In the dynamic world of web development, mastering JS JavaScript’s asynchronous programming is a game-changer. It can enhance the efficiency and responsiveness of your web applications, making them more robust and user-friendly. This article delves deeply into the principles of async/await, promises, and callbacks in JS JavaScript asynchronous programming.
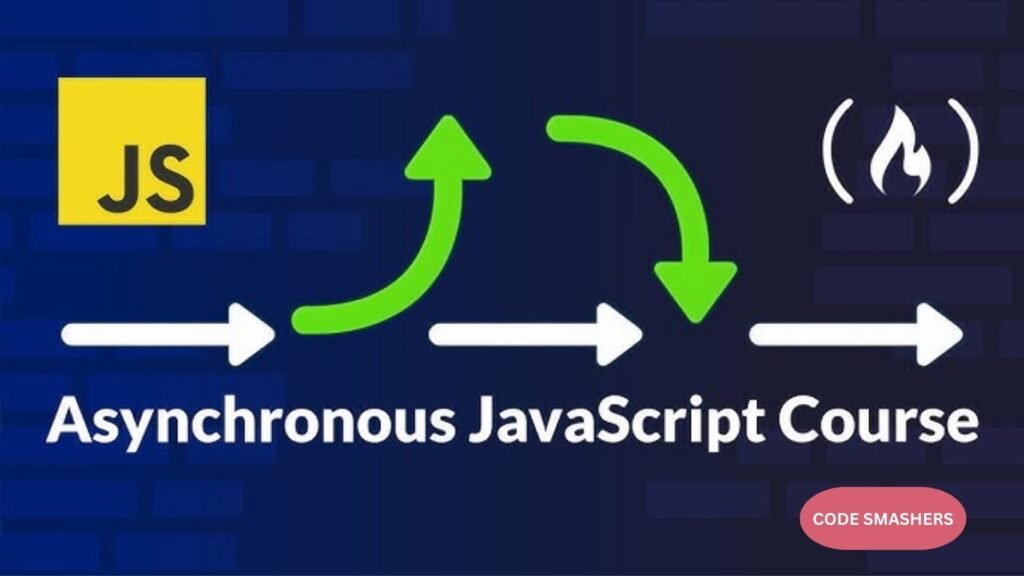
Introduction to Asynchronous Programming in JS JavaScript
What Is Asynchronous Programming?
At its core, asynchronous programming allows multiple tasks to run concurrently. Instead of waiting for one task to complete before starting another, asynchronous programming lets JS JavaScript initiate other operations in parallel. This approach is particularly useful in web development, where tasks like fetching data from an API or reading a file can take time.
Why Is It Important?
Websites that lag or freeze during data processing can frustrate users and drive them away. By leveraging asynchronous programming, developers can ensure that their applications remain responsive and efficient, even when performing resource-intensive tasks.
Understanding Callbacks
Definition and Syntax
A callback is a function that is supplied to another function as an argument. This allows the callback function to be executed after the completion of the initial function.
Example:
Here’s a basic callback function that logs “Hello, World!” after 2 seconds:
JS JavaScript
function greet(callback) {
setTimeout(() => {
callback(“Hello, World!”);
}, 2000);
}
function logGreeting(greeting) {
console.log(greeting);
}
greet(logGreeting);
Common Use Cases and Examples
Callbacks are ubiquitous in JS JavaScript, from handling events to managing asynchronous operations like reading files or making API requests. They provide a straightforward way to handle functions that need to execute after a certain task is completed.
Callback Hell: Challenges and Solutions
What Is Callback Hell?
Callback hell occurs when callbacks are nested within other callbacks, leading to code that is difficult to read and maintain. This can make debugging and adding new features a nightmare.
Techniques to Manage Callback Hell
To manage callback hell, developers can:
- Modularize functions to break down complex operations into smaller, more manageable chunks.
- Use promises to avoid deep nesting.
- Leverage Async/Await for cleaner and more readable asynchronous code.
Here’s an example of nested callbacks with file operations:
JS JavaScript
readFile(‘file1.txt’, function (err, data1) {
if (err) throw err;
readFile(‘file2.txt’, function (err, data2) {
if (err) throw err;
combineFiles(data1, data2, function (err, result) {
if (err) throw err;
writeFile(‘output.txt’, result, function (err) {
if (err) throw err;
console.log(‘Files combined and written to output.txt’);
});
});
});
});
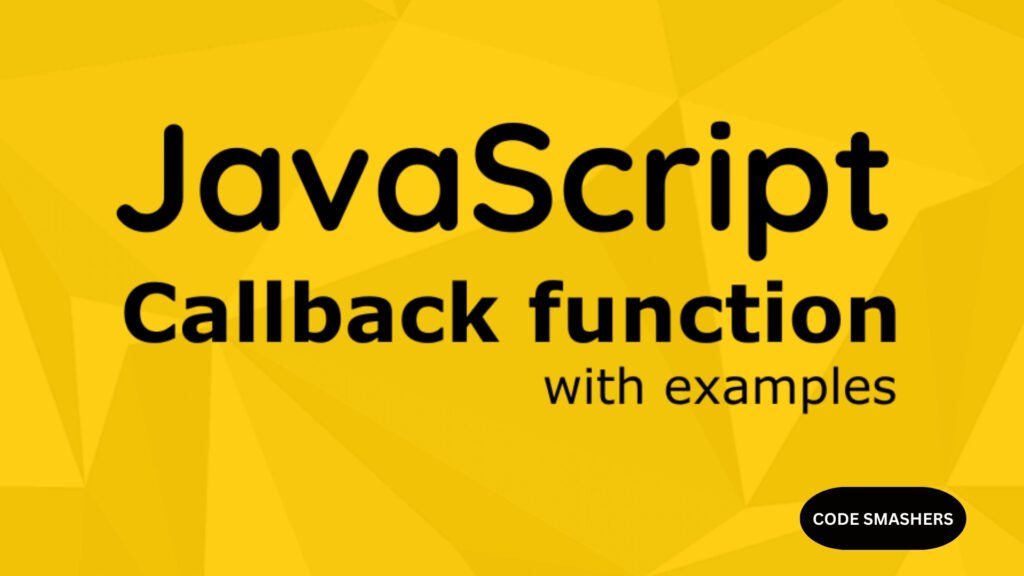
Introduction to Promises
What Are Promises?
An easier and more natural approach to manage asynchronous processes is through promises. A value represented by a promise might be available right now, later on, or never at all. It has three states:
- Pending: In the beginning, neither accepted nor rejected.
- Fulfilled: Operation completed successfully.
- Rejected: Operation failed.
Basic Promise Syntax and States
Creating a simple promise looks like this:
JS JavaScript
let promise = new Promise((resolve, reject) => {
setTimeout(() => resolve(“Done!”), 2000);
});
promise.then(alert);
Creating and Using Promises
`new Promise` Constructor
Use the `new Promise` constructor, which accepts a function and two parameters, `resolve` and `reject}, to create a promise.
`.then()`, `.catch()`, and `.finally()` Methods
- `.then()` handles the successful resolution of a promise.
- `.catch()` handles errors or rejections.
- `.finally()` executes once the promise is settled, regardless of its outcome.
JS JavaScript
function wait(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}managing
wait(2000).then(() => console.log(‘2 seconds passed’));
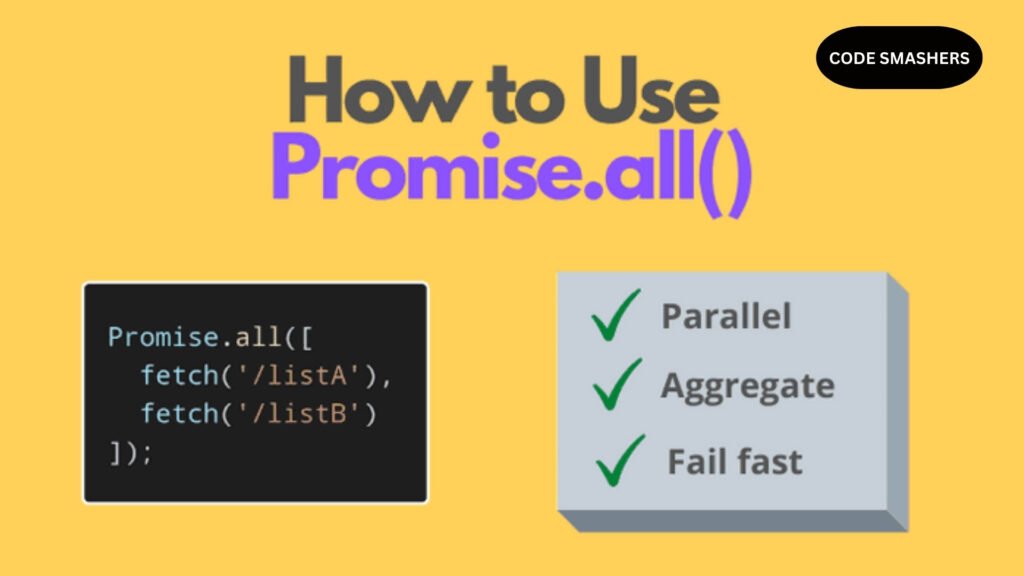
Handling Errors with Promises
Error Handling in `.catch()`
Promise errors can be handled with the `.catch()} method:
JS JavaScript
new Promise((resolve, reject) => {
setTimeout(() => reject(new Error(“Failed!”)), 2000);
}).catch(alert);
Chaining Promises and Error Propagation
allowChaining promises allows sequential asynchronous operations. Errors propagate down the chain until caught.
Promise.all and Promise.race
Using `Promise.all` for Concurrent Operations
When all of the promises in the iterable have resolved, `Promise.all` permits multiple promises to run concurrently and resolves:
JS JavaScript
let urls = [
‘https://api.github.com/users/username1’,
‘https://api.github.com/users/username2’
];
let requests = urls.map(url => fetch(url));
Promise.all(requests)
.then(responses => responses.forEach(
response => console.log(`${response.url}: ${response.status}`)
));
How `Promise.race` Works
`Promise.race` returns the first promise that settles, whether it resolves or rejects.
Introduction to Async/Await
What are `async` and `await`?
`async` and `await` simplify working with promises. The `async` keyword makes a function return a promise, and `await` pauses the execution until the promise settles.
Syntax and Basic Usage
Here’s an example of an asynchronous function using `async` and `await`:
JS JavaScript
async function fetchData() {
let response = await fetch(‘https://api.github.com/users/username’);
let data = await response.json();
console.log(data);
}
Error Handling with Async/Await
Using `try/catch` with `await`
Async function errors can be handled with `try/catch}:
JS JavaScript
async function fetchData() {
try {
let response = await fetch(‘https://api.github.com/users/username’);
let data = await response.json();
console.log(data);
} catch (error) {
console.error(‘Error:’, error);
}
}
Handling Multiple Errors in Async Functions
For handling multiple errors, multiple `try/catch` blocks can be nested or chained.
Comparing Callbacks, Promises, and Async/Await
Key Differences and Use Cases
- Callbacks are easy, but they can result in callback hell.
- Promises offer a structured way to handle async operations without deep nesting.
- Async/Await provides a synchronous-like code structure for better readability.
When to Use Each Approach
- For straightforward, one-time asynchronous actions, utilize callbacks.
- Use promises for more complex sequences of async operations.
- Use async/await for most modern JS JavaScript projects to ensure clean and readable code.
Event Loop and the Call Stack
Understanding the Event Loop Mechanism
The event loop is how JS JavaScript handles asynchronous operations, allowing non-blocking execution despite being single-threaded.
How the Call Stack Affects Asynchronous Code
The call stack manages function execution, while the event loop processes asynchronous callbacks once the stack is clear.
Microtasks and Macrotasks
What Are Microtasks and Macrotasks?
- Microtasks include promises and process.nextTick.
- Macrotasks consist of I/O activities, setTimeout, and setInterval.
How They Affect Asynchronous Execution
Microtasks have a higher priority and are executed before macrotasks, affecting how asynchronous code runs.
Handling Asynchronous Operations in Loops
Using Async Functions in Loops
To handle async operations in loops, use `await` inside a loop to ensure proper sequencing.
Managing Sequential and Concurrent Execution
Combine async functions and `Promise.all` for efficient loop operations.
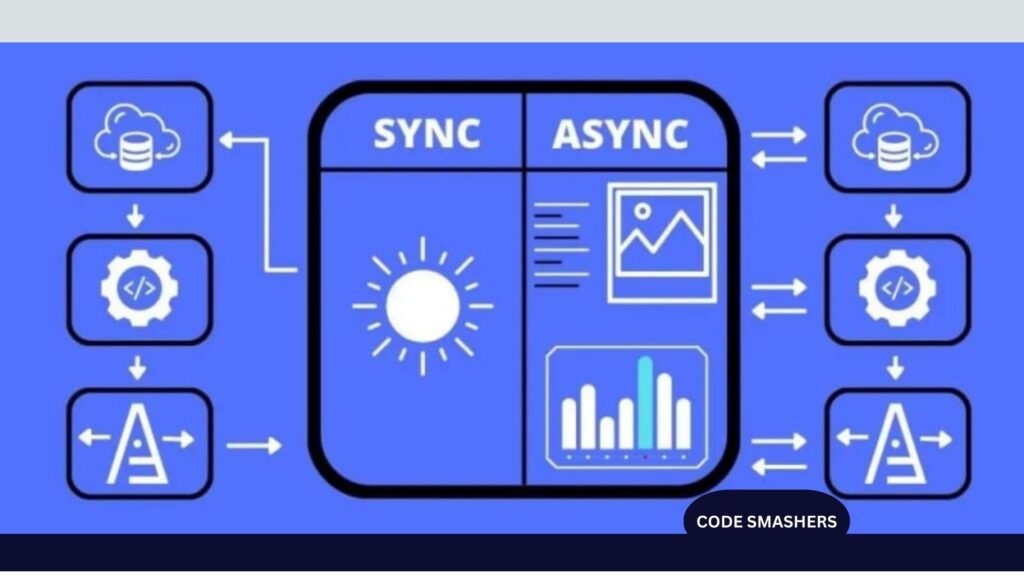
Interacting with APIs Asynchronously
Making API Requests with Fetch
Fetch API simplifies making HTTP requests in JS JavaScript:
JS JavaScript
fetch(‘https://api.github.com/users/username’)
then(response => response.json())
then(data => console.log(data));
Handling Responses with Promises and Async/Await
Use `.then()` for promises and `await` for async functions to handle API responses.
Testing Asynchronous Code
Techniques for Testing Callbacks and Promises
Use testing frameworks like Mocha or Jest to test async code, ensuring it handles all cases correctly.
Using Testing Libraries with Async/Await
Testing libraries support async/await, making it easier to write and run tests for asynchronous code.
Asynchronous Iteration with `for await…of`
Understanding `for await…of`
The `for await…of` loop is a powerful feature in JS JavaScript that allows developers to iterate over asynchronous data sources. This syntax is particularly useful when dealing with streams of data, where each element is retrieved one by one over time, such as reading a file line-by-line or processing multiple HTTP requests.
How It Works
When using `for await…of`, the loop waits for a promise to resolve before moving to the next iteration, making it an elegant solution for sequential asynchronous processing. It simplifies the consumption of asynchronous data by combining promises and iterables into a single construct, reducing the need for nested promises or callbacks within loop structures.
Example Use Cases
One common use case of `for await…of` is iterating over a list of file names and processing each file’s contents as they are read:
JS JavaScript
const fs = require(‘fs/promises’);
async function processFiles(filePaths) {
for await (const filePath of filePaths) {
const fileContent = await fs.readFile(filePath, ‘utf-8’);
console.log(fileContent);
}
}
processFiles([‘file1.txt’, ‘file2.txt’, ‘file3.txt’]);
In this example, `for await…of` iterates over `filePaths`, waiting for each `fs.readFile` promise to resolve before proceeding. This ensures that each file’s content is processed in sequence, handling potential asynchronous outcomes efficiently.
Benefits and Considerations
The `for await…of` loop combines the benefits of `for…of` and async functions, enhancing code readability and maintainability. However, it is important to note that `for await…of` cannot be used with non-iterable objects or directly with synchronous collections. Its usage is best suited for handling cases where async iteration is necessary, offering a structured way to deal with asynchronous streams in JS JavaScript.
Impact on Performance
While asynchronous programming patterns like Promises and `async/await` can dramatically improve the responsiveness of applications, they also have implications for performance that developers must consider. By allowing non-blocking execution, asynchronous functions enable JS JavaScript to handle multiple tasks concurrently without freezing the user interface.
However, the efficient handling of tasks requires careful management of both microtasks and macrotasks to prevent performance bottlenecks like excessive task queuing or race conditions. Furthermore, improper use of asynchronous iterations, such as over-reliance on `await` within loops, can introduce unnecessary delays if each iteration waits for the previous one to complete. Balancing between sequential and concurrent execution is crucial.
Techniques such as `Promise.all` can be leveraged for optimizing performance by executing independent asynchronous operations concurrently, thus reducing the total execution time and improving application throughput. Thoughtful design and testing are essential to harnessing the full potential of asynchronous features while maintaining optimal performance.
Best Practices for Asynchronous Programming
Writing Clean and Maintainable Async Code
- Keep functions modular.
- Avoid deep nesting.
- Use meaningful names.
Avoiding Common Pitfalls
- Handle errors properly.
- Use async/await for better readability.
- Test thoroughly.
Debugging Asynchronous Code
Tools and Techniques for Debugging
Use tools like Chrome DevTools or Node.js debugger for step-by-step debugging of async code.
Common Issues and Solutions
- Uncaught Errors: Use `try/catch` and `.catch()`.
- Callback Hell: Refactor using async/await or promises.
Real-World Examples of Async Programming
Practical Use Cases and Patterns
- Fetching Data: Use async/await for API requests.
- File Operations: Use promises for reading/writing files.
Case Studies of Async Code in Action
Explore case studies of successful async programming implementations in various projects.
Future Trends in Asynchronous JS JavaScript
Emerging Features and Proposals
Stay updated with new features like top-level await and improvements in the event loop mechanism.
How Asynchronous Programming Is Evolving
The future of async programming promises more intuitive and efficient ways to handle concurrent operations in JS JavaScript.
Conclusion
Mastering JS JavaScript’s asynchronous programming is not just a technical skill but a gateway to creating more efficient, responsive, and user-friendly web applications. By understanding and implementing callbacks, promises, and async/await, you can significantly enhance your coding prowess and deliver superior user experiences.
Ready to take your JS JavaScript skills to the upgrade level? Start experimenting with these techniques today and see the difference they make in your projects. Happy coding!