In the realm of JavaScript, Asynchronous javascript programming is a fundamental skill that every developer needs to master. From handling user inputs to fetching data from APIs, understanding how to manage asynchronous tasks effectively can make or break your projects. Welcome to our Asynchronous JavaScript patterns with a focus on `async/await` and callbacks.
Introduction to Asynchronous JavaScript
Why Asynchronous Programming Is Important in JavaScript
JavaScript is a single-threaded language, meaning it can execute one task at a time. However, in real-world applications, you often need to perform multiple tasks simultaneously. This is where Asynchronous JavaScript comes into play. It ensures that your web applications remain responsive and efficient, allowing other tasks to continue while waiting for longer operations to complete.
The Evolution of Asynchronous JavaScript Patterns
Over the years, JavaScript has evolved significantly in terms of handling Asynchronous JavaScript operations. Developers initially relied heavily on callbacks to manage these tasks. However, this approach soon led to convoluted and hard-to-maintain code, commonly referred to as “callback hell.” To address these issues, JavaScript introduced Promises and later `async`/`await`, which offer more elegant and efficient ways to handle Asynchronous JavaScript code.
Understanding Callbacks in JavaScript
What Are Callbacks and How Do They Work?
Callbacks are functions passed as arguments to other functions, allowing them to be executed at a later time. This mechanism enables Asynchronous JavaScript behavior, letting your code continue running while waiting for an operation to complete.
Example:
javascript
function fetchData(callback) {
setTimeout(() => {
callback(‘Data received’);
}, 1000);
}
fetchData((data) => {
console.log(data);
});
Common Use Cases for Callbacks in Asynchronous JavaScript Code
Callbacks are commonly used in tasks such as event handling (e.g., click events), file reading, and making network requests. Despite their utility, they can quickly become cumbersome as the complexity of your application grows.

Callback Hell and Its Problems
What Is Callback Hell and Why Is It Problematic?
Callback hell occurs when callbacks are nested within other callbacks, leading to deeply indented, hard-to-read, and difficult-to-debug code. This makes it challenging to maintain and scale your application.
Strategies to Avoid Callback Hell
To mitigate callback hell, you can:
- Modularize your code by breaking it into smaller, reusable functions.
- Use Promises to simplify Asynchronous JavaScript operations.
- Adopt `async`/`await` for cleaner and more readable code.
Introduction to Promises in JavaScript
What Are Promises, and How Do They Improve Asynchronous Code?
Promises offer a more structured way to handle asynchronous tasks. They represent an operation that will either resolve (complete successfully) or reject (encounter an error) in the future.
Example:
javascript
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(‘Data received’);
}, 1000);
});
}
fetchData().then((data) => {
console.log(data);
});
The Promise Lifecycle
Promises have three states:
- Pending: The initial state, neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully.
- Rejected: The operation failed.
Handling Errors with Promises
Using `.catch()` for error handling in promises
Promises make error handling straightforward. You can chain a `.catch()` method to handle any errors that occur during the promise’s execution.
Example:
javascript
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error(‘Failed to fetch data’));
}, 1000);
});
}
fetchData()
.then((data) => {
console.log(data);
})
.catch((error)=> {
console.error(error.message);
});
The Importance of Proper Error Handling in Asynchronous Code
Proper error handling ensures that your application can gracefully handle unexpected issues, improving its reliability and user experience.
Chaining Promises
How Promise Chaining Simplifies Code
Promise chaining allows you to execute multiple asynchronous operations in sequence, reducing nesting and improving readability.
Example:
javascript
function stepOne() {
return new Promise((resolve)=> {
resolve(‘Step One Complete’);
});
}
function stepTwo() {
return new Promise((resolve)=> {
resolve(‘Step Two Complete’);
});
}
stepOne()
.then((result) => {
console.log(result);
return stepTwo();
})
.then((result) => {
console.log(result);
});
Best Practices for Creating Readable Promise Chains
Keep your promise chains clean by:
- Returning promises from functions.
- Avoiding deeply nested chains.
- Using descriptive function names.
Introduction to `async` and `await`
What are `async` and `await`?
`async` and `await` are syntax sugar built on Promises, making Asynchronous JavaScript code look and behave more like synchronous code. `async` functions return a promise, and `await` pauses the function execution until the promise resolves.
How `async/await` Simplifies Working with Promises
By using `async/await`, you can write Asynchronous JavaScript code that is easier to read and maintain.
Example:
javascript
async function fetchData() {
const data = await new Promise((resolve)=> {
setTimeout(() => {
resolve(‘Data received’);
}, 1000);
});
console.log(data);
}
fetchData();
Using `await` in Asynchronous Functions
How `await` Works in JavaScript
`await` pauses the execution of an `async` function until the promise it is awaiting resolves, ensuring that the subsequent code runs only after the promise is fulfilled.
Examples of Using `await` to Pause Code Execution
Example:
javascript
async function fetchData() {
const data = await new Promise((resolve)=> {
setTimeout(() => {
resolve(‘Data received’);
}, 1000);
});
console.log(data);
}
fetchData();
Error Handling in `async/await`
Using `try…catch` for Error Handling in Asynchronous JavaScript Functions
Error handling in `async/await` is done using `try…catch` blocks, allowing you to catch errors in a more familiar and structured way.
Example:
javascript
async function fetchData() {
try {
const data = await new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error(‘Failed to fetch data’));
}, 1000);
});
console.log(data);
} catch (error){
console.error(error.message);
}
}
fetchData();
Common Pitfalls and How to Avoid Them
- Avoid forgetting to use `await` with promises.
- Ensure `try…catch` is used to handle errors.
- Be mindful of blocking the event loop with synchronous code.
Comparing Callbacks, Promises, and `async/await`
Key Differences Between Callbacks, Promises, and `async/await`
- Callbacks can lead to nested and hard-to-maintain code.
- Promises offer a cleaner way to handle asynchronous operations and include built-in error handling.
- `async/await` further simplifies asynchronous code, making it more readable and easier to debug.
When to Use Each Asynchronous Pattern
- Use callbacks for simple, short-lived Asynchronous JavaScript tasks.
- Use Promises for more complex operations requiring chaining and error handling.
- Use `async/await` when readability and maintainability are a priority.
Handling Multiple Asynchronous Operations
Using `Promise.all` for Concurrent Operations
`Promise.all` allows you to run multiple promises concurrently and wait for all of them to resolve before proceeding.
Example:
javascript
const promise1 = new Promise((resolve) => setTimeout(resolve, 1000, ‘One’));
const promise2 = new Promise((resolve) => setTimeout(resolve, 2000, ‘Two’));
Promise.all([promise1, promise2]). then((values) => {
console.log(values); // [‘One’, ‘Two’]
});
How to Use `async/await` with Parallel and Sequential Tasks
You can use `await` within a loop to handle tasks sequentially or use `Promise.all` for parallel execution.
Example:
javascript
async function fetchData() {
const [data1, data2] = await Promise. all([
new Promise((resolve) => setTimeout(resolve, 1000, ‘One’)),
new Promise((resolve) => setTimeout(resolve, 2000, ‘Two’))
]);
console.log(data1, data2);
}
fetchData();
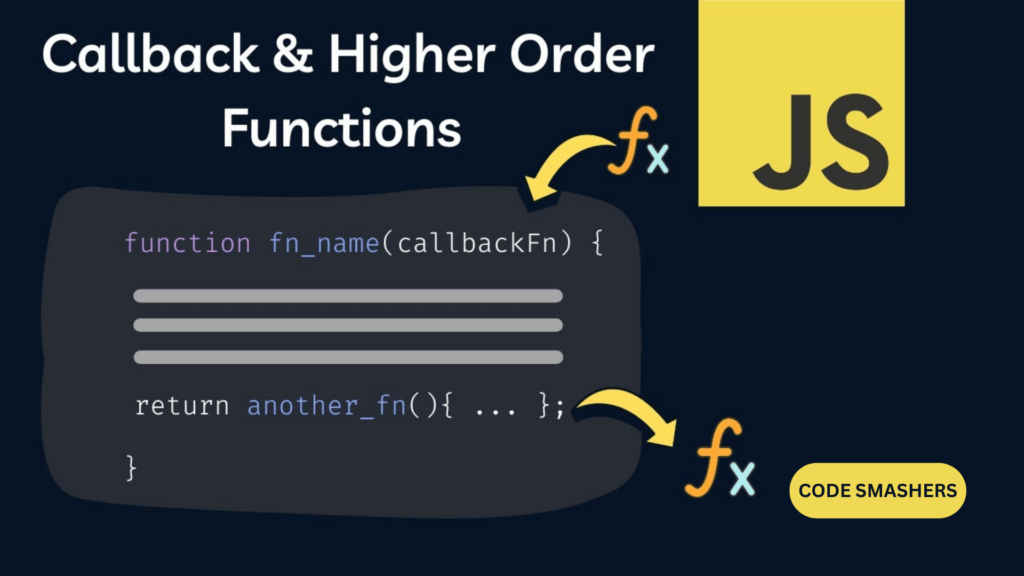
Callback Functions vs. Higher-Order Functions
Understanding the Role of Callbacks in Higher-Order Functions
Higher-order functions like’map`, `filter`, and `forEach` use callbacks to process array elements, making them a powerful tool for functional programming.
Using Callbacks in Functions Like’map`, `filter`, and `forEach`
Example:
javascript
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map((number) => number * 2);
console.log(doubled); // [2, 4, 6, 8, 10]
The Event Loop and Asynchronous Patterns
How the Event Loop Works with Callbacks and Promises
The event loop is responsible for managing Asynchronous JavaScript operations in JavaScript. It processes callbacks and promises, ensuring that the main thread remains non-blocking.
How `async/await` Interacts with the Event Loop
`async/await` is built on top of Promises and interacts with the event loop to pause and resume function execution.
Working with Asynchronous Iteration
How to Use `for await…of` with Async Iterables
`for await…of` allows you to iterate over asynchronous data sources, pausing execution until each promise resolves.
Practical Examples of Asynchronous JavaScript Loops
Example:
javascript
async function* asyncGenerator() {
yield new Promise((resolve) => setTimeout(resolve, 1000, ‘One’));
yield new Promise((resolve) => setTimeout(resolve, 2000, ‘Two’));
}
(async ()=> {
for await (const value of asyncGenerator()) {
console.log(value);
}
})();
Real-World Use Cases for Callbacks
Callbacks in Event Handling and DOM Manipulation
Callbacks are commonly used in event handling, such as button clicks and form submissions, to execute code in response to user interactions.
Using Callbacks in Node.js APIs and Asynchronous File Handling
Node.js APIs often use callbacks for handling asynchronous operations like file reading and writing.
Real-World Use Cases for `async/await`
Fetching Data with `async/await` in API Calls
`async/await` simplifies fetching data from APIs, allowing you to write cleaner and more readable code.
Example:
javascript
async function fetchData() {
const response = await fetch(‘https://api.example.com/data’);
const data = await response. json();
console.log(data);
}
fetchData();
Using `async/await` in server-side code with Node.js
`async/await` is also useful in server-side code, allowing you to handle asynchronous operations like database queries more efficiently.
Performance Considerations
Performance Implications of Using Callbacks
Callbacks can lead to performance bottlenecks if not managed properly, especially in complex applications.
How `async/await` Can Optimize Asynchronous Code
`async/await` can improve performance by reducing the complexity of asynchronous code, making it easier to optimize and debug.
Debugging Asynchronous Code
Tools and Techniques for Debugging Callback-Based Code
Debugging callback-based code can be challenging due to the nested nature of callbacks. Using tools like Chrome DevTools can help identify issues.
Debugging `async/await` and promises in Chrome DevTools
Chrome DevTools provides excellent support for debugging `async/await` and Promises, allowing you to set breakpoints and inspect the state of your code.
Refactoring Callback-Based Code to `async/await`
Steps to Convert Callback Functions to `async/await`
To refactor callback-based code to `async/await`:
- Identify the asynchronous operations.
- Wrap them in promises.
- Use `async/await` to handle the operations sequentially.
Benefits of Refactoring Legacy Code
Refactoring legacy code to use `async/await` can improve readability, maintainability, and overall performance.
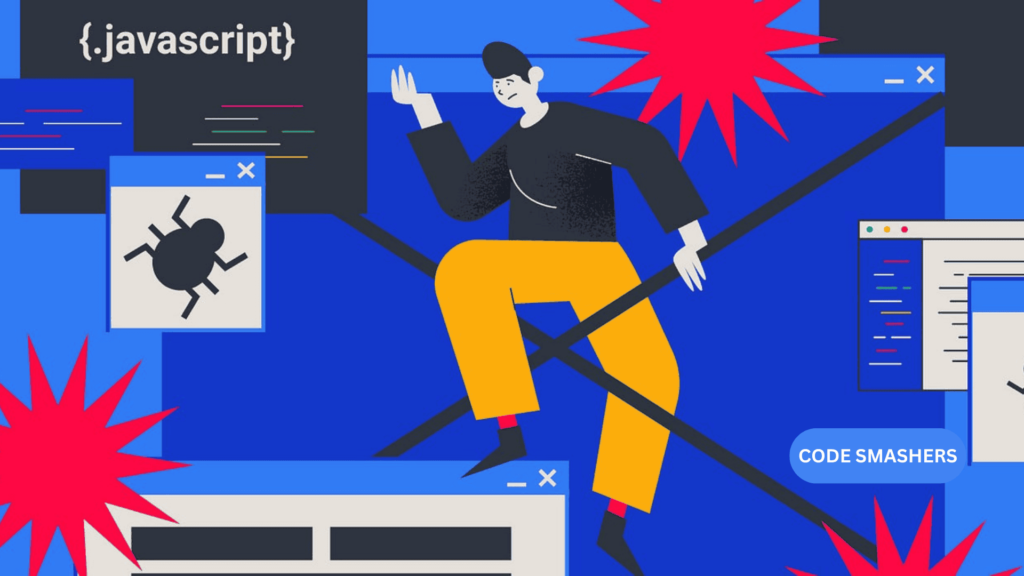
Best Practices for Debugging Asynchronous Code
Debugging asynchronous JavaScript code can present unique challenges due to the complex flow of execution and multiple levels of nested callbacks or promises. However, following some best practices can significantly enhance the debugging process:
- Leverage Developer Tools: Utilize the built-in developer tools in browsers like Chrome DevTools or Firefox Developer Tools. These tools offer features such as setting breakpoints, inspecting variables, and stepping through code even in asynchronous events, providing clarity on how your asynchronous operations are executed.
- Use descriptive logging: Implement thorough and descriptive logging at critical points within your asynchronous code. Including timestamps and context-specific messages can help trace the execution flow and identify where issues may arise.
- Consistently Handle Errors: Ensure robust error handling in your code by always using `.catch()` with Promises and `try/catch` blocks with `async/await`. Logging errors with relevant details can prevent silent failures and aid in diagnosing problems.
- Simplify with Code Linting: Use linting tools configured to flag common asynchronous pitfalls, such as unhandled promises or callbacks. Linting can catch issues before the code is even run, saving time in the debugging process.
- Break Down Complex Logic: Simplify and isolate sections of your asynchronous code by breaking them down into smaller, testable units. This practice makes it easier to pinpoint the location of issues and test each component independently.
- Employ Async-Friendly Libraries: Where possible, incorporate libraries designed for asynchronous operations, which often include built-in debugging features or more informative error messages, aiding in identifying problems.
By applying these best practices, developers can navigate the intricacies of asynchronous debugging more effectively, leading to more stable and reliable code.
Future Trends in Asynchronous JavaScript
Emerging Features and Patterns
New features and patterns in asynchronous JavaScript, such as async iterators and enhanced Promise APIs, continue to emerge, offering more powerful ways to handle asynchronous operations.
The Future of JavaScript Asynchronous Programming
The future of JavaScript asynchronous programming looks promising, with ongoing improvements and innovations that will make it even more efficient and developer-friendly.
Conclusion
Mastering JavaScript asynchronous patterns is essential for modern web development. By understanding and effectively using callbacks, promises, and `async/await`, you can write cleaner, more efficient, and more maintainable code. Whether you’re handling API calls, implementing complex workflows, or optimizing performance, these tools and techniques will help you achieve your goals. If you’re looking to take your JavaScript skills to the next level, consider experimenting with these patterns and exploring their potential in your own projects. Happy coding!