In today’s rapidly evolving tech landscape, writing clean and efficient code is more crucial than ever. For software developers and tech enthusiasts exploring the intricacies of Object-Oriented Programming (OOP), understanding and implementing the SOLID principles can be a game-changer. These principles not only guide you in writing cleaner code but also ensure that your software designs are scalable and maintainable. This blog post will walk you through the SOLID principles, offering practical insights and expert tips to elevate your coding skills.
Introduction to SOLID Principles Writing Cleaner More Efficient Code
The SOLID principles are a set of five design principles intended to make software designs more understandable, flexible, and maintainable. At their core, these principles help developers avoid common pitfalls in software development, such as tightly coupled code and complex class hierarchies. By adopting SOLID principles, you can write code that is easier to manage and evolve.
The Foundation of SOLID Why It Matters in Object-Oriented Design
Object-Oriented Design (OOD) aims to facilitate the creation of software that is easy to understand and modify. SOLID principles serve as the foundation for OOD by providing a structured approach to designing software components. They focus on improving code readability and reducing the risk of errors, making it easier for teams to collaborate and build robust applications.
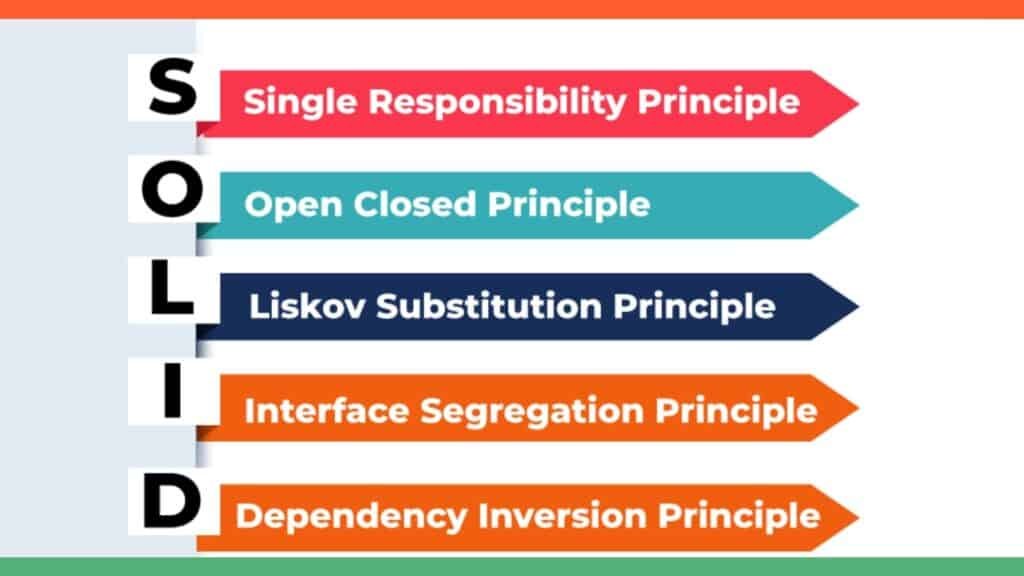
S is for Single Responsibility The Key to Focused Classes
The Single Responsibility Principle (SRP) emphasizes that a class should have only one reason to change, meaning it should have a single responsibility or function. By adhering to SRP, you create classes that are easier to test and debug, significantly simplifying the codebase.
How the Single Responsibility Principle Keeps Code Manageable
Imagine a user authentication class that’s responsible for both logging in users and managing their permissions. This violates SRP, as it combines multiple responsibilities into a single class. By splitting these functions into separate classes, you can enhance code clarity and maintainability. This approach allows developers to focus on individual tasks without being overwhelmed by extraneous responsibilities.
python
Before applying Single Responsibility Principle
class UserAuthManager:
def login_user(self, username, password):
# Logic for logging in a user
pass
def manage_permissions(self, user, permissions):
# Logic for managing user permissions
pass
After applying Single Responsibility Principle
class UserLogin:
def login_user(self, username, password):
# Logic for logging in a user
pass
class PermissionManager:
def manage_permissions(self, user, permissions):
# Logic for managing user permissions
pass
In this example, the initial `UserAuthManager` class violates the Single Responsibility Principle by handling both user login and permission management. By refactoring the code, we create two focused classes: `UserLogin` for handling login operations and `PermissionManager` for managing permissions. This separation of concerns improves code manageability and makes the system easier to update and debug.
O is for Open/Closed Extending Without Modifying Code
The Open/Closed Principle (OCP) dictates that software entities should be open for extension but closed for modification. This principle ensures that existing code remains untouched when new features are added, minimizing the risk of introducing bugs.
python
Violation of Open/Closed Principle
class VolumeCalculator:
def calculate(self, shapes):
total_volume = 0
for shape in shapes:
if isinstance(shape, Cube):
total_volume += shape.side ** 3
elif isinstance(shape, Sphere):
total_volume += (4/3) * 3.14159 * (shape.radius ** 3)
return total_volume
Applying the Open/Closed Principle
class Shape:
def volume(self):
raise NotImplementedError(“You should implement this!”)
class Cube(Shape):
def init(self, side):
self.side = side
def volume(self):
return self.side ** 3
class Sphere(Shape):
def init(self, radius):
self.radius = radius
def volume(self):
return (4/3) * 3.14159 * (self.radius ** 3)
class VolumeCalculator:
def calculate(self, shapes):
total_volume = 0
for shape in shapes:
total_volume += shape.volume()
return total_volume
Example usage
shapes = [Cube(3), Sphere(2)]
calculator = VolumeCalculator()
print(calculator.calculate(shapes))
In this example, the `VolumeCalculator` class adheres to the Open/Closed Principle by allowing new shapes to be added without modifying existing code. Each shape class implements a `volume` method, ensuring the `VolumeCalculator` only needs to call this method, regardless of the shape type. This way, when new shapes are introduced, developers can simply create a new class without altering the calculator logic.
Mastering the Open/Closed Principle for Flexible Software Design
Consider a shape-drawing application that initially supports circles and squares. By applying OCP, you can introduce new shapes, like triangles or hexagons, without altering the original code. This promotes code reuse and reduces development time, as you’re building on a solid foundation without the need for extensive refactoring.
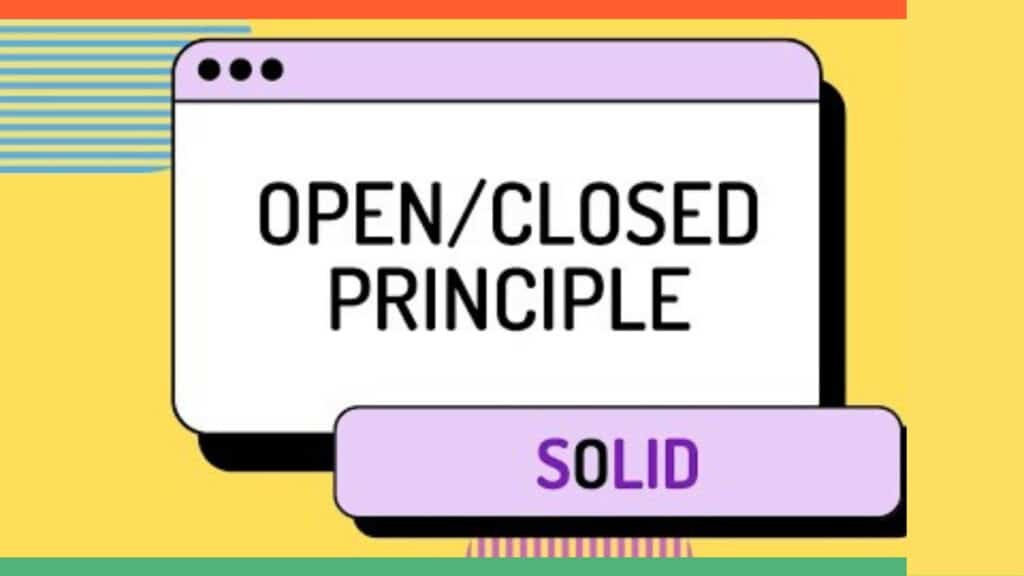
L is for Liskov Substitution Ensuring Consistency in Inheritance
The Liskov Substitution Principle (LSP) states that objects of a superclass should be replaceable with objects of its subclasses without affecting the program’s correctness. LSP ensures that inheritance is used appropriately, maintaining consistency in behavior across related classes.
python
Violation of Liskov Substitution Principle
class Bird:
def fly(self):
print(“Flying”)
class Penguin(Bird):
def fly(self):
raise Exception(“Penguins cannot fly!”)
def let_bird_fly(bird: Bird):
bird.fly()
Example usage
penguin = Penguin()
This will raise an exception because Penguin violates LSP
let_bird_fly(penguin)
Adhering to Liskov Substitution Principle
class Bird:
def move(self):
pass
class FlyingBird(Bird):
def fly(self):
print(“Flying”)
class Penguin(Bird):
def swim(self):
print(“Swimming”)
def let_bird_move(bird: Bird):
if isinstance(bird, FlyingBird):
bird.fly()
elif isinstance(bird, Penguin):
bird.swim()
Example usage
flying_bird = FlyingBird()
penguin = Penguin()
let_bird_move(flying_bird) # Outputs: Flying
let_bird_move(penguin) # Outputs: Swimming
In this example, the initial design violates the Liskov Substitution Principle by having a `Penguin` subclass that can’t perform the fly operation that a `Bird` superclass promises. The revised design introduces a `move` method in the `Bird` class, separating flying and swimming behaviors, allowing both `FlyingBird` and `Penguin` subclasses to honor the contract without exceptions, thus adhering to LSP.
Implementing the Liskov Substitution Principle in Real-World Projects
A common example of LSP is a vehicle class hierarchy. Suppose you have a parent class for vehicles with a method to calculate speed. When creating child classes for cars and bicycles, you must ensure that these subclasses can be used interchangeably with the parent class, maintaining predictable behavior.
I is for Interface Segregation Keeping Interfaces Lean and Clean
The Interface Segregation Principle (ISP) asserts that clients should not be forced to depend on interfaces they do not use. By designing smaller, more targeted interfaces, ISP helps prevent unnecessary dependencies and promotes modular, decoupled code.
The Interface Segregation Principle Designing Interfaces for Specific Clients
Imagine a printer interface with methods for both color and black & white printing. If a specific printer only supports black & white printing, it’s inefficient to force it to implement the color printing method. By splitting the interface into separate components, you allow clients to focus on the functionalities they require, enhancing flexibility and maintainability.
D is for Dependency Inversion Breaking the Tight Coupling Between Classes
The Dependency Inversion Principle (DIP) emphasizes that high-level modules should not depend on low-level modules; both should rely on abstractions. This principle fosters loose coupling between components, allowing for easier modifications and updates.
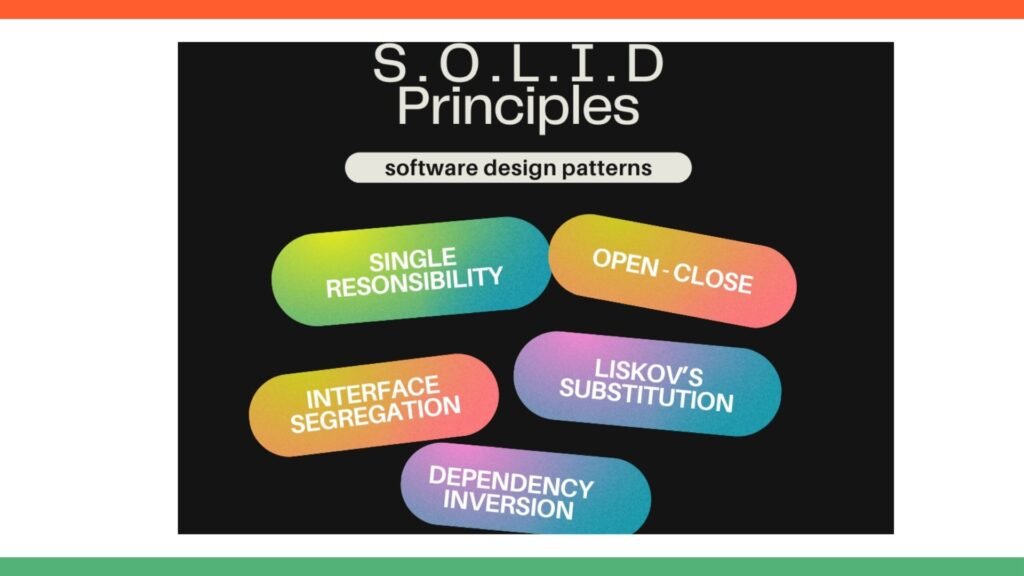
Leveraging the Dependency Inversion Principle for Scalable Applications
Consider a web application where high-level business logic depends on low-level data access code. By introducing abstractions, such as interfaces, you can decouple these layers, enabling you to replace or modify the underlying data access logic without impacting the rest of the application.
Common Mistakes When Implementing SOLID Principles and How to Avoid Them
While the SOLID principles offer significant advantages, they can be challenging to implement correctly. Common mistakes include over-engineering solutions, misapplying principles, and disregarding performance considerations. By understanding these pitfalls and actively working to avoid them, you can ensure successful implementation.
Refactoring Legacy Code to Follow the SOLID Principles
Legacy code often lacks adherence to SOLID principles, resulting in tangled dependencies and difficult-to-maintain systems. By gradually refactoring legacy code and applying SOLID principles, you can improve code quality, reduce technical debt, and extend the software’s lifespan.
Benefits of SOLID Principles Writing Maintainable and Scalable Code
Adopting SOLID principles can lead to numerous benefits, including improved code readability, reduced maintenance costs, and enhanced scalability. By fostering modular, reusable components, SOLID principles empower developers to create software that can adapt to changing requirements over time.
SOLID Principles and Design Patterns How They Work Together
Design patterns are reusable solutions to common software design problems, while SOLID principles provide guidelines for creating flexible and maintainable code. By combining these concepts, you can develop robust software architectures that handle complexity gracefully.
Real-Life Examples of SOLID Principles in Modern Software Development
Numerous modern software projects successfully implement SOLID principles. For instance, a large-scale e-commerce platform may apply LSP to ensure consistent behavior across different product categories, while an open-source library might leverage OCP to promote code reuse and reduce the likelihood of bugs. By understanding how SOLID principles can be applied in various scenarios, you can make informed decisions when developing your projects.
Overall, the use of SOLID principles can greatly improve the quality and longevity of software projects by promoting maintainability, scalability, and flexibility. Developers need to understand these principles and apply them appropriately to create well-designed, robust systems. With practice and careful consideration, incorporating SOLID principles into your development process can lead to more efficient, reliable, and successful software projects.
Best Practices for Applying SOLID in JavaScript Python C# [Insert Language]
Regardless of the programming language you’re using, SOLID principles can be applied effectively to improve code quality. By following best practices, such as writing clear documentation and conducting thorough code reviews, you can ensure successful implementation and ongoing adherence to these principles.
Summary of Key SOLID Benefits
Implementing the SOLID principles offers numerous advantages for software development. First, they enhance code maintainability by ensuring that code is modular and components are easily understandable. This modularity not only reduces the time and effort required for updates but also simplifies debugging processes. Further, SOLID principles facilitate scalability, allowing software to grow and adapt to new requirements without major refactoring. They promote code reuse through well-defined interfaces and abstractions, reducing redundancy and increasing efficiency. Adherence to SOLID helps minimize technical debt by encouraging best practices in design, leading to robust and reliable software systems. Overall, embracing these principles supports the development of clean, efficient, and adaptable software solutions that can evolve with changing demands.
SOLID vs Other Design Methodologies How Does It Stack Up
While SOLID principles offer significant advantages, it’s essential to consider other design methodologies, such as Domain-Driven Design (DDD) or Model-View-Controller (MVC), when architecting software. By understanding the strengths and weaknesses of each approach, you can make informed decisions about the best methodologies for your specific projects.
The Future of Software Design Why SOLID Principles Still Matter Today
Despite the rapid pace of technological advancements, the fundamental principles of good software design remain unchanged. SOLID principles continue to provide valuable guidance, helping developers create maintainable, scalable, and efficient code that stands the test of time.
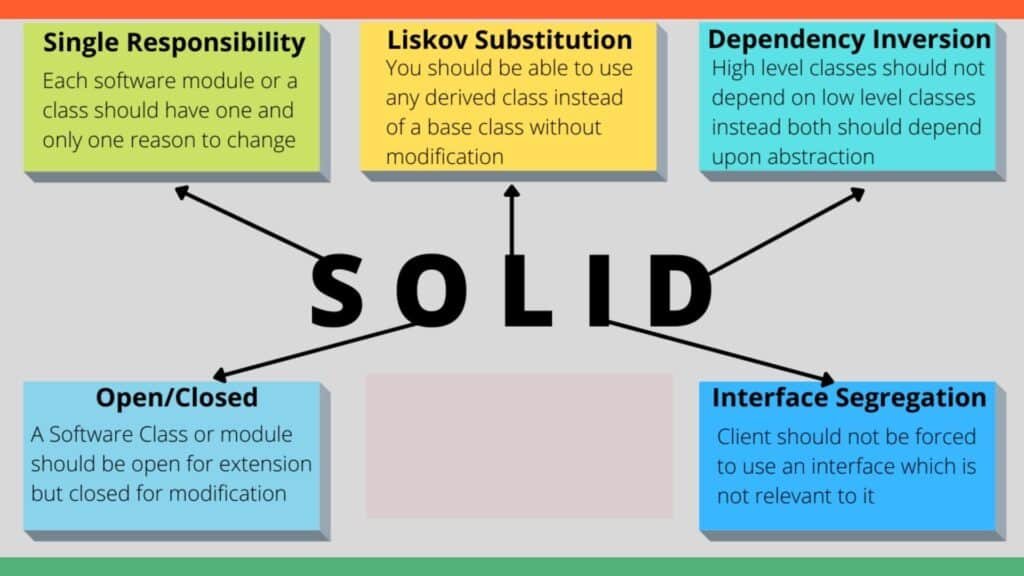
In conclusion, the SOLID principles serve as a vital foundation for any software developer looking to improve their coding skills and build robust applications. By incorporating these principles into your daily practice, you can write cleaner, more efficient code that meets the demands of today’s dynamic tech landscape. To further explore SOLID principles and enhance your understanding, consider reviewing case studies, practical examples, and additional resources tailored to your preferred programming language.
Resources for Further Learning
- Books:
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides
- The Pragmatic Programmer: Your Journey to Mastery by Andrew Hunt and David Thomas
- Online Courses:
- SOLID Principles of Object-Oriented Design on Pluralsight
- Design Patterns in Modern C++ on Udemy
- Java Programming: SOLID Principles on Coursera
- Articles and Blogs:
- “Understanding the SOLID Principles” on Medium
- “A Beginner’s Guide to SOLID Principles” by SitePoint
- “Better Programming Through SOLID Principles” on Dev.to
- Videos and Webinars:
- “SOLID Principles: Introduction and Overview” on YouTube
- “Understanding SOLID Principles” webinar on a reputable tech platform like TechRepublic
- Communities and Forums:
- Stack Overflow: Engage with experts and seek guidance on specific SOLID implementation challenges.
- Reddit: Participate in subreddits like r/programming or r/softwaredevelopment for discussions and insights.
- Practice Platforms:
- Codewars: Practice SOLID principles with coding challenges that involve real-world scenarios.
- HackerRank: Enhance your understanding by solving problems related to software design principles.
These resources can significantly bolster your understanding of SOLID principles and their application in modern software development practices.
Frequently Asked Questions
1. What are the SOLID principles in programming?
Answer:
SOLID is an acronym for five design principles that help software developers write better, more maintainable, and scalable code. These principles are:
- S: Single Responsibility Principle (SRP)
- O: Open/Closed Principle (OCP)
- L: Liskov Substitution Principle (LSP)
- I: Interface Segregation Principle (ISP)
- D: Dependency Inversion Principle (DIP)
2. What is the Single Responsibility Principle (SRP)?
Answer:
SRP states that a class should have only one reason to change, meaning it should have only one job or responsibility.
Real-Life Example:
A class representing a “User” should only manage user-related data and behavior. If you add functionality to send emails within this class, it would violate SRP. Instead, you should create a separate EmailSender class for email-related functionality.
3. Can you explain the Open/Closed Principle (OCP)?
Answer:
The Open/Closed Principle states that software entities (like classes, modules, functions) should be open for extension but closed for modification.
Real-Life Example:
Consider a PaymentProcessor class. Instead of modifying this class every time you add a new payment method (e.g., PayPal, Credit Card, Bitcoin), you should extend the class by implementing new payment methods in separate classes (e.g., PayPalProcessor, BitcoinProcessor) that adhere to a common PaymentMethod interface.
4. What is the Liskov Substitution Principle (LSP)?
Answer:
LSP suggests that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
Real-Life Example:
If you have a base class Bird with a method fly()
, and a subclass Penguin that cannot fly, replacing a Bird object with a Penguin object would break the code. To adhere to LSP, Penguin should not inherit from Bird but rather from a more general class that doesn’t assume all birds can fly.
5. Can you provide an example of the Interface Segregation Principle (ISP)?
Answer:
ISP states that clients should not be forced to depend on interfaces they do not use.
Real-Life Example:
A MultifunctionPrinter interface should not force a client to implement methods like scan()
or fax()
if they only need printing functionality. Instead, you could create smaller, more specific interfaces such as Printer, Scanner, and Fax, and let the client implement only the relevant ones.
6. What is the Dependency Inversion Principle (DIP)?
Answer:
DIP states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Also, abstractions should not depend on details.
Real-Life Example:
Suppose a ReportGenerator class depends on a FileWriter class to generate reports. Instead of directly coupling them, you introduce an abstraction, such as a ReportWriter interface, and have FileWriter implement this interface. The ReportGenerator now depends on the abstraction, not the concrete class.
7. What happens if you violate the Single Responsibility Principle (SRP)?
Answer:
Violating SRP can lead to classes becoming too complex and difficult to maintain. Changes in one part of the class might require changes to unrelated parts, making the code harder to understand, test, and extend.
Example:
If you have a UserManager class that handles user data validation, user storage, and user notifications, modifying the notification logic might unintentionally affect the data validation logic, causing bugs.
8. How do you ensure a class adheres to the Open/Closed Principle?
Answer:
You can achieve OCP by using inheritance, interfaces, or composition. When adding new features, you extend or compose existing classes without modifying their code.
Example:
If you add a new discount strategy for an online store, you can create a new DiscountStrategy class and inject it into the ShoppingCart without changing the cart’s implementation.
9. What are the consequences of violating the Liskov Substitution Principle?
Answer:
Violating LSP can lead to unexpected behavior, bugs, or crashes. Substituting a subclass object for a parent class object might break the functionality, causing the program to fail.
Example:
If a Rectangle class has methods setHeight()
and setWidth()
, and you create a Square subclass where height equals width, you might face issues when you substitute a Square object for a Rectangle, as it would violate the expected behavior of the setHeight()
and setWidth()
methods.
10. Can ISP help reduce the size of classes?
Answer:
Yes, ISP helps reduce the size of classes by breaking large, monolithic interfaces into smaller, more specialized ones. This way, a class implements only the methods it actually needs, which keeps it focused and concise.
Example:
A UserService interface could be split into smaller interfaces such as UserAuthentication and UserProfile to avoid forcing a client to implement methods unrelated to their specific needs.
11. How does the Dependency Inversion Principle improve code flexibility?
Answer:
By depending on abstractions (e.g., interfaces or abstract classes) instead of concrete classes, DIP allows you to swap out implementations without affecting high-level modules. This makes the system more flexible and extensible.
Example:
You can easily switch the implementation of a DatabaseService (e.g., from MySQL to MongoDB) in a UserService class by just changing the concrete implementation without modifying the UserService class itself.
12. Can you give a real-life example where SRP helps in code maintainability?
Answer:
Imagine a Customer class that handles both customer data and order processing. If you want to update the order processing logic, you risk breaking customer-related functionality. By separating concerns (e.g., having a Customer class for data and an OrderProcessor class for processing), changes to one aspect do not impact the other, making maintenance easier.
13. What is the difference between OCP and DIP?
Answer:
OCP is about making your code open for extension (adding new functionality) while keeping it closed for modification (without changing existing code). DIP, on the other hand, focuses on decoupling high-level and low-level modules via abstractions.
Example:
OCP would be relevant when adding a new Discount calculation to a checkout system, while DIP would ensure that the checkout system depends on an abstraction of the discount strategy, not on a concrete class.
14. How do SOLID principles help with testing?
Answer:
SOLID principles help create more modular, decoupled code, which is easier to test. For example, adhering to SRP ensures that classes are focused on a single task, making them more predictable and easier to unit test. Similarly, DIP allows you to mock dependencies, making testing simpler and more isolated.
15. What is an example of OCP in real-world software?
Answer:
In an e-commerce system, you might have a ShippingCostCalculator class. As new shipping methods are added, you extend the class by adding new subclasses (e.g., ExpressShippingCalculator, StandardShippingCalculator) without changing the original ShippingCostCalculator class.
16. How do you refactor code to follow the Liskov Substitution Principle?
Answer:
You refactor by ensuring that subclasses adhere to the expected behavior of their parent classes. If a subclass cannot meet the expectations (e.g., a subclass Dog should not inherit from Bird), you should reconsider the class hierarchy and use composition or interfaces instead of inheritance.
17. What role does polymorphism play in OCP?
Answer:
Polymorphism allows you to extend behavior without modifying existing code. By defining common interfaces or base classes, you can extend functionality through new classes without altering the core system, making it conform to OCP.
18. How does Interface Segregation reduce code bloat?
Answer:
ISP reduces code bloat by allowing clients to only implement the methods they need. This avoids unnecessary functionality from piling up in a single, large interface, keeping classes lean and focused.
19. Why is the Dependency Inversion Principle considered a high-level design principle?
Answer:
DIP is considered high-level because it affects the architecture of your entire system. It encourages you to design your system in a way that abstracts away low-level details, promoting flexibility and modularity at a broader level.
20. What’s the importance of SOLID principles in team-based development?
Answer:
SOLID principles promote code that is easier to understand, extend, and maintain. In team-based development, they help ensure that the codebase remains clean and modular, allowing multiple developers to work on different features without causing conflicts or making the system fragile.