Introduction to File Handling in Python
One of the most important skills in programming is file handling. Whether you’re storing configuration settings, processing large datasets, or creating logs, knowing how to work with files in Python is essential. This blog post aims to guide you through the key concepts and practical techniques for reading and writing files in Python. By the end, you’ll have a solid grasp of file handling that can be applied to a myriad of projects.
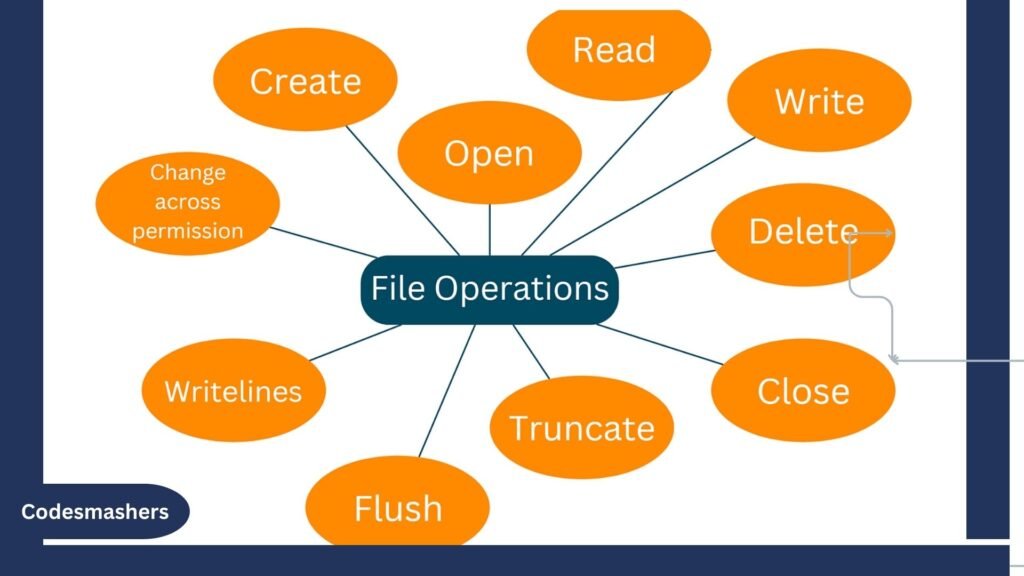
Understanding the Basic File Operations: open(), read(), and write()
The `open()` function
The `open()` function serves as your entry point for manipulating files in Python. It opens a file and returns a file object, which provides methods and attributes for interacting with the file content. The basic syntax is:
file = open (‘filename’, ‘mode’)
Here, `filename` is the name of the file you wish to open, and’mode` is a string indicating how you want to open it (e.g., ‘r’ for reading, ‘w’ for writing).
Reading Files with’read()`
The’read()` function retrieves a file’s contents in its entirety and outputs it as a string. It’s perfect for small files but can be impractical for larger ones. Example:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
Writing Files with `write()`
One way to write a string to a file is via the `write()` method. If the file doesn’t exist, it’s created. If it does, its content is overwritten (unless you open it in append mode). Example:
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
Reading Text Files in Python
Using `read()`
Because it reads one line at a time, “readline()” is more effective for huge files.
with open(‘largefile.txt’, ‘r’) as file:
content = file.read()
print(content[:100]) # Print the first 100 characters
Using `readline()`
For large files,’readline()` is more efficient as it reads one line at a time.
with open(‘largefile.txt’, ‘r’) as file:
line = file.readline()
while line:
print(line.strip())
line = file.readline()
Using `readlines()`
The’readlines()` function reads the text in its entirety into a list, with each line representing an item:
with open(‘largefile.txt’, ‘r’) as file:
lines = file.readlines()
for line in lines:
print(line.strip())
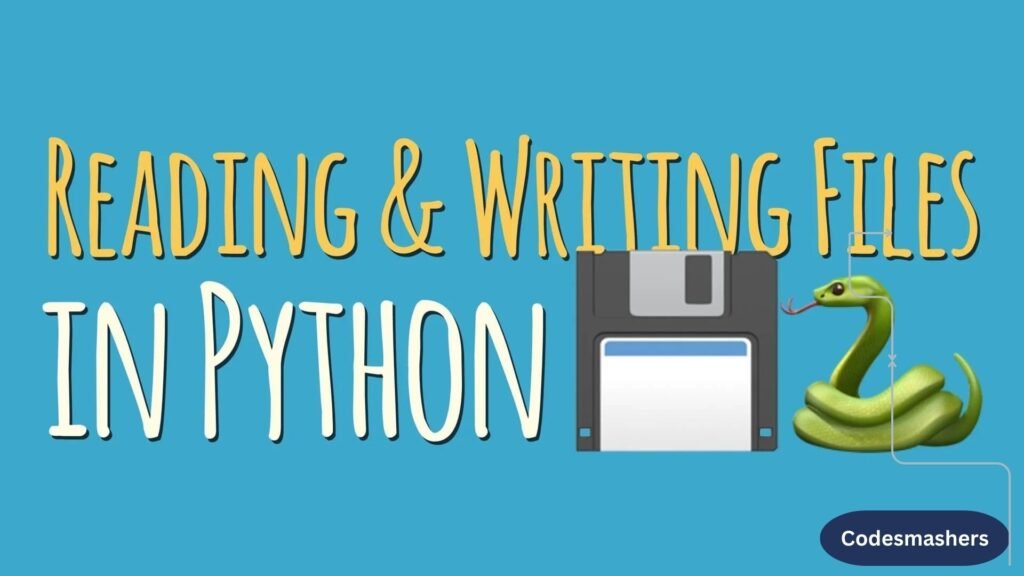
Writing to Text Files in Python
Using `write()`
This method writes a string to the file. Here’s an example:
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
Using `writelines()`
Use `writelines()} if you need to write more than one line.
lines = [‘1st line’, ‘2nd line’, ‘3rd line’]
with open(‘example.txt’, ‘w’) as file:
file.writelines(lines)
Using Directories and File Paths in Python
Python’s `os` and `pathlib` modules provide robust tools for handling file paths and directories.
Using `os` Module
To interface with the operating system, use the `os` module. For example:
import os
os.listdir(‘.’) # Enumerate every file and folder within the current directory.
Using `pathlib` Module
The `pathlib` module offers a more intuitive interface for path manipulation:
from pathlib import Path
path = Path(‘example.txt’)
print(path.exists()) # Check if the file exists.
Common Path Operations
- Join Paths: `os.path.join(‘folder’, ‘file.txt’)`
- Get File Extension: `os.path.splitext(‘file.txt’)[1]`
- Check Path Validity: `os.path.isfile(‘file.txt’)`
Best Practices in File Handling for Efficiency and Security
Closing files properly
To guarantee that resources are released, always close files.
file = open(‘example.txt’, ‘r’)
content = file.read()
file.close()
Or better yet, use a `with` statement:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
Handling Exceptions
Use try-except blocks to handle potential errors:
try:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
except FileNotFoundError:
print(“File not found!”)
Security Considerations
Be cautious with file paths to avoid security risks like path traversal attacks. Always validate and sanitize user inputs.
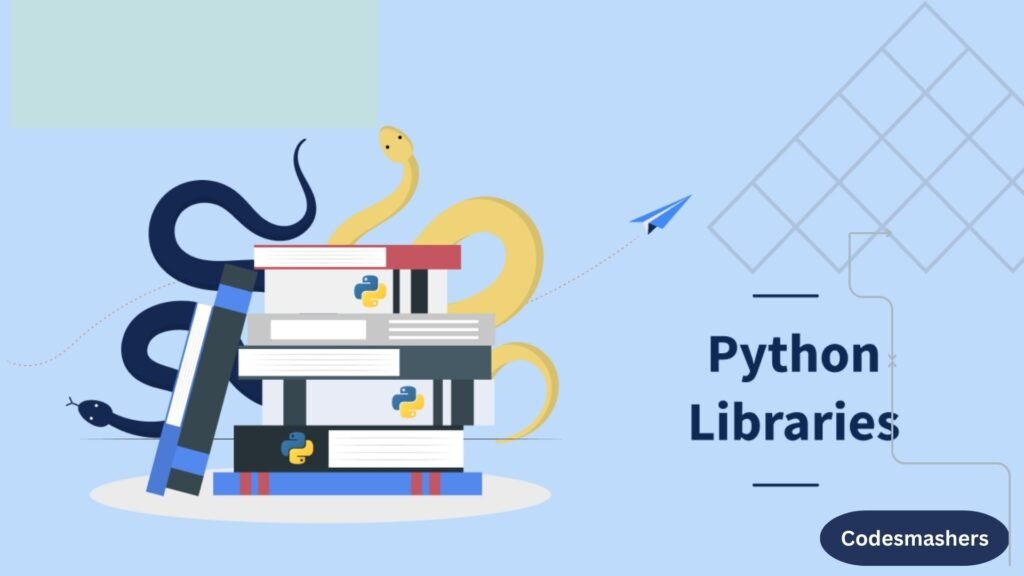
Advanced File Operations and Libraries in Python
Python offers several libraries for advanced file operations.
CSV Files
To read and write CSV files, using the `csv{ module:
import csv
with open(‘data.csv’, ‘r’) as file:
reader = csv.reader(file)
for row in reader:
print(row)
JSON Files
For working with JSON data, the `json` module is handy:
import json
with open(‘data.json’, ‘r’) as file:
data = json.load(file)
print(data)
Binary Files
To handle binary data, use the’struct` module:
import struct
with open(‘data.bin’, ‘rb’) as file:
data = file.read(4)
value = struct.unpack(‘i’, data)
print(value)
Practical Examples and Use Cases for File Handling in Python
Data Analysis
Imagine a project where you’re analyzing sales data from CSV files:
import csv
with open(‘sales.csv’, ‘r’) as file:
reader = csv.reader(file)
total_sales = sum(float(row[2]) for row in reader)
print(f”Total Sales: ${total_sales}”)
Web Scraping
Consider a web scraper that downloads pages and saves them locally:
import requests
url = ‘http://example.com’
response = requests.get(url)
with open(‘example.html’, ‘w’) as file:
file.write(response.text)
Configuration Management
Manage application settings through configuration files:
with open(‘config.txt’, ‘r’) as file:
config = file.read()
print(config)
Report Automation
Generate daily reports by fetching data from a database:
import sqlite3
conn = sqlite3.connect(‘example.db’)
cursor = conn.cursor()
cursor.execute(‘SELECT * FROM sales’)
with open(‘daily_report.txt’, ‘w’) as file:
for row in cursor.fetchall():
file.write(str(row) + ‘\n’)
Exploring Advanced File Operations Libraries in Python
Python supports a multitude of libraries to enhance the efficiency and capability of file operations. Beyond basic reading and writing, these libraries allow for more complex interactions with different file types and formats.
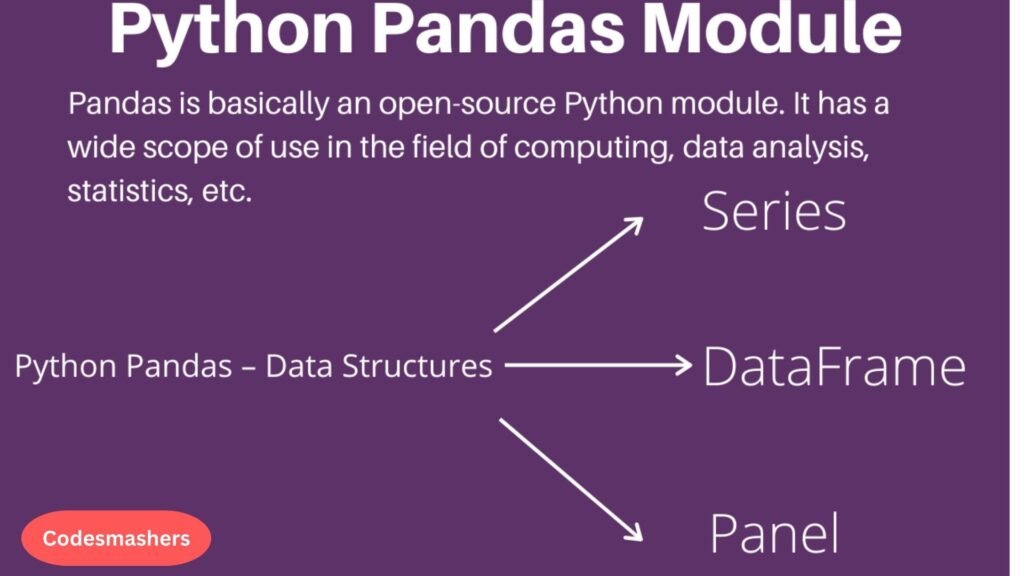
Pandas for data manipulation
The `pandas` library is invaluable for data manipulation, particularly when dealing with large datasets stored in files like CSVs or Excel spreadsheets. Pandas can significantly simplify the reading, processing, and writing of data through its DataFrame objects. For example, reading a CSV file into a dataframe is as straightforward as:
python
import pandas as pd
df = pd.read_csv(‘data.csv’)
print(df.head())
PyPDF2 for PDF Files
To work with PDF files, `PyPDF2` is a handy library that lets you extract text, rotate pages, and merge documents among other operations. This can be particularly suitable for automating tasks that involve PDF manipulation:
python
import PyPDF2
with open(‘document.pdf’, ‘rb’) as file:
reader = PyPDF2.PdfReader(file)
page = reader.pages[0]
print(page.extract_text())
Openpyxl for Excel Files
`openpyxl` provides tools to create, modify, and extract data from Excel spreadsheets (.xlsx files). It’s especially useful in environments that heavily utilize Microsoft Office for data management.
python
import openpyxl
workbook = openpyxl.load_workbook(‘spreadsheet.xlsx’)
sheet = workbook.active
print(sheet[‘A1’].value)
HDF5 Files with h5py
For scientific data, the `h5py` library is excellent for interacting with HDF5 files, enabling efficient storage and manipulation of large datasets. It is extensively used in fields that require the handling of complex datasets:
python
import h5py
with h5py.File(‘data.h5’, ‘r’) as file:
data = file[‘dataset’]
print(data[:])
Utilizing these libraries can greatly enhance the robustness and efficiency of file handling in Python, especially when dealing with specialized file formats or large-scale data processing tasks.
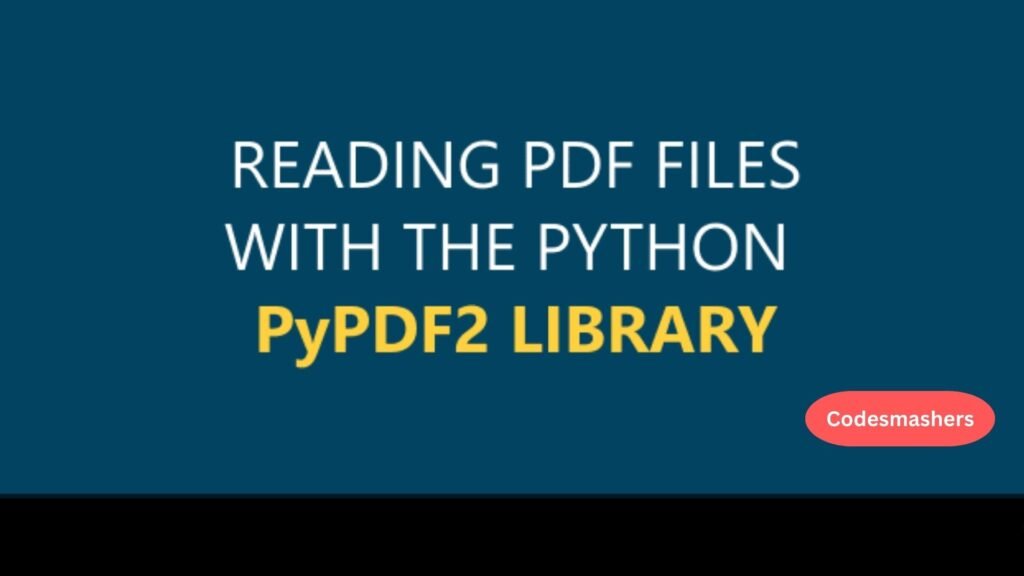
Benefits of Using PyPDF2 for PDF Manipulation
A flexible and strong library created especially for using with PDF files in Python is called PyPDF2. One of its primary benefits is its ability to extract text from PDF documents efficiently, which is invaluable for both data analysis and content processing tasks. Additionally, PyPDF2 supports operations such as rotating pages, merging multiple PDF files, and splitting a single document into several parts, providing ample flexibility for document management.
Another significant advantage is its ability to add or modify metadata, such as author or title information, which can be critical for maintaining organized digital libraries. The library’s ability to encrypt and decrypt PDF files also adds an essential layer of security and control over sensitive documents. With its comprehensive features, PyPDF2 empowers users to easily automate and simplify complex PDF handling tasks.
In this blog post, we’ve covered the essentials of file handling in Python, from basic operations like reading and writing to advanced techniques using specialized libraries. Whether you’re a beginner or an experienced programmer, mastering file operations in Python can significantly boost your productivity and efficiency.
Ready to take your skills to the upgrade level? Explore more resources and experiment with file handling in your projects today. Happy coding!