JavaScript is a powerful language that has transformed how we build web applications. At the heart of its performance lies the V8 Engine, an advanced piece of software that powers both Google Chrome and Node.js. By leveraging Just-in-Time (JIT) compilation and sophisticated memory management techniques, V8 has turned JavaScript into a high-performance language suitable for a wide range of applications. This post dives deep into how these technologies work together to optimize code execution and memory management.
Introduction to JavaScript Engines
JavaScript engines are essential components that drive the execution of JavaScript code. They convert human-readable code into machine code that a computer can execute. These engines are embedded in web browsers and runtime environments like Node.js, enabling seamless interaction between web applications and users.
The role of JavaScript engines extends beyond mere code execution. They are designed to optimize performance, ensuring that your web applications run smoothly and efficiently. By understanding how these engines work, developers can create faster and more responsive applications that meet the demands of modern users.
What is the V8 Engine?
The V8 Engine, developed by Google, is one of the most popular JavaScript engines. Launched in 2008, it was created to enhance the performance of the Chrome browser. Its success led to its integration into other platforms, including Node.js, enabling server-side JavaScript programming.
V8’s architecture includes several key features that set it apart from other JavaScript engines. It uses advanced compilation techniques and memory management strategies to deliver rapid execution speeds. These capabilities make V8 an ideal choice for developers looking to maximize the performance of their JavaScript applications.
V8’s Architecture
The architecture of the V8 Engine consists of several critical components, each responsible for different aspects of code execution. Parsing and lexical analysis are the first steps, where the engine reads and interprets JavaScript code. This stage involves breaking down code into tokens and creating an abstract syntax tree (AST).
Ignition serves as V8’s interpreter, converting JavaScript code into bytecode. This intermediary format allows for efficient execution by the engine. TurboFan, V8’s optimizing compiler, then takes over, transforming bytecode into highly-optimized machine code, speeding up execution significantly.
Understanding JIT Compilation
JIT compilation is a central feature of modern JavaScript engines. Unlike traditional interpreters, which execute code line by line, JIT compilers translate code into machine code at runtime. This process allows for optimization based on actual program behavior, resulting in faster execution.
The difference between interpreters and JIT compilers lies in their approach to code execution. While interpreters focus on simplicity and flexibility, JIT compilers prioritize performance. JIT enhances JavaScript performance by dynamically optimizing code paths, ensuring that applications run smoothly even under heavy loads.
The Two Phases of JIT in V8: Ignition and TurboFan
V8 employs a two-phase JIT compilation process, starting with Ignition. As the bytecode interpreter, Ignition handles initial code execution, ensuring that applications start quickly. It provides a baseline level of performance, allowing for rapid application loading.

TurboFan, the optimizing compiler, kicks in during the second phase, focusing on code that executes frequently. This “hot” code is aggressively optimized, resulting in significant performance gains. By balancing Ignition’s speed with TurboFan’s efficiency, V8 achieves a remarkable level of execution speed.
Code Optimization in V8
V8’s code optimization strategies are essential for maximizing JavaScript performance. Inline caching, for instance, speeds up property access by caching the location of object properties. Monomorphic functions, which operate on single data types, are optimized for faster execution.
Hidden classes play a crucial role in optimization, providing a blueprint for object layout. They enable efficient property access and method invocation. However, when code execution patterns change, de-optimization occurs, reverting to less-optimized code paths to maintain correctness.
Example of Code Optimization in V8 Engine Using JavaScript
Here’s a simple example demonstrating how V8 optimizes JavaScript code using hidden classes and inline caching to improve performance:
javascript
function Point(x, y) {
this.x = x;
this.y = y;
}
// Initial object creation
let p1 = new Point(1, 2);
let p2 = new Point(3, 4);
// Adding a new property
p1.z = 5;
// Accessing properties
function calculateDistance(p) {
return Math.sqrt(p.x * p.x + p.y * p.y + (p.z ? p.z * p.z : 0));
}
console.log(calculateDistance(p1));
console.log(calculateDistance(p2));
Explanation:
- Hidden Classes: When `p1` and `p2` are created, V8 assigns hidden classes to these objects. Hidden classes are akin to creating a fixed structure for the object’s properties, which helps V8 optimize property access.
- Inline Caching: When `p.x`, `p.y`, and `p.z` are accessed within the `calculateDistance` function, V8 utilizes inline caching to remember the location of these properties. This minimizes lookup time across repeated calls.
- Polymorphic Behavior: The addition of a new property `z` to `p1` alters the hidden class for `p1`, making `calculateDistance` potentially handle different object shapes. V8 manages these changes and optimizes the code’s execution paths efficiently.
By understanding how these optimization techniques work, developers can write code that takes full advantage of V8’s powerful engine capabilities, resulting in faster and more efficient JavaScript applications.
Garbage Collection in V8
Garbage collection is a vital aspect of memory management in JavaScript. It automatically reclaims memory used by objects that are no longer needed, preventing memory leaks and reducing the risk of application crashes. V8 employs advanced garbage collection techniques to achieve efficient memory management.
Generational garbage collection is a key feature of V8, dividing memory into young and old generations. This approach optimizes memory allocation and reclamation, ensuring that short-lived objects are quickly discarded while long-lived objects remain in memory.
Code Examples of Garbage Collection in V8 Engine Using JavaScript
While direct control over garbage collection is limited in JavaScript, understanding object lifecycle and memory management in V8 can help developers write efficient code. V8’s garbage collector automatically manages memory, focusing on reclaiming memory from objects no longer in use. Below are examples that illustrate some aspects related to garbage collection:
Example 1: Object Creation and Automatic Garbage Collection
javascript
function createObjects() {
let obj1 = { name: ‘Object 1’ };
let obj2 = { name: ‘Object 2’ };
}
// Objects obj1 and obj2 become eligible for garbage collection
// after the createObjects function execution ends as they are no longer accessible
createObjects();
Explanation:
In this example, `obj1` and `obj2` are local variables inside the `createObjects` function. Once the function execution is completed, these objects can no longer be accessed, making them eligible for garbage collection.
Example 2: Avoiding Memory Leaks with Closures
javascript
function createClosure() {
let largeArray = new Array(1000000).fill(‘*’);
return function() {
console.log(‘This closure holds a large array in memory’);
};
}
let closure = createClosure();
// The closure still references largeArray, preventing garbage collection
closure = null; // Removing the reference allows garbage collection
Explanation:
Here, `largeArray` is captured by the closure created in `createClosure`. This prevents `largeArray` from being garbage collected. Setting `closure` to `null` removes the reference, allowing V8 to reclaim the `largeArray` memory.
Example 3: Circular References and Garbage Collection
javascript
function CircularReference() {
let obj1 = {};
let obj2 = {};
obj1.reference = obj2;
obj2.reference = obj1;
// Breaking the circular reference for garbage collection
obj1 = null;
obj2 = null;
}
CircularReference();
Explanation:
In this example, `obj1` and `obj2` have circular references. By setting both to `null`, the circular reference is broken, allowing the garbage collector to reclaim the memory used by these objects.
Understanding these examples helps developers ensure that their code allows V8’s garbage collector to manage memory effectively, preventing memory leaks and promoting efficient memory usage in JavaScript applications.
V8’s Heap and Memory Structure
Understanding the heap and memory structure of V8 is essential for effective memory management. The JavaScript heap is where all dynamic memory allocations occur. It is divided into two regions, the young and old generations, each with distinct roles in memory management.
The stack, on the other hand, is a region of memory used for storing function call frames and local variables. V8’s memory allocation strategies ensure efficient use of both heap and stack memory, minimizing memory overhead while maximizing application performance.
Mark-and-Sweep Garbage Collection in V8
The mark-and-sweep algorithm is a foundational garbage collection technique used by V8. It identifies live objects by marking them during execution and then sweeps away unmarked objects, freeing up memory. This process ensures that memory is efficiently reclaimed, preventing leaks.
V8’s implementation of mark-and-sweep involves several optimizations, including incremental marking, which reduces pause times during garbage collection. These enhancements ensure that applications remain responsive, even under heavy memory loads.

Scavenging and Compaction in V8
Scavenging is a garbage collection technique used to clean up the young generation. It involves copying live objects to a new space, discarding dead objects, and compacting memory. This process reduces fragmentation and increases memory availability.
Compaction, on the other hand, defragments memory in the old generation, ensuring that large objects can be allocated without delay. Together, scavenging and compaction optimize memory usage, maintaining high levels of application performance.
Memory Leaks in JavaScript
Memory leaks occur when unused memory is not released, leading to increased memory consumption and potential application crashes. They are a common issue in JavaScript applications, often caused by retaining references to unused objects.
Detecting and fixing memory leaks requires careful analysis of memory usage patterns. Tools like Chrome DevTools provide valuable insights into memory allocation and utilization, helping developers identify and resolve memory leaks.
Tools for Monitoring and Optimizing Memory Usage
Monitoring memory usage is crucial for maintaining application performance. Chrome DevTools offers a suite of tools for tracking memory consumption and identifying bottlenecks. Memory profiling allows developers to analyze memory usage patterns and optimize resource allocation.
Heap snapshots provide detailed information about memory usage, enabling developers to pinpoint potential issues. By leveraging these tools, developers can ensure that their applications remain efficient and responsive, even under heavy loads.
Advanced Tools for Monitoring and Optimizing Memory Usage
To effectively monitor and optimize memory usage, developers can leverage a variety of advanced tools beyond the standard offerings of Chrome DevTools. These tools provide deep insights and help ensure that applications use memory efficiently, thus maintaining high performance.
1. Node.js Memory Tools
Node.js provides specific tools for profiling and debugging memory usage in server-side JavaScript applications. The `–inspect` flag allows developers to attach a debugger that can be used to analyze memory usage and identify memory leaks in Node.js. Tools like `clinic` offer a user-friendly interface that visualizes the memory profile of a running application, allowing for real-time diagnosis and optimization.
2. Performance Monitoring Platforms
Platforms such as New Relic, Dynatrace, and DataDog offer comprehensive monitoring solutions that include memory usage tracking as part of their suite. These platforms not only track memory allocation but also provide insights into application performance under various load conditions, helping developers identify memory-related issues at scale.
3. Heap Memory Analyzers
Tools like Heapster and MemLab specialize in analyzing heap memory, allowing developers to run and compare memory snapshots over time. These tools can help uncover patterns that lead to memory bloat and provide visual representation of memory trends, making analysis intuitive.
4. Automated Testing and Load Testing Tools
Incorporating automated tests that include memory performance metrics can ensure that changes in code do not introduce memory regressions. Tools like Apache JMeter and LoadRunner can simulate high-traffic scenarios, stressing the application to identify potential memory bottlenecks.
5. Static Analysis Tools
Static code analyzers such as ESLint and SonarQube can be configured to flag code patterns known to cause memory issues. This proactive approach helps prevent memory leaks by identifying problematic code before it reaches production.
Using these advanced tools, developers can maintain their applications’ memory efficiency, ensuring that they remain responsive and reliable. These insights help significantly reduce the risk of memory-related issues leading to performance degradation or application crashes.
V8 and ECMAScript Standards
V8’s alignment with ECMAScript standards ensures compatibility with the latest JavaScript features. The engine adapts to changes in the ECMAScript specification, implementing new capabilities and optimizing existing ones. This responsiveness keeps V8 at the forefront of JavaScript development.
V8’s role in the evolution of JavaScript is significant, driving the adoption of new features and best practices. By staying current with ECMAScript changes, V8 continues to deliver cutting-edge performance and functionality.
V8’s Handling of Asynchronous Code
Asynchronous programming is a core component of modern web development. V8’s event loop efficiently manages asynchronous tasks, ensuring that applications remain responsive. The engine optimizes the execution of callbacks, promises, and async/await, enhancing the performance of asynchronous code.
By understanding how V8 handles asynchronous programming, developers can create applications that are both efficient and user-friendly. This knowledge enables the seamless integration of asynchronous features, improving the overall user experience.
Security Features in the V8 Engine
Security is a top priority for JavaScript engines. V8 incorporates numerous security features, including sandboxing and isolation, to protect applications from vulnerabilities. These measures ensure that code execution remains safe and secure, preventing unauthorized access and data breaches.
V8’s commitment to security extends to regular updates and patches, addressing potential threats and vulnerabilities. By maintaining a robust security posture, V8 provides developers with the confidence to build and deploy applications without compromising user safety.
V8’s Performance Tuning Techniques
Optimizing JavaScript code for performance is essential for achieving high levels of application efficiency. V8 offers a range of performance tuning techniques, including micro-optimizations and code refactoring. These strategies enable developers to maximize the potential of their applications.
By implementing best practices for code optimization, developers can ensure that their applications remain competitive in an increasingly demanding landscape. V8’s performance tuning capabilities provide the tools needed to achieve this goal.
Comparing V8 with Other JavaScript Engines
V8 is not the only JavaScript engine available. Mozilla’s SpiderMonkey and Apple’s JavaScriptCore are notable alternatives, each with unique features and capabilities. Comparing these engines provides valuable insights into the strengths and weaknesses of each.
While V8 excels in performance and compatibility, SpiderMonkey is known for its advanced debugging tools and JavaScriptCore for its seamless integration with Apple’s ecosystem. Understanding these differences helps developers choose the right engine for their specific needs.
SpiderMonkey: A Competitor to the V8 JavaScript Engine
SpiderMonkey, developed by Mozilla, is a powerful competitor to the V8 JavaScript engine. As the first JavaScript engine ever written, SpiderMonkey is known for its extensive history and constant innovation. It is embedded in Mozilla Firefox and other products, offering a diverse range of features such as precise generational garbage collection and Just-In-Time (JIT) compilation.
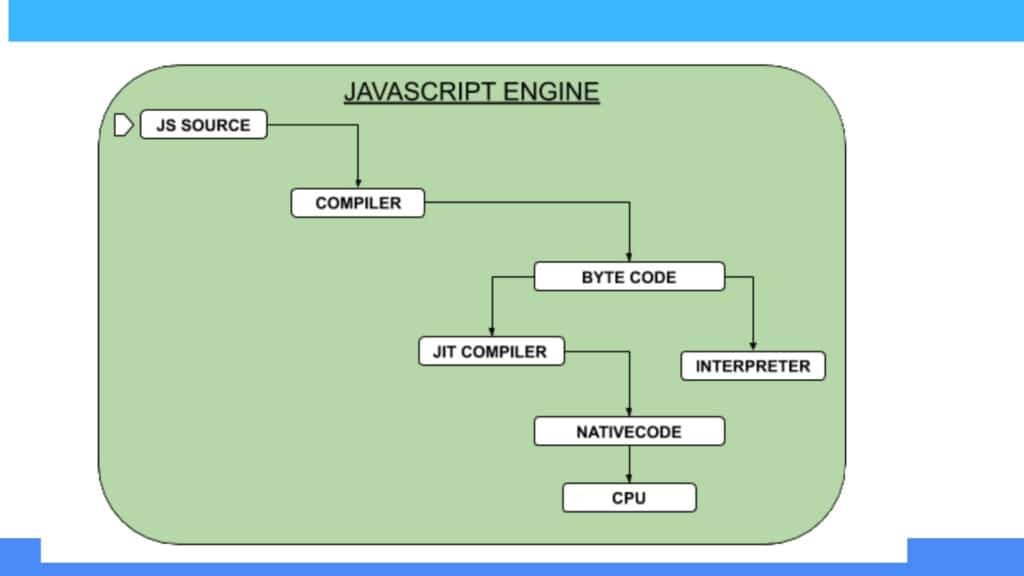
Additionally, SpiderMonkey is renowned for its robust debugging capabilities, which provide developers with advanced tools to identify and resolve issues efficiently. This comprehensive set of features makes SpiderMonkey a formidable alternative to V8, particularly in scenarios where debugging and fine-grained memory management are priorities. Despite the similarities between the two engines, SpiderMonkey’s strengths lie in providing a balance of performance and strong adherence to JavaScript standards, ensuring a reliable and predictable development experience.
JavaScriptCore: Another Alternative to V8
JavaScriptCore, also known as SquirrelFish, is the JavaScript engine used in Apple’s Safari browser. It offers similar capabilities to V8, such as JIT compilation and garbage collection. However, its unique selling point lies in its seamless integration with Apple’s ecosystem. This tight integration allows for optimization between the different components of an application, providing a better user experience on Apple devices.
Additionally, JavaScriptCore has features like automatic memory management and low-level optimizations that make it ideal for developing applications specific to iOS or macOS platforms. While V8 may be more versatile in terms of platform compatibility, JavaScriptCore excels in optimizing performance within the Apple ecosystem. Ultimately, both V8 and JavaScriptCore offer robust performance and compatibility with the latest JavaScript features, making them strong contenders in the world of JavaScript engines. So, it is up to developers to weigh the specific needs of their projects before choosing between these two options.
Summary
V8’s innovative features and continuous evolution make it a leading choice for developers looking to build efficient and high-performing applications. From its alignment with ECMAScript standards to its advanced handling of asynchronous code, V8 provides the tools needed to create cutting-edge web experiences. With a focus on security and performance optimization, V8 remains at the forefront of JavaScript development and continues to drive innovation in the field. And while there
JIT Compilation and Memory Management in Other Engines
JIT compilation and memory management are critical features of all modern JavaScript engines. SpiderMonkey and JavaScriptCore implement these capabilities differently, resulting in variations in performance and efficiency.
Exploring these differences provides a deeper understanding of how JIT compilation and memory management impact JavaScript execution. By examining the approaches of different engines, developers can make informed decisions about which platform best suits their needs.
Comparing V8 with Upcoming JavaScript Engines
As technology evolves, new JavaScript engines are emerging, each bringing innovative capabilities and enhancements to the table. One such engine is GraalVM, which aims to provide language interoperability across a range of programming languages, including JavaScript. Unlike V8, GraalVM is built on the Java Virtual Machine (JVM) and is designed to execute code in a multi-language runtime environment. This capability opens up opportunities for integrating JavaScript with Java and other languages seamlessly, offering flexibility in application development.
Another promising engine is WebAssembly (Wasm) focused engines, which are being developed to run Wasm more efficiently alongside JavaScript. Though not direct replacements for engines like V8, these new engines come with the promise of executing heavier computations more effectively, thus complementing JavaScript where V8 may lag, especially in performance-critical applications.
Both GraalVM and Wasm-targeted engines highlight a trend towards diversifying the capabilities and use cases of JavaScript engines. Their focus on interoperability and performance offers developers new possibilities beyond what V8 currently offers, suggesting a future landscape where multiple engines might coexist to serve different needs, thereby enhancing the overall performance and scope of web applications.
Future Developments in V8
The future of V8 is bright, with ongoing developments and enhancements aimed at improving performance and functionality. Upcoming features include advancements in JIT compilation, memory management, and security, ensuring that V8 remains at the cutting edge of JavaScript technology.
By staying informed about future developments, developers can prepare for upcoming changes and leverage new capabilities to enhance their applications. V8’s commitment to innovation ensures that it will continue to lead the way in JavaScript performance and optimization.
Future JavaScript Engine Trends
The future of JavaScript engines is poised to witness remarkable advancement and diversification, driven by the need for increased performance, security, and versatility across platforms. As applications grow in complexity and demand finer control over execution, engines are expected to focus more on optimizing for specific workloads, such as intensive computations or server-side processing. Innovations in JIT compilation techniques are also anticipated to play a crucial role, enabling faster execution by dynamically adapting to code patterns in real-time.
Another significant trend is the convergence of JavaScript with other programming languages, enhancing interoperability. Engines like GraalVM are pioneering this multi-language execution environment, allowing developers to seamlessly blend JavaScript with languages like Java, Python, and Ruby to enrich the app ecosystem. This trend towards hybrid solutions could redefine how developers approach problem-solving, providing more tools in a unified runtime.
Security and privacy will likely be front and center, with engines implementing more sophisticated techniques for safe execution of third-party code and protecting user data. Moreover, as WebAssembly becomes more mainstream, JavaScript engines are expected to integrate further with Wasm to leverage its strengths in performance optimization, especially for compute-heavy tasks.
Developments in AI and machine learning are also influencing engine evolution, with future engines potentially incorporating machine learning models to optimize code execution patterns dynamically. As browsers and environments diversify, engines will continue to adapt, ensuring that JavaScript remains a versatile and robust language for the next generation of web and software development.
Conclusion
The V8 Engine, with its advanced JIT compilation and memory management techniques, has revolutionized JavaScript performance. By understanding the intricacies of V8’s architecture and capabilities, developers can optimize their applications for maximum efficiency and responsiveness.
For web developers and JavaScript enthusiasts, mastering the inner workings of the V8 Engine is essential for building high-performance applications. By leveraging the power of V8, developers can create robust, scalable, and efficient web applications that meet the demands of today’s users.
I’m extremely inspired along with your writing talents and also with the structure in your blog. Is this a paid subject matter or did you customize it yourself? Anyway keep up the nice quality writing, it’s uncommon to see a nice blog like this one today!