Introduction to Control Flow
Concerning programming, control flow is the secret sauce that makes your code intelligible and functional. Whether you’re a beginner in Python or a tech enthusiast looking to deepen your skills, understanding control flow is essential. It determines the direction in which your program will execute and how it will react to various conditions and inputs.
In this blog, we’ll break down Python’s control flow mechanisms, offering in-depth insights into various statements and best practices. You’ll discover how to use control flow effectively to develop Python programs that are more legible and efficient.
By the end of this guide, you’ll have a solid grasp of control flow, from basic if statements to more complex decision-making logic. Let’s jump in!
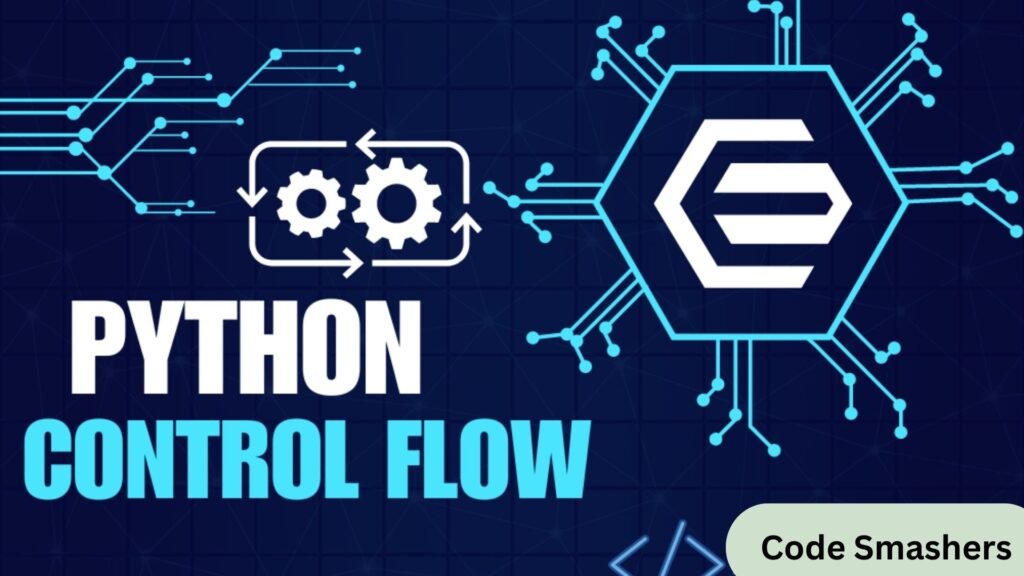
What is Control Flow in Programming?
The sequence in which certain commands, statements, or function calls are carried out within a script or program is known as the control flow. Function calls, loops, and conditional statements are examples of control flow structures in Python. These constructs enable developers to manage the execution path of the code based on different conditions.
Understanding control flow is crucial because it allows you to:
- Make decisions within your code
- Repeatedly execute blocks of code
- Simplify complex logical operations
- Handle errors and exceptions
Control flow gives you the power to write efficient and flexible programs that can handle various scenarios and user inputs.
Python has a straightforward and flexible syntax for control flow, making it suitable for building complex and scalable applications. Let’s look at the different types of control flow statements in Python.
Conditional Statements
Conditional statements allow you to execute specific blocks of code only when certain conditions are met. Their foundation consists of Boolean expressions with a true or false evaluation.
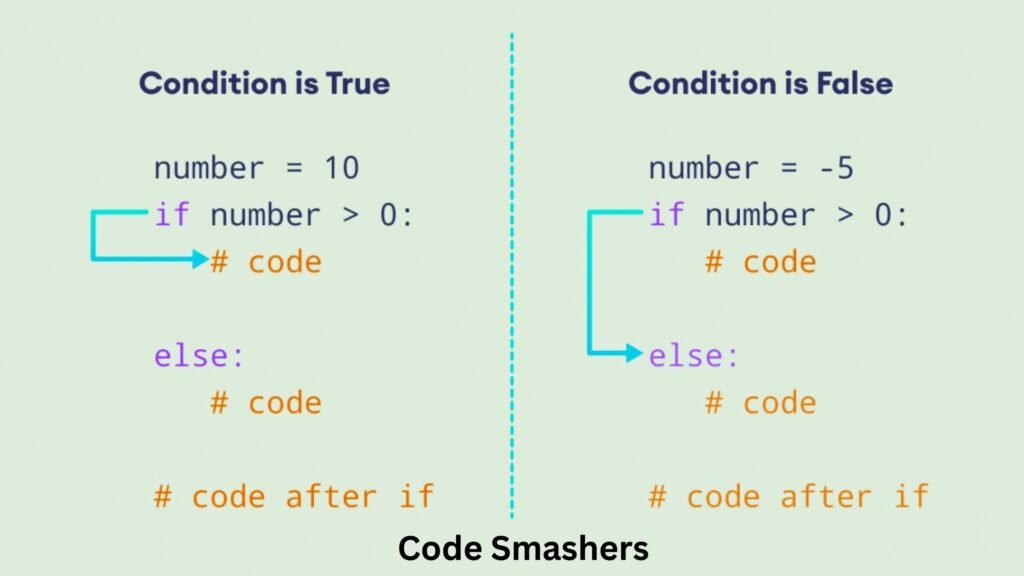
Python’s if, elsif, and else clauses are used to create conditional statements. An if statement is structured as follows:
python
if condition:
# Code block to be executed if the condition evaluates to True
Here’s an example of how we can use an if statement
Python control flow structures are intuitive and easy to read, making them ideal for both beginners and experienced developers.
Python If Statements
Syntax and Basics
The if statement is the building block of control flow in Python. It evaluates a condition and executes a block of code only if that condition is true. The syntax is straightforward:
if condition:
# code to execute if condition is true
For example:
age = 18
if age >= 18:
print(“You are eligible to vote.”)
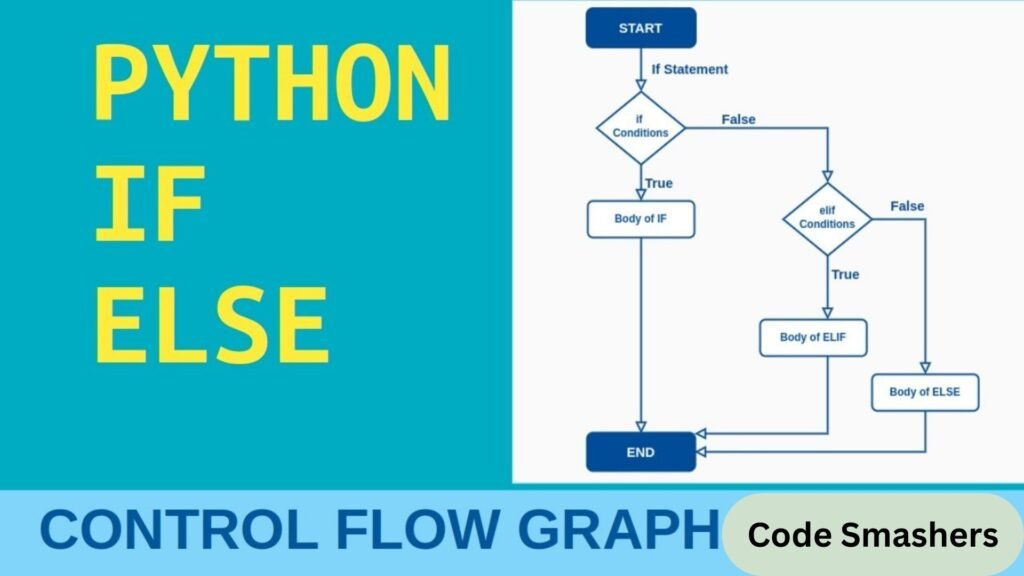
This straightforward if statement determines whether the age variable is equal to or greater than 18 and, in the event that it is, produces a message.
Practical Examples
To see if statements are in action, consider this scenarios.
temperature = 30
if temperature > 25:
print(“It’s a hot day!”)
In this example, the program checks the temperature and prints a message if it’s over 25 degrees.
Best Practices
When using if statements, always ensure your conditions are clear and concise. Avoid overly complex conditions that can make your code difficult to read. Additionally, use comments to explain the purpose of each if statement, especially in larger scripts.
Else Statements
Complementing If Statements
Else statements provide an alternative path for code execution when the if condition is not met. They work with if statements to handle scenarios where the condition is false.
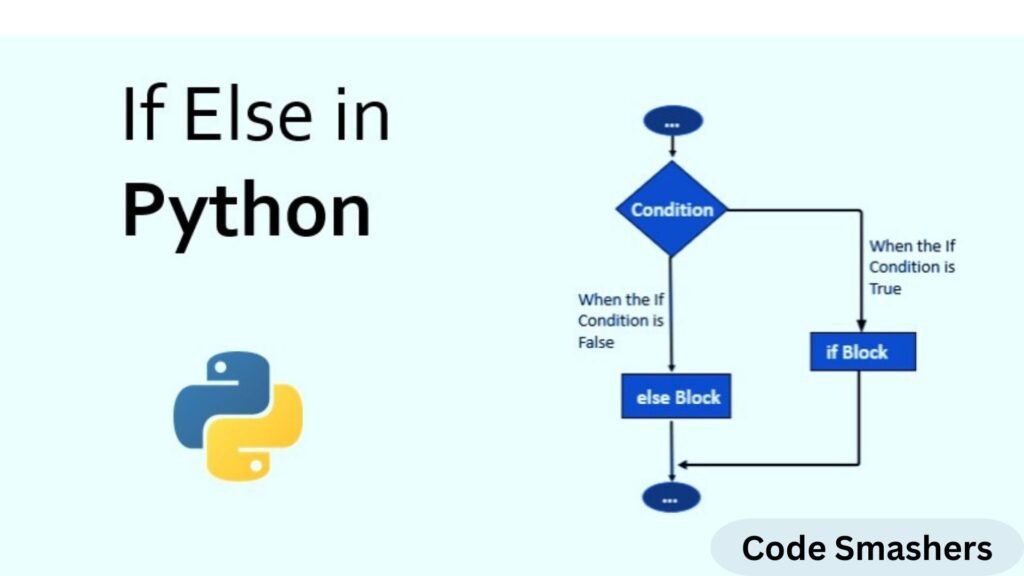
Syntax and Usage
The syntax for else statements is as follows:
if condition:
# code if condition is true
else:
# code if condition is false
For example:
age = 16
if age >= 18:
print(“You are eligible to vote.”)
else:
print(“You are not eligible to vote.”)
“`
Here, the else statement ensures a message is printed regardless of whether the condition is true or false.
Practical Examples
Consider a login system:
“`
password = “secure123”
if password == “secure123”:
print(“Access granted.”)
else:
print(“Access denied.”)
“`
In this case, the else clause provides an alternative action when the password does not match.
Best Practices
Use else statements to handle default actions or catch cases where the condition might fail. Ensure that the logic within else blocks is relevant to the context of the if condition.
Elif Statements
Adding More Conditions
Elif, short for “else if,” allows you to add multiple conditions to your control flow. This is particularly useful when you need to evaluate several conditions sequentially.
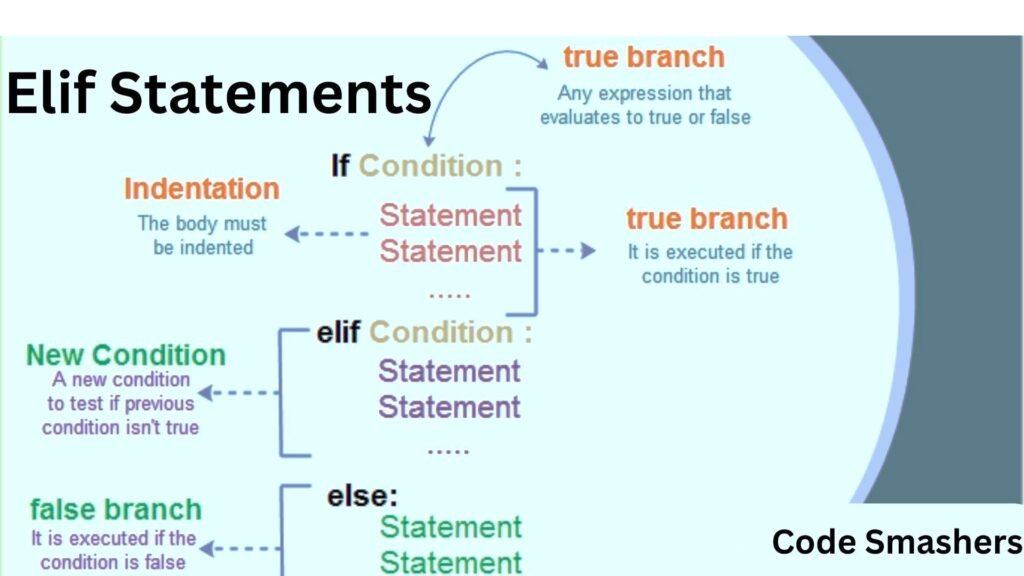
Syntax and Usage
Elif statements follow this syntax:
“`
if condition:
# code if condition1 is true
elif condition2:
# code if condition2 is true
else:
# code if both conditions are false
“`
For example:
“`
score = 85
if score >= 90:
print(“Grade A”)
elif score >= 80:
print(“Grade B”)
else:
print(“Grade C”)
“`
This snippet evaluates the score and assigns a grade based on predefined ranges.
Practical Examples
Imagine a traffic light system:
“`
light = “green”
if light == “red”:
print(“Stop”)
elif light == “yellow”:
print(“Slow down”)
else:
print(“Go”)
“`
Here, the elif statement helps manage multiple traffic light conditions.
Best Practices
When using elif statements, order your conditions logically. You ought to put the most likely conditions first to optimize performance. Also, limit the number of elif statements to keep your code readable.
Combining Control Flow Statements
Complex Decision Making
Combining if, else, and elif statements allows you to create intricate decision-making logic in your programs. This is crucial for handling real-world scenarios that require multiple conditions to be evaluated.
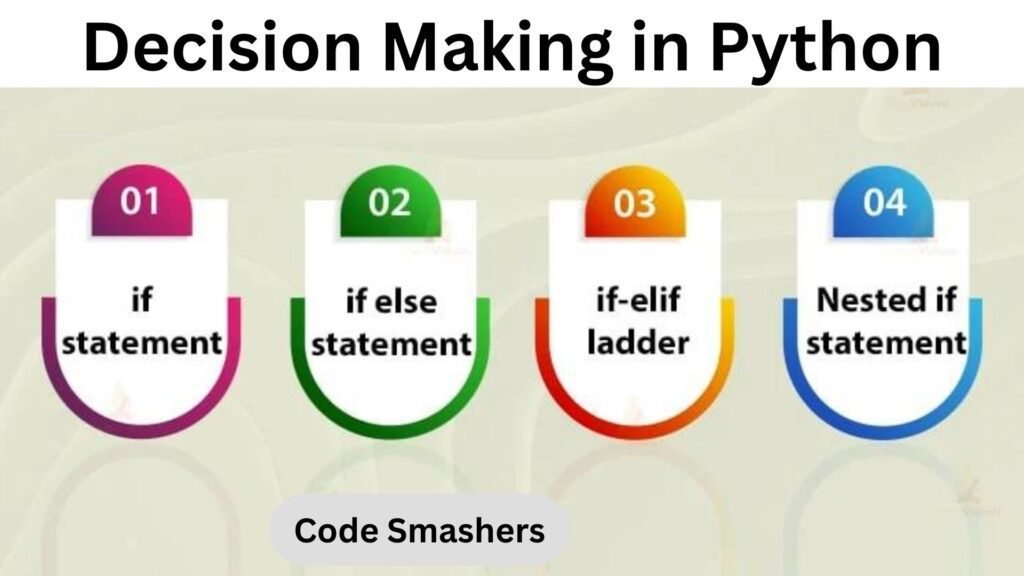
Practical Examples
Consider a grading system with additional conditions:
“`
score = 75
attendance = 90
if score >= 90 and attendance >= 85:
print(“Grade A”)
elif score >= 80 and attendance >= 85:
print(“Grade B”)
elif score >= 70 and attendance >= 80:
print(“Grade C”)
else:
print(“Grade D”)
“`
This example combines conditions using logical operators to determine the final grade.
Best Practices
When combining control flow statements, aim for clarity and readability. Use parentheses to group conditions and avoid nesting too many levels of if-else statements. This practice makes your code easier to debug and maintain.
Real-World Examples
Solving Common Problems
Applying control flow statements in real-world scenarios helps solidify your understanding. Here are a few examples:
Example 1: Temperature Converter
“`
temp = input(“Enter temperature (e.g., 45F, 102C): “)
degree = int(temp[:-1])
unit = temp[-1]
if unit.upper() == “F”:
celsius = (degree – 32) * 5/9
print(f”The temperature in Celsius is {celsius:.2f}C”)
if unit.upper() == “C”:
Fahrenheit = (degree * 9/5) + 32
print(f”The temperature in Fahrenheit is {fahrenheit:.2f}F”)
else:
print(“Invalid unit”)
“`
This script converts temperatures between Fahrenheit and Celsius based on user input.
Example 2: Simple Calculator
“`
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
operator = input(“Enter operator (+, -, *, /): “)
if operator == ‘+’:
print(f”Result: {num1 + num2}”)
elif operator == ‘-‘:
print(f”Result: {num1 – num2}”)
elif operator == ‘*’:
print(f”Result: {num1 * num2}”)
elif operator == ‘/’:
if num2 != 0:
print(f”Result: {num1 / num2}”)
else:
print(“Error! Division by zero.”)
else:
print(“Invalid operator”)
“`
This example demonstrates a basic calculator that performs different arithmetic operations based on user input.
Example 3: Loan Eligibility Checker
“`
income = int(input(“Enter your annual income ($): “))
credit_score = int(input(“Enter your credit score: “))
if income >=50,000 and credit score >=$700:
print(“You are eligible for the loan.”)
If income >= $50,000 and credit score < 700:
print(“Improve your credit score to become eligible.”)
If income < $50,000 and credit score >$700:
print(“Increase your income to become eligible.”)
else:
print(“You are not eligible for the loan.”)
“`
This script assesses loan eligibility based on income and credit score.
Best Practices and Pitfalls
Writing Efficient Code
Mastering control flow involves not only knowing how to use the statements but also how to write efficient, readable code.
Use Clear and Concise Conditions
Always opt for clear and concise conditions. Avoid trying to pack too many logical operations into a single line of code. For easier reading, divide complicated circumstances into simpler ones.
Avoid Deep Nesting
Deeply nested control flow statements can make your code difficult to read and maintain. Instead of nesting multiple levels of if-else statements, consider using functions or breaking the logic into smaller, manageable pieces. This approach helps reduce complexity and makes your code more modular.
Handle All Possible Outcomes
Always consider all possible outcomes in your conditions, even those that seem unlikely. For example, when dealing with user inputs, handle potential invalid inputs to avoid unexpected errors.
Understanding Truth Values
In control flow statements, truth values are used to evaluate conditions and determine which code branches execute The following values are regarded as “False” in Python:
- False bool object
- None type object
• Zero, any kind of numeric (0, 0.0)
• Any mapping or sequence that is empty (“”, [], {})
Any other value is considered “True”.
“`
Examples of “False” values
if
Comment Your Code
Although Python control flow statements are intuitive, adding comments to explain your logic can be beneficial, especially for larger scripts. This practice helps others (and future you) understand the purpose behind each condition.
Common Mistakes to Avoid
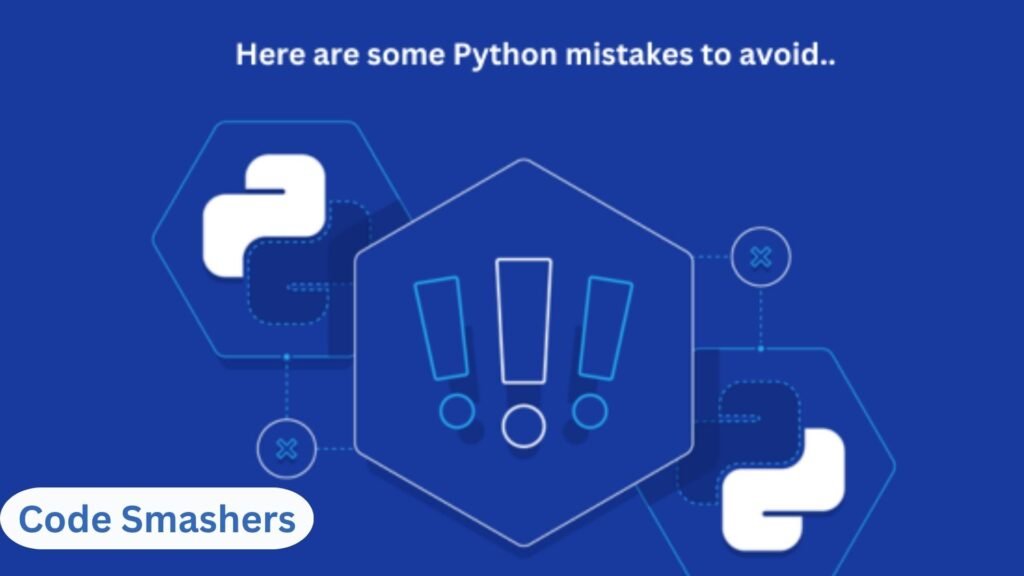
Forgetting the Else Clause
While if and elif statements can handle multiple conditions, forgetting the else clause can lead to unhandled cases. Always include an else statement to cover all possible scenarios.
Overusing Elif Statements
Your code may become clumsy if it has an excessive number of elif statements. Look for opportunities to simplify your logic or use dictionaries for specific cases, such as mapping user inputs to functions.
Mixing Logical Operators
Mixing logical operators like “and” and “or” in the same condition can produce unexpected results. To aggregate conditions and increase readability, always use parentheses.
Ignoring Edge Cases
When writing control flow statements, consider edge cases and how your program should handle them. Testing your code with various inputs helps identify potential issues early on.
Conclusion
Mastering Python’s control flow is essential for anyone looking to write efficient and readable code. Through the proper use of if, else, and if statements, you can effectively manage complex conditions and decision-making processes. By understanding these constructs and implementing best practices, you’ll be well-equipped to tackle real-world programming problems.
Remember, practice makes perfect. The more you experiment with Python control flow, the more confident you’ll become in your coding abilities.
If you’re eager to take your skills further, engage with the community, share your experiences, and continue learning. Happy coding!