In the world of software development, mastering Object-Oriented Programming (OOP) principles is akin to unlocking the secrets of the universe. Okay, maybe not the entire universe, but definitely the universe of creating efficient, scalable, and maintainable software. From encapsulation to polymorphism, these principles form the backbone of modern programming. Today, we’re taking a deep dive into these fascinating concepts, demystifying them with clear examples and practical insights.
Whether you’re a seasoned software developer, a computer science student, or a tech enthusiast eager to expand your knowledge, this comprehensive guide is designed to offer you valuable insights into OOP. Ready to elevate your coding prowess? Let’s jump right in!
Introduction to Object-Oriented Programming (OOP)
Definition and Importance of OOP
Object-Oriented Programming is a paradigm that centers around objects rather than actions. It’s a way to model real-world scenarios and entities in programming. By focusing on objects, developers can create code that is organized, reusable, and easier to conceptualize.
History and Evolution of OOP
OOP has evolved significantly since its inception in the 1960s. Initially developed to tackle the shortcomings of procedural programming, it brought a revolutionary approach to software design. Languages like Simula and Smalltalk laid the groundwork, with giants like Java and C++ cementing its role in the industry. Understanding this evolution helps appreciate the sophistication of modern programming languages.
Core Principles of OOP
Overview of OOP Principles
At the heart of OOP are four core principles—Encapsulation, Abstraction, Inheritance, and Polymorphism. These principles guide the design and implementation of software, making it modular and adaptable to change. Each principle contributes unique advantages, which we’ll explore in detail.
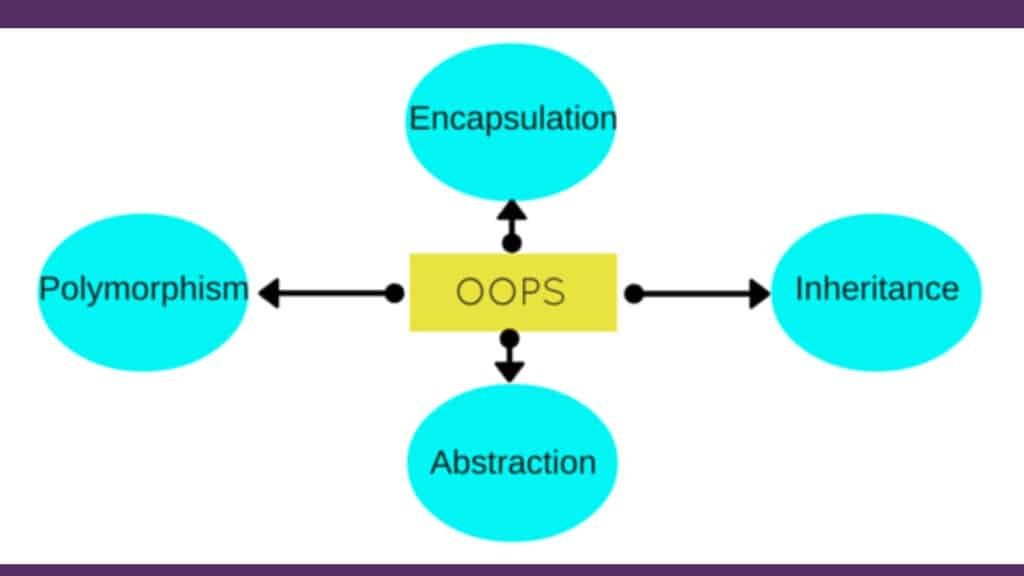
How OOP Differs from Procedural Programming
While procedural programming focuses on functions or procedures, OOP emphasizes data encapsulation through objects. This shift allows for a more natural mapping of real-world problems and offers increased flexibility and scalability in software development.
Encapsulation
What is Encapsulation?
Encapsulation is the process of bundling data and methods that operate on the data within one unit, or object, and restricting access to some components. This design approach keeps the data safe from outside interference and misuse, promoting data integrity and security.
Benefits of Encapsulation
Encapsulation offers several advantages, including enhanced data protection, reduced complexity, and improved code maintenance. By controlling access to data, developers can ensure consistency and reliability in their applications.
Code Example: Implementing Encapsulation
Here’s how encapsulation works in practice:
javascript
class BankAccount {
constructor(balance) {
this._balance = balance; // Private variable
}
deposit(amount) {
this._balance += amount;
}
getBalance() {
return this._balance;
}
}
const account = new BankAccount(1000);
account.deposit(500);
console.log(account.getBalance()); // 1500
The BankAccount class encapsulates the balance and controls access through its methods, demonstrating how encapsulation protects data.
Abstraction
What is Abstraction?
Abstraction focuses on hiding the complex reality while exposing only the necessary parts. It simplifies interactions by providing a simplified interface to complex systems, allowing users to operate without understanding the intricate details.
Benefits of Abstraction
By reducing complexity, abstraction enhances code readability and reusability. It shields users from the complexities beneath the surface, enabling them to focus on high-level interactions with the system.
Code Example: Implementing Abstraction
Consider this example:
javascript
class Shape {
area() {
throw new Error(‘Area method must be implemented’);
}
}
class Circle extends Shape {
constructor(radius) {
super();
this.radius = radius;
}
area() {
return Math.PI * this.radius * this.radius;
}
}
const circle = new Circle(5);
console.log(circle.area()); // 78.54
Here, the Shape class abstracts the concept of a geometric shape, allowing derived classes like Circle to implement their specific logic.
Inheritance
What is Inheritance?
Inheritance is an OOP principle where one class can inherit fields and methods from another, promoting code reusability. This hierarchy allows for the extension and modification of existing code without altering it directly.

Types of Inheritance
Inheritance can be single, multiple, multilevel, or hierarchical. Each type serves different purposes, ranging from simple extensions to complex hierarchies. Understanding these types is crucial for effective software design.
Code Example: Implementing Inheritance
Let’s look at a simple inheritance example:
javascript
class Animal {
speak() {
console.log(‘Animal speaks’);
}
}
class Dog extends Animal {
speak() {
console.log(‘Woof! Woof!’);
}
}
const dog = new Dog();
dog.speak(); // Woof! Woof!
In this scenario, Dog inherits from Animal and overrides its speak method, showcasing how inheritance supports specialization.
Polymorphism
What is Polymorphism?
Polymorphism allows methods to do different things based on the object they are acting upon. It enhances flexibility and integration by enabling a single function to process objects in different ways.
Types of Polymorphism
Polymorphism can be compile-time (method overloading) or runtime (method overriding). These types allow developers to create versatile and robust systems that handle various scenarios effortlessly.
Code Example: Implementing Polymorphism
Here’s a demonstration of polymorphism:
javascript
class Cat extends Animal {
speak() {
console.log(‘Meow! Meow!’);
}
}
function makeSound(animal) {
animal.speak();
}
makeSound(new Dog()); // Woof! Woof!
makeSound(new Cat()); // Meow! Meow!
This example illustrates how a function can work with different object types, highlighting the essence of polymorphism.
Benefits of Encapsulation
Data Protection and Security
Encapsulation ensures that data is accessed in a controlled manner, enhancing security by preventing unauthorized access and modifications. It acts as a protective shield for sensitive information.
Reducing Complexity
By hiding complex implementations, encapsulation reduces the perceived complexity of a system, making it easier for developers to understand and maintain code.
Real-World Examples of Encapsulation
Encapsulation is prevalent in banking systems, where sensitive information like account balances and transactions are protected. User interfaces also utilize encapsulation to manage component interactions without exposing internal processes.
Benefits of Abstraction
Simplifying Complex Systems
Abstraction simplifies complex systems by breaking them down into manageable units. This simplification aids in understanding and managing large-scale applications.
Enhancing Code Readability
By focusing on essential interactions, abstraction enhances code readability, allowing developers to grasp functionality without getting bogged down in details.
Real-World Examples of Abstraction
Database management systems use abstraction to hide complex query processes from users. User interface components also leverage abstraction, presenting a simple interface for complex functionality.
Benefits of Inheritance
Code Reusability
Inheritance promotes code reusability by allowing existing code to be extended and modified without direct changes. This efficiency reduces duplication and enhances maintainability.
Extensibility
Through inheritance, developers can enhance existing systems by adding new features and functionalities without disrupting existing codebases.
Real-World Examples of Inheritance
Game development frequently employs inheritance, where base classes like Character are expanded into player and enemy classes. Web development frameworks also utilize inheritance to extend functionality across components.
Benefits of Polymorphism
Flexibility and Maintainability
Polymorphism increases flexibility by allowing methods to adapt to different object types. This flexibility enhances maintainability, allowing systems to evolve without extensive modifications.
Improved Code Organization
With polymorphism, code is organized around behaviors rather than specific object types, enabling cleaner and more intuitive designs.
Real-World Examples of Polymorphism
Event handling in graphical user interfaces often employs polymorphism, allowing the same event listener to handle different event types. API design also benefits from polymorphism, enabling APIs to offer consistent interfaces across various implementations.
Challenges with Encapsulation
Balancing Access Levels
While encapsulation provides data protection, balancing access levels can be challenging. Striking the right balance between exposing and hiding data is crucial for effective encapsulation.
Managing Complexity in Large Systems
In large systems, encapsulation can introduce complexity by requiring careful management of data access and interactions. Over-relying on encapsulation can lead to intricate dependencies.
Challenges with Abstraction
Finding the Right Level of Abstraction
Determining the appropriate level of abstraction can be tricky. Over-abstraction may lead to unnecessary complexity, while under-abstraction can expose too much detail.
Over-Abstracting and Creating Unnecessary Complexity
Over-abstracting can result in convoluted designs that obscure functionality, making it difficult for developers to comprehend and manage codebases.
Challenges with Inheritance
Issues with Multiple Inheritance
Multiple inheritance can lead to conflicts and ambiguity in class hierarchies. The diamond problem, where a class inherits from two classes that share a common ancestor, is a notable challenge.
Challenges with Polymorphism
Type Safety Concerns
Polymorphism introduces type safety concerns, where incorrect assumptions about object types can lead to runtime errors. Careful design and testing are essential to mitigate these risks.

Performance Implications
Polymorphism can impact performance, especially in resource-intensive applications. The overhead of dynamic method resolution requires optimization strategies to maintain efficiency.
Best Practices in OOP
Designing with OOP Principles
Employ OOP principles thoughtfully, aligning design decisions with project requirements and goals. Prioritize code clarity, maintainability, and flexibility to create robust solutions.
Code Readability and Maintainability
Maintain code readability by adhering to naming conventions, using meaningful identifiers, and organizing code logically. Clean and concise code enhances maintainability and collaboration.
Key Takeaway Points
- Polymorphism’s Role in Flexibility: Allows for adaptable systems where methods can work with various object types, enhancing maintainability and organization.
- Encapsulation for Security: Acts as a protective layer for data, promoting secure and organized access while reducing complexity in systems.
- Abstraction’s Simplifying Power: Breaks down complex systems into understandable elements, improving code readability and system management.
- Inheritance to Maximize Reusability: Facilitates code extension and modification, promoting efficient development without redundancy.
- Challenges in OOP: Balancing encapsulation, managing abstraction, handling inheritance conflicts, and addressing polymorphism’s type safety ensure robust object-oriented design.
- Best Practices: Emphasizes thoughtful application of OOP principles, maintaining code readability, and aligning design with project objectives.
Conclusion
In conclusion, Object-Oriented Programming principles—Encapsulation, Abstraction, Inheritance, and Polymorphism—are essential tools for modern software development. Each principle offers unique advantages that enhance code quality, flexibility, and maintainability. By understanding and applying these principles, developers can create efficient and scalable software solutions that meet the demands of today’s dynamic technological landscape.
For those eager to explore further, consider experimenting with these principles in your projects or joining online communities to exchange ideas and insights. The world of OOP is vast and exciting, and there is always more to learn and discover.
Frequently Asked Questions
- What are the four main principles of Object-Oriented Programming (OOP)?
- The four main principles of OOP are:
- Encapsulation: Bundling data (variables) and methods (functions) that operate on the data into a single unit called a class.
- Inheritance: A mechanism to create a new class using an existing class, inheriting its properties and behaviors.
- Polymorphism: The ability to use a single interface to represent different types or classes, allowing methods to behave differently based on the object that invokes them.
- Abstraction: Hiding complex implementation details and exposing only the necessary parts of an object.
- The four main principles of OOP are:
- How does encapsulation improve software development?
- Encapsulation improves software development by making code more maintainable and secure. It ensures that an object’s internal state can only be modified through well-defined interfaces, protecting data integrity and reducing the risk of unintended side effects. It also improves modularity by separating the object’s functionality from the external code.
- What is inheritance, and why is it important in OOP?
- Inheritance allows a new class (child class) to inherit properties and methods from an existing class (parent class). It promotes code reuse, reduces redundancy, and helps create a hierarchical structure in software systems. This results in more efficient code management and easier maintenance.
- What is polymorphism in OOP, and how does it help in building flexible software?
- Polymorphism allows objects of different classes to be treated as objects of a common superclass, enabling the same method or operation to behave differently based on the object type. This flexibility helps in creating more scalable and reusable code.
- How does abstraction contribute to better software design?
- Abstraction simplifies complex systems by exposing only the essential features to the user, hiding implementation details. This leads to cleaner, easier-to-understand code and reduces the complexity for developers working with the system. It also makes the code more adaptable to change.
- Can you explain the difference between method overloading and method overriding in OOP?
- Method Overloading: Occurs when multiple methods with the same name exist in a class but differ in the number or type of their parameters.
- Method Overriding: Happens when a subclass provides a specific implementation for a method that is already defined in its superclass, allowing the subclass to modify the behavior of that method.
- Why is designing a class and object structure important in OOP?
- Proper class and object design is crucial because it defines the structure, behavior, and relationships of the entities within a system. A well-designed class hierarchy and object relationships make the software more modular, reusable, maintainable, and scalable, helping avoid unnecessary complexity.
- What role does data hiding play in ensuring software security and maintainability?
- Data hiding, implemented through encapsulation, restricts direct access to an object’s internal state and data. This ensures that the data can only be modified through controlled methods, enhancing security, preventing accidental data corruption, and making the software easier to maintain by providing clear interfaces for interaction.
- How do OOP principles help in code reuse and reduce redundancy?
- OOP promotes code reuse through inheritance (reusing base class functionality) and composition (building complex objects using simpler ones). This reduces redundancy by eliminating the need to write repetitive code and makes the software easier to maintain and extend.
- What is the significance of constructors and destructors in OOP?
- Constructors are special methods used to initialize objects when they are created, ensuring that an object starts in a valid state. Destructors are used to clean up resources, such as closing file handles or freeing memory, when an object is no longer needed, helping prevent resource leaks.
- What is the concept of “composition over inheritance” and how does it affect OOP design?
- “Composition over inheritance” refers to the design principle where objects are composed of other objects rather than inheriting from a parent class. This promotes flexibility and modularity, as composition allows for greater reuse and avoids the complications of deep inheritance hierarchies.
- What are the advantages of using interfaces in OOP?
- Interfaces define a contract for classes without specifying how the methods should be implemented. This promotes loose coupling between classes, enabling multiple classes to implement the same interface in different ways. It also enhances flexibility and makes it easier to change and extend the software system.
- How do design patterns relate to OOP principles, and can they help in achieving success in software development?
- Design patterns are general, reusable solutions to common problems that arise in software design. They often align with OOP principles (e.g., Factory Pattern, Observer Pattern, Strategy Pattern). By applying design patterns, developers can create more maintainable, flexible, and scalable systems that follow best practices.
- What is the SOLID principle, and how does it improve OOP design?
- SOLID is an acronym for five principles that improve object-oriented design:
- Single Responsibility Principle (SRP): A class should have only one reason to change.
- Open/Closed Principle (OCP): A class should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types without altering the correctness of the program.
- Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules, but both should depend on abstractions.
- These principles promote cleaner, more maintainable, and extensible code.
- SOLID is an acronym for five principles that improve object-oriented design:
- How do you know if an OOP design is successful or effective for a project?
- An effective OOP design is successful if it leads to code that is modular, flexible, reusable, and easy to maintain. Success can be measured by the ease of adding new features, the clarity of the codebase, the reduced likelihood of bugs, and the ability to adapt to changes in requirements or technology.