In the world of programming, data storage and retrieval are foundational skills. Yet, how you manage your data can significantly impact the efficiency and readability of your code. Enter Python dictionaries—a powerhouse of Python data structures that offer unmatched flexibility and efficiency. Whether you’re a seasoned software engineer, a budding Python developer, or a data analyst looking to refine your skills, mastering dictionaries is essential. This blog post will guide you through the essentials of Python dictionaries, from understanding key-value pairs to optimizing their performance in your projects.
Introduction to Key-Value Pairs and Their Significance in Programming
A basic idea in computer science, key-value pairs allow for effective data storing and retrieval.
You can quickly access and manipulate data by assigning a unique key to a specific value. Key-value pairs are prevalent in various programming scenarios, from database management to configuration files.
The efficiency and simplicity of key-value pairs are what make them significant. Unlike other data structures, where access to an element could involve traversing multiple nodes or indices, key-value pairs allow direct access to the data. This makes them ideal for applications that require fast lookups, such as caching, indexing, and user session management.
Understanding key-value pairs also paves the way for mastering dictionaries in Python, as these pairs form the core of dictionary operations. By grasping this concept, you’ll be better equipped to leverage the full potential of Python dictionaries in your projects.
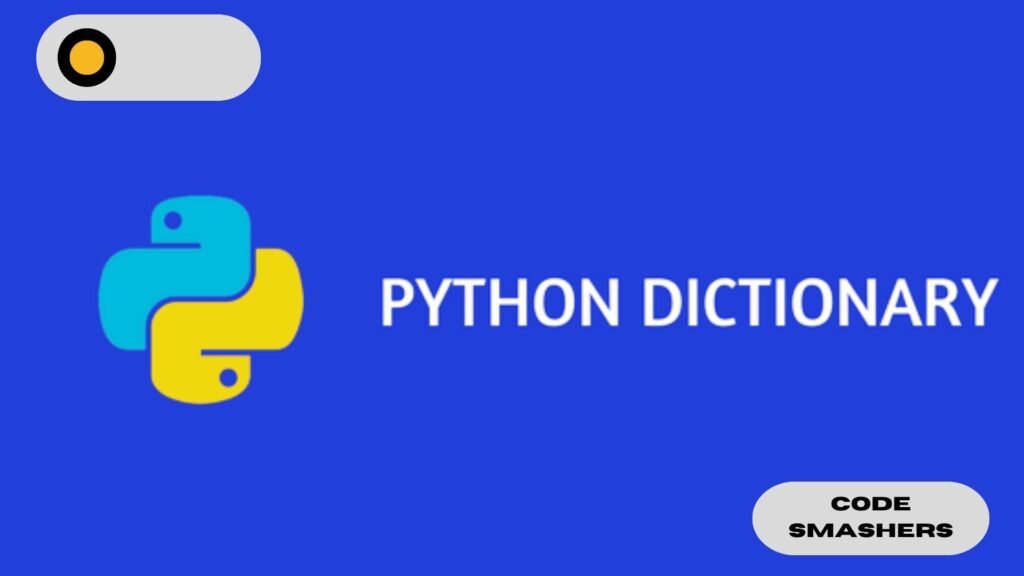
Overview of Dictionaries in Python: A Powerful Data Structure
Python dictionaries are a built-in data structure that uses key-value pairs to store and organize data efficiently. They can have their contents changed after they are created since they are malleable. This flexibility makes dictionaries suitable for various applications, from simple data storage to complex data manipulation tasks.
The power of dictionaries lies in their ability to provide constant time complexity for lookups, insertions, and deletions. This efficiency is achieved through hash functions, which map keys to specific memory locations. Python’s implementation of dictionaries ensures that even with large datasets, accessing or modifying data remains fast and efficient.
By understanding how dictionaries work under the hood, you can appreciate their versatility and robustness. Whether you’re managing configurations, implementing caches, or processing large datasets, dictionaries offer a reliable solution for efficient data handling.
Exploring the Syntax and Basic Operations of Dictionaries
Working with dictionaries in Python is straightforward, thanks to its intuitive syntax. Here’s a quick overview of how to create and manipulate dictionaries:
Creating a Dictionary
To create a dictionary, use curly braces `{}` with key-value pairs separated by a colon `:`. For example:
my_dict = {
“name”: “Alice”,
“age”: 30,
“city”: “New York”
}
In this example, `name`, `age`, and `city` are keys, while `”Alice”`, `30`, and `”New York”` are their corresponding values.
Accessing Elements
You can access dictionary elements using their keys. For instance:
print(my_dict[“name”]) # Output: Alic“`
Python will raise a `KeyError` if you try to access a non-existent key.
Adding and Modifying Elements
Adding or updating elements in a dictionary is simple:
my_dict[“email”] = “alice12@example.com” # Adding a new key-value pair
my_dict[“age”] = 31 # Updating an existing key-value pair
Deleting Elements
To remove elements, use the `del` statement or the `pop` method:
del my_dict[“cities”] # Deletes the key-value pair with key “cities.”
email = my_dict.pop(“email”) # Removes “email” and returns its value
These basic operations form the foundation for working with dictionaries in Python. By mastering these, you can perform more complex data manipulations with ease.
an perform more complex data manipulations with ease.
Use Cases of Dictionaries in Real-World Applications
Dictionaries are incredibly versatile and find applications across various domains. Here are some common use cases:
Data Storage and Retrieval
In web development, dictionaries are often used to manage user sessions. By storing session data in a dictionary, you can quickly access and update user information based on session IDs.
Configuration Management
Many applications use dictionaries to store configuration settings. This allows easy access and modification of configuration parameters, making your code more flexible and maintainable.
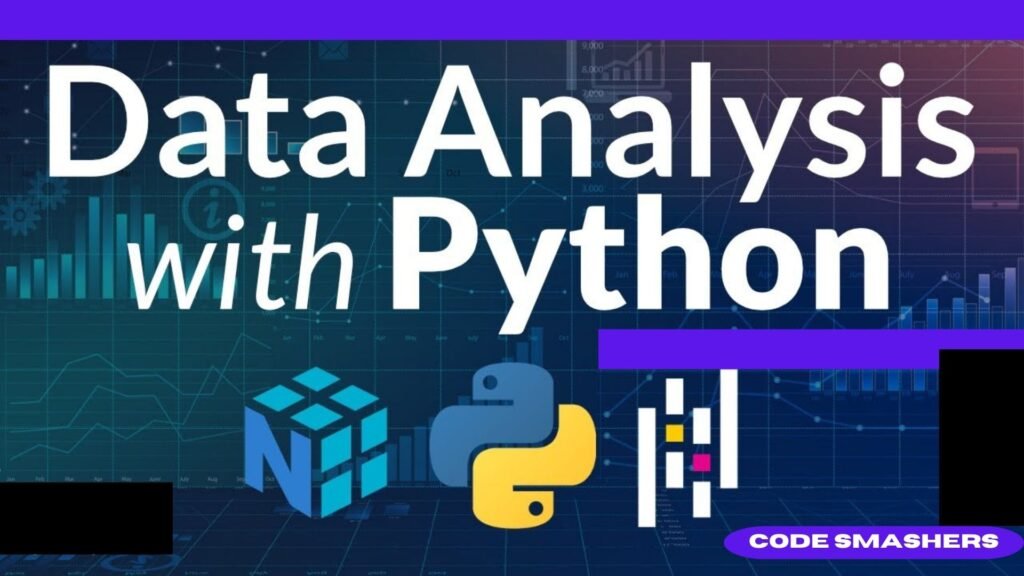
Data Analysis
Data analysts frequently use dictionaries to process and analyze large datasets. For example, you can create dictionaries to store and count occurrences of unique items, making it easier to generate frequency distributions and other statistical analyses.
By exploring these use cases, you can see how dictionaries simplify data handling, making your code more efficient and readable.
Comparing Dictionaries with Other Data Structures in Python
While dictionaries are powerful, it’s essential to understand how they compare with other Python data structures like lists, sets, and tuples.
Lists
Lists are ordered collections of elements that allow duplicate items. While they are great for sequential data, accessing elements involves traversing the list, making them less efficient for frequent lookups.
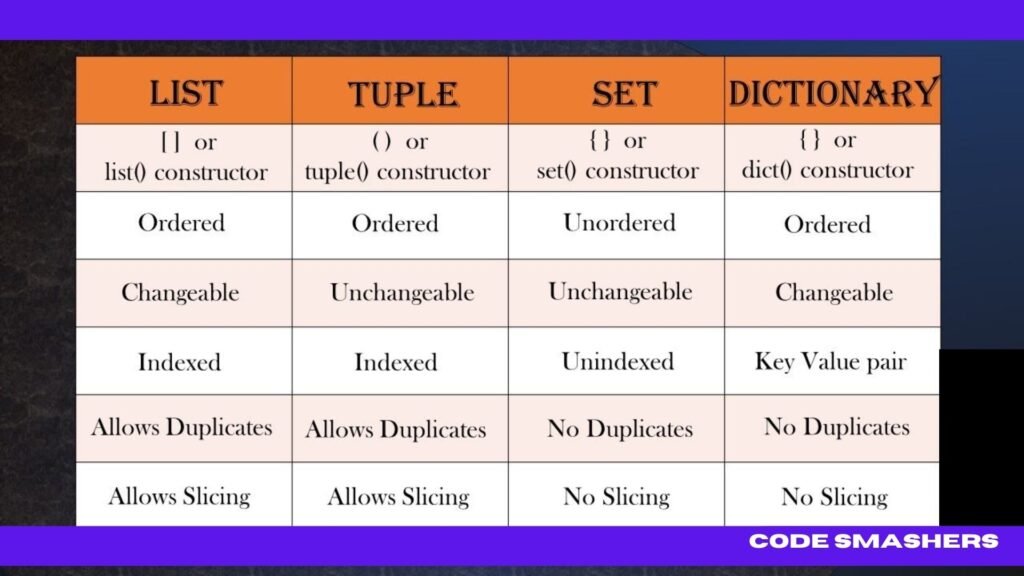
Sets
Sets do not permit key-value pairs; instead, they are unordered collections of unique items.
Sets are suitable for cases where you need to manage unique items without caring about the order.
Tuples
Tuples are immutable ordered collections of elements. They are useful for fixed-size collections but lack the flexibility of dictionaries for data modification and retrieval.
Understanding these differences helps you choose the right data structure for your specific use case, maximizing efficiency and readability in your code.
Best Practices for Efficient Use of Dictionaries and Common Mistakes to Avoid
To make the most of dictionaries, follow these best practices:
Use Meaningful Keys
Choose keys that are descriptive and meaningful. This improves code readability and reduces the likelihood of errors.
Avoid Nested Dictionaries
While nesting dictionaries can be powerful, it can also make your code harder to read and maintain. Use nested dictionaries sparingly and consider alternative data structures if necessary.
Handle Missing Keys Gracefully
Instead of allowing your code to throw `KeyErrors`, use the `get` method or `defaultdict` from the `collections` module to handle missing keys gracefully.
By adhering to these best practices, you can write more efficient and maintainable code, avoiding common pitfalls that can lead to errors and inefficiencies.
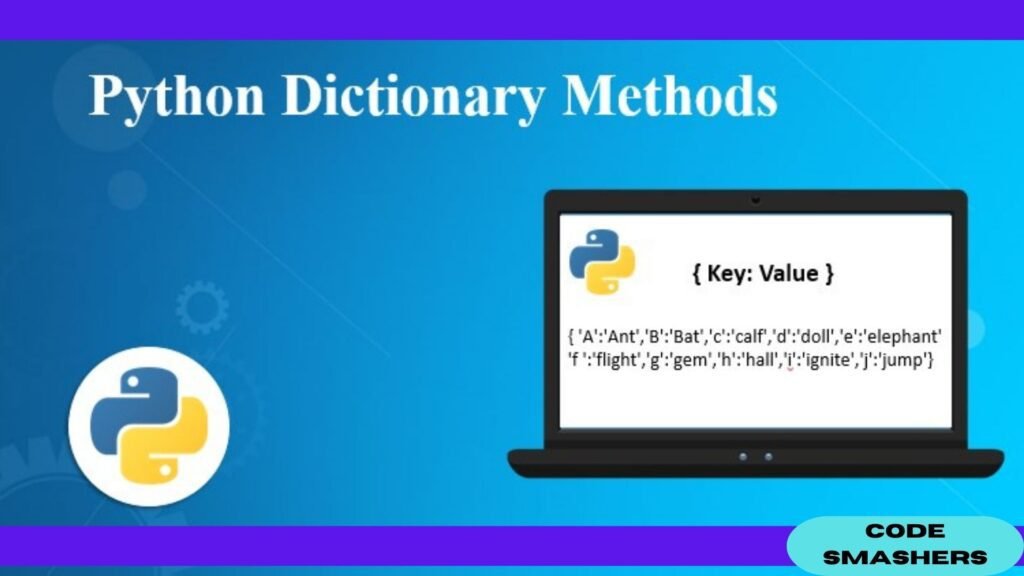
Tips for Optimizing Dictionary Performance in Python Programs
Performance optimization is crucial when working with large datasets. Here are some tips to enhance dictionary performance:
Use Appropriate Hash Functions
Python’s default hash function works well in most cases, but for custom objects, implementing an efficient `hash` method can improve performance.
Minimize Key Collisions
Key collisions occur when multiple keys have the same hash value. Use unique and diverse keys to minimize collisions and maintain dictionary performance.
Preallocate Dictionaries
If you know the expected size of your dictionary, preallocate it using the `defaultdict` or `dict.fromkeys` method. This reduces the overhead associated with dynamic resizing.
By implementing these optimization strategies, you can ensure that your dictionaries perform efficiently, even with large datasets.
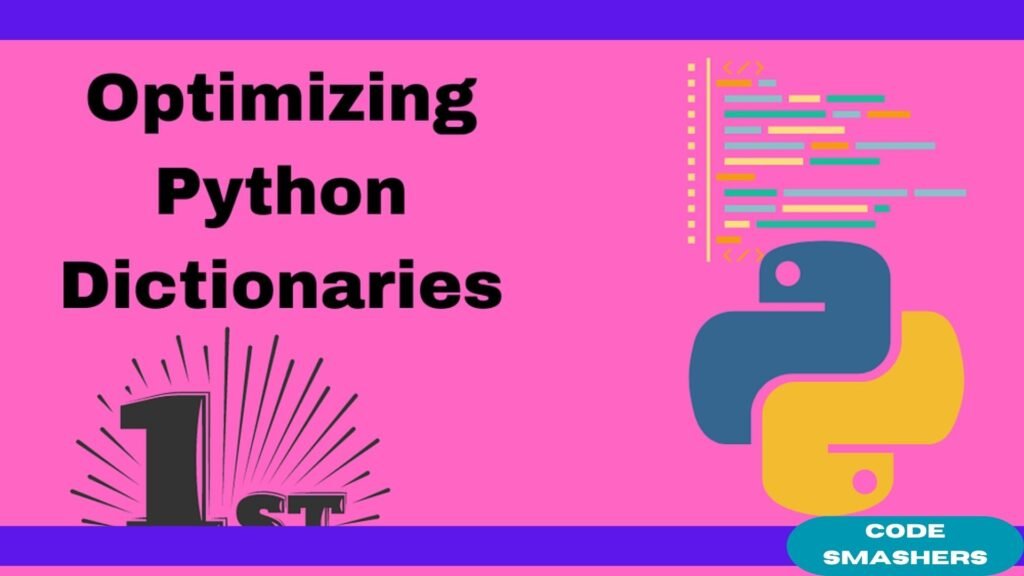
Conclusion
Understanding Python dictionaries is crucial for efficient data handling in modern programming. From basic operations to advanced optimization techniques, mastering dictionaries empowers you to write more efficient and readable code. Whether managing configurations, processing data, or developing web applications, dictionaries offer a robust solution for your data storage and retrieval needs.
To further enhance your skills, explore additional resources and practice using dictionaries in your projects. By doing so, you’ll unlock the full potential of Python’s powerful data structures, positioning yourself as a proficient and efficient programmer.
Dive into the world of Python dictionaries and elevate your coding prowess today!