Object-Oriented Programming (OOP) is a paradigm that has revolutionized the way we approach software design and development. Among the core principles of OOP, inheritance and polymorphism stand out as fundamental concepts that empower developers to write reusable, modular, and maintainable code. In this comprehensive guide, we will explore inheritance and polymorphism in Python, their significance, and practical applications, providing valuable insights for Python developers and OOP learners.
Introduction to Inheritance and Polymorphism in Python
Inheritance and polymorphism in Python are two cornerstones of OOP that enable developers to create a hierarchy of classes and define behaviors that can be overridden or extended by subclasses. In Python, these concepts play a crucial role in creating scalable and efficient software.
One class (the subclass) can inherit properties and functions from another class (the superclass) through inheritance. This promotes code reuse and logical organization. Polymorphism, on the other hand, refers to the ability of different classes to respond to the same method call in different ways, providing flexibility and dynamic behavior in code.
In this guide, we will cover the basics of inheritance and polymorphism in Python, how to implement them, their real-world use cases, and best practices for effectively leveraging these concepts.
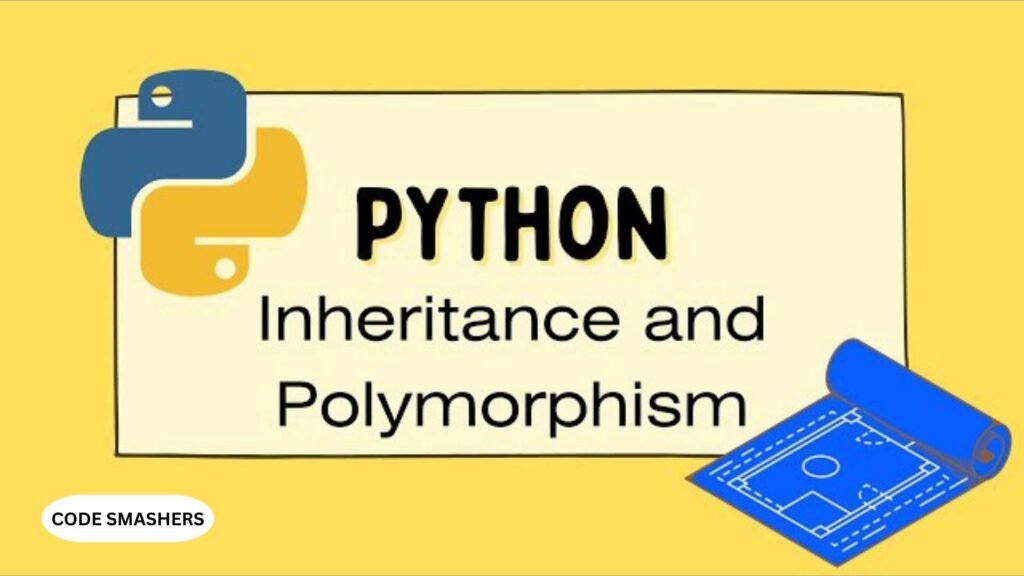
Understanding Inheritance: The Foundation of OOP
Inheritance is a mechanism that allows a new class to inherit the properties and behaviors of an existing class. The existing class is called the superclass or parent class, while the new class is called the subclass or child class. This relationship forms a hierarchy, enabling subclasses to reuse and extend the functionality of super classes.
Superclass and Subclass Relationships
In a superclass and subclass relationship, the subclass inherits all the attributes and methods of the superclass. This allows the subclass to utilize and override the inherited methods, providing specialized behavior while maintaining the common functionalities.
Example of inheritance in Python
Consider the following example:
class Animal:
definit(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return {self.name} says Woof!”
class Cat(Animal):
def speak(self):
return {self.name} says Meow!”
dog = Dog(“Buddy”)
cat = Cat(“Whiskers”)
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers say Meow!
In this example, the `Dog` and `Cat` classes inherit from the `Animal` class and override the `speak` method to provide specific behaviors.
Implementing Inheritance in Python: Syntax and Examples
Implementing inheritance in Python is straightforward. To define a subclass that inherits from a superclass, the syntax requires the use of parentheses. Let’s examine the syntax and a few real-world instances.
Basic Syntax of Inheritance
The basic syntax for creating a subclass is as follows:
class SubclassName(SuperclassName):
# class body
pass
Using the `super` Keyword
The `super` keyword allows you to call methods from the superclass in the subclass. This is very helpful if you wish to increase an inherited method’s capabilities.
Example:
class Parent:
definit(self, name):
self.name = name
def introduce(self):
return “I am {self.name}, the parent.”
class Child(Parent):
definit(self, name, age):
super().init(name)
self.age = age
def introduce(self):
parent_intro = super().introduce()
return f”{parent_intro} I am {self.age} years old.”
child = Child(“Alice”, 10)
print(child. introduce()) # Output: I am Alice, the parent. I am 10 years old.
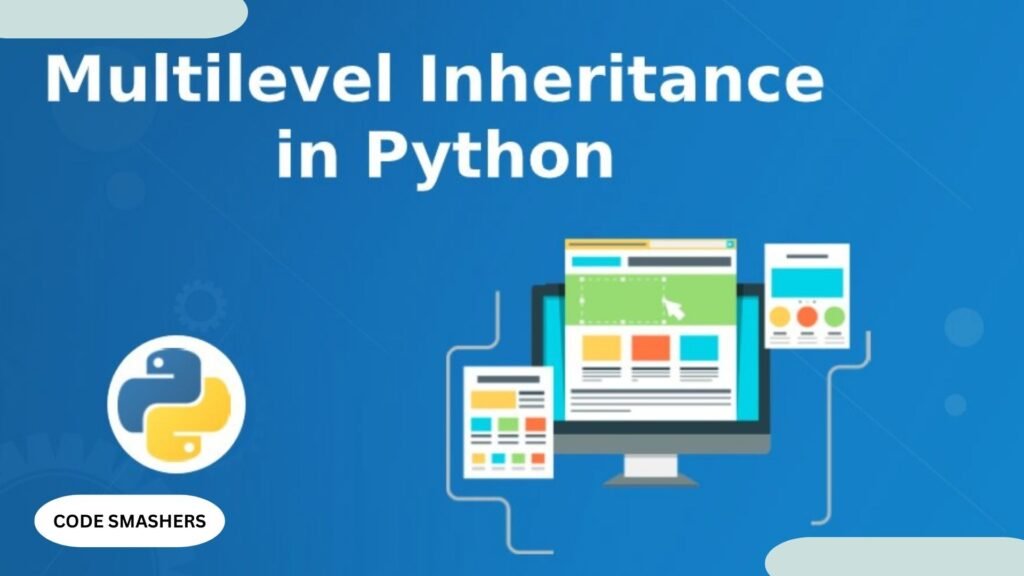
Multiple Levels of Inheritance
Python supports multiple levels of inheritance, where a subclass can inherit from another subclass, forming a chain of inheritance.
Example:
class Grandparent:
def describe(self):
return “I am the grandparent.”
class Parent(Grandparent):
def describe(self):
return f”{super().describe()} I am the parent.”
class Child(Parent):
def describe(self):
return f”{super().describe()} I am the child.”
child = Child()
print(child.describe()) # Output: I am the grandparent. I am the parent. I am the child.
Polymorphism in Python: Concept and Significance in OOP
The capacity of various classes to react differently to the same method call is known as polymorphism. In Python, polymorphism is achieved through method overriding and method overloading, allowing flexible and dynamic code.
Method Overriding
When a subclass offers a unique implementation of a method that is already defined in its superclass, this is known as method overriding. This enables the subclass to alter or expand the inherited method’s functionality.
Example:
class Bird:
def fly(self):
return “Birds can fly.”
class Penguin(Bird):
def fly(self):
return “Penguins cannot fly.”
bird = Bird()
penguin = Penguin()
print(bird.fly()) # Output: Birds can fly.
print(penguin.fly()) # Output: Penguins cannot fly.
Method Overloading
Method overloading allows a class to have many methods with the same name but different parameters. Python does not support method overloading directly, but similar behavior can be achieved using default arguments.
Example:
class MathOperations:
def add(self, a, b, c=0):
return a + b + c
math_op = MathOperations()
print(math_op.add(2, 3)) # Output: 5
print(math_op.add(2, 3, 4)) # Output: 9
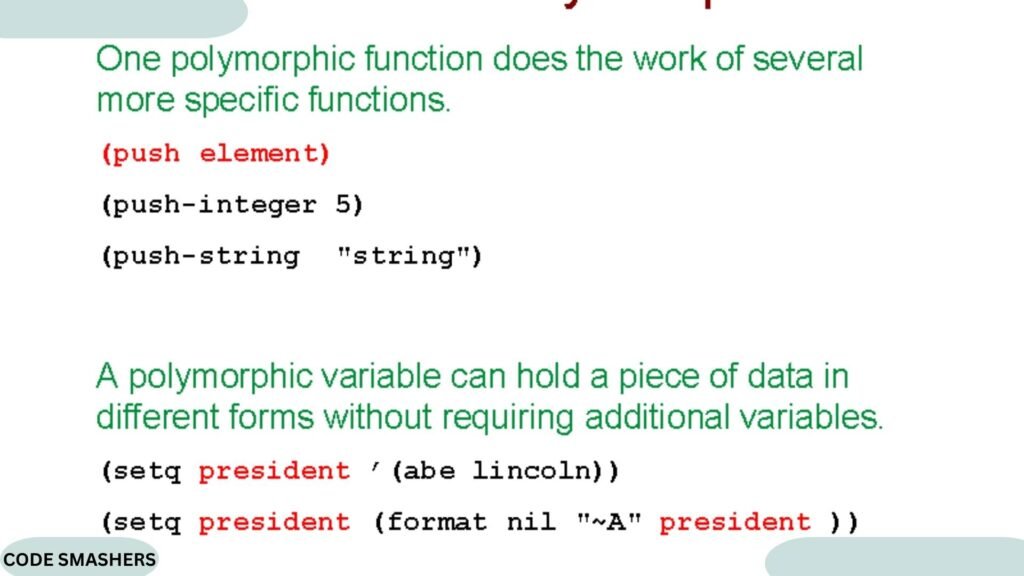
Benefits of Polymorphism
Polymorphism offers several advantages in object-oriented programming (OOP), which makes it a critical concept for building scalable and maintainable software. One primary benefit is code flexibility. Polymorphism allows for designing functions and classes that can work on objects of different types, enhancing code reusability and reducing redundancy. For instance, a single function can operate seamlessly on a collection of different objects, each providing its own implementation of a specific method.
This adaptability simplifies code maintenance and updates, as changes in one method do not require extensive rewrite or refactoring in the consuming code. Furthermore, polymorphism facilitates more intuitive and clear abstractions by allowing developers to interact with objects at a generic level without worrying about specific implementations. This abstraction capability leads to better-organized code, where high-level operations are decoupled from concrete manipulations of objects, ultimately supporting cleaner, more robust designs that align closely with real-world problem-solving approaches.
Case Study: Inheritance and Polymorphism in the Automotive Industry
In the automotive industry, inheritance and polymorphism are extensively deployed in the software development of vehicle control systems. For example, consider a base class `Vehicle` that represents core vehicle functionalities such as start, stop, speed up, and brake. More specific classes can inherit this foundational class, like `Car`, `Motorcycle`, and `Truck`, each of which might override certain methods to accommodate unique behaviors or constraints related to that vehicle type.
Python
class Vehicle:
def start(self):
return “Starting the vehicle.”
def stop(self):
return “Stopping the vehicle.”
class Car(Vehicle):
def start(self):
return “Starting the car with a push-button ignition.”
class Motorcycle(Vehicle):
def start(self):
return “Starting the motorcycle with a kick-starter.”
class Truck(Vehicle):
def start(self):
return “Starting the truck with key ignition.”
car = Car()
motorcycle = Motorcycle()
truck = Truck()
print(car.start()) Output: Starting the car with a push-button ignition.
print(motorcycle.start()) # Output: Starting the motorcycle with a kick-starter.
print(truck.start()) # Output: Starting the truck with key ignition.
This setup efficiently uses inheritance to position all vehicle classes under a unified hierarchy, while polymorphism allows each vehicle type to start in a manner that is suitable for its design and operation. Such a structure not only promotes clear code organization but also ensures scalability and flexibility when integrating further vehicle types or modifying existing functionalities.
Real-world Use Cases of Polymorphism in Python
Polymorphism is widely used in real-world applications to create flexible and extensible code. Let’s explore practical scenarios where polymorphism proves beneficial.
GUI Frameworks
In graphical user interface (GUI) frameworks, polymorphism allows different UI elements to be treated uniformly. For example, a button, text box, and label can all be drawn on the screen using a common `draw` method, even though each element has its own specific implementation.
Example:
class UIElement:
def draw(self):
pass
class Button(UIElement):
def draw(self):
return “Drawing a button.”
class TextBox(UIElement):
def draw(self):
return “Drawing a text box.”
elements = [Button(), TextBox()]
for element in elements:
print(element.draw())
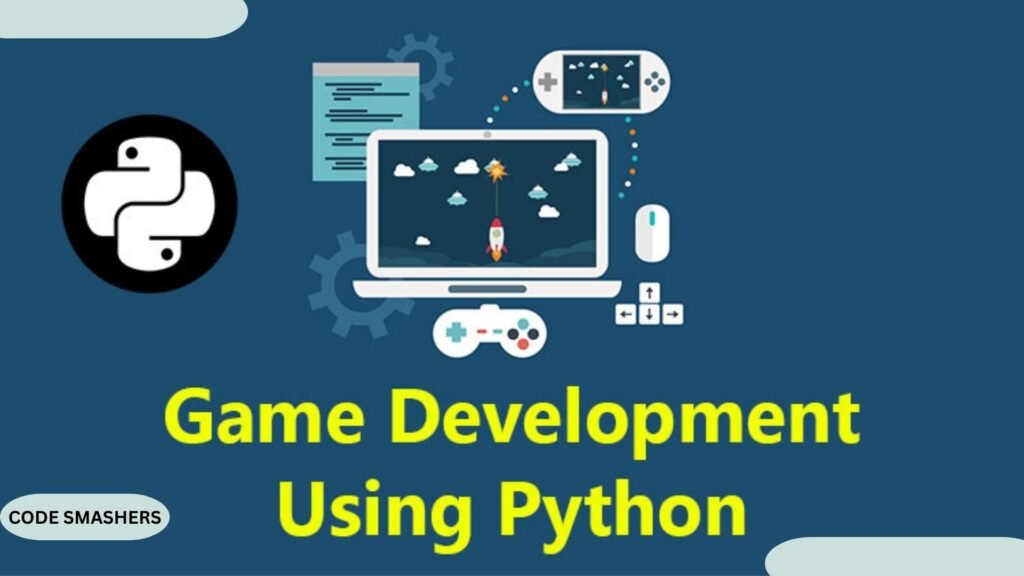
Game Development
In game development, polymorphism enables the creation of diverse game objects that share common behaviors. For instance, different types of enemies can have their own unique attack methods while still being treated as generic game objects.
Example:
class GameObject:
def attack(self):
pass
class Zombie(GameObject):
def attack(self):
return “Zombie attacks with bite.”
class Vampire(GameObject):
def attack(self):
return “Vampire attacks with fangs.”
enemies = [Zombie(), Vampire()]
for enemy in enemies:
print(enemy.attack())
Plugin Systems
Polymorphism is essential in building plugin systems where different plugins can be loaded and executed dynamically. Each plugin can implement a common interface, allowing the host application to interact seamlessly with various plugins.
Example:
class Plugin:
def execute(self):
pass
class PluginA(Plugin):
def execute(self):
return “Executing Plugin A.”
class PluginB(Plugin):
def execute(self):
return “Executing Plugin B.”
plugins = [PluginA(), PluginB()]
for plugin in plugins:
print(plugin.execute())
Best Practices for Using Inheritance and Polymorphism
While inheritance and polymorphism are powerful tools, it’s essential to follow best practices to ensure maintainable and efficient code.
Favor Composition Over Inheritance
In some cases, composition (where a class contains instances of other classes) is preferable to inheritance. Composition provides greater flexibility and decouples classes, making them easier to modify and test.
Avoid Deep Inheritance Hierarchies
Code with intricate inheritance hierarchies may be difficult to maintain. Aim for shallow hierarchies to keep your class structures simple and understandable.
Use Polymorphism Judiciously
While polymorphism offers flexibility, overuse can lead to confusing and hard-to-debug code. Use polymorphism when appropriate and ensure that method names and signatures remain consistent across subclasses.
Conclusion and Next Steps for Further Learning
Inheritance and polymorphism are essential concepts in Python’s OOP paradigm. By understanding and applying these principles, developers can create reusable, modular, and maintainable code. In this guide, we explored the basics of inheritance and polymorphism, their implementation in Python, real-world use cases, and best practices.
To continue your learning journey, consider exploring advanced topics, such as design patterns, metaclasses, and the SOLID principles of OOP. Engage with the Python community, participate in forums, and collaborate on projects to deepen your understanding.
Additional Resources
For further reading and hands-on practice, check out the following resources:
- Python Documentation on Classes
- OOP Design Patterns
- Real Python Tutorials
- Python Community on Reddit
By mastering inheritance and polymorphism, you’ll be well-equipped to tackle complex software projects and contribute to the Python community. Happy coding!