JavaScript can’t find a property on an object, it looks up the prototype chain until it finds the property or reaches the end of the chain (where the `[[Prototype]]` is `null`).
The Prototype Chain Explained
How JS JavaScript Looks Up Properties
When you try to access a property on an object, JS JavaScript looks for the property in the following order:
- On the object itself.
- On the object’s prototype.
- On the prototype’s prototype, and so on, until it reaches `null`.
Navigating the Prototype Chain
Understanding how to traverse the prototype chain is crucial for debugging and writing efficient code. The `Object.getPrototypeOf` method allows you to inspect the prototype of an object, helping you understand the inheritance structure.
Creating and Modifying Prototypes
Adding Methods and Properties to Prototypes
You can add methods and properties to an object’s prototype to share them across all instances. For example, adding a method to a constructor’s prototype ensures that all instances created with that constructor can access the method.
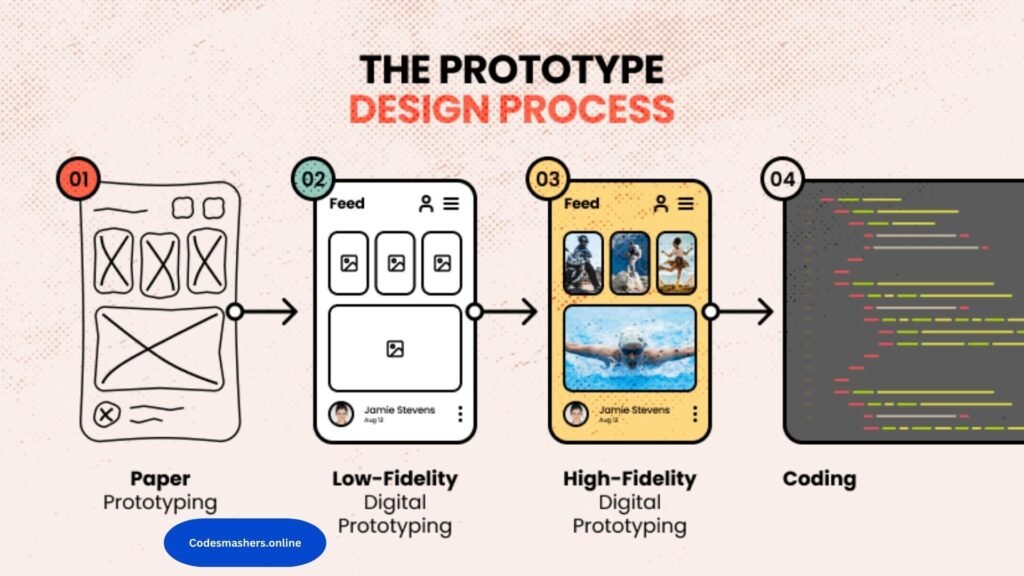
Using `Object.create()` to Set Prototypes
The `Object. Create ()` method creates a new object with the specified prototype object and properties. It’s a powerful way to create objects with a predefined prototype, allowing for more flexible inheritance patterns.
Constructor Functions and Prototypes
How Constructors and Prototypes Work Together
Constructor functions in JS JavaScript work with prototypes to enable object creation and inheritance. When you create an object using a constructor function, the object’s prototype is set to the constructor’s `prototype` property.
Example: Adding Methods to Construction Prototypes
By adding methods to a constructor’s `prototype`, you can ensure that all of its instances share the same ways. This approach conserves memory and improves code organization.
Prototype vs. Instance Properties
Differences Between Prototype and Instance Properties
Instance properties are properties defined directly on an object, while prototype properties are shared among all instances of an object. Understanding the distinction helps in managing data and behavior efficiently.
Managing Prototype and Instance Data
Balancing prototype and instance properties is key to effective JS JavaScript programming. Use prototype properties for shared behavior and instance properties for unique data specific to each object.
The `Object.prototype` and Its Importance
Overview of `Object. prototype`
`Object.prototype` is the root of all objects in JS JavaScript. It’s the final link in the prototype chain, providing basic methods like `toString` and `hasOwnProperty`.
Modifying the Base Prototype Object
While it’s possible to modify `Object.prototype`, it’s generally discouraged. Changes to `Object.prototype` affect all objects, potentially leading to unexpected behavior and difficult-to-debug issues.
Inheritance Patterns in JS JavaScript
Classical vs. Prototypal Inheritance
Classical inheritance, common in many programming languages, uses classes to define and inherit behavior. JS JavaScript prototypical inheritance, on the other hand, allows objects to inherit directly from other objects, offering more flexibility.
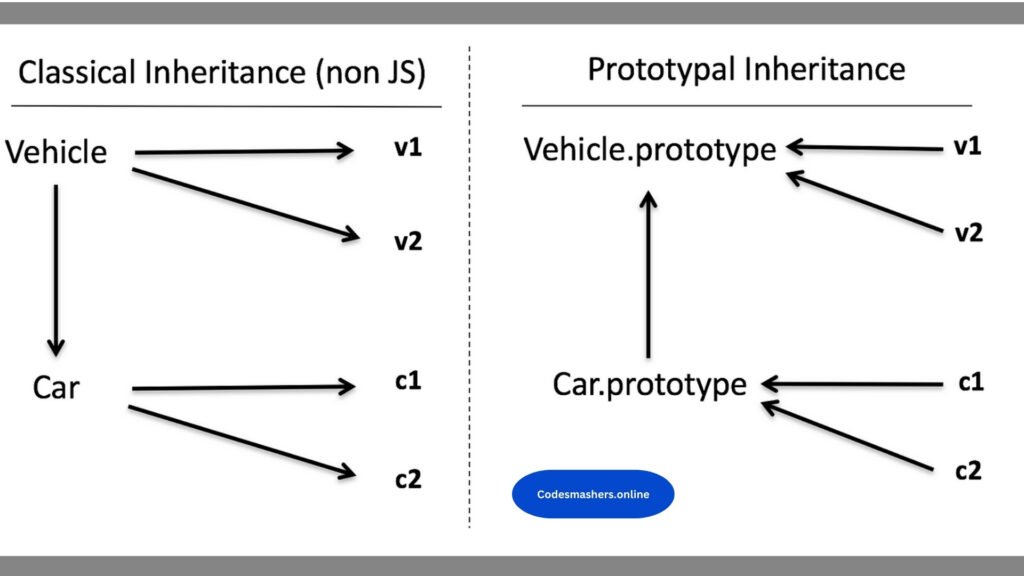
Implementing Inheritance with Prototypes
Implementing inheritance with prototypes involves setting up the prototype chain correctly. By using constructor functions and setting prototypes, you can create a robust inheritance structure.
Using ES6 Classes for Inheritance
Introduction to ES6 Class Syntax
ES6 introduced the `class` syntax, which provides a more familiar way to define and inherit from classes. Although it’s syntactic sugar over prototypes, it simplifies the syntax and makes the code more readable.
How Classes Simplify Prototype-Based Inheritance
Classes in ES6 make it easier to define and manage inheritance. The `extends` keyword allows one class to inherit from another, and the `super` keyword enables calling methods from the parent class.
Class Inheritance and Method Overriding
Extending Classes and Overriding Methods
ES6 classes support method overriding, allowing subclasses to redefine methods inherited from parent classes. This feature is essential for customizing behavior in different parts of an application.
Super Calls and Constructor Inheritance
The `super` keyword in ES6 enables calling methods from the parent class, including the constructor. This functionality is crucial for initializing subclasses and extending parent class behavior.
Understanding the `Object.create()` Method
Creating Objects with a Custom Prototype
`Object.create()` allows you to create objects with a custom prototype, providing more control over the inheritance structure. This method is suitable for creating object hierarchies and sharing behavior.
Practical Uses of `Object. create()`
Using `Object.create()`, you can create objects with shared prototypes, enabling more efficient inheritance patterns. This method is ideal for scenarios where multiple objects with a common prototype are required.
Prototypes and Performance Considerations
Impact of Prototype Chain on Performance
The prototype chain can impact performance, especially when dealing with deep inheritance hierarchies. Understanding how property lookup works can help you optimize your code for better performance.
Optimizing Prototype-Based Inheritance
To optimize prototype-based inheritance, minimize the depth of the prototype chain and use caching techniques. These strategies can significantly improve the performance of your JS JavaScript applications.
Prototype Inheritance and Property Lookup
How Property Lookup Works in the Prototype Chain
Property lookup in the prototype chain involves searching each prototype until the desired property is found or the chain ends. Knowing how this process works can help you design more efficient inheritance structures.
Avoiding Common Pitfalls in Property Lookup
Common pitfalls in property lookup include unintentionally shadowing properties and creating circular references. Being aware of these issues helps you write more robust and maintainable code.
Inheritance with Factory Functions
Creating Inheritance Patterns Using Factory Functions
Factory functions offer an alternative to constructor functions for creating objects and implementing inheritance. They provide more flexibility and can simplify the creation of complex inheritance structures.
Advantages and Limitations of Factory Functions
Factory functions offer several advantages, including better encapsulation and easier testing. However, they may also have limitations, such as increased memory usage compared to constructor functions.
Mixins and Multiple Inheritance
Implementing Mixins in JS JavaScript
Mixins allow you to combine multiple objects’ behaviors, enabling a form of multiple inheritance. They provide a way to share functionalities across different objects without using the prototype chain.
Combining Multiple Inheritance Patterns
Combining mixins and prototypes allows you to create flexible and reusable inheritance structures. This approach is suitable for large applications with diverse requirements.
Extending Built-In Objects
Adding Methods to Built-In Objects Like Arrays and Objects
Extending built-in objects, such as `Array` and `Object`, allows you to add custom methods and behaviors. This technique can enhance the functionality of standard objects for specific use cases.
Best Practices for Extending Native Prototypes
While extending native prototypes can be powerful, it should be done cautiously. Follow best practices to avoid conflicts with existing properties and methods, ensuring compatibility with other code.
Prototype Inheritance in Asynchronous Code
Managing Inheritance in Asynchronous Scenarios
Managing inheritance in asynchronous scenarios requires careful planning. Ensure that asynchronous operations do not interfere with the expected inheritance structure and property access.
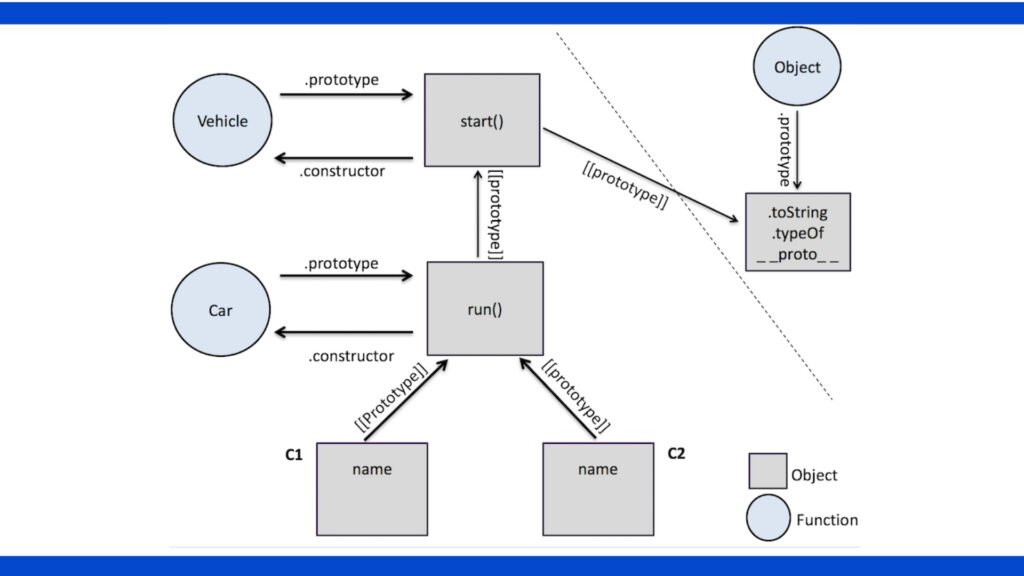
Common Issues and Solutions
Common issues in asynchronous code include race conditions and inconsistent states. Understanding how to manage these issues helps you write robust and reliable asynchronous JS JavaScript applications.
Debugging Prototype and Inheritance Issues
Techniques for Identifying and Fixing Problems
Debugging prototype and inheritance issues often involves inspecting the prototype chain and property access. Use tools like `console.log` and browser developer tools to identify and resolve problems.
Tools and Strategies for Debugging Prototypes
Several tools and strategies can help you debug prototype-related issues. Tools like Chrome DevTools and libraries like `debug` provide insights into the prototype chain and property access.
Case Studies: Real-World Uses of Prototypes
Examples of Prototype-Based Inheritance in Modern JavaScript
Real-world examples of prototype-based inheritance illustrate how these concepts are applied in practice. Studying these examples helps you understand the benefits and challenges of using prototypes.
Lessons Learned from Practical Applications
Lessons learned from practical applications provide valuable insights into designing and implementing prototype-based inheritance. These lessons can guide you in creating efficient and maintainable code.
Future Trends and Evolving Concepts
Emerging Trends in Prototype and Inheritance Patterns
Emerging trends in prototype and inheritance patterns reflect the evolving nature of JavaScript development. Staying informed about these trends helps you anticipate changes and adapt your code accordingly.
Predictions for the Evolution of Prototypal Inheritance in JS JavaScript
Predictions for the evolution of prototypal inheritance provide insights into where JS JavaScript might be headed. Understanding these predictions helps you prepare for future developments in the language.
Conclusion
Empowering JavaScript prototypes and inheritance is essential for any front-end developer. These concepts enable you to create efficient, maintainable, and scalable code. By understanding how prototypes work and leveraging inheritance patterns, you can enhance your JavaScript applications and stay ahead in the rapidly evolving field of web development.
Ready to take your JS JavaScript skills to the next level? Explore more resources and start experimenting with prototypes and inheritance in your projects. The future of web development is bright, and with the right knowledge, you can lead the way.