In the dynamic world of web development, storing data effectively is pivotal for creating seamless and responsive user experiences. Whether you’re a seasoned developer or just starting, understanding web storage techniques like Local Storage and Session Storage is essential. These tools offer efficient ways to store data directly on a user’s browser, making your applications faster and more reliable. In this guide, we’ll explore the intricacies of web storage, providing you with the knowledge needed to leverage these techniques in your projects.
Introduction to Web Storage in JavaScript
Web storage is a vital part of modern web development. It allows you to store data locally within a user’s browser without needing a server database. This means you can create faster, more responsive applications. Web storage in JavaScript is typically divided into two categories: Local Storage and Session Storage . Both offer unique benefits and are crucial for different scenarios in web applications. By the end of this guide, you’ll understand how to utilize these tools effectively in your projects.
What is Local Storage ?
Local Storage is a type of web storage that allows you to store data with no expiration date. This means the data persists even after the browser is closed or reopened. It’s perfect for saving user preferences, login information, or any data that needs to be retained over time. Local Storage offers a simple and efficient way to store key-value pairs, making it easy to retrieve and manipulate data when needed. Understanding its capabilities is essential for creating persistent user experiences.
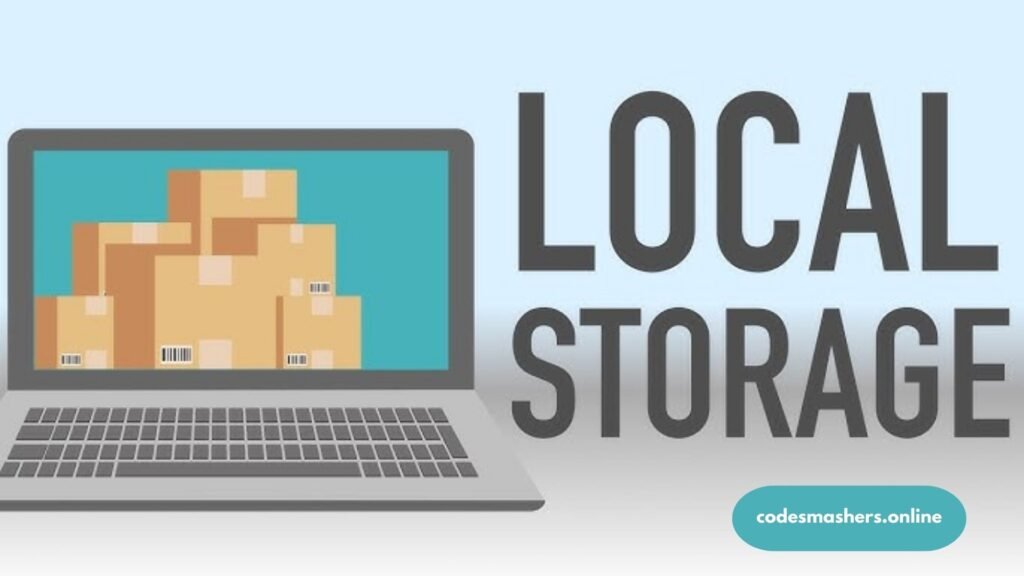
What is Session Storage ?
Unlike Local Storage , Session Storage stores data for the duration of a page session. This means the data is lost when the tab or window is closed. It’s ideal for storing temporary data, such as form inputs or session-specific settings. Session Storage provides the same API as Local Storage , allowing for easy implementation and retrieval of data. Knowing when to use Session Storage can enhance the functionality and responsiveness of your web applications.
Differences Between Local Storage and Session Storage
While Local Storage and Session Storage may seem similar, they serve different purposes. The primary difference lies in their data persistence. Local Storage is persistent across sessions, whereas Session Storage is not. Local Storage is better suited for data that needs to be retained long-term, like user preferences or settings. On the other hand, Session Storage is optimal for temporary data that only needs to exist during a single session, such as form data on a checkout page. Understanding these differences is crucial for deciding which storage method to use in your projects.
Benefits of Using Web Storage Over Cookies
Web storage offers several advantages over traditional cookies. Firstly, web storage provides a larger storage capacity, allowing up to 5MB of data, compared to the mere 4KB provided by cookies. Secondly, data stored in web storage is not sent to the server with every HTTP request, enhancing performance by reducing unnecessary data transfer. Finally, web storage provides a simple API for storing and retrieving data, making it easier to work with than cookies. These benefits make web storage a superior choice for modern web applications.
How Local Storage Works in JavaScript
Local Storage is part of the Window interface in JavaScript. It allows you to store data in key-value pairs, with each key being a string. Setting data in Local Storage is straightforward using `Local Storage .setItem(key, value)`, and retrieving data is just as simple with `Local Storage .getItem(key)`. This ease of use makes Local Storage an excellent choice for storing persistent data. Understanding these basic operations is the first step in mastering Local Storage .
How Session Storage Works in JavaScript
Session Storage functions similarly to Local Storage but with a few key differences. Session Storage stores data for a single session, meaning the data is deleted when the session ends. To store data, use `Session Storage .setItem(key, value)`, and retrieve it with `Session Storage .getItem(key)`. This makes Session Storage ideal for temporary data that only needs to exist during a session. Understanding how to effectively use Session Storage can greatly enhance the user experience in your web applications.
Storing Data in Local Storage
Storing data in Local Storage is simple and effective. You can use the `setItem` method to save data in the form of key-value pairs. For example, to store a user’s preferred theme, use `Local Storage .setItem(‘preferredTheme’, ‘dark’)`. This method ensures that the data remains accessible across sessions. By consistently using Local Storage for persistent data, you can create applications that remember user preferences and settings.
Storing Data in Session Storage
Session Storage is perfect for storing data that only needs to persist during a single session. To store data, use `Session Storage .setItem(‘userLanguage’, ‘en-US’)`. This method is ideal for saving temporary data, such as a user’s language preference during a single visit. By utilizing Session Storage for session-specific data, you can enhance the responsiveness and efficiency of your applications.
Retrieving Data from Local Storage
Retrieving data from Local Storage is straightforward with the `getItem` method. For example, `Local Storage .getItem(‘preferredTheme’)` will return the stored theme preference. This method allows you to easily access and manipulate persistent data. By efficiently retrieving data from Local Storage , you can create applications that provide a seamless and personalized user experience.
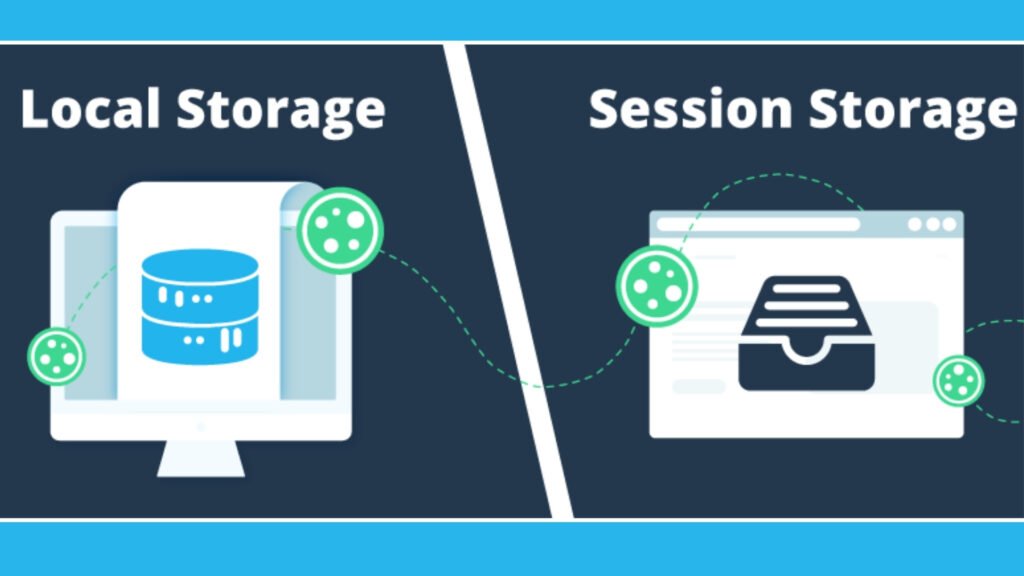
Retrieving Data from Session Storage
Accessing data in Session Storage is similar to Local Storage . Use `Session Storage .getItem(‘userLanguage’)` to retrieve the stored language preference. This method ensures that temporary session data can be accessed and used efficiently. By effectively retrieving data from Session Storage , you can maintain continuity and responsiveness in your applications, even within a single session.
Removing Data from Local Storage
Removing data from Local Storage is easy with the `removeItem` method. For instance, `Local Storage .removeItem(‘preferredTheme’)` will delete the stored theme preference. This method is useful for managing and updating stored data as needed. By understanding how to remove data from Local Storage , you can maintain accurate and relevant data in your applications.
Removing Data from Session Storage
Session Storage allows you to remove data quickly with the `removeItem` method. Use `Session Storage .removeItem(‘userLanguage’)` to delete the stored language preference. This method is essential for managing temporary session data efficiently. By mastering data removal in Session Storage , you can ensure your applications remain responsive and up-to-date throughout sessions.
Clearing All Data from Local Storage
Clearing all data from Local Storage can be done with the `clear` method. Simply use `Local Storage .clear()` to remove all stored data. This method is beneficial for resetting data and starting fresh. By knowing how to clear Local Storage , you can manage data effectively and ensure your applications function smoothly.
Clearing All Data from Session Storage
Session Storage offers an easy way to clear all data with the `clear` method. Use `Session Storage .clear()` to remove all session-specific data. This method is useful for resetting a session’s data entirely. By understanding how to clear Session Storage , you can manage session data effectively and maintain a responsive user experience.
Storing Complex Objects in Local Storage and Session Storage
Storing complex objects in web storage requires converting them to a string format. By using `JSON.stringify(object)`, you can store objects as strings. Retrieve them using `JSON.parse(string)`. This method allows you to store and manipulate complex data structures, enhancing the functionality and flexibility of your applications.
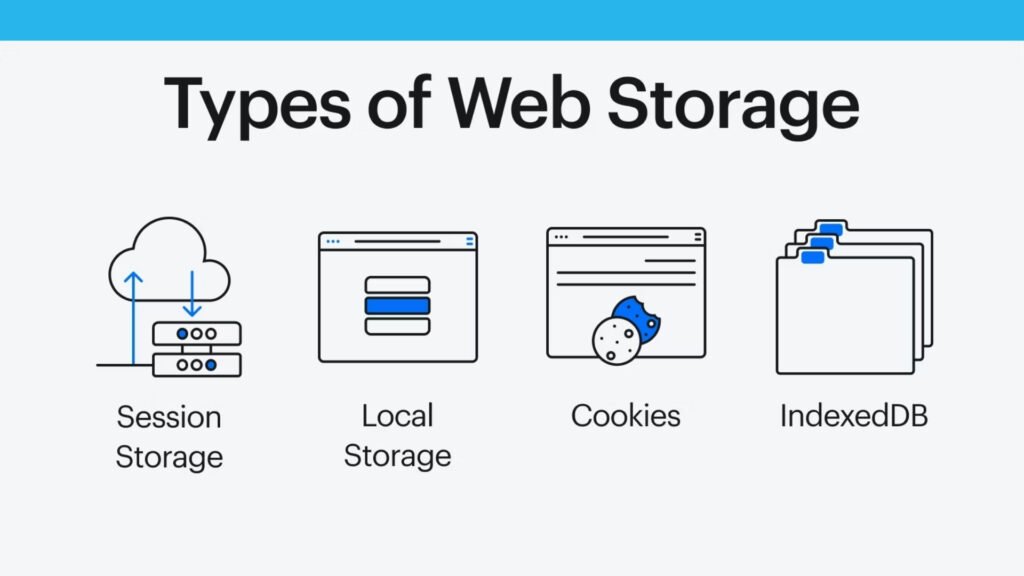
Web Storage Limits and Quotas
Web storage has limits and quotas, typically allowing 5MB of data per origin. It’s important to monitor storage usage and ensure data is managed efficiently. Understanding these limitations helps you optimize storage strategies and maintain performance in your web applications.
Security Considerations for Using Web Storage
Security is crucial when using web storage. Avoid storing sensitive data, as web storage is accessible to any script running on the page. Implementing security best practices, such as data encryption and access controls, ensures your applications remain secure and trustworthy.
Understanding the Limitations of Web Storage
While web storage offers a convenient method for storing data locally, it does come with several limitations that developers must navigate. The most notable constraint is the storage size limit, which typically allows for up to 5MB of data per origin. This restriction can vary based on the browser and device, but it’s important to plan around these maximum capacities to ensure that your applications run smoothly without data overwrites or loss.
Additionally, web storage operates on a per-origin basis, meaning that data is isolated to the protocol, domain, and port. This means that data stored for `http://example.com` is not accessible to `https://example.com`. Another important limitation is that web storage data is only accessible to the client-side JavaScript and cannot be automatically sent to a server, requiring explicit requests for data synchronization between the client and server. These limitations necessitate careful management and optimization of storage strategies to maintain the performance and reliability of web applications.
Best Practices for Using Local Storage and Session Storage
Following best practices ensures effective use of web storage. Use Local Storage for persistent data and Session Storage for temporary data. Regularly update and clear unused data to optimize performance. By adhering to these guidelines, you can create robust and efficient web applications.
1. Use Web Storage for Non-Sensitive Data Only
Best Practice:
Never store sensitive or personal data (e.g., passwords, financial details) in Local Storage or Session Storage, as the data is stored as plain text and is accessible via JavaScript. For secure storage, consider using server-side storage or encrypting the data before saving it.
Example:
// Avoid storing sensitive data like passwords directly
localStorage.setItem('username', 'user123'); // Okay
localStorage.setItem('password', 'securePassword'); // Not secure
2. Serialize Complex Data Types
Best Practice:
Since Web Storage can only store strings, always serialize objects, arrays, and other non-string data types before saving them. Use JSON.stringify()
to convert objects/arrays into strings and JSON.parse()
to convert them back.
Example:
let user = { name: 'John', age: 30 };
localStorage.setItem('user', JSON.stringify(user)); // Store serialized object
let storedUser = JSON.parse(localStorage.getItem('user')); // Retrieve and deserialize
console.log(storedUser.name); // Output: John
3. Limit Storage Size
Best Practice:
Local Storage typically offers around 5MB of space per domain, and Session Storage is usually limited to the same. Be mindful of storage limits and avoid storing too much data. Implement checks before saving large amounts of data to prevent exceeding the storage quota.
Example:
try {
localStorage.setItem('key', 'someLargeData');
} catch (e) {
if (e.name === 'QuotaExceededError') {
console.log('Local storage limit exceeded.');
}
}
4. Use Web Storage for State Management, Not as a Database
Best Practice:
Use Local Storage and Session Storage for storing small amounts of data like user preferences, form inputs, or session state, rather than as a full-fledged database. Web Storage is not designed for handling complex queries, relationships, or large datasets.
Example:
// Good use case
localStorage.setItem('theme', 'dark'); // Store user preference
// Bad use case: Trying to store an entire user profile with complex relationships
localStorage.setItem('userProfile', JSON.stringify({ /* large object */ }))
5. Handle Data Expiry Properly
Best Practice:
Unlike cookies, Local Storage and Session Storage do not automatically expire. If you need to store data with an expiration time, implement custom expiration logic by adding a timestamp when you store the data, and check the timestamp before using the data.
Example:
// Set data with an expiration time of 1 hour
let data = {
value: 'someValue',
timestamp: Date.now()
};
localStorage.setItem('data', JSON.stringify(data));
// Check expiry before use
let storedData = JSON.parse(localStorage.getItem('data'));
if (Date.now() - storedData.timestamp > 3600000) { // 1 hour = 3600000 ms
console.log('Data expired');
localStorage.removeItem('data');
}
6. Clear Data Appropriately
Best Practice:
Clear data from Local Storage or Session Storage when it’s no longer needed. This helps in avoiding potential data leakage or cluttering the storage. Use localStorage.removeItem()
or sessionStorage.removeItem()
for specific items, and localStorage.clear()
or sessionStorage.clear()
for clearing all data.
Example:
// Remove specific item
localStorage.removeItem('theme');
// Clear all items from localStorage
localStorage.clear();
7. Be Aware of Cross-Tab and Cross-Browser Behavior
Best Practice:
Local Storage is shared across different tabs and windows in the same browser, but Session Storage is isolated to a single tab. Be cautious when relying on storage data across multiple tabs, and use the storage
event to synchronize data between tabs or windows.
Example:
// Listen for storage changes across tabs
window.addEventListener('storage', function(event) {
console.log('Data updated:', event);
});
8. Use Descriptive Keys
Best Practice:
Choose descriptive and meaningful keys for your storage items to make your code more readable and maintainable. Avoid generic names like “data” or “value,” which could lead to confusion in larger applications.
Example:
// Good key naming
localStorage.setItem('userPreferences.theme', 'dark');
localStorage.setItem('session.cart', JSON.stringify(cart));
// Bad key naming
localStorage.setItem('data1', 'value');
9. Test for Browser Compatibility
Best Practice:
Although most modern browsers support Web Storage, it’s always a good idea to check for availability before using Local Storage or Session Storage to ensure compatibility with older browsers.
Example:
if (typeof(Storage) !== "undefined") {
// Local Storage or Session Storage is supported
localStorage.setItem('user', 'John');
} else {
console.log('Web Storage not supported in this browser');
}
10. Handle Edge Cases
Best Practice:
Ensure your code handles potential edge cases, such as exceeding storage limits, retrieving non-existent items, or parsing corrupted data. Always use error handling techniques like try...catch
to manage unexpected issues.
Example:
try {
let user = JSON.parse(localStorage.getItem('user'));
console.log(user.name);
} catch (e) {
console.log('Error parsing stored data', e);
}
11. Avoid Overusing Web Storage for Large Files
Best Practice:
Web Storage is not designed for storing large files like images, videos, or other binary data. Use specialized storage solutions like IndexedDB for larger datasets or consider uploading large files to a server and storing metadata in Local Storage.
12. Keep the UI Synchronized with Storage
Best Practice:
When Web Storage is used to store user preferences or application state, ensure that the UI reflects the changes in real-time. For example, if the theme preference is saved in Local Storage, the page should immediately reflect the change when the page is reloaded.
Example:
// Apply theme from localStorage on page load
let theme = localStorage.getItem('theme');
if (theme) {
document.body.classList.add(theme);
}
13. Use Session Storage for Temporary Data
Best Practice:
Use Session Storage for data that only needs to persist within a single session (e.g., form data, temporary settings). This ensures that once the user closes the tab, the data is automatically discarded, reducing the risk of data being stored unnecessarily.
14. Avoid Storing Too Many Items in Storage
Best Practice:
While Local Storage can hold many items, too many entries can slow down your web application. Store only essential data, and periodically clean up old or unused items.
15. Document Your Use of Web Storage
Best Practice:
Document where and why Web Storage is used in your codebase. This helps other developers (and your future self) understand the purpose of the stored data, the structure of the keys, and any expiration logic in place.
Examples of Local Storage and Session Storage in Real-world Applications
Real-world examples illustrate the power of web storage. A weather app stores user preferences in Local Storage , providing a personalized experience. An e-commerce site uses Session Storage for shopping carts, maintaining data across sessions. Combining both enhances functionality and user satisfaction.
Conclusion
Understanding and mastering web storage techniques like Local Storage and Session Storage is essential for modern web development. By utilizing these tools, you can create applications that are efficient, responsive, and user-friendly. Implementing best practices and security measures ensures your applications remain robust and reliable. Whether you’re developing a weather app or an e-commerce site, web storage plays a crucial role in delivering exceptional user experiences. Explore these techniques further and elevate your web development skills to new heights.
Frequently Asked Questions
1. What is Web Storage in JavaScript?
Answer:
Web Storage is a feature in HTML5 that allows web applications to store data locally in the browser. It provides two storage options: Local Storage and Session Storage. Local Storage persists data even after the browser is closed, while Session Storage is only available for the duration of the session.
2. What is the difference between Local Storage and Session Storage?
Answer:
- Local Storage: Data persists even when the browser is closed and reopened.
- Session Storage: Data is only available for the duration of the page session, i.e., as long as the browser tab is open.
3. How do I set data in Local Storage using JavaScript?
Answer:
You can use the localStorage.setItem()
method to store data:
localStorage.setItem('key', 'value');
4. How do I retrieve data from Local Storage?
Answer:
You can use the localStorage.getItem()
method to retrieve data:
let value = localStorage.getItem('key');
console.log(value);
5. How do I remove data from Local Storage?
Answer:
Use the localStorage.removeItem()
method to remove an item:
localStorage.removeItem('key');
6. Can I store objects in Local Storage or Session Storage?
Answer:
Both Local Storage and Session Storage store data as strings. To store objects, you need to serialize them using JSON.stringify()
and deserialize them with JSON.parse()
:
let obj = { name: 'John', age: 30 };
localStorage.setItem('user', JSON.stringify(obj));
// Retrieving the object
let user = JSON.parse(localStorage.getItem('user'));
console.log(user.name); // John
7. What is the maximum storage capacity for Local Storage?
Answer:
Most browsers allow around 5MB of data per domain for Local Storage. However, this limit can vary slightly across different browsers.
8. Can data in Session Storage be accessed across different tabs?
Answer:
No, Session Storage is only available to the tab where it was created. It is not shared across tabs or windows, unlike Local Storage, which is shared across all tabs for the same domain.
9. Is Web Storage (Local and Session) secure?
Answer:
Web Storage is not encrypted, so sensitive information (like passwords or personal data) should not be stored in Local or Session Storage. Always encrypt sensitive data before storing it.
10. How can I clear all data from Local Storage?
Answer:
Use the localStorage.clear()
method to remove all data from Local Storage:
localStorage.clear();
11. Can I store arrays in Local Storage or Session Storage?
Answer:
Yes, you can store arrays, but like objects, they need to be serialized into a string using JSON.stringify()
before storing:
let arr = [1, 2, 3];
localStorage.setItem('numbers', JSON.stringify(arr));
// Retrieving the array
let numbers = JSON.parse(localStorage.getItem('numbers'));
console.log(numbers);
12. What happens if I try to store data larger than the storage limit?
Answer:
If you exceed the storage limit, the browser will throw a QuotaExceededError
. It is a good practice to check the storage space before attempting to store large data.
13. How can I store data in Session Storage?
Answer:
You can use the sessionStorage.setItem()
method:
sessionStorage.setItem('key', 'value');
14. How do I get data from Session Storage?
Answer:
Use the sessionStorage.getItem()
method to retrieve data:
let value = sessionStorage.getItem('key');
console.log(value);
15. How long does data in Session Storage persist?
Answer:
Data in Session Storage persists only for the duration of the page session. Once the browser tab is closed, the data is cleared.
16. Can I check if an item exists in Local or Session Storage?
Answer:
You can check if an item exists using getItem()
:
if (localStorage.getItem('key') !== null) {
console.log('Item exists');
}
17. How do I handle errors when using Web Storage?
Answer:
You can use try...catch
to handle potential errors, such as exceeding storage limits or trying to access non-existent items:
try {
localStorage.setItem('key', 'value');
} catch (e) {
console.log('Error:', e);
}
18. Does Web Storage work in all browsers?
Answer:
Web Storage (Local Storage and Session Storage) is supported by all modern browsers, including Chrome, Firefox, Safari, Edge, and Opera. However, it is always good practice to check for support using if (typeof(Storage) !== "undefined")
.
19. Can I use Local Storage or Session Storage with cookies?
Answer:
Yes, you can use Web Storage alongside cookies, but they serve different purposes. Cookies are sent with every HTTP request, while Local and Session Storage are client-side only and do not get transmitted with requests.
20. How do I listen for changes to Local Storage or Session Storage?
Answer:
You can use the storage
event to listen for changes in Local Storage across different windows or tabs:
window.addEventListener('storage', function(event) {
console.log('Storage changed:', event);
});