In the realm of object-oriented programming (OOP), inheritance is a foundational concept that promises enhanced code reusability and a clearer organizational structure. For programmers and software engineers, understanding inheritance is key not only to writing efficient code but also to structuring programs in a way that’s both logical and scalable.
Introduction to Inheritance
Inheritance is not just a tool—it’s the bedrock of object-oriented programming. But why is it so integral, and how does it shape the way we write software? Aimed at tech enthusiasts and seasoned developers alike, this exploration begins with the basics before expanding into its broader implications.
For many, It is synonymous with efficiency. It allows programmers to build upon existing code, layering new functionalities without starting from scratch. This article will walk you through the essentials and offering insights into its advantages, limitations, and applications across various programming languages like Java, Python, and C++.
What Is Inheritance in OOP?
At its core, inheritance allows one class to inherit the properties and methods of another. This relationship forms a hierarchy, where a ‘child’ class (or subclass) derives attributes and behaviors from a ‘parent’ class (or superclass). But what does this mean in practice?
Consider a basic example from the world of software development. Suppose you have a class called “Vehicle.” You can create subclasses such as “Car” and “Bike” that inherit properties like speed and fuel capacity from “Vehicle.” This means you don’t need to rewrite these properties for every new vehicle type.
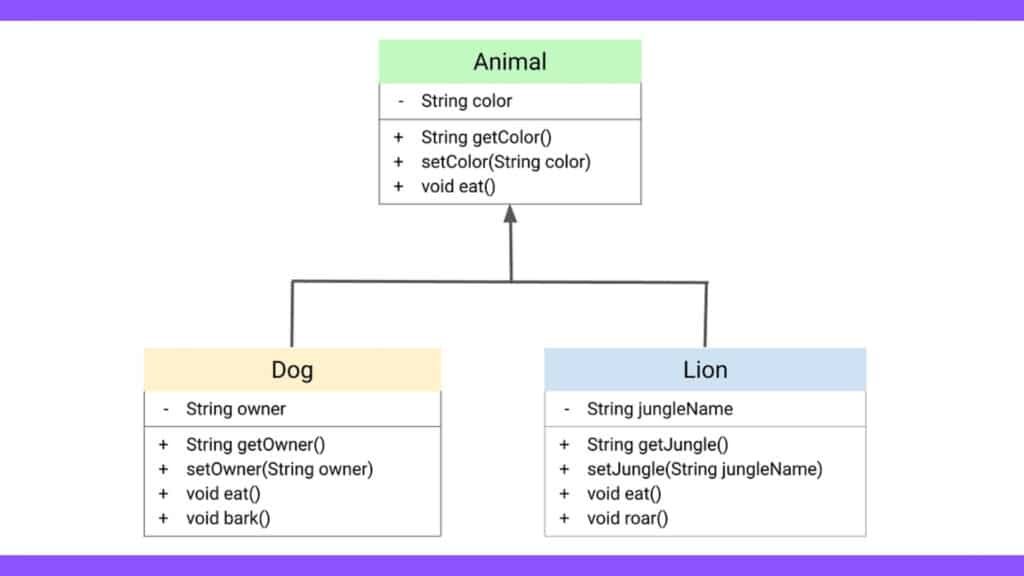
It provides a way to model real-world relationships in software, making programs more intuitive and reflective of actual entities. It also simplifies code maintenance, as any change in the parent class can automatically propagate to its subclasses.
python
Define the parent class
class Vehicle:
def init(self, brand, speed):
self.brand = brand
self.speed = speed
def display_info(self):
print(f”Brand: {self.brand}, Speed: {self.speed} km/h”)
Define the child class inheriting from Vehicle
class Car(Vehicle):
def init(self, brand, speed, fuel_type):
super().init(brand, speed)
self.fuel_type = fuel_type
def display_info(self):
super().display_info()
print(f”Fuel Type: {self.fuel_type}”)
Define another child class inheriting from Vehicle
class Bike(Vehicle):
def init(self, brand, speed, type_of_bike):
super().init(brand, speed)
self.type_of_bike = type_of_bike
def display_info(self):
super().display_info()
print(f”Type of Bike: {self.type_of_bike}”)
Example usage
car = Car(“Toyota”, 120, “Gasoline”)
bike = Bike(“Yamaha”, 80, “Sport”)
car.display_info()
bike.display_info()
In this example, the `Car` and `Bike` classes inherit from the `Vehicle` class, allowing them to share common properties such as `brand` and `speed`. Each subclass can also have its own unique attributes and methods, demonstrated here by `fuel_type` in `Car` and `type_of_bike` in `Bike`. The `super()` function is used to call the constructor of the parent class, ensuring shared properties are initialized correctly.
The Advantages of Inheritance: Code Reusability and Beyond
Inheritance is celebrated for its ability to promote code reusability, reducing redundancy and enhancing efficiency. By reusing pre-existing code, developers can save time, minimize errors, and focus on implementing new features.
Another advantage lies in extensibility. Programs built on this are easier to extend, making them adaptable to future needs or modifications. Imagine needing to add a “Truck” subclass under “Vehicle.” With inheritance, this process becomes straightforward and streamlined.
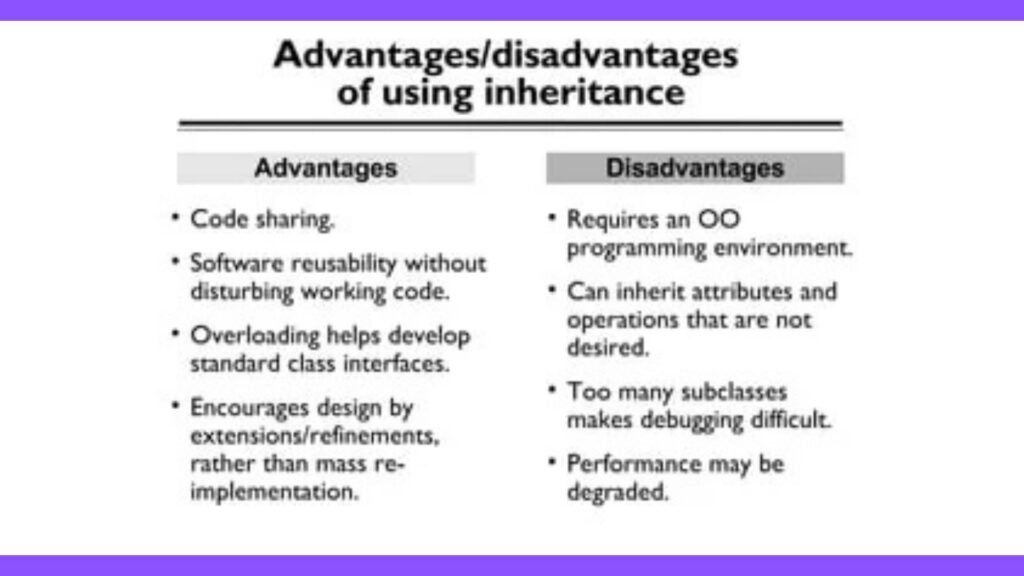
Beyond technical benefits, It supports a clean organizational structure. It aligns code with the logical hierarchy of related objects, making software easier to understand and manage. This clarity is particularly beneficial in large projects where team collaboration is essential.
How Inheritance Promotes Code Reusability and Extensibility
Code reusability is often touted as one of the primary benefits of inheritance. But how exactly does this work in practice? Let’s take a closer look at the mechanics.
When you create a subclass, it inherits all the non-private members (i.e., methods and properties) of its parent class. This means you can leverage existing code without having to duplicate it. For example, if your parent class includes a method to calculate fuel efficiency, all subclasses automatically acquire this capability.
Extensibility goes hand-in-hand with reusability. By building on a solid foundation, developers can introduce new functionalities with minimal disruption. In essence, It provides a framework that supports ongoing enhancement and adaptation.
Inheritance vs Composition: Which Should You Use?
When it comes to structuring software, developers often face a critical decision between inheritance and composition. While both approaches have their merits, choosing the right one can significantly impact the design and functionality of your program.
Inheritance is ideal for ‘is-a’ relationships, where one class is a specialized version of another. For instance, a “Sedan” is a type of “Car,” making it the logical choice.
Composition, on the other hand, is better suited for ‘has-a’ relationships. If a “Car” has a “Driver,” it’s more effective to compose these classes, giving “Car” an instance of “Driver” rather than inheriting from it.
The choice between inheritance and composition isn’t always clear-cut. It requires a deep understanding of the problem domain and careful consideration of how classes interact within the system.
Real-World Examples of Inheritance in Software Development
Inheritance is more than a theoretical concept—it’s widely used in real-world software development. Let’s explore some practical examples to illustrate its application.
In web development, it is often employed in frameworks like Django and Ruby on Rails. These frameworks use it to create reusable components, streamlining the development process and promoting consistency across applications.
In game development, it allows for the creation of complex hierarchies of game objects. A “Character” class might serve as a base for various playable and non-playable characters, each inheriting shared behaviors and attributes.
Even in enterprise software, it plays a crucial role. It enables the implementation of business logic that’s both flexible and scalable, accommodating changing requirements without necessitating a complete overhaul.
The Drawbacks and Limitations
Despite its many benefits, it is not without its challenges. In certain situations, it can complicate code and introduce maintenance difficulties. Understanding these limitations is essential for making informed design decisions.
One common issue is the potential for tight coupling between classes. When subclasses are heavily dependent on their parent class, changes in the superclass can lead to cascading effects, increasing the risk of errors.
It can also lead to issues with encapsulation. By exposing parent class internals to subclasses, it may inadvertently violate data hiding principles, reducing modularity and flexibility.
Lastly, it can sometimes result in an overly rigid class hierarchy. This inflexibility can hinder adaptation to new requirements and make it challenging to refactor code as projects evolve.
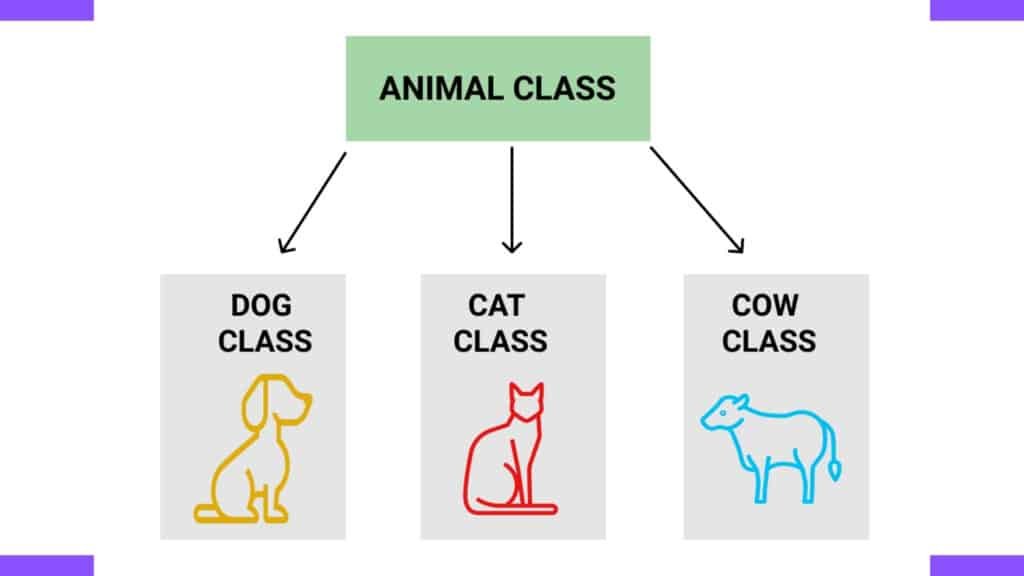
When Inheritance Can Lead to Fragile Base Class Problems
The term “fragile base class problem” refers to a situation where changes to a base class inadvertently break subclass functionality. This problem underscores one of the inherent risks of using inheritance.
The fragility arises when subclasses rely on specific behaviors or implementations within the base class. If these details change, it can disrupt the operation of subclasses, leading to unexpected bugs and inconsistencies.
To mitigate this issue, developers must exercise caution when modifying base classes. They should prioritize backward compatibility and thoroughly test changes to ensure they don’t adversely affect existing subclasses.
Inheritance in Java/Python/C++: How Different Languages Implement It
While the concept of inheritance is consistent across programming languages, its implementation can vary. Let’s examine how inheritance is handled in Java, Python, and C++.
In Java, inheritance is achieved using the `extends` keyword. Java supports single inheritance, where a class can inherit from only one superclass, but interfaces allow for multiple inheritance-like behavior.
Python, in contrast, supports multiple inheritance directly. By listing multiple parent classes in a class definition, Python allows subclasses to inherit from more than one superclass, providing greater flexibility.
C++ offers a combination of both approaches. It supports multiple inheritance, similar to Python, and also provides virtual inheritance to address the diamond problem, a common issue in complex class hierarchies.
Single Inheritance vs Multiple Inheritance: Pros and Cons
The debate between single inheritance and multiple inheritance has long been a topic of discussion among programmers. Each approach offers distinct advantages and challenges.
Single inheritance is straightforward and often easier to manage. It reduces complexity by limiting the number of potential inheritance paths, making it easier to understand class hierarchies.
Multiple inheritance, on the other hand, provides greater flexibility. It allows classes to inherit behaviors from multiple sources, facilitating more dynamic and versatile designs.
However, multiple inheritance can introduce ambiguity, particularly when different parent classes have overlapping methods or attributes. This can lead to conflicts that require careful resolution to maintain program stability.
Python Code Examples: Single Inheritance and Multiple Inheritance
Single Inheritance Example
In single inheritance, a subclass inherits from one superclass. Here’s a simple example in Python:
python
Superclass
class Vehicle:
def init(self, make, model):
self.make = make
self.model = model
def display_info(self):
print(f”Vehicle Make: {self.make}, Model: {self.model}”)
Subclass
class Car(Vehicle):
def init(self, make, model, doors):
super().init(make, model)
self.doors = doors
def display_car_info(self):
self.display_info()
print(f”Number of Doors: {self.doors}”)
Creating an instance of Car
my_car = Car(“Toyota”, “Corolla”, 4)
my_car.display_car_info()
Multiple Inheritance Example
In multiple inheritance, a subclass can inherit from more than one superclass. Below is an example in Python:
python
Superclass 1
class Drivable:
def start_engine(self):
print(“Engine started”)
Superclass 2
class Electric:
def charge_battery(self):
print(“Battery charging”)
Subclass
class ElectricCar(Drivable, Electric):
def drive(self):
self.start_engine()
print(“Driving the electric car…”)
Creating an instance of ElectricCar
my_electric_car = ElectricCar()
my_electric_car.charge_battery()
my_electric_car.drive()
These examples illustrate the fundamental concepts of single and multiple inheritance in Python, showcasing how subclasses can inherit functionalities from their respective superclasses.
Common Misconceptions About Inheritance in OOP
Despite its prevalence in software development, inheritance is often misunderstood. Let’s dispel some common misconceptions to clarify its role in OOP.
One misconception is that inheritance is always necessary for code reuse. While inheritance is a powerful tool for reusing code, composition and interfaces can provide alternative pathways to achieve similar outcomes without the complexities of inheritance hierarchies.
Another misconception is that inheritance should be used as widely as possible. In reality, overusing it can lead to bloated class hierarchies and reduced program flexibility. It’s essential to use it judiciously and only where it truly reflects the problem domain.
Lastly, some believe inheritance is a silver bullet for software design. While it offers significant benefits, it’s not a one-size-fits-all solution. Understanding its strengths and limitations is crucial for making informed design choices.
Use for Specialization and Generalization
Inheritance is particularly valuable for achieving specialization and generalization in software design. By structuring classes in a hierarchical manner, developers can model real-world relationships and encapsulate common behaviors.
Specialization allows subclasses to extend or override the behaviors of their parent class. This is useful for creating specific variations of a general concept, such as different types of vehicles inheriting from a “Vehicle” class.
Generalization, on the other hand, involves identifying shared behaviors and attributes and consolidating them into a common superclass. This reduces duplication and promotes code reuse across related classes.
How Inheritance Supports Hierarchical Class Structures
Hierarchical class structures are a hallmark of object-oriented programming, and it plays a pivotal role in their creation. By establishing clear parent-child relationships, developers can organize code logically and intuitively.
In a hierarchical structure, subclasses inherit from a common superclass, forming a tree-like arrangement. This organization simplifies code navigation and fosters a modular design, where changes in one part of the hierarchy have minimal impact on others.
An example of a hierarchical structure might be a “Shape” class with subclasses like “Circle,” “Square,” and “Triangle.” Each subclass inherits common properties from “Shape” while implementing its unique characteristics.
The Diamond Problem in Multiple Inheritance and How to Avoid It
The diamond problem is a well-known issue in multiple inheritance. It occurs when a class inherits from two classes that share a common ancestor, leading to ambiguity in the its hierarchy.
Consider a scenario where class “C” inherits from classes “A” and “B,” both of which inherit from “D.” If “C” calls a method defined in “D,” it’s unclear whether to use the implementation from “A” or “B.”
To address the diamond problem, many programming languages offer solutions like virtual inheritance (in C++) or using interfaces and mixins (in languages like Python and Java). These techniques ensure that the most appropriate method is invoked, maintaining program clarity and consistency.
Implementation
Implementing inheritance may seem daunting, but it’s a crucial skill for any programmer. Here’s a step-by-step guide to help you get started.
- Identify the hierarchical relationships between entities in your program. Determine which classes share common attributes or behaviors.
- Define a superclass that encapsulates shared properties and methods. Use this class as a foundation for your hierarchy.
- Create subclasses that extend the superclass, inheriting its features while adding their unique elements. Use the `extends` or `inherits` keyword, depending on your programming language.
- Override methods in subclasses as needed to tailor behaviors or provide specialized implementations. This allows for customization while maintaining a consistent interface.
- Test your hierarchy to ensure it functions as expected. Make sure changes in the superclass don’t inadvertently affect subclass operations.
By following these steps, you’ll be well-equipped to implement It effectively, creating robust and maintainable software.
When Not to Use Inheritance: Identifying Bad Practices
While inheritance offers numerous benefits, it’s not always the best choice. Recognizing when not to use it is key to avoiding pitfalls and ensuring optimal software design.
Avoid using it when it leads to excessive coupling between classes. If subclasses become too dependent on their parent class, it can hinder flexibility and future modifications.
Additionally, steer clear of inheritance when the relationship between classes isn’t genuinely hierarchical. Forcing an its structure where none exists can result in convoluted and difficult-to-maintain code.
Finally, don’t rely on it as a shortcut for code reuse. Consider alternatives like composition or interfaces when they better align with your program’s design goals.
Inheritance and Polymorphism: How They Work Together
Inheritance and polymorphism are closely intertwined concepts in object-oriented programming. Understanding how they work together can enhance your ability to design flexible and adaptable software.
Polymorphism allows objects to be treated as instances of their parent class, enabling a unified interface for different subclass implementations. This means you can write code that operates on a general level, while still accommodating specific behaviors.
You can create programs that are both dynamic and extensible. This synergy supports the creation of modular, reusable components that adapt to changing requirements with ease.
Real-World Use Cases of Inheritance: From Banking Systems to Games
Inheritance is a foundational concept in many real-world software applications. Let’s explore how it’s used in diverse domains, from banking systems to game development.
In the financial sector, It supports the creation of flexible and scalable banking systems. A “BankAccount” class might serve as a base for more specialized account types like “SavingsAccount” and “CheckingAccount,” each inheriting shared properties and behaviors.
In game development, It allows for the creation of complex hierarchies of game objects. A “Character” class might serve as a base for various playable and non-playable characters, each inheriting shared behaviors and attributes.
Even in enterprise software, It plays a crucial role. It enables the implementation of business logic that’s both flexible and scalable, accommodating changing requirements without necessitating a complete overhaul.
The Role in OOP Design Patterns
Design patterns are proven solutions to common problems in software design, and it plays a key role in many of them. Understanding these patterns can enhance your ability to create robust and maintainable software.
One common pattern that relies on inheritance is the Template Method pattern. This pattern defines a skeleton of an algorithm in a superclass, allowing subclasses to redefine specific steps without changing the overall structure.
Another example is the Factory Method pattern, which uses it to delegate the instantiation of objects to subclasses. This pattern promotes flexibility and extensibility, allowing new object types to be introduced without modifying existing code.
By incorporating design patterns into your software, you can leverage the power of it to create elegant and scalable solutions.
Alternatives : Using Interfaces and Composition
While inheritance is a powerful tool, it’s not always the best choice. In some cases, alternatives like interfaces and composition may offer more flexibility and simplicity.
Interfaces provide a way to define shared behaviors without dictating how they should be implemented. This allows different classes to adhere to a common contract while maintaining their unique implementations.
Composition, on the other hand, involves building classes by combining smaller, reusable components. This approach promotes loose coupling and greater modularity, making it easier to adapt to changing requirements.
By exploring these alternatives, you can create software that’s both flexible and maintainable, ensuring it stands the test of time.
Conclusion
Inheritance is a fundamental concept in object-oriented programming, offering significant benefits in terms of code reusability, extensibility, and organization. However, it’s not without its challenges, and understanding when to use it—and when to explore alternatives—is key to successful software design.
Whether you’re a seasoned programmer or a tech enthusiast, mastering it can enhance your ability to create efficient and adaptable software. By leveraging its strengths and recognizing its limitations, you can create programs that are both robust and scalable.
Frequently Asked Questions
1. What are the main benefits of using inheritance in software design?
Inheritance allows for code reusability, extensibility, and organization. By enabling subclasses to inherit attributes and behaviors from parent classes, it reduces redundancy and enhances the maintainability of software.
2. When should inheritance be avoided in programming?
Inheritance should be avoided when it leads to excessive coupling between classes, when the relationship isn’t genuinely hierarchical, or when it’s used merely as a shortcut for code reuse without considering alternatives like composition or interfaces.
3. How do inheritance and polymorphism work together?
Inheritance and polymorphism are interconnected; it creates a hierarchical structure, while polymorphism allows objects to be treated as instances of their parent class. This combination enables a unified interface for different subclass implementations, providing flexibility and adaptability in software design.
4. What are some real-world use cases for inheritance?
Inheritance is widely used in domains such as banking systems and game development. For instance, a base “BankAccount” class can be extended into specialized account types, or a “Character” class can be expanded to various characters in a game, allowing shared attributes and behaviors.
5. What role does inheritance play in design patterns?
Inheritance is integral to many design patterns, such as the Template Method and Factory Method patterns. These patterns rely on it to define algorithms’ structures or delegate object creation to subclasses, promoting flexibility and elegant solution design.
6. What are some alternatives to inheritance?
Interfaces and composition are viable alternatives to inheritance. Interfaces allow for shared behavior without implementation details, while composition involves combining smaller components to build classes, promoting loose coupling and better modularity.
7. How can I determine whether to use inheritance or an alternative solution?
Consider the specific needs and design goals of your software. If the relationship is hierarchical and benefits from shared implementations, It might be suitable. If flexibility, minimized coupling, or unique class implementations are priorities, explore interfaces or composition.
Additional Resources for Further Learning
If you’re interested in expanding your knowledge of inheritance and its alternatives in software design, consider exploring the following online resources:
- Khan Academy’s Introduction to Object-Oriented Programming – A beginner-friendly course that covers the fundamentals of object-oriented programming, including inheritnce.
- Coursera’s Object-Oriented Programming in Java – A comprehensive course that dives deeper into OOP concepts and design patterns.
- GeeksforGeeks Inheritance in C++ – A detailed guide on inheritnce in C++, covering various types and use cases.
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides – While this is a book rather than a website, it is an essential resource for anyone looking to master design patterns, including those that utilize inheritnce.
- Stack Overflow – A community-driven Q&A platform where you can find discussions and clarifications on inheritance-related topics.
By exploring these resources, you can deepen your understanding of how inheritnce and its alternatives can be effectively utilized in software design.