Unlocking the full potential of Python programming often lies in mastering the use of decorators. These handy tools can transform your code’s functionality with minimal effort, making them a must-know for developers at any level. Whether you are new to Python or a seasoned coder, understanding decorators can take your skills to the next level. In this blog post, we’ll explore what decorators are, how they work, and why they are invaluable for enhancing functions.
Introduction to Python Decorators
Python decorators might sound like an advanced topic, but they are incredibly useful once you understand their power. Decorators allow you to modify the behavior of a function or method without changing its actual code. This ability makes them a versatile tool in a developer’s toolkit.
The concept of decorators stems from the need to add functionality to existing code efficiently. Instead of rewriting functions, decorators provide a cleaner, more readable way to extend their capabilities. By the end of this post, you’ll have a solid grasp of how to implement and utilize Python decorators to seamlessly enhance your functions.
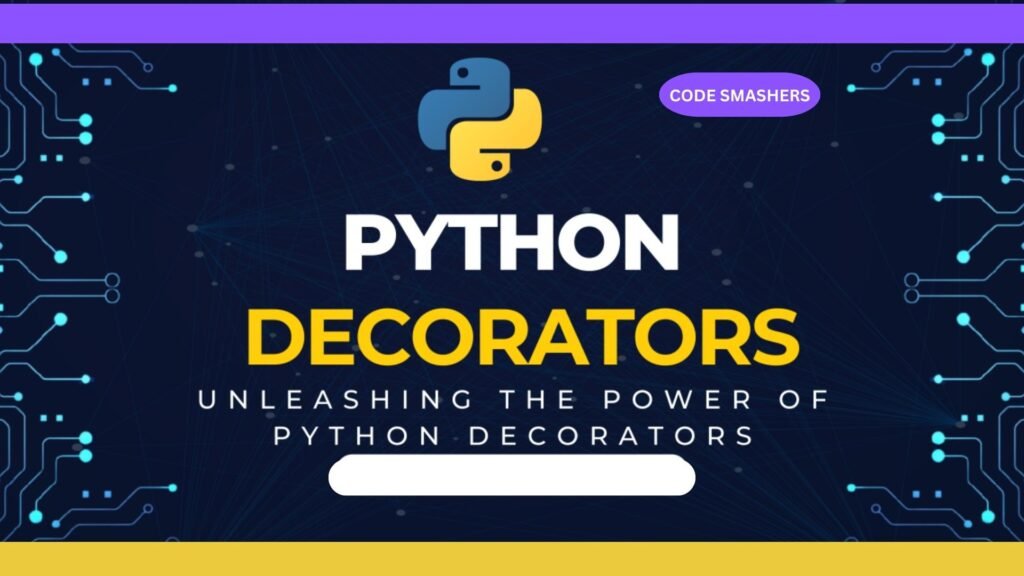
The Basics: Understanding Functions in Python
Before we dive into decorators, let’s recap some fundamentals about functions in Python. Functions are reusable programming segments that carry out particular tasks. They help organize your code into manageable sections, making it more readable and maintainable.
Functions can accept parameters, return values, and even be nested within other functions. Their versatility makes them a core feature of Python programming. Understanding these basics is crucial, as decorators work closely with functions to modify their behavior.
For example, let’s look at a basic function that outputs a message:
def greet():
print(“Hello, World!”)
This function performs a straightforward task, but what if we wanted to extend its functionality without altering its core logic? That’s where decorators come into play.
Exploring the Concept of Decorators
Decorators are higher-order functions that take another function as an argument and extend or alter its behavior. Think of them as wrappers that add extra functionality to an existing function. Modular programming aids in the maintenance of organized, tidy code.
The name of the decorator function is preceded by the {@} sign when defining decorators in Python. Here’s a basic example:
def my_decorator(func):
def wrapper():
print(“Something is happening before the function is called.”)
func()
print(“Something is happening after the function is called.”)
return wrapper
@my_decorator
def say_hello():
print(“Hello!”)
say_hello()
In this example, `my_decorator` wraps around `say_hello`, adding extra print statements before and after the original function’s execution. The `@my_decorator` syntax is a shortcut for applying the decorator to the function.
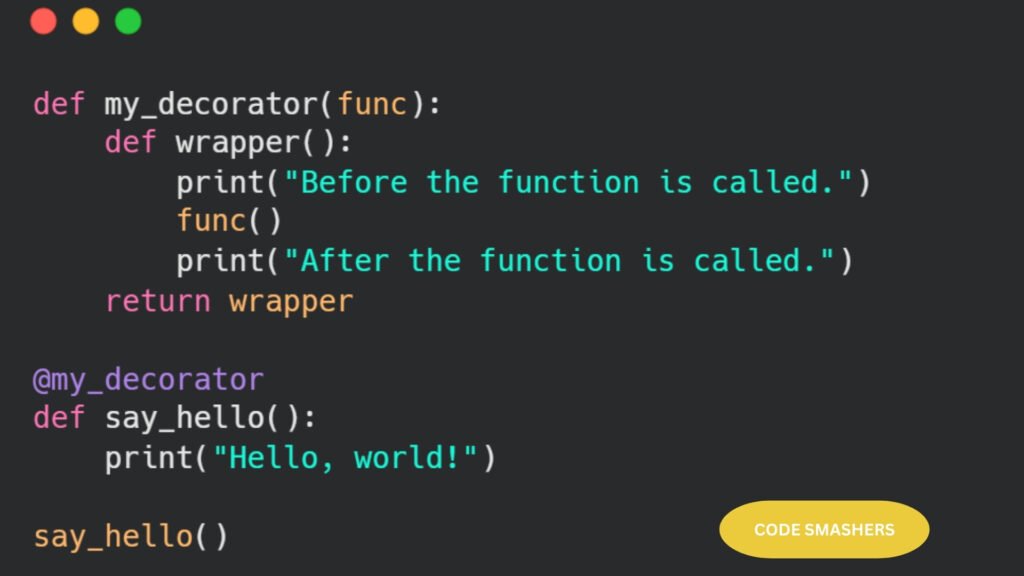
Implementing a Simple Decorator in Python
Creating your first decorator involves defining a function that returns another function. Let’s construct a basic decorator piece by piece. First, define the decorator function:
def simple_decorator(func):
def wrapper():
print(“Decorator is executing!”)
func()
print(“Decorator has finished.”)
return wrapper
Next, apply this decorator to a simple function:
@simple_decorator
def my_function():
print(“My function is running.”)
my_function()
When `my_function` is called, the decorator adds additional print statements, demonstrating its ability to modify the original function’s behavior without altering its code.
Enhancing Functions with Decorators: Real-world Examples
Decorators shine in real-world applications where repetitive tasks need to be automated or additional functionality is required. Consider a scenario where you need to log function calls for debugging purposes. A decorator can streamline this process:
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f”Function {func.name} is about to be called.”)
result = func(*args, **kwargs)
print(f”Function {func.name} has been called.”)
return result
return wrapper
@log_decorator
def add(a, b):
return a + b
print(add(5, 3))
In this example, `log_decorator` logs when `add` is called and executed, providing valuable debugging information without modifying the `add` function itself. This method maintains the cleanliness and maintainability of your codebase while also being efficient.
Advanced Decorator Techniques: Using Multiple Decorators
Decorators can be stacked to apply multiple layers of functionality to a single function. This technique is particularly insightful when you need to combine several modifications.
Consider the following example:
def uppercase_decorator(func):
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return result.upper()
return wrapper
def exclaim_decorator(func):
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return result + “!”
return wrapper
@uppercase_decorator
@exclaim_decorator
def greet(name):
return f”Hello, {name}”
print(greet(“Alice”))
Here, `greet` is first modified by `exclaim_decorator` to add an exclamation mark, then modified by `uppercase_decorator` to convert the message to uppercase. The order of stacking matters, as each decorator applies its changes in sequence.
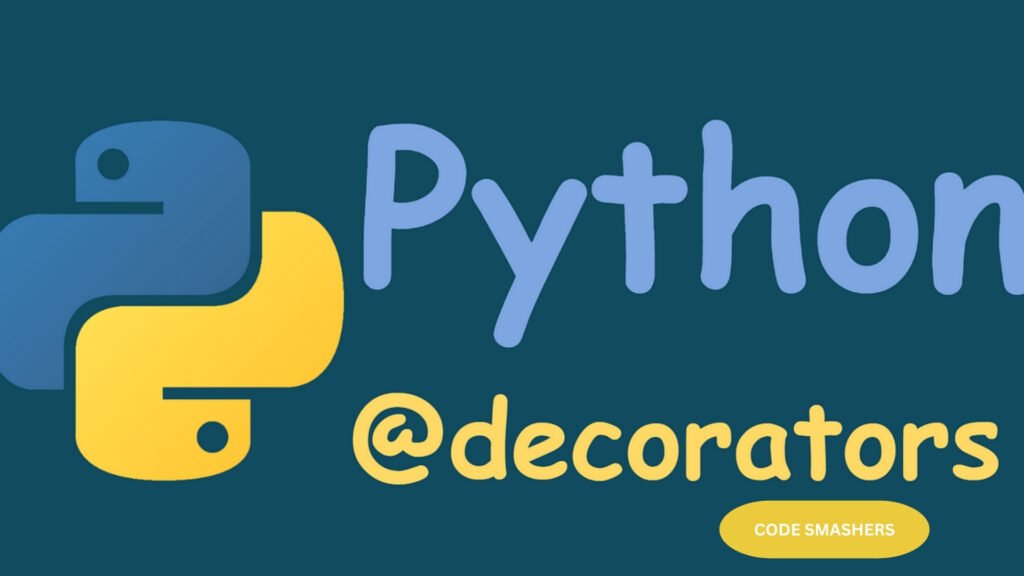
Best Practices and Common Pitfalls of Decorator Usage
Decorators are strong tools, so take caution when using them. Observe the following recommended practices:
- Keep Decorators Simple:
Ensure your decorators are easy to understand and maintain. Complex decorators can lead to confusion and bugs.
- Use Decorators Consistently:
Consistently apply decorators across your codebase to maintain readability and uniformity.
- Document Your Decorators:
Provide clear documentation for your decorators, explaining what they do and how they should be used. This practice helps maintain and share your code. Common pitfalls to avoid include:
- Overusing Decorators:
Too many decorators can complicate your code. Utilize them only when they are truly beneficial.
5. Ignoring Function Signatures:
Ensure your decorators preserve the original function’s signature using `functools.wraps` to avoid unexpected behavior.
6. Neglecting Performance:
Be mindful of the performance impact of your decorators. Inefficient decorators can slow down your program significantly.
Conclusion: The Power of Python Decorators
Python decorators offer a remarkable way to enhance your functions without altering their core logic. By understanding and implementing decorators, you can write more efficient, readable, and maintainable code. Whether you’re logging function calls, validating inputs, or stacking multiple decorators for complex tasks, the possibilities are endless.
By mastering decorators, you unlock a new level of coding proficiency that can set you apart as a Python developer. Experiment with decorators in your projects and see how they can simplify and enhance your codebase.
Call to Action
Are you prepared to increase your coding abilities? Try incorporating Python decorators into your next project, and experience their benefits firsthand.