Programming can initially seem daunting, especially when faced with a multitude of concepts and terminologies. However, understanding the core principles of Object-Oriented Programming (OOP) can make the learning curve smoother. Among these principles, it plays a crucial role. This beginner-friendly guide will help you grasp its essence, its importance, and its applications across various programming languages. By the end of this post, you’ll have a comprehensive understanding of how encapsulation can enhance your coding practice.
Introduction to Encapsulation The Foundation of OOP
Encapsulation is a fundamental concept in Object-Oriented Programming that involves bundling data and methods that operate on the data within a single unit, known as a class. This data provides a mechanism for restricting access to some components of an object, which is a crucial aspect of OOP. It acts as the backbone for creating modular and maintainable code structures. By confining the internal state of an object and requiring all interaction to occur through well-defined interfaces, It not only improves the organization of code but also ensures the integrity of our data.
What Is Encapsulation?
Think of encapsulation as a protective shield that prevents outside interference and misuse of the internal workings of an object. Imagine a car; you don’t need to understand the intricate workings of the engine to drive it. It allows you to use public methods to interact with the car while keeping the complex engine mechanics hidden. In programming, It hides the internal state of an object and only exposes a controlled interface.

This concept can be illustrated with a simple example. Consider a class `Car` encapsulating data such as `speed` and `fuelLevel`, and methods like `accelerate()` and `brake()`. It ensures that the details of how data is managed are hidden from the user, promoting cleaner and more efficient code.
Example of Encapsulation in Python
To better understand, let’s look at an example in Python. Suppose we define a class `Car` that encapsulates the data related to a car’s speed and fuel level and provides methods to interact with this data:
python
class Car:
def init(self, speed=0, fuel_level=100):
# Private attributes
self.__speed = speed
self.__fuel_level = fuel_level
# Public method to get the speed
def get_speed(self):
return self.__speed
# Public method to set the speed
def set_speed(self, speed):
if speed >= 0:
self.__speed = speed
# Public method to get the fuel level
def get_fuel_level(self):
return self.__fuel_level
# Public method to refuel
def refuel(self, amount):
self.__fuel_level += amount
Example usage
my_car = Car()
print(“Initial speed:”, my_car.get_speed())
my_car.set_speed(60)
print(“Updated speed:”, my_car.get_speed())
print(“Initial fuel level:”, my_car.get_fuel_level())
my_car.refuel(10)
print(“Fuel level after refueling:”, my_car.get_fuel_level())
In this code, the `Car` class encapsulates the attributes `speed` and `fuel_level` by marking them as private using the double underscore prefix (`__`). The public methods `get_speed()`, `set_speed()`, `get_fuel_level()`, and `refuel()` provide controlled access to these private attributes. This ensures that the internal state of `Car` is modified only through the defined interfaces, maintaining the integrity and security of the data.
Why Encapsulation Matters Protecting Your Data and Code
Encapsulation is vital for several reasons. Firstly, it enhances security by protecting an object’s integrity. By controlling how data is accessed and modified, It minimizes the risk of unintended interference. Imagine if any part of your program could arbitrarily change a car’s speed—chaos would ensue. It ensures that only designated methods can alter an object’s state, safeguarding it against unauthorized actions.
Secondly, It improves code organization. By grouping related variables and methods, it becomes easier to manage and understand code. It also supports maintenance since changes to the encapsulated data do not affect the external code. This results in a cleaner, more modular codebase that is easier to debug and extend.
Encapsulation vs Abstraction Understanding the Differences
While encapsulation and abstraction are both pillars of OOP, they serve distinct purposes. Encapsulation is about hiding the internal state and requiring all interactions to occur through an object’s methods. Abstraction, on the other hand, focuses on hiding complexity by providing a simplified representation of an entity. Think of abstraction as designing the car interface—steering wheel, pedals—without exposing how these components work internally.

A practical way to distinguish them is through code examples. In a class `Car`, encapsulation would involve private variables like `engineStatus`, whereas abstraction would define what actions can be performed, such as `startEngine()`, without detailing how the operations are executed.
Practical Applications of Abstraction and Encapsulation in OOP
In Object-Oriented Programming (OOP), abstraction and encapsulation are both pivotal for creating robust, maintainable, and scalable applications. Abstraction allows developers to focus on designing user interfaces and functional aspects without getting bogged down by implementation details. This is achieved by defining what actions an object can perform, describing the essential characteristics while hiding complex background processes. On the other hand, It protects these internal processes by limiting access and modification to an object’s state through well-defined interfaces.
This ensures data integrity and security, making It an invaluable tool for managing larger codebases and collaborating within teams. Together, abstraction and encapsulation facilitate the development of efficient software systems, enabling programmers to create clear hierarchies, improve code reusability, and streamline user interactions with the system.
Real-World Examples
Encapsulation isn’t just a programming concept; it’s mirrored in many real-world systems. Consider a vending machine. Consumers interact with a simple interface to select products and make payments, while the internal processes of inventory management and product dispensing remain hidden. This separation allows users to obtain their desired outcome without needing to understand or manipulate the complex internal mechanisms.
Another example is a smartphone. Users interact with apps through a user-friendly interface, while the operating system handles the intricate details of processing user inputs. In these cases It ensures that users can efficiently accomplish tasks without needing to understand the underlying complexities.
How Encapsulation Enhances Security and Code Organization
Encapsulation plays a crucial role in enhancing the security of software applications. By limiting how data can be accessed or modified, it prevents unauthorized access and potential data corruption. This is akin to having a safe for valuables where only authorized individuals have the key. By using private variables and methods in classes, developers can maintain control over sensitive information.
Additionally, it contributes significantly to code organization. It allows for clearer separation of concerns, where each class has a well-defined purpose and interface. This clarity makes it easier for teams to collaborate on large projects, as each team member can work on encapsulated components without interfering with others’ work.
Private, Public, and Protected Access Modifiers Explained
Access modifiers are the gatekeepers of encapsulation, dictating how different parts of a program can interact with an object. Understanding the distinction between private, public, and protected access is essential for implementing effectively.
- Private access restricts visibility to within the class itself. It is the most secure level, ensuring that sensitive data is not accessible from outside the class.
- Public access allows unrestricted interaction with class members from any part of the program. While useful for defining an interface, it should be used judiciously to prevent exposing too much of a class’s implementation.
- Protected access strikes a balance, allowing access within the class and its subclasses. This modifier is particularly useful in inheritance hierarchies.
By strategically using these access modifiers, programmers can control the level of exposure and ensure that their code adheres to good practices.
Encapsulation in Java Python C++ Language-Specific Implementations
Encapsulation is a universal principle in OOP, but its implementation can vary across programming languages like Java, Python, and C++. Each language provides its syntax and conventions to support it.
In Java, encapsulation is achieved through classes, and access modifiers are strictly enforced. Java’s robust encapsulation features make it a popular choice for enterprise-level applications that demand high security and maintainability.
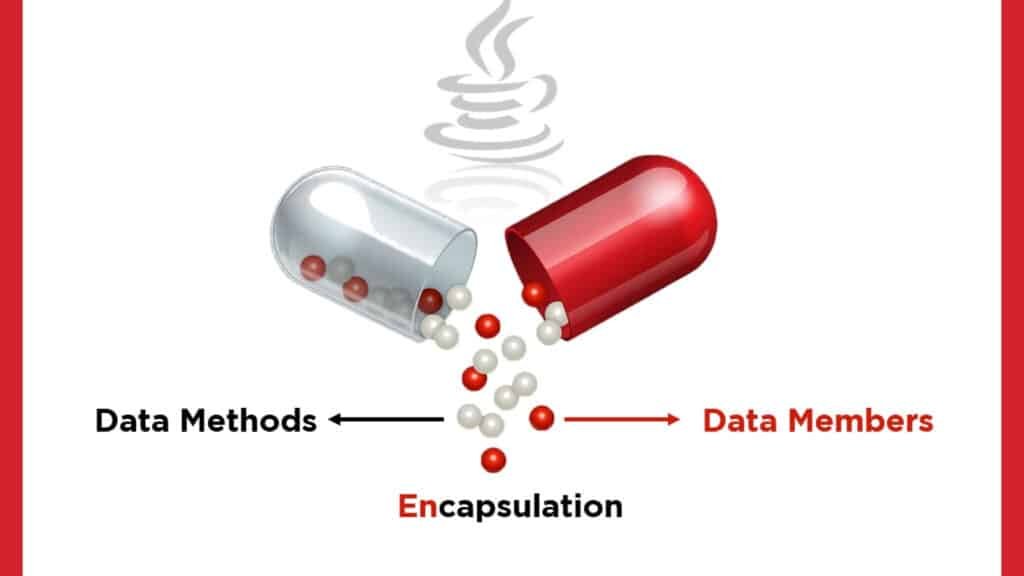
Python takes a slightly different approach. Although it lacks explicit access modifiers, encapsulation is practiced using naming conventions. Prefixing an attribute with an underscore denotes it as “protected,” and double underscores indicate a “private” attribute, though these are more so conventions than enforced restrictions.
In C++, encapsulation is implemented similarly to Java, with access specifiers like private, public, and protected. C++ also offers additional features like friend functions, which can access private members, showcasing its flexibility in managing encapsulation.
The Role of Getters and Setters in Encapsulation
Getters and setters are essential tools for implementing encapsulation. They provide controlled access to an object’s properties, allowing for validation and transformation while maintaining it. By using getters and setters, developers can ensure that data manipulation adheres to specific rules and conditions.
For instance, consider a class `BankAccount` with a private variable `balance`. A getter method might return the balance, while a setter could enforce a rule that prevents setting a negative balance. This helps maintain the integrity of the `BankAccount` class.
java
public class BankAccount {
// Private member variable to store the balance
private double balance;
// Constructor to initialize the balance
public BankAccount(double initialBalance) {
if (initialBalance >= 0) {
this.balance = initialBalance;
} else {
System.out.println(“Initial balance cannot be negative.”);
}
}
// Getter method to retrieve the balance
public double getBalance() {
return this.balance;
}
// Setter method to update the balance
public void setBalance(double newBalance) {
if (newBalance >= 0) {
this.balance = newBalance;
} else {
System.out.println(“Balance cannot be set to a negative value.”);
}
}
// Method to deposit an amount
public void deposit(double amount) {
if (amount > 0) {
this.balance += amount;
} else {
System.out.println(“Deposit amount must be positive.”);
}
}
// Method to withdraw an amount
public void withdraw(double amount) {
if (amount > 0 && amount <= this.balance) {
this.balance -= amount;
} else {
System.out.println(“Invalid withdrawal amount.”);
}
}
}
In this example, the `BankAccount` class employs encapsulation by keeping the `balance` variable private and providing public getter and setter methods. The `getBalance` method allows users to query the balance, while the `setBalance` method ensures that any updates meet the condition of being non-negative, thus maintaining the class’s integrity. Additional methods `deposit` and `withdraw` further demonstrate controlled access and modification to the account’s balance.
Additionally, getters and setters provide a layer of abstraction. Users interact with these methods without needing to know how data is stored or processed internally. This separation of concerns enhances code readability and maintainability.
How Encapsulation Promotes Code Reusability and Flexibility
It contributes significantly to code reusability and flexibility. By bundling data and methods within self-contained classes, It allows these classes to be reused across different projects and contexts. This modular approach reduces redundancy and promotes efficient use of resources.
It also facilitates flexibility by enabling changes to be made to the internal workings of a class without affecting its external interface. For example, a class that encapsulates a data storage mechanism can switch from using a file system to a database without impacting other parts of the program. This adaptability is crucial in dynamic environments where requirements evolve over time.
Ultimately, It empowers developers to create robust, adaptable, and reusable code that can meet diverse needs and challenges.
Encapsulation and Data Hiding Protecting Sensitive Information
Data hiding is a critical aspect of encapsulation that focuses on concealing internal details and protecting sensitive information. This practice is essential for maintaining the integrity and security of an application.
By restricting access to internal data through encapsulation, developers can prevent unauthorized or accidental modifications. For instance, in a healthcare application, patient records should be accessible only through specific methods that enforce data validation and privacy regulations. It ensures that sensitive information remains confidential and secure.

Data hiding also simplifies maintenance by reducing dependencies between components. With encapsulation in place, changes to the internal implementation of a class do not ripple through the entire codebase, making it easier to update and enhance individual components without compromising overall functionality.
Breaking Down Complex Systems with Encapsulation
It is a powerful tool for managing complexity in large systems. By breaking down a system into smaller, encapsulated components, developers can create manageable units that are easier to understand and maintain.
Imagine a software application with multiple interconnected modules, each responsible for a specific function. It enables these modules to operate independently, communicating through well-defined interfaces. This modular structure allows teams to work concurrently on different parts of the system, accelerating development and reducing the risk of errors.
It also enhances scalability, as new features can be added or existing components modified without disrupting the entire system. This flexibility is invaluable in today’s rapidly changing technological landscape, where adaptability is a key competitive advantage.
Encapsulation in Action A Step-by-Step Example for Beginners
To illustrate encapsulation in action, let’s walk through a simple example. Consider a class `Rectangle` that encapsulates data such as `width` and `height`, along with methods to calculate the area and perimeter.
First, define the private variables `width` and `height` within the class. Then, create public getter and setter methods for each variable, ensuring that they validate input data to prevent negative values.
Finally, implement the methods `calculateArea()` and `calculatePerimeter()`, which use the encapsulated data to perform calculations. By interacting with the rectangle through these methods, users gain access to its functionality without needing to manipulate the internal data directly.
This example demonstrates how It promotes secure, organized, and efficient code that is easy to understand and use.
Common Mistakes When Implementing Encapsulation and How to Avoid Them
While encapsulation is a fundamental principle of OOP, beginners often make mistakes when implementing it. One common error is exposing too many variables through public access, which undermines the benefits of its. To avoid this, ensure that only essential methods and variables are made public, keeping the rest private or protected.
Another mistake is overusing getters and setters, leading to code that is unnecessarily complex and difficult to maintain. Strike a balance by using these methods judiciously and only when necessary to enforce rules or validation.
Finally, beginners may neglect to use encapsulation consistently across their codebase. Make it a habit to encapsulate data and methods within cohesive classes, as this practice reinforces the modularity and maintainability of your code.
Encapsulation and Class Design Creating Well-Structured Code
Effective class design is integral to successful encapsulation. By adhering to best practices for class design, developers can create well-structured code that leverages the full potential of encapsulation.
Start by identifying the core responsibilities of each class, ensuring that they align with the principles of single responsibility and cohesion. This clarity helps determine which data and methods should be encapsulated within the class.
Next, use access modifiers strategically to control visibility and interaction. Limit the exposure of internal data while providing clear, well-documented interfaces for public methods. This approach enhances both security and usability.
Finally, consider the relationships between classes, using this to define boundaries and interactions. By thoughtfully designing classes and their encapsulated components, developers can create a codebase that is robust, scalable, and adaptable to change.
Benefits of Encapsulation Why Every Programmer Should Master It
It offers numerous benefits that every programmer should strive to master. Firstly, it enhances data security by providing a controlled environment for data access and modification. This protection safeguards sensitive information and ensures data integrity.
Secondly, It improves code organization and readability. By grouping related variables and methods within cohesive classes, encapsulation reduces complexity and fosters a clean, modular codebase.
Lastly, It promotes adaptability and reusability. Encapsulated components can be easily modified, extended, and reused across projects, streamlining development and reducing redundancy. This versatility is invaluable in dynamic environments where requirements are constantly evolving.
Encapsulation and Object-Oriented Design Patterns How They Work Together
Encapsulation is a foundational concept in OOP that underpins many design patterns. By understanding how encapsulation interacts with design patterns, developers can create more effective and maintainable code.
For example, the Singleton pattern leverages encapsulation to ensure that a class has only one instance, providing a global access point while controlling instantiation. Similarly, the Factory pattern uses encapsulation to create objects without exposing the creation logic, promoting flexibility and modularity.
By integrating It with design patterns, developers can enhance the scalability, maintainability, and performance of their applications. This synergy empowers programmers to tackle complex challenges with confidence and creativity.
How to Refactor Legacy Code to Implement Encapsulation
Refactoring legacy code to implement encapsulation can be a challenging but rewarding process. Begin by identifying areas where encapsulation is lacking, such as public variables or tightly coupled components.
Next, encapsulate data and methods within cohesive classes, using access modifiers to control visibility and interaction. This restructuring enhances code organization and reduces dependencies.
Finally, test the refactored code thoroughly to ensure that encapsulation has been implemented correctly without introducing new issues. By iteratively applying these steps, developers can breathe new life into legacy code, making it more secure, maintainable, and adaptable.
The Evolution of Encapsulation in Modern Programming Languages
Encapsulation has evolved alongside modern programming languages, adapting to new paradigms and technologies. In recent years, languages have introduced features that enhance encapsulation, such as improved access modifiers, advanced data structures, and sophisticated development tools.
For example, modern languages like Kotlin and Swift offer advanced its features that streamline code organization and enhance security. These languages prioritize developer productivity and code quality, reflecting the ongoing evolution of encapsulation practices.
By staying informed about these advancements, developers can harness the latest encapsulation techniques to create innovative, high-performance applications that meet the demands of today’s fast-paced digital landscape.
Mastering Encapsulation Best Practices for Writing Secure, Maintainable Code
Mastering encapsulation is crucial for writing secure, maintainable code. By following best practices, developers can leverage encapsulation to create robust, adaptable applications.
Begin by applying the principle of least privilege, limiting access to data and methods through strategic use of access modifiers. This approach enhances security and reduces the risk of unintended interactions.
Next, focus on creating cohesive, well-structured classes that encapsulate related data and methods. This modular organization simplifies code maintenance and promotes reusability.
Finally, continuously refine your understanding by exploring emerging patterns, tools, and languages. By staying informed and adaptable, developers can master encapsulation and create high-quality code that stands the test of time.
Frequently Asked Questions
Q1: What is encapsulation in programming?
Encapsulation is a fundamental concept in object-oriented programming (OOP) that involves bundling data and methods that operate on that data within a single unit, or class. It restricts direct access to some of an object’s components, which is a means of preventing unintended interference and misuse.
Q2: Why is it important in software development?
It enhances data security by controlling access, improves code organization and readability by grouping related functions, and promotes adaptability and reusability by allowing encapsulated components to be modified independently. This results in a more robust and maintainable code.
Q3: How does encapsulation improve security?
By using access modifiers to limit accessibility, encapsulation protects sensitive data from being accessed or altered by external code. This restricted access helps ensure that data integrity is maintained, reducing the risk of malicious or accidental modifications.
Q4: Can you give examples of encapsulation in design patterns?
Yes, It is integral to many design patterns. For instance, the Singleton pattern encapsulates the instance creation to guarantee only one instance exists, while the Factory pattern encapsulates the object creation process, promoting modularization without exposing creation logic.
Q5: What are common challenges in implementing encapsulation?
Common challenges include identifying which data and methods should be encapsulated, refactoring legacy code that lacks encapsulation, and understanding the specific access modifiers and encapsulation features of different programming languages to effectively manage visibility and interaction.
Q6: How can encapsulation be applied to legacy code?
To apply It to legacy code, begin by identifying areas lacking encapsulation, such as public variables. Encapsulate them within classes, apply appropriate access modifiers, and refactor dependencies. Testing the refactored code ensures that new encapsulation schemes work effectively.
Q7: How has encapsulation evolved with modern programming languages?
Modern programming languages have introduced features that enhance encapsulation, such as sophisticated access modifiers and language constructs that streamline code organization. Languages like Kotlin and Swift have advanced these features, reflecting ongoing innovations in encapsulation practices.
Additional Resources and Useful Links
Books and Articles
- “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin: This book provides fundamental insights into writing clean and maintainable code, with a strong emphasis on the principles of encapsulation and other OOP concepts.
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides: Often referred to as the “Gang of Four” book, it delves into design patterns including those that make extensive use of encapsulation.
Online Courses
- Coursera: Object-Oriented Programming in Java: A comprehensive course that covers encapsulation, inheritance, and polymorphism.
- Udemy: The Complete Java Developer Course: Offers in-depth explanations and practical exercises on encapsulation within Java programming.
Websites and Online Communities
- Stack Overflow: An invaluable resource for finding answers to specific questions about encapsulation and other programming topics.
- GitHub: A platform where you can explore open-source projects that effectively implement encapsulation practices.
Tutorials and Documentation
- Oracle’s Java Tutorials: Offers detailed tutorials on encapsulation and other OOP principles in Java.
- Swift Documentation (Apple Developer): Includes extensive information on Swift’s encapsulation features and best practices.
Tools and Software
- Eclipse IDE: An integrated development environment that includes tools for managing encapsulation in large-scale projects.
- IntelliJ IDEA: A Java IDE offering advanced tools for refactoring and improving encapsulation in codebases.