Introduction to Python Multithreading and Multiprocessing
In today’s fast-paced tech landscape, optimizing code performance is crucial for every developer. One of the most effective ways to achieve this is by leveraging parallelism through Python’s multi-threading and multiprocessing capabilities. Parallelism allows tasks to run concurrently, significantly boosting performance and efficiency.
But what exactly are multi-threading and multiprocessing, and how do they differ? In essence, multi-threading involves running multiple threads (smaller units of process) within a single program, while multiprocessing involves simultaneously running multiple processes. Understanding these concepts is vital for any developer aiming to optimize their code.
In this guide, we’ll explore the ins and outs of Python multi-threading and multiprocessing, providing practical tips, real-world examples, and best practices to enhance your coding skills.
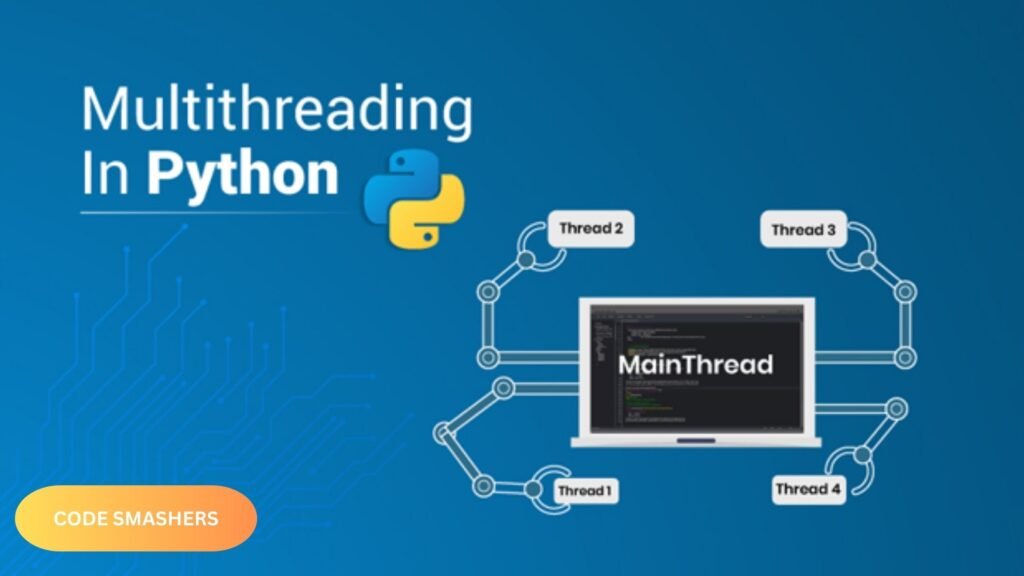
Understanding Multithreading in Python
What are threads?
Threads are the smallest process unit; the operating system can schedule that. In Python, threads perform tasks simultaneously within a single process. This means multiple threads can run in parallel, allowing for more efficient use of resources.
Benefits and Limitations of Multithreading
Multi-threading offers several benefits, including improved performance, better resource utilization, and reduced latency. For instance, Instagram uses multi-threading to handle user requests simultaneously, enhancing the user experience by reducing response times.
However, multi-threading also comes with limitations. The Global Interpreter Lock (GIL) in Python can prevent true parallel execution of threads, limiting performance gains in CPU-bound tasks. Additionally, managing threads can be complex, especially when dealing with thread synchronization and data sharing.
Implementing multi-threading in Python
Creating and Managing Threads
Implementing multi-threading in Python is straightforward, thanks to the `threading` module. Here’s an example of a multi-threaded web scraper:
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(f”Fetched {url} with status {response.status_code}”)
urls = [
“https://example.com”,
“https://example.org”,
“https://example.net”
]
threads = []
for url in urls:
thread = threading. Thread(target=fetch_url, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
Best Practices for Safe Multithreading
To ensure safe and efficient multi-threading, follow these best practices:
- Avoid shared states between threads or use thread-safe data structures.
- Use locks, semaphores, or other synchronization primitives to manage access to shared resources.
- Limit the number of threads to prevent excessive context switching and resource contention.
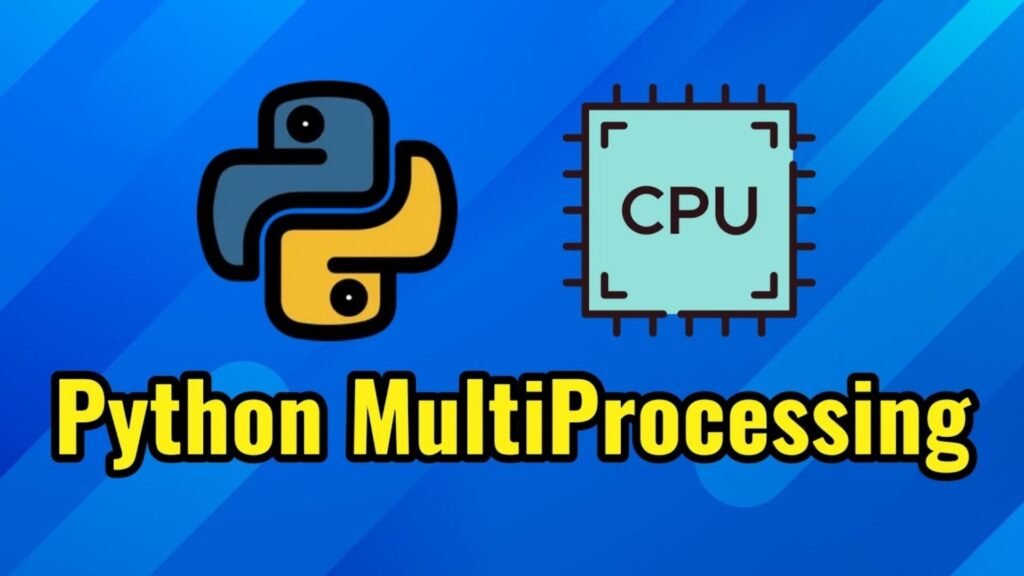
Introduction to Multiprocessing in Python
Overview of the Multiprocessing Module
Multiple processes may be created and managed by developers using Python’s `multiprocessing` module. Since each process has its own memory space, they are not constrained by the GIL like threads are.
Advantages and Challenges of Using Multiprocessing
Multiprocessing offers several advantages, including true parallelism, better performance for CPU-bound tasks, and improved fault isolation. For example, video game developers use multiprocessing to parallelize tasks like AI and physics simulations, enhancing game performance.
However, multiprocessing also comes with challenges. Inter-process communication, process synchronization, and memory consumption can be complex.
Utilizing Multiprocessing in Python
Creating and managing processes
Here’s an example of how to create and manage processes using the’multiprocessing` module:
from multiprocessing import Process
def print_square(num):
print(f”Square of {num} is {num * num}”)
If name == “main“:
processes = []
for i in range(5):
process = Process(target=print_square, args=(i,))
processes.append(process)
process.start()
for process in processes:
process.join()
Comparing Multiprocessing with Multithreading
To illustrate the difference between multi-threading and multiprocessing, consider a CPU-bound task like calculating the factorial of large numbers. Benchmarks show that multiprocessing performs significantly better than multi-threading in such scenarios due to true parallelism.
Real-world Applications and Use Cases
Multi-threading in Action
Quora uses Python multi-threading to handle concurrent requests, ensuring real-time content delivery and enhancing the user experience. Similarly, Reddit leverages multi-threading to process comments in real-time, managing a high volume of user-generated content efficiently.
Multiprocessing in Action
YouTube uses Python multiprocessing for encoding and decoding videos, significantly reducing processing time. Additionally, genetic algorithm optimization in bioinformatics benefits from multiprocessing, enabling parallel evaluation of multiple solutions.
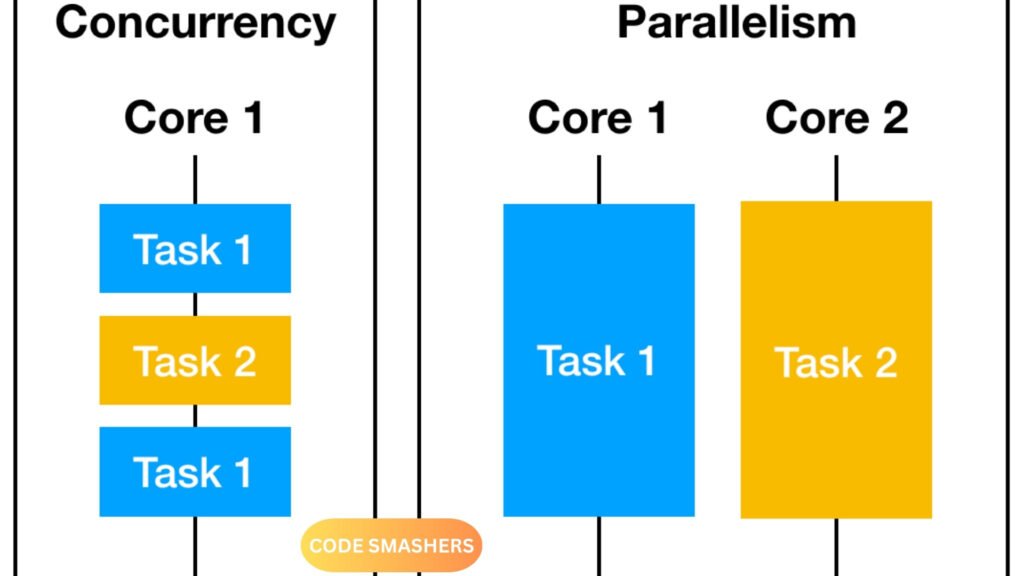
Best Practices for Python Concurrency
Optimizing Code Performance
To optimize your code performance using multi-threading and multiprocessing:
- Profile your code to identify bottlenecks and determine the best concurrency model.
- Use thread pools or process pools to manage a fixed number of worker threads or processes, reducing overhead.
- Leverage asynchronous programming (e.g., `asyncio`) for I/O-bound tasks to achieve concurrency without the complexity of thread management.
Avoiding Common Pitfalls
Common pitfalls in Python concurrency include deadlocks, race conditions, and excessive resource consumption. To avoid these issues:
- Use proper synchronization mechanisms (e.g., locks, semaphores) to manage access to shared resources.
- Monitor and limit the number of concurrent threads or processes to prevent resource exhaustion.
- Test and debug your concurrent code thoroughly to identify and fix concurrency-related bugs.
Future of Parallelism in Python
Upcoming Features and Improvements
Python continues to evolve, with ongoing efforts to improve support for parallelism. Upcoming features like interpreters and per-interpreter GIL aim to provide better concurrency performance and isolation.
Latest Trends in Python Concurrency
Trends in Python concurrency include increased adoption of asynchronous programming, better integration of GPU computing, and advancements in distributed computing frameworks. Staying updated with these trends will help you leverage the latest tools and techniques for parallelism.
Conclusion
Multi-threading and multiprocessing are powerful tools for optimizing Python code performance. Understanding their differences, benefits, and limitations is crucial for any developer aiming to harness the power of parallelism. By following best practices and staying updated with the latest trends, you can build scalable and efficient applications.
Implement these concepts in your projects and share your experiences with us. We’d love to hear how Python concurrency has enhanced your code performance.
For more in-depth resources and expert tips, please contact us or check out our additional guides on Python concurrency. Happy coding!