Functional programming has gained significant traction in the JavaScript community for its ability to create robust, maintainable code. Whether you’re a seasoned developer or a programming novice, understanding functional programming can take your skills to the next level. This blog post will walk you through all aspects of functional programming in JavaScript, from the basic principles to advanced applications.
Introduction to Functional Programming
What Is Functional Programming?
Functional programming (FP) is a programming paradigm that treats computation as the evaluation of mathematical functions. Unlike imperative programming, which focuses on how to perform tasks, FP emphasizes what to perform using expressions and declarations.
Key Principles of Functional Programming
The foundational principles of functional programming include immutability, pure functions, higher-order functions, and function composition. These principles help create predictable, bug-free code by eliminating side effects and making functions easy to test and reuse.
Understanding Pure Functions
Definition and Characteristics of Pure Functions
A pure function is a function where its input values determine the output without observable side effects. This means no matter how many times you call a pure function with the same arguments, it will always return the same result.
Benefits of Using Pure Functions
Pure functions offer numerous advantages, such as easier testing, better debugging, and enhanced readability. Since they’re self-contained and predictable, pure functions can be effortlessly reused across different parts of your application.

Immutability in Functional Programming
What Is Immutability?
Immutability means that once a data structure is created, it cannot be changed. Instead of modifying existing data, operations produce new data structures. This concept is vital for avoiding unintended side effects and ensuring data integrity.
Techniques for Maintaining Immutability in JavaScript
JavaScript offers multiple ways to maintain immutability. You can use methods like `Object.freeze()` to prevent modifications, or libraries like Immutable.js to create immutable data structures effortlessly.
Higher-Order Functions: An Overview
What Are Higher-Order Functions?
Higher-order functions are functions that can take other functions as arguments or return them as results. They are a keystone in functional programming, allowing for more abstract and concise code.
Common Examples of Higher-Order Functions
Common higher-order functions include’map`, `filter`, ” and “reduce.” These functions accept another function as an argument and apply it to each element of a collection, making them incredibly versatile for data processing tasks.
Using Functions as First-Class Citizens
How Functions Are Treated as Values
In JavaScript, functions are first-class citizens, meaning they can be stored in variables, passed as arguments, and returned from other functions. This flexibility is essential for enabling powerful functional programming techniques.
Practical Examples of Function Manipulation
You can store functions in arrays, pass them as callbacks, or return them from other functions to create dynamic, high-order utilities. For instance, you could create a function that generates other functions based on given parameters.
Function Composition
What Is Function Composition?
Function composition involves combining multiple functions to produce a new function. This technique allows you to build complex operations by chaining simpler functions, thereby improving code readability and reusability.
Techniques for Composing Functions Together
You can compose functions manually or use utility libraries like Ramda and Lodash. Functions like `compose` and `pipe` are specifically designed to facilitate function composition in a straightforward, readable manner.
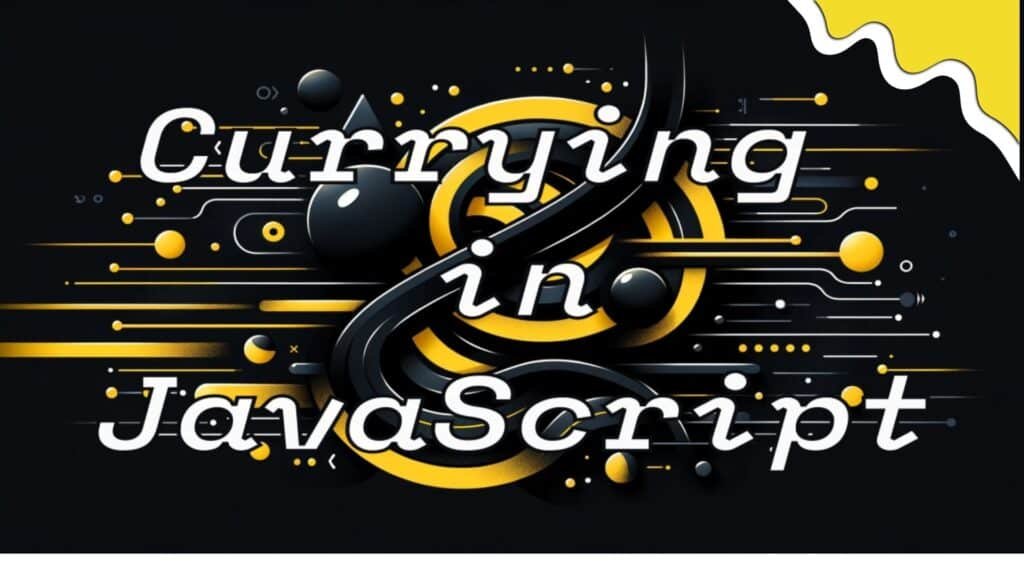
Currying and Partial Application
What Is Currying?
Currying is the process of transforming a function that takes multiple arguments into a series of functions that each take a single argument. This enables more flexible and reusable code.
Using Partial Applications to Create Customized Functions
Partial application involves fixing a few arguments of a function, producing a new function with fewer arguments. This technique is handy for creating specialized functions from general-purpose ones.
Declarative vs. Imperative Programming
Differences Between Declarative and Imperative Styles
Imperative programming focuses on describing how to achieve a result, using statements to change a program’s state. In contrast, declarative programming emphasizes what to achieve, using expressions and declarations.
Advantages of Declarative Programming in Functional Paradigms
Declarative programming simplifies the code by focusing on the ‘what’ rather than the ‘how’. It reduces complexity, making code easier to read and maintain.
Immutable Data Structures
Overview of Immutable Data Structures
Immutable data structures do not change after they are created. Modifications create new structures, leaving the original unchanged, which is crucial for functional programming.
Using Libraries Like Immutable. JS for immutability
Libraries like Immutable.js offer a range of immutable data structures such as List, Map, and Set. These libraries make it easier to implement immutability in JavaScript projects.
Map, Filter, and Reduce Functions
How’map`, `filter`, and’reduce” Align with Functional Programming
These functions are essential tools in functional programming. `map` transforms each element, `filter` selects elements that match criteria, and’reduce` combines elements into a single value.
Examples of Using These Functions Effectively
Using’map`, you can convert an array of numbers to their squares. With `filter`, you can extract even numbers from an array. `reduce` can sum all numbers in an array, demonstrating their versatility.
Avoiding Side Effects
What Are Side Effects?
Side effects occur when a function affects something outside its scope, such as modifying a global variable or writing to a file. This unpredictability makes code harder to debug and test.
Strategies for Writing Functions Without Side Effects
To avoid side effects, ensure that functions only depend on their input arguments and return values. Use local variables instead of global ones, and prefer immutable data structures.
Using `compose` and `pipe` Functions
How to Implement `compose` for Function Composition
The `compose` function combines multiple functions from right to left. It enables cleaner and more readable code by reducing nested function calls.
Using `pipe` to Create a Pipeline of Functions
The `pipe` function is similar to `compose` but works left to right. It creates a sequence of operations, making it easier to follow the flow of data transformations.

Functional Programming in Asynchronous Code
Applying functional concepts to promises and async/await
Functional programming principles can be applied to asynchronous code using Promises and async/await. These help manage asynchronous operations more predictably and maintainably.
Managing Async Operations with Functional Techniques
Use higher-order functions and function composition to handle asynchronous operations. Libraries like RxJS provide powerful tools for working with asynchronous streams.
Recursive Functions in Functional Programming
Understanding Recursion and Its Role
Recursion involves a function calling itself to solve a problem. It’s a common technique in functional programming for tasks like traversing trees and generating sequences.
Examples of Recursive Functions for Problem Solving
Examples include calculating factorials, Fibonacci numbers, and navigating nested structures. Recursion simplifies these tasks by breaking them into smaller, manageable sub-problems.
Implementing Functional Patterns in JavaScript
Common Functional Patterns and Practices
Common patterns include using higher-order functions, immutability, and pure functions. These practices enhance code clarity, maintainability, and testability.
Applying Functional Patterns to Real-World Problems
Functional patterns can solve real-world problems like data transformation, event handling, and state management. By applying these patterns, you can write cleaner, more efficient code.
Using Functional Programming Libraries
Overview of Libraries Like Lodash and Ramda
Lodash and Ramda are popular functional programming libraries for JavaScript. They provide a wide array of utility functions that simplify data manipulation and transformation.
How These Libraries Enhance Functional Programming
These libraries offer pre-built functions for common tasks, making it easier to implement functional programming principles. They reduce boilerplate code and improve code readability.
Testing Functional Code
Techniques for Testing Pure Functions
Testing pure functions is straightforward because their output depends solely on their input. Use unit tests to verify that each function behaves as expected.
Tools and Frameworks for Testing Functional JavaScript
Popular testing frameworks for JavaScript include Jest, Mocha, and Jasmine. These tools provide robust testing capabilities for verifying the correctness of functional code.
Performance Considerations in Functional Programming
Analyzing the Performance Implications of Functional Techniques
Functional programming can have performance overhead due to immutability and higher-order functions. However, these impacts are often negligible compared to the benefits.
Tips for Optimizing Functional Code
Optimize functional code by minimizing redundant calculations, using memoization, and leveraging efficient data structures. Profiling tools can help identify performance bottlenecks.
Practical Applications of Functional Programming
Streamlining Data Processing
Functional programming is particularly suited for data processing tasks where predictability, concurrency, and maintainability are crucial. By leveraging immutability and pure functions, developers can efficiently handle large data sets, ensuring that transformations and computations are consistent and robust. This approach is invaluable in fields like financial analytics and machine learning, where data integrity is paramount.
Enhancing reliability in backend development
In backend development, functional programming enhances the reliability and scalability of applications. The use of stateless functions allows for easy horizontal scaling and reduces the risk of side effects, which is critical for developing highly-tolerant distributed systems. As a result, functional paradigms are being adopted in microservice architectures to improve service isolation and robustness.
Improving User Interfaces with Declarative Programming
Functional programming principles, such as declarative code and component-based architecture, are widely used in front-end frameworks like React. These principles allow developers to create dynamic, responsive user interfaces that are easier to understand and maintain. By embracing state management techniques grounded in immutability and pure functions, UI components can be built to efficiently respond to user interactions and data changes.
Facilitating Test-Driven Development
Test-driven development (TDD) benefits from the predictability of functional code, as it simplifies writing and maintaining tests. Pure functions, which guarantee no hidden state changes, make it easier to create unit tests that are reliable and simple to set up. This approach not only increases the codebase’s stability but also ensures that new features and fixes do not introduce unintended side effects.
Enabling Predictable Software Behavior
By minimizing side effects, developers can create software components that are easy to reason about and debug. This predictability is essential in applications where precise behavior is required, such as real-time systems, robotics, and automated trading platforms. As a result, functional programming is increasingly adopted in industries that prioritize reliability and precision in software execution.
Case Studies: Functional Programming in Practice
Examples of Functional Programming in Modern Applications
Modern applications like React and Redux leverage functional programming principles. These frameworks promote immutability, pure functions, and declarative programming.
Lessons Learned from Real-World Use Cases
Real-world use cases demonstrate the scalability, maintainability, and testability benefits of functional programming. These examples highlight best practices and common pitfalls.
Future Trends in Functional Programming
Emerging Concepts and Features in Functional Programming
Emerging concepts include functional reactive programming and type-safe functional programming. These areas are gaining traction in the JavaScript community.
Predictions for the Evolution of Functional Programming in JavaScript
The future of functional programming in JavaScript looks promising. With increasing adoption and evolving best practices, functional programming is set to become a standard approach in web development.
Conclusion
Functional programming in JavaScript offers a plethora of benefits, from improved code clarity to enhanced maintainability. By understanding and applying its principles, JavaScript developers can write more robust, efficient, and scalable code. Whether you’re new to functional programming or looking to deepen your knowledge, these concepts will undoubtedly elevate your coding skills.
Ready to harness the power of functional programming? Start experimenting with these techniques and exploring how they can transform your approach to JavaScript development. For further learning, check out resources like online courses, documentation, and community forums dedicated to functional programming in JavaScript.