JS JavaScript is a powerful and flexible language that has become the backbone of modern web development. One of its most intriguing and sometimes perplexing features is its event loop and asynchronous operations. Understanding how these mechanisms work can elevate your coding skills and improve the performance and responsiveness of your applications. Let’s break down the concepts, mechanisms, and practical applications of the JS JavaScript event loop and asynchronous operations.
Introduction to the JS JavaScript Event Loop
Overview of the Event Loop Concept
At its core, the JS JavaScript event loop is a mechanism that allows the language to perform non-blocking operations despite being single-threaded. This means it can execute long-running tasks without freezing the entire program, giving users a smooth experience.

Importance of the Event Loop in JS JavaScript
The event loop is crucial because it enables JS JavaScript to handle asynchronous operations such as network requests, file I/O, and timers efficiently. Without it, JS JavaScript would not manage multiple tasks simultaneously, leading to unresponsive applications.
Understanding the JS JavaScript Concurrency Model
How JS JavaScript Handles Concurrency
JS JavaScript handles concurrency using a combination of the call stack, the event loop, and various queues. The call stack is where functions are executed, while the event loop manages the execution of tasks queued for later processing.
The Role of the Call Stack and Event Queue
When an asynchronous operation completes, its callback is added to the event queue. The event loop then processes these callbacks one by one, ensuring they don’t interfere with the execution of other code on the call stack.
The Event Loop Mechanism Explained
How the Event Loop Processes Events
The event loop continuously checks the call stack and the event queue. If the call stack is empty, it takes the first task from the event queue and pushes it onto the call stack for execution. This loop ensures that tasks are processed in a non-blocking manner.
The Role of the Microtask Queue and Macrotask Queue
JS JavaScript maintains two types of queues for managing tasks—microtask and macrotask queues. Microtasks have higher priority and are processed before any macrotasks. This distinction helps optimize the execution of asynchronous code for better performance.
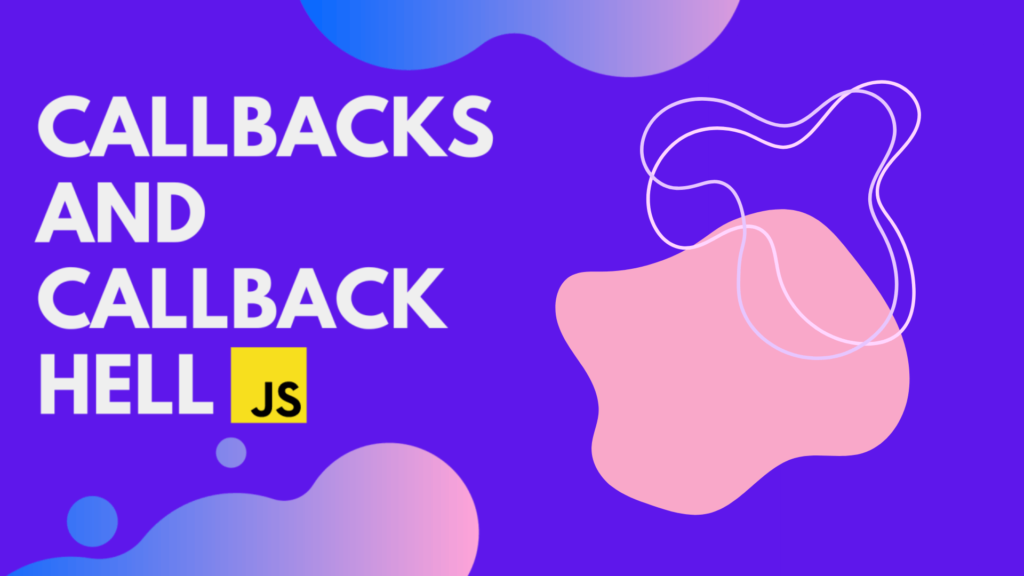
Handling Callbacks in JS JavaScript
What Are Callbacks?
Callbacks are functions passed as arguments to other functions, which are then invoked once the initial function completes its task. They are a fundamental concept in asynchronous programming.
How Callbacks Fit into the Event Loop
When an asynchronous operation completes, its callback is placed in the event queue. The event loop then processes these callbacks, executing them when the call stack is empty.
Callback Hell and the Pyramid, the Pyramid of Doom
What Is Callback Hell?
Callback hell, also known as the pyramid of doom, occurs when multiple nested callbacks create hard-to-read and maintain code. This often happens in complex applications that rely heavily on asynchronous operations.
Techniques for Managing Nested Callbacks
To avoid callback hell, developers can use techniques such as modularizing code, using named functions, and employing more advanced constructs like promises and async/await.
Introduction to Promises
What Are Promises and Their States?
Promises are objects representing the eventual completion or failure of an asynchronous operation. They have three states: pending, fulfilled, and rejected. Promises provide a cleaner and more manageable way to handle asynchronous code compared to callbacks.
How Promises Work with the Event Loop
When a promise is resolved or rejected, its corresponding handlers (.then(),.catch(),.finally.then(),.catch(),.finally()) are added to the microtask queue. The event loop processes these handlers after the current code execution completes.
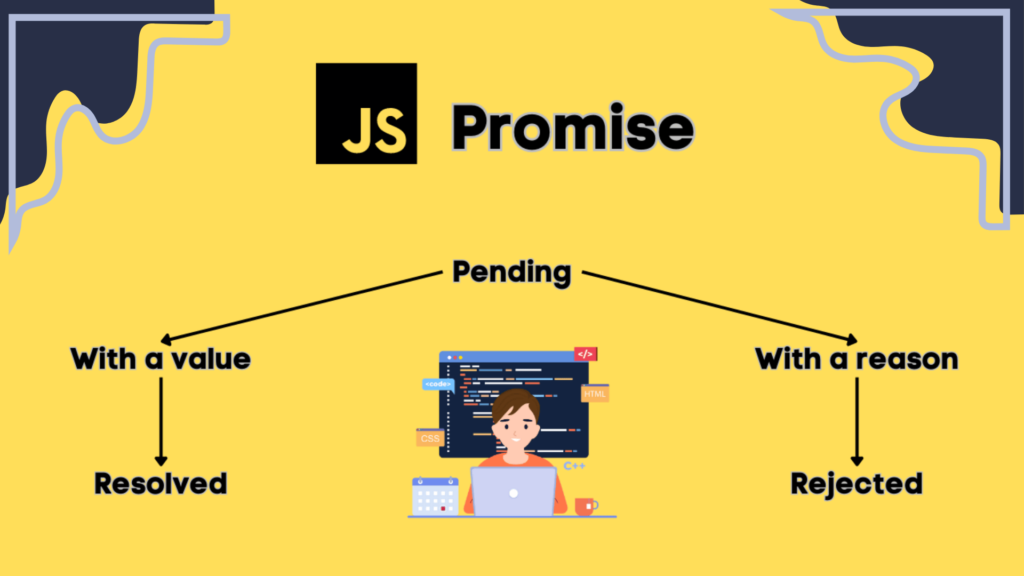
Chaining promises and error handling
Using `.then()`, `.catch()`, and `.finally()`
Promise chaining allows developers to execute multiple asynchronous operations sequentially. The.then() method handles fulfilled promises,.catchpromises,.catch() deals with rejections, and.finallyand.finally() runs code regardless of the promise’s outcome.
Managing Errors in Promise Chains
Proper error handling in promise chains is crucial to prevent unhandled rejections and ensure robust code. Using catchcatch() at the end of a promise chain helps catch and manage errors effectively.
Async/Await Syntax for Asynchronous Code
Introduction to `async` and `await`
The async/await syntax provides a more readable and synchronous way to write asynchronous code. An async function always returns a promise, and the await keyword pauses the execution of the function until the promise is resolved.
How `async/await` Simplifies Asynchronous Code
Async/await simplifies code by eliminating the need for chained.then() methods, making it easier to write and understand. It also helps manage asynchronous operations in a more structured manner.
The Interaction Between `async/await` and the Event Loop
How `await` Pauses Execution
When the await keyword is used, the execution of the async function is paused until the awaited promise is resolved. This pause allows other code to run, ensuring non-blocking behavior.
Managing Asynchronous Code Execution with `async`
Async functions allow developers to write non-blocking code that appears synchronous. By combining async with await, complex asynchronous operations can be simplified and made more readable.
Microtasks vs. Macrotasks
Differences Between Microtasks and Macrotasks
Microtasks are tasks that need to be executed immediately after the currently executing script, while macrotasks are scheduled to run after the microtasks. Examples of microtasks include promise callbacks, while macrotasks include setTimeout and setInterval.
How Each Affects the Event Loop
Microtasks have higher priority and are executed before any pending macrotasks. This prioritization ensures that critical tasks are handled promptly, improving the performance and responsiveness of applications.
Handling Asynchronous Operations with’setTimeoutwith’setTimeout` and’setInterval”and’setInterval”
Using’setTimeout Using’setTimeout` and’setInterval` for delayed execution
The setTimeout and setInterval functions allow developers to schedule code execution after a specified delay. These functions are useful for creating time-based events and animations in web applications.
How These Methods Interact with the Event Loop
When setTimeout or setInterval is called, the specified callback is added to the macrotask queue. The event loop processes these callbacks after all microtasks have been executed.
Event Loop and UI Rendering
How the Event Loop Affects User Interface Updates
The event loop plays a crucial role in updating the user interface. Processing tasks efficiently ensures smooth and responsive UI interactions, even during heavy computations.
Techniques for Ensuring Smooth UI Performance
To maintain a smooth UI, developers should minimize long-running tasks, use requestAnimationFrame for animations, and leverage web workers for concurrent operations.
Asynchronous Iteration with `for await…of`
Syntax and Use Cases of `for await…of`
The for await…of syntax allows developers to iterate over asynchronous data sources, such as streams or APIs. It provides a clean and readable way to handle asynchronous iterations.
Iterating Over Async Data Sources
By using for await…of, developers can process data from asynchronous sources sequentially, ensuring that each iteration completes before moving to the next.

Performance Considerations for Asynchronous Code
Impact of Asynchronous Operations on Performance
While asynchronous operations can improve the responsiveness of applications, they can also introduce performance bottlenecks if not properly managed. Developers should be mindful of the impact of these operations on performance.
Optimizing Asynchronous Code Execution
To optimize asynchronous code, developers should use techniques such as debouncing, throttling, and batching. These techniques help manage the frequency and timing of asynchronous operations, improving performance.
Debugging Asynchronous Code and the Event Loop
Tools and Techniques for Debugging
Debugging asynchronous code can be challenging, but modern tools like Chrome DevTools and Visual Studio Code offer features to inspect and debug async operations effectively.
Common issues and how to resolve them
Common issues in asynchronous code include race conditions, unhandled rejections, and callback hell. By understanding these issues and using appropriate debugging techniques, developers can resolve them effectively.
Using Web Workers for Concurrency
Introduction to Web Workers
Web workers enable developers to run scripts in background threads, allowing concurrent execution of code. This approach helps offload heavy computations from the main thread, improving application performance.
How Web Workers Complement the Event Loop
Web workers operate independently of the event loop, enabling true parallelism in JS JavaScript applications. They communicate with the main thread via message passing, ensuring efficient and responsive performance.
Handling Concurrency and Parallelism
Differences Between Concurrency and Parallelism
Concurrency involves executing multiple tasks simultaneously, while parallelism involves dividing a task into subtasks that run concurrently on multiple processors. Understanding these concepts is crucial for effective asynchronous programming.
Techniques for Achieving Concurrency in JS JavaScript
Techniques such as using promises, async/await, and web workers help achieve concurrency in JS JavaScript. By leveraging these techniques, developers can build responsive and efficient applications.
Event Loop and Node.js
How the Event Loop Works in Node.js
Node.js uses the same event loop mechanism as the browser, but with additional features tailored for server-side applications. Understanding the event loop in Node.js is essential for building scalable and performantperformant back-end systems.
Differences Between Browser and Node.js Event Loops
While the core event loop concepts are the same, Node.js introduces additional constructs like the Libuv library for handling I/O operations. These differences impact how asynchronous code is executed in Node.js compared to the browser.
Case Studies: Real-World Examples of Asynchronous Code
Examples of Asynchronous Operations in Modern Applications
Real-world examples of asynchronous code include web applications that fetch data from APIs, handle user interactions, and perform background processing. Studying these examples helps developers understand practical use cases of asynchronous programming.
Lessons Learned from Real-World Use Cases
Analyzing real-world use cases provides valuable insights into common challenges and best practices for writing asynchronous code. These lessons help developers build more robust and maintainable applications.
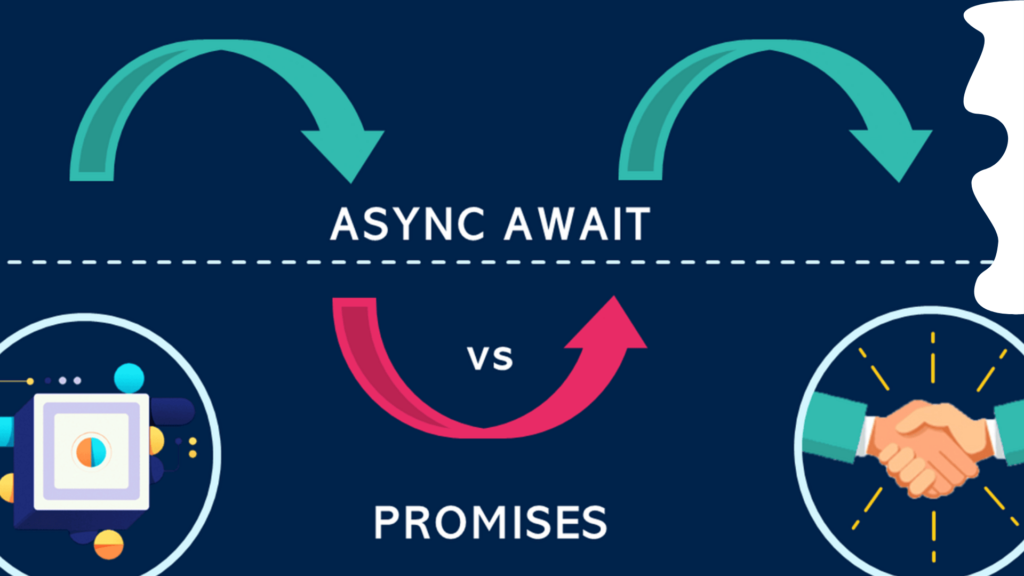
Future Trends in Asynchronous JS JavaScript
Emerging Features and Proposals for Asynchronous Programming
New features and proposals, such as async iterators, top-level await, and worker threads, are shaping the future of asynchronous JS JavaScript. Staying informed about these trends helps developers prepare for upcoming changes.
Predictions for the Evolution of Asynchronous Patterns
As JS JavaScript continues to evolve, new patterns and techniques for asynchronous programming will emerge. Understanding these trends helps developers stay ahead of the curve and leverage the latest advancements in their projects.
In conclusion, mastering the JS JavaScript event loop and asynchronous operations is essential for modern web development. By understanding these concepts and applying best practices, developers can build responsive, efficient, and maintainable applications. Whether you’re a beginner or an experienced developer, this guide provides valuable insights and practical tips to enhance your skills and knowledge. Happy coding!