JavaScript developers, are you ready to deepen your understanding of Object-Oriented Programming (OOP) in JavaScript? This blog post will guide you through the fascinating world of OOP, exploring the evolution from traditional prototypes to modern ES6 classes. Whether you’re an experienced developer or just starting, you’ll gain practical insights into how these concepts can enhance your JavaScript projects.
Introduction to Object-Oriented Programming in JavaScript
Object-Oriented Programming (OOP) is a paradigm that uses “objects” to design software. In JavaScript, OOP allows developers to create more modular, reusable, and scalable code. By encapsulating data and behavior within objects, JavaScript developers can tackle complex applications with clarity and efficiency.
JavaScript stands out with its unique take on OOP, using prototypes—a feature distinct from the classical inheritance found in languages like Java or C++. This approach offers flexibility, but it also demands a keen understanding of JavaScript’s intricacies. This post will unravel these complexities, paving the way for mastery in JavaScript OOP.
Throughout this exploration, you’ll encounter concepts like prototypal inheritance, ES6 classes, and how they converge to offer powerful solutions for modern programming challenges. Stay tuned as we break down these concepts into digestible insights and practical examples.
Understanding the Evolution from Prototypes to ES6 Classes
Before ES6 introduced classes, JavaScript developers relied heavily on prototypes. Prototypes are the original mechanism for inheritance in JavaScript, allowing objects to inherit properties and methods from other objects. This setup, while powerful, often led to complexity and was less intuitive for those accustomed to classical OOP.
The advent of ES6 classes wasn’t about replacing prototypes but rather offering a more straightforward syntax for creating objects and handling inheritance. Classes in ES6 provide a clearer structure, making code more readable and maintainable, especially for developers transitioning from other OOP languages.
By understanding this evolution, you gain insight into JavaScript’s dual nature—embracing both traditional and modern practices. This duality empowers developers to choose the best tool for their specific needs, combining the strengths of prototypes and classes.
The Basics of Prototypal Inheritance in JavaScript
Prototypal inheritance allows objects to inherit directly from other objects. Unlike classical inheritance, which involves classes and instances, prototypal inheritance is more dynamic, enabling objects to share functionalities seamlessly.
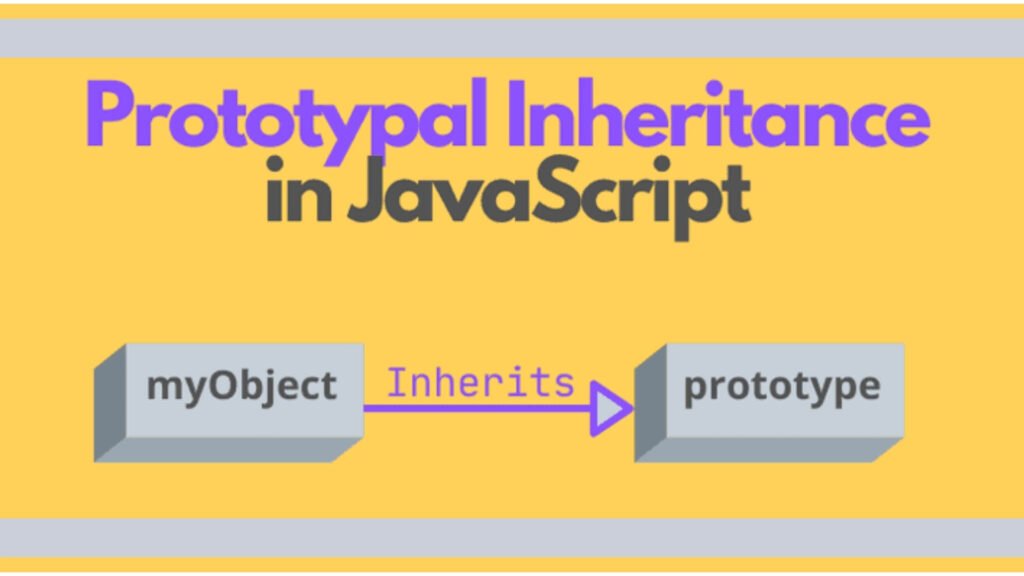
In JavaScript, every object has a hidden [[Prototype]] property, which can point to another object. This property forms the basis of inheritance, allowing properties and methods to be passed down the chain. The prototype chain continues until it reaches an object with a null prototype, usually `Object.prototype`.
Understanding prototypal inheritance is crucial for writing efficient JavaScript code. It enables powerful patterns like delegation, which can significantly optimize performance by having objects delegate requests to their prototypes.
How Prototypes Work Behind the Scenes in JavaScript
Under the hood, JavaScript’s prototype system operates via a series of linked objects. When a property or method is accessed, JavaScript first checks the object itself. If unavailable, it traverses up the prototype chain, searching each ancestor until the property is found or the chain ends.
This mechanism allows for shared behavior across multiple objects without duplicating code. For example, adding a method to an object’s prototype makes it available to all instances derived from that prototype, fostering reusability and consistency.
Developers can harness prototypes to extend native JavaScript objects. For instance, adding a custom method to `Array.prototype` means every array can utilize this new functionality, providing a unified approach to enhancing built-in types.
Creating Objects with ES6 Classes A Modern Approach
ES6 classes present a modern, syntactical sugar over JavaScript’s existing prototype-based inheritance. They simplify object creation and provide a clearer, more familiar structure for those from classical OOP backgrounds.
Using the `class` keyword, developers define templates for creating objects. These templates include a `constructor` method for initializing object properties and can contain other methods for shared behaviors. Here’s a simple example showcasing a `Car` class:
Example
class Car {
constructor(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
describe() {
return `${this.year} ${this.make} ${this.model}`;
}
}
const myCar = new Car(‘Toyota’, ‘Corolla’, 2020);
console.log(myCar.describe()); // Outputs: 2020 Toyota Corolla
This example illustrates how classes streamline object creation, encapsulating properties and methods within a cohesive structure. This readability and ease of use make ES6 classes a popular choice for modern JavaScript development.
The Role of the `constructor` Method in JavaScript Classes
The `constructor` method is a special function within a class that’s automatically called when a new instance is created. Its primary role is to initialize object properties and set up any necessary state.
In JavaScript, each class has a default constructor, but developers can define their own to specify custom initialization logic. The `constructor` is where parameters are passed during instantiation, allowing each object to be configured uniquely.

The importance of the `constructor` extends beyond initialization. It’s often used to establish connections with other objects or set up event listeners, playing a critical role in integrating new instances into broader application architectures.
Instance vs. Prototype Methods in JavaScript
JavaScript classes differentiate between instance and prototype methods. Instance methods are defined within the class body and become part of each object instance. Prototype methods, while also defined in the class, are shared among all instances.
This distinction is vital for optimizing performance. Prototype methods reduce memory consumption because they’re not duplicated across instances. Instead, they’re stored once on the prototype, accessible by all objects linked to that prototype.
By leveraging this mechanism, developers can create lean, efficient applications. Shared functionality is defined once, while instance-specific behavior is encapsulated within individual objects, maintaining a balance between performance and flexibility.
Extending Classes Inheritance in ES6
Inheritance allows developers to create new classes based on existing ones, facilitating code reuse and establishing hierarchies. In ES6, the `extends` keyword provides a clear, concise syntax for creating subclasses.
Subclasses inherit properties and methods from their parent class, while also adding or overriding features to tailor functionality. This pattern is prevalent in scenarios where multiple objects share common behaviors but require specialization.
Example
class SportsCar extends Car {
constructor(make, model, year, horsepower) {
super(make, model, year);
this.horsepower = horsepower;
}
calculatePowerToWeight(weight) {
return this.horsepower / weight;
}
}
const mySportsCar = new SportsCar(‘Ferrari’, ‘488’, 2019, 661);
console.log(mySportsCar.calculatePowerToWeight(1475)); // Outputs power-to-weight ratio
This example demonstrates how inheritance simplifies creating specialized classes. By extending `Car`, `SportsCar` retains core functionality while introducing new methods like `calculatePowerToWeight`.
Supercharging Inheritance with the `super()` Keyword
The `super()` keyword enhances inheritance by providing access to the parent class’s constructor and methods. It allows subclasses to call and utilize parent functionality, ensuring a cohesive integration of inherited and new features.
Within a subclass, `super()` must be called before accessing `this`, as it sets up the instance with properties from the parent class. It also facilitates calling methods from the parent, ensuring that overridden methods can still leverage original functionality.
The use of `super()` is especially advantageous in complex hierarchies or when implementing advanced behaviors. It offers the flexibility needed to build robust applications that efficiently combine inherited and new capabilities.
Understanding the `prototype` Property in JavaScript
The `prototype` property is central to JavaScript’s inheritance model. Each function in JavaScript has a `prototype` property, which is an object used as a blueprint for creating new instances.
When a function is used as a constructor, its `prototype` becomes the prototype of all instances created by that constructor. This linkage forms the basis of prototypal inheritance, enabling shared behavior across related objects.

Developers can augment this property by adding methods and properties, ensuring all objects created from the constructor have access to them. This mechanism underpins much of JavaScript’s flexibility and power in creating modular, reusable code.
Static Methods in ES6 Classes When and How to Use Them
Static methods are functions defined on a class itself, rather than instances of the class. They’re used for operations not tied to specific objects, often involving utility functions or processing data relevant to the class.
In ES6, static methods are defined using the `static` keyword. They can be invoked directly from the class, without instantiating an object, making them ideal for functionality that applies broadly to the class concept.
Example
class MathUtils {
static calculateDistance(x1, y1, x2, y2) {
return Math.sqrt((x2 – x1) ** 2 + (y2 – y1) ** 2);
}
}
console.log(MathUtils.calculateDistance(0, 0, 3, 4)); // Outputs: 5
“`
This example shows how static methods can encapsulate functionality applicable to all instances of a class. By keeping certain methods static, developers maintain a clean separation between instance-specific and class-wide logic.
Working with `this` in ES6 Classes and Prototypes
The `this` keyword is pivotal in JavaScript, referring to the object context in which a function is executed. Within ES6 classes, `this` points to the current instance, allowing developers to access and modify properties and methods.
Understanding `this` is crucial, as its value can vary depending on how and where a function is called. Arrow functions, for instance, inherit `this` from their enclosing lexical context, offering a predictable alternative to traditional function expressions.
In prototypes, `this` refers to the object on which the method is called. This consistency underpins JavaScript’s dynamic nature, enabling methods to operate on diverse objects while maintaining a unified interface.
How JavaScript Resolves Methods Using the Prototype Chain
JavaScript’s method resolution relies on the prototype chain. When a method is called, JavaScript searches the object for the method. If absent, it ascends the prototype chain, checking each ancestor until the method is found or the chain ends.
This resolution process allows for method overriding and polymorphism, where objects can redefine inherited methods to suit specific needs. It also facilitates mixin-like behavior, enabling objects to borrow and share methods dynamically.
Understanding this chain is vital for debugging, optimizing performance, and designing scalable applications. By leveraging this mechanism, developers can create flexible systems that adapt to changing requirements with minimal refactoring.
The Difference Between Classical Inheritance and Prototypal Inheritance
Classical inheritance, as seen in languages like Java, involves creating classes and instances with a fixed hierarchy. Prototypal inheritance, by contrast, is more flexible, allowing objects to inherit directly from other objects.
In JavaScript, prototypal inheritance offers dynamic prototypes that can be altered at runtime, fostering adaptability and efficiency. This flexibility supports patterns like mixins and composition, enabling developers to design robust, scalable solutions.
Understanding these differences helps developers choose the appropriate inheritance model for their needs. By combining the strengths of both systems, JavaScript offers unparalleled versatility in crafting sophisticated applications.
ES6 Classes vs. Constructor Functions Key Differences
Before ES6, JavaScript developers used constructor functions to create objects. ES6 classes formalized this process, providing a more intuitive syntax that resembles class-based languages.
While both approaches achieve similar outcomes, classes offer improved readability and structure. They encapsulate behavior within a cohesive unit, making code easier to understand and maintain, especially for developers transitioning from other languages.
Constructor functions remain relevant, particularly for developers seeking low-level control. By appreciating these differences, developers can select the approach that best aligns with their project’s requirements and goals.
Object Composition and Prototypal Inheritance in JavaScript
Object composition emphasizes combining objects with specific functionalities, rather than relying on inheritance. This pattern promotes flexibility, enabling developers to create more modular and reusable code.
JavaScript’s prototypal inheritance complements composition, allowing objects to share behavior while remaining independent. By composing objects with specific roles, developers can build systems that adapt to change with minimal effort.
Example
const canDrive = {
drive() {
console.log(‘Driving’);
}
};
const canFly = {
fly() {
console.log(‘Flying’);
}
};
const flyingCar = Object.assign({}, canDrive, canFly);
flyingCar.drive(); // Outputs: Driving
flyingCar.fly(); // Outputs: Flying
This example showcases how composition offers a flexible alternative to traditional inheritance. By assembling objects with distinct capabilities, developers maximize code reuse and adaptability.
Overriding and Extending Methods in ES6 Class Inheritance
ES6 class inheritance supports method overriding, enabling subclasses to redefine parent methods. This capability is essential for tailoring functionality to specific requirements while retaining inherited behavior.
Overriding allows developers to leverage the `super` keyword to access the parent method, integrating new features with existing logic. This approach ensures a seamless transition between inherited and customized behavior, fostering robust application design.
The ability to extend methods is crucial for scaling applications. By refining inherited methods, developers can optimize performance, enhance functionality, and ensure compatibility with evolving requirements.
Prototypes and Performance Optimizing Object-Oriented JavaScript
Prototypes offer performance advantages in JavaScript by enabling code reuse without duplication. By defining methods on a prototype, developers ensure all instances share the same reference, reducing memory consumption.
JavaScript engines optimize prototype lookups, making method access efficient even in extensive prototype chains. This optimization supports scalable applications that maintain high performance despite complex inheritance structures.
To maximize performance, developers should avoid modifying prototypes at runtime, as this can hinder engine optimizations. By adhering to best practices, developers can harness prototypes to build fast, efficient applications.
Private and Protected Members in JavaScript ES6 Classes
While JavaScript lacks native support for private and protected members, ES6 classes offer workarounds. Developers can use closures or Symbols to encapsulate data, simulating private properties.
Closures provide a straightforward method for securing object state. By defining variables within the constructor and returning methods that access them, developers achieve data encapsulation without exposing internals.
Symbols offer another approach, creating unique property keys that remain inaccessible to external code. This technique, while less common, provides enhanced security for sensitive data, safeguarding critical application components.
Encapsulation in JavaScript Using Closures and Symbols
Encapsulation is a core tenet of OOP, shielding object state from external interference. In JavaScript, closures and Symbols offer effective means of achieving encapsulation, promoting secure, maintainable code.
Closures enable developers to define private variables within functions, limiting access to specific methods. This approach ensures data integrity while providing controlled interfaces for interaction.
Symbols, as unique, immutable identifiers, offer an alternative means of encapsulation. By associating properties with Symbols, developers prevent accidental modification, preserving object state amidst complex interactions.
Mixins in JavaScript Extending Functionality Without Inheritance
Mixins are a pattern for extending object functionality without relying on inheritance. By combining objects with shared behavior, developers create flexible, scalable solutions that adapt to diverse requirements.
In JavaScript, mixins are implemented by copying methods from one object to another. This approach enables developers to compose objects with specific capabilities, fostering modular, reusable code.
Example
const mixin = (target, …sources) => Object.assign(target, …sources);
const person = {
speak() {
console.log(‘Hello!’);
}
};
const musician = {
play() {
console.log(‘Playing music’);
}
};
const singingMusician = {};
mixin(singingMusician, person, musician);
singingMusician.speak(); // Outputs: Hello!
singingMusician.play(); // Outputs: Playing music
This example illustrates how mixins empower developers to extend functionality dynamically, creating robust systems that accommodate evolving needs.
How Prototypes Enable Polymorphism in JavaScript
Polymorphism is a hallmark of OOP, enabling objects to present a unified interface despite differing implementations. In JavaScript, prototypes facilitate polymorphism by allowing objects to share methods that adapt to specific contexts.
Through the prototype chain, JavaScript objects can override inherited methods, tailoring behavior to individual requirements. This capability supports dynamic systems where objects can operate interchangeably, enhancing flexibility and scalability.
By leveraging polymorphism, developers create adaptable applications that respond to changing requirements with minimal refactoring. This approach fosters resilience, ensuring systems can evolve alongside user needs.
Creating Reusable Components with ES6 Classes
ES6 classes provide a framework for creating reusable components, promoting modular, maintainable code. By encapsulating behavior within classes, developers can define clear interfaces that facilitate integration and extension.
Reusable components streamline development, enabling rapid iteration and reducing duplication. By designing components with well-defined APIs, developers ensure consistent functionality across applications, enhancing efficiency and reliability.
The use of classes supports composability, allowing developers to assemble complex systems from simple, interchangeable parts. This approach fosters innovation, empowering developers to build sophisticated applications that adapt to diverse requirements.
Understanding the Difference Between `Object.create()` and ES6 Classes
`Object.create()` and ES6 classes represent distinct approaches to object creation in JavaScript. While both serve similar purposes, they offer different advantages and trade-offs.
`Object.create()` enables direct manipulation of prototypes, providing low-level control over inheritance and object composition. This method is ideal for scenarios requiring fine-grained customization, offering flexibility beyond traditional class-based patterns.
ES6 classes, by contrast, offer a structured, intuitive syntax for creating objects and handling inheritance. They encapsulate behavior within cohesive units, promoting readability and maintainability, especially for developers transitioning from other OOP languages.
Understanding these differences helps developers choose the approach that best aligns with their project’s goals and constraints. By leveraging both techniques, developers maximize their ability to create efficient, scalable applications.
Future Trends The Role of ES6 Classes and Prototypes in Modern JavaScript Development
The future of JavaScript development lies in the harmonious integration of ES6 classes and prototypes. These constructs, while distinct, complement each other, offering developers a versatile toolkit for building sophisticated applications.
As JavaScript evolves, new features and enhancements will continue to expand the possibilities for classes and prototypes. Developers who master these concepts position themselves to capitalize on emerging trends, driving innovation and efficiency.
The key to success lies in understanding when to apply each technique. By balancing the strengths of classes and prototypes, developers create resilient systems that adapt to changing requirements and deliver exceptional user experiences.
In conclusion, mastering OOP in JavaScript through ES6 classes and prototypes equips developers with the skills needed to excel in modern web development. By understanding these concepts, developers can create efficient, scalable applications that meet the demands of today’s dynamic digital landscape. Ready to take your JavaScript skills to the next level? Explore more resources and start experimenting with classes and prototypes today!
Frequently Asked Questions
- What is the advantage of using Symbols for encapsulation in JavaScript?
- Symbols in JavaScript provide a means to create unique property keys, ensuring privacy and preventing accidental interference by other code components.
- How do mixins differ from traditional inheritance in JavaScript?
- Mixins allow the combination of multiple objects to add functionality, whereas traditional inheritance involves a hierarchy where child classes inherit from a parent class.
- Can you provide an example of polymorphism in JavaScript?
- Polymorphism in JavaScript could be illustrated through different objects that inherit a base method from their prototype but implement it to perform varied tasks specific to their context.
- Why are ES6 classes beneficial for creating reusable components?
- ES6 classes offer a clear and organized syntax for defining object blueprints, making it easier for developers to create modular and maintainable code.
- What are some key differences between `Object.create()` and ES6 classes?
- `Object.create()` allows precise prototype manipulation, ideal for fine-tuned customization, whereas ES6 classes provide a structured approach to creating objects and handling inheritance.
- How can mastering prototypes and ES6 classes enhance my JavaScript development skills?
- Mastering these concepts allows for the development of efficient and scalable applications, adapting to evolving project requirements and improving code maintainability.
- What is a potential downside of using mixins in JavaScript?
- Mixins may lead to conflicts and difficulties in tracking changes if they are overused or poorly managed, as multiple objects may inject similar methods or properties into a target object.
- How do prototypes enable dynamic behavior in JavaScript applications?
- Prototypes allow objects to share and override methods, enabling them to adapt behavior dynamically at runtime to suit specific situations.
- In what scenarios might `Object.create()` be preferable over classes?
- When needing granular control over an object’s prototype chain or in low-level cases where performance and memory footprint are critical concerns.
- What should developers consider when deciding between using prototypes and ES6 classes in a project?
- Developers should consider the complexity of the application, team familiarity with OOP paradigms, and project requirements to determine whether the flexibility of prototypes or the structure provided by ES6 classes is more suitable.