The foundation of the Python programming language is its lists. They are versatile, powerful, and easy to use, making them a favorite among beginners and experts. Whether you’re just starting or looking to deepen your understanding, mastering Python lists is essential for efficient coding. In this comprehensive guide, we’ll explore everything you need to know about Python lists—from basic operations to advanced techniques.
Introduction to Python Lists
Python lists are a built-in data type that allows you to store collections of items in a single variable. They can hold items of different types, including strings, integers, and even other lists. This makes them incredibly flexible and useful for a variety of programming tasks.
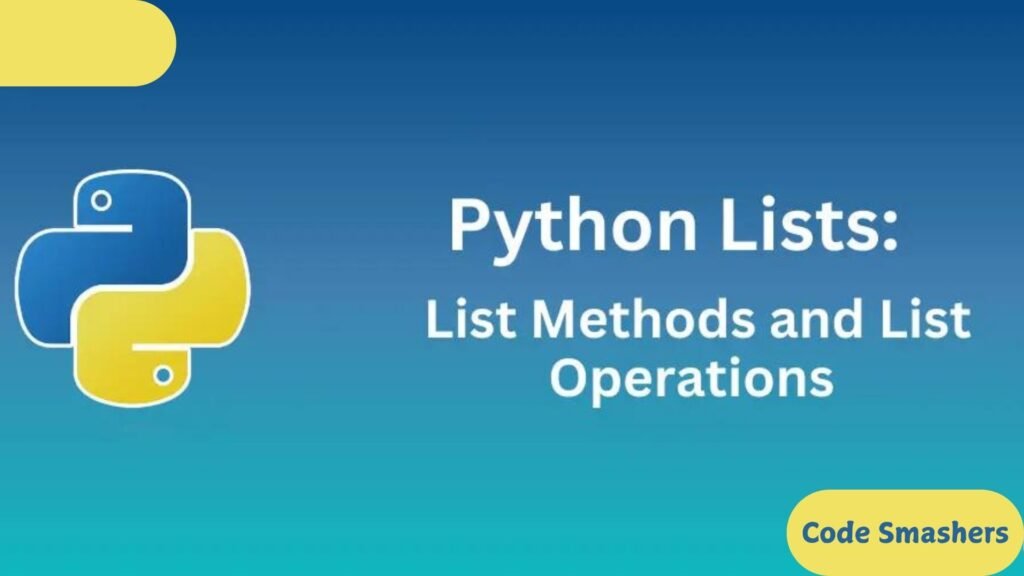
Lists are ordered, meaning the items have a defined sequence, and this sequence will not change unless you explicitly modify the list. Lists are also mutable, allowing you to change their content without changing their identity. This makes lists particularly useful for tasks that involve dynamic data.
In this blog post, we’ll cover the basics of Python lists, explore common operations and built-in methods, and offer some best practices to help you make the most out of this versatile data structure.
Understanding the Basics of Python Lists
Before we dive into the more complex operations and methods, it’s important to understand the basics of Python lists. Creating a list is straightforward; simply enclose your items in square brackets `[]`.
For example:
my_list = [4, 5, 6, ‘c’, ‘d’, ‘e’]
In this list, we have both integers and strings. The list’s elements can be accessed by using their index, which begins at 0.
For example:
print(my_list[0]) # Outputs 4
print(my_list[3]) # Outputs ‘c’
Lists can also contain other lists, allowing you to create nested lists for more complex data structures.
For example:
nested_list = [3, [5, 7], [8, 8, 9]]
Understanding these basics will set the foundation for more advanced operations and methods.
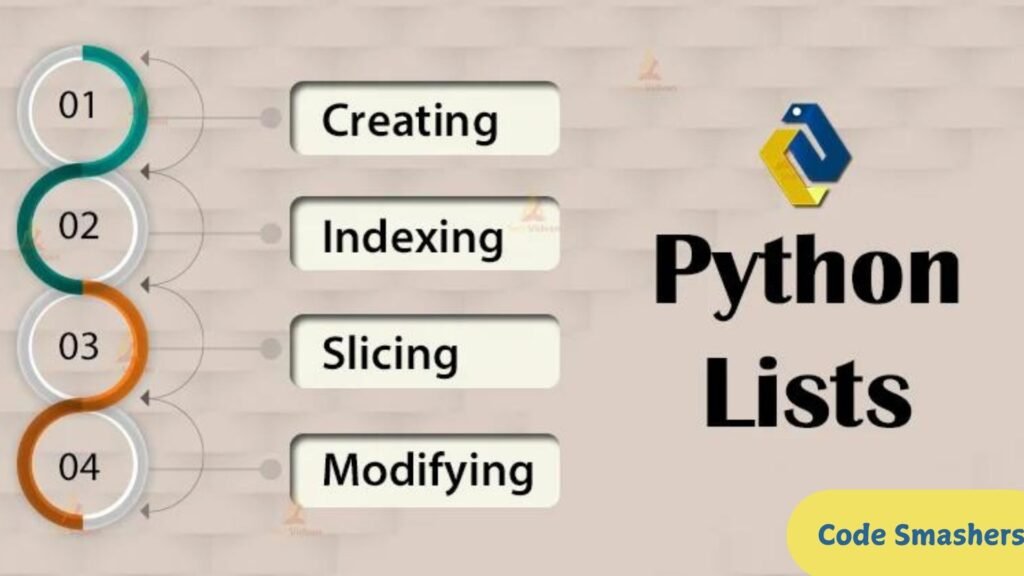
Common Operations with Python Lists
Indexing and Slicing
Indexing and slicing are the most common operations you’ll perform on lists. Indexing allows you to access individual elements, while slicing lets you access a subset of lists.
For example:
my_list = [1, 2, 3, 4, 5]
print(my_list[1]) # Outputs 2
print(my_list[1:4]) # Outputs [2, 3, 4]
Slicing uses a start and end index, `my_list[start: end]`, and includes the start index but excludes the end index.
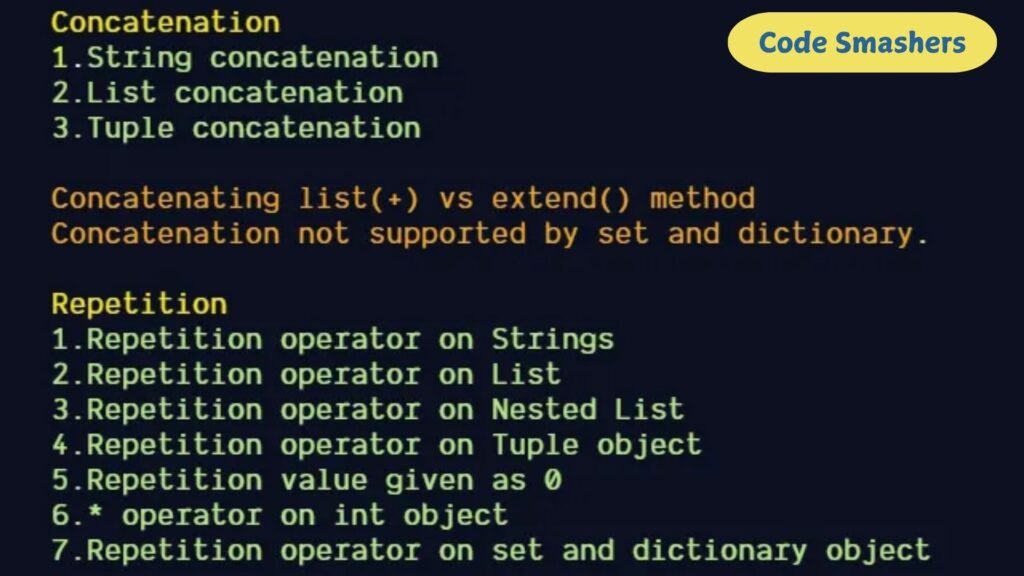
Concatenation and Repetition
Concatenation and repetition are useful for combining lists or repeating elements. You can concatenate lists using the `+` operator and repeat elements using the `*` operator.
For example:
list1 = [3, 6, 5]
list2 = [6, 7, 8]
combined_list = list1 + list2 # Outputs [3, 6, 5, 6, 7, 8]
repeated_list = list1 * 2 # Outputs [3, 6, 5, 3, 6, 5]
These operations are particularly insightful for initializing lists or combining data from multiple sources.
Length and Membership
The `len()` function and the `in` keyword are essential for working with lists. The `len()` function returns the number of items in the list, while `in` checks for membership.
For example:
my_list = [1, 2, 3, 4, 5]
print(len(my_list)) # Outputs 5
print(3 in my_list) # Outputs True
Functions can help you manage and validate the data in your lists more effectively.
Overview of Built-in List Methods
Append, Extend, and Insert
Python provides several built-in methods for modifying lists. The `append()` method adds an item to the end of the list, `extend()` adds multiple items, and `insert()` allows you to add an item at a specific position.
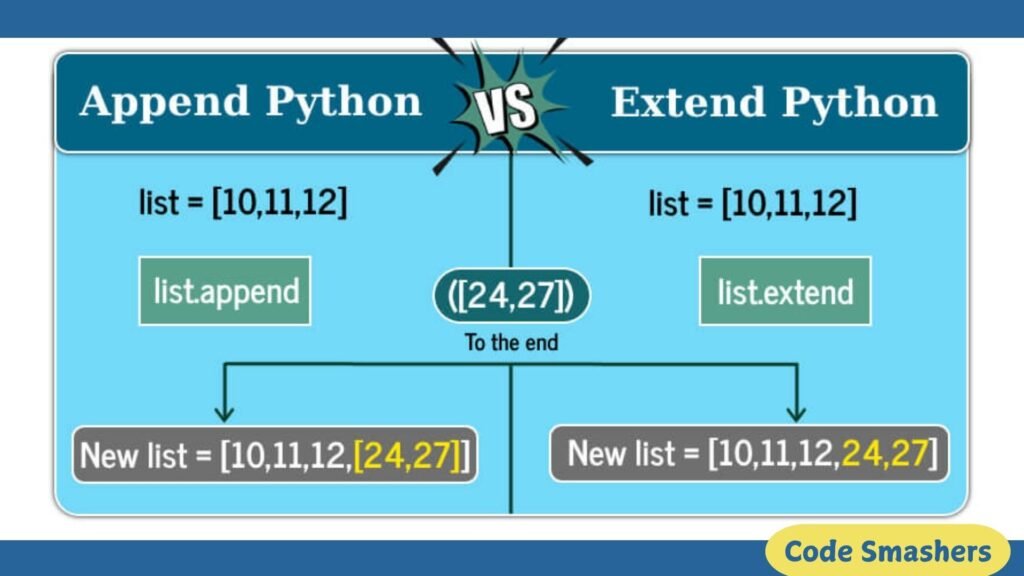
For example:
my_list = [1, 2, 3]
my_list.append(4) # Outputs [1, 2, 3, 4]
my_list.extend([5, 6]) # Outputs [1, 2, 3, 4, 5, 6]
my_list.insert(1, ‘a’) # Outputs [1, ‘a’, 2, 3, 4, 5, 6]
These methods are invaluable for dynamically modifying the contents of your lists.
Remove, Pop, and Index
The `remove()` method deletes the first occurrence of a specified value, `pop()` removes an item by index and returns it, and `index()` finds the position of an item.
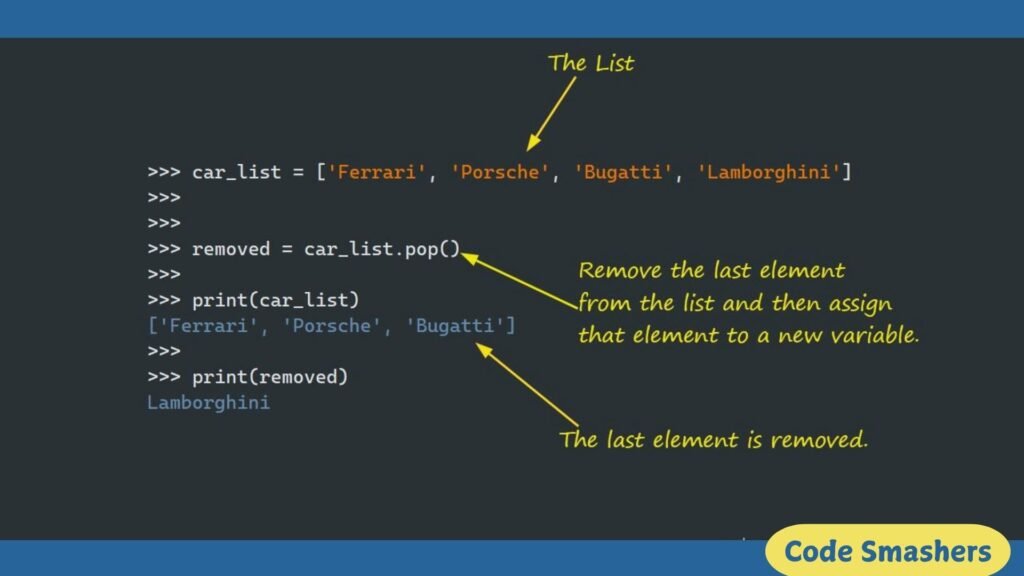
For example:
my_list = [1, 2, 3, 4, 5]
my_list.remove(3) # Outputs [1, 2, 4, 5]
print(my_list.pop(1)) # Outputs 2: my_list becomes [1, 4, 5]
print(my_list.index(4)) # Outputs 1
“`
These methods provide a robust way to manage and interact with the elements in your list.
Sort and Reverse
Sorting and reversing lists are common operations that can be performed using the `sort()` and `reverse()` methods. `sort()` arranges the list in ascending order by default, while `reverse()` reverses the order of the list.
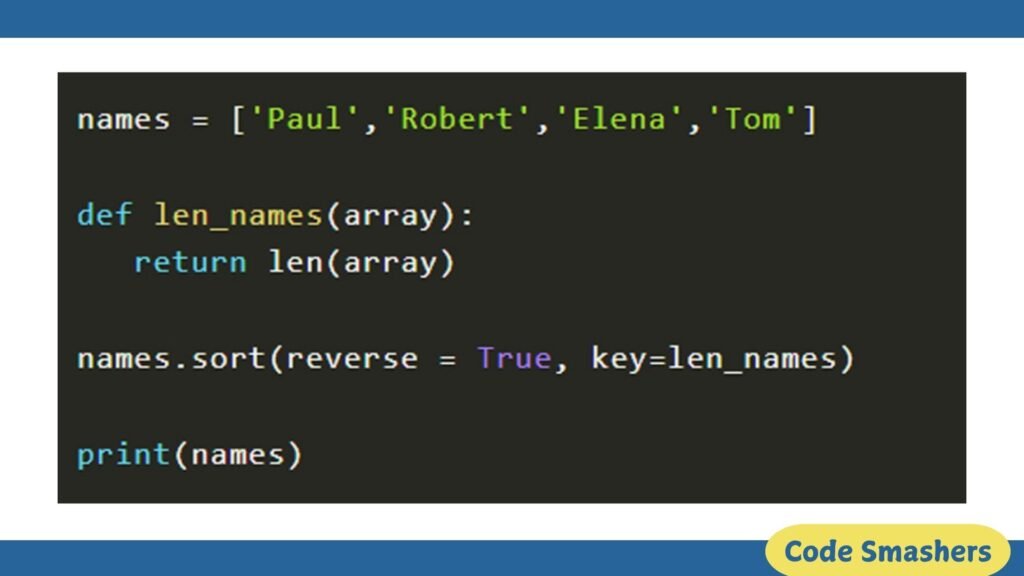
For example:
my_list = [3, 1, 4, 2, 5]
my_list.sort() # Outputs [1, 2, 3, 4, 5]
my_list.reverse() # Outputs [5, 4, 3, 2, 1]
These methods are especially insightful when you prepare your data for processing or display.
Advanced List Techniques
List Comprehensions
List comprehensions provide a brief way to create lists. They can replace the need for loops and make your code more readable.
For example:
squares = [x**2 for x in range(10)] # Outputs [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Conditional statements are another tool that list comprehensions can use to filter items.
For example:
even_squares = [x**2 for x in range(10) if x % 2 == 0] # Outputs [0, 4, 16, 36, 64]
Using list comprehensions can make your code more efficient and easier to understand.
Nested Lists
Nested lists allow you to create complex data structures by embedding lists within other lists. This is particularly useful for representing matrices or tables.
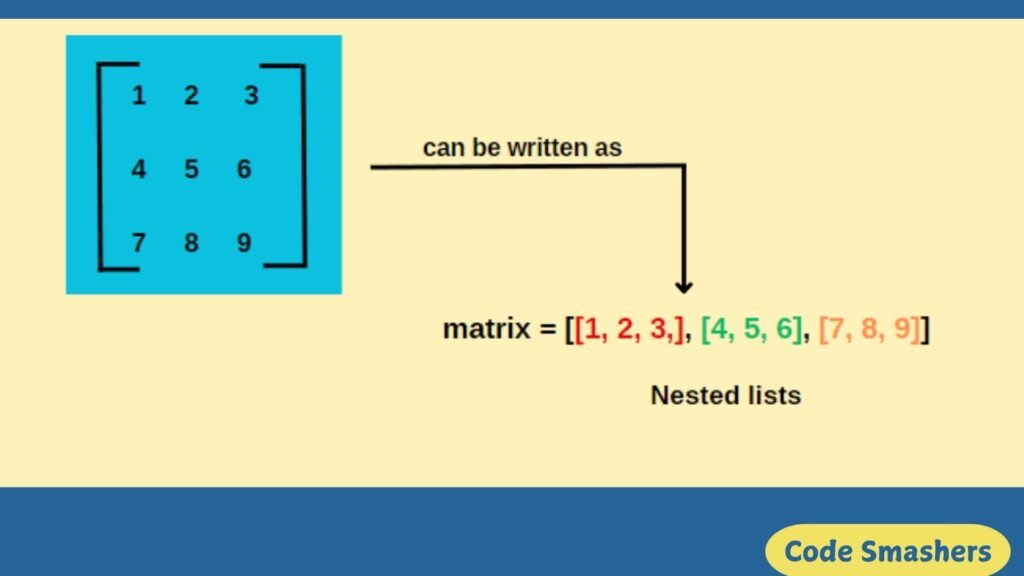
For example
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(matrix[1][2]) # Outputs 6
Working with nested lists can be challenging, but they offer powerful ways to organize and manipulate your data.
Best Practices for Working with Python Lists
Efficiency and Memory Management
When working with large lists, it’s important to consider efficiency and memory management. Using list comprehensions can be more efficient than loops, and built-in methods are usually optimized for performance.
For example:
large_list = [i for i in range(1000000)]
Be mindful of memory usage, especially when working with nested lists or lists of large objects.
Error-Handling and List Integrity
Ensuring the integrity of your lists is crucial for maintaining data accuracy. Always validate inputs before adding them to a list and handle exceptions gracefully.
For example:
try:
my_list.remove(‘nonexistent’)
except ValueError:
print(“Item not found in the list”)
Implementing error-handling practices can save you from unexpected crashes and data corruption.
Conclusion and Next Steps
Recap of Key Points
In this blog post, we’ve explored the essentials of Python lists. From basic operations like indexing and slicing to advanced techniques like list comprehensions and nested lists, mastering these skills will make you a more efficient and effective programmer.
Suggested Projects for Practice
To solidify your understanding, consider working on projects that involve extensive use of lists. For example, you could create a program to manage a to-do list, build a simple inventory system, or even develop a basic data analysis tool.
Resources for Further Learning
There are a lot of resources available for those who wish to explore Python lists further. Websites like Real Python, tutorials on YouTube, and Python’s official documentation are excellent places to start.