Introduction to Tuples and Sets in Python
If you’re a programmer or data scientist working with Python, you’re likely familiar with its broad range of data types. Each type has unique characteristics that make it suitable for different tasks. Among these data types, tuples and sets stand out for their unique properties and diverse applications. In this blog post, we’ll explore the ins and outs of these data types, understanding their distinctions, practical applications, and best practices.
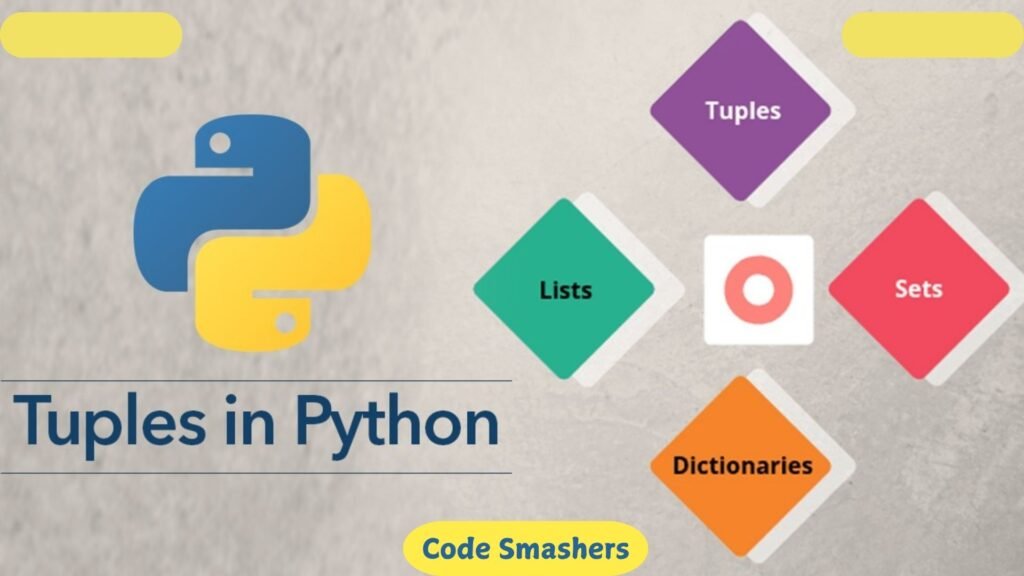
Data types in Python can be broadly categorized as either immutable or mutable. Lists and dictionaries are examples of mutable data structures that permit changes after they are created. On the other hand, immutable data types like strings and tuples cannot be altered once created. Sets, while mutable, have unique properties that distinguish them from other information types. By the end of this post, you’ll have a solid grasp of tuples and sets and how to leverage them effectively in your Python projects.
Understanding Tuples in Python
Definition and Characteristics of Tuples
As one of Python’s immutable data types, tuples’ contents are unchangeable once they are generated. They are therefore the preferred option for storing data that must not change while the program is running. Parentheses `()` are used to define tuples, which can hold elements of various data types such as strings, floats, integers, and other tuples.
Tuple Creation, Indexing, and Slicing
Creating a tuple is straightforward. You just put the elements in parenthesis and list them, separating them with commas.
For example:
my_tuple = (1, 2, 3, “apple”, “banana”)
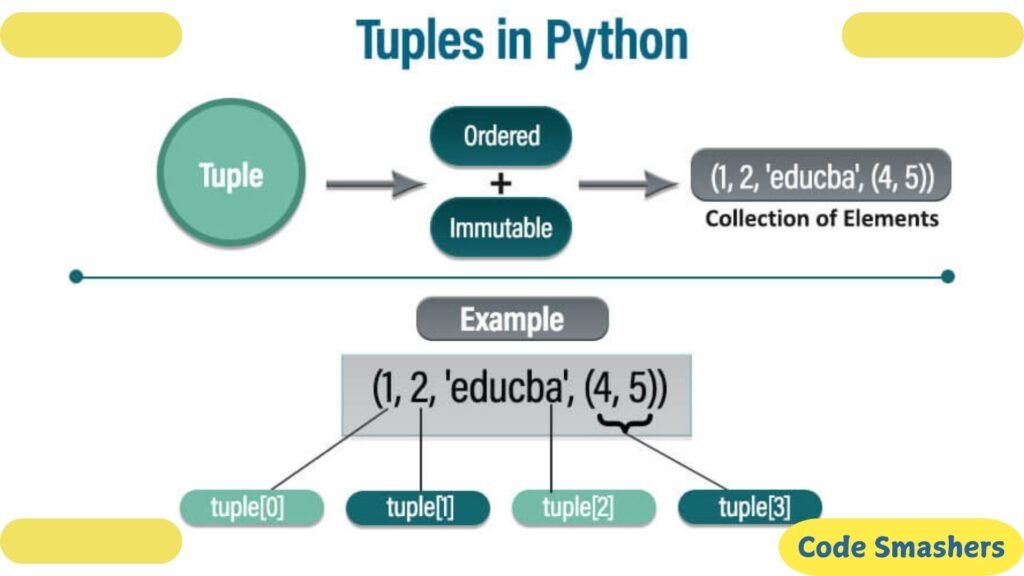
Once created, you can access tuple elements using indexing. For instance, `my_tuple[0]` returns `1`, and `my_tuple[3]` returns `”apple”`. Tuples also support slicing, allowing you to retrieve a subset of elements using the colon `:` operator:
my_slice = my_tuple[1:4]
This would yield `(2, 3, “apple”)`.
Benefits of Using Tuples
Tuples are incredibly useful for various scenarios. Their immutability ensures data safety, which is essential in applications where the integrity of the data must be maintained. Additionally, tuples are more memory-efficient than lists, making them a better choice for storing large amounts of data that do not require modification.
Working with Sets in Python
Definition and Characteristics of Sets
Sets are another powerful data type in Python, known for storing unordered collections of unique elements. Unlike lists or tuples, sets do not allow duplicate values. They are defined using curly braces `{}` or the `set()` function and can hold elements of different data types.
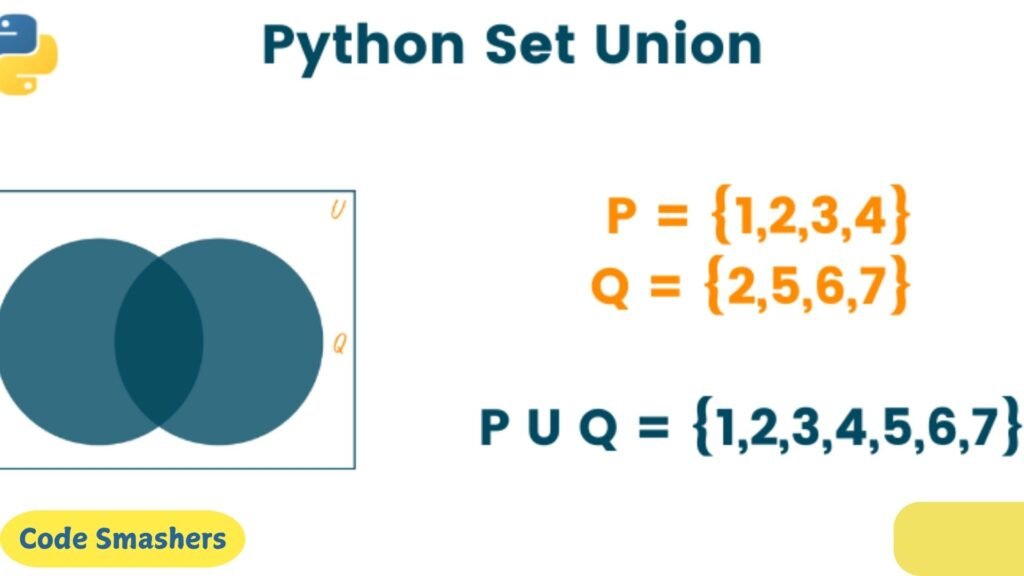
Set Operations, Methods, and Use Cases
Sets are extremely versatile since they offer a variety of operations and approaches. Union, intersection, difference, and symmetric difference are examples of common operations. For example:
set_a = {1, 2, 3}
set_b = {3, 4, 5}
Union
union_set = set_a | set_b # {1, 2, 3, 4, 5}
Intersection
intersection_set = set_a & set_b # {3}
Difference
difference_set = set_a – set_b # {1, 2}
Symmetric Difference
sym_diff_set = set_a ^ set_b # {1, 2, 4, 5}
Sets are particularly beneficial in scenarios where you need to ensure the uniqueness of elements, such as removing duplicates from a list or performing membership tests.
Practical Applications of Sets
In real-world applications, sets can be used for various tasks. One common use case is filtering out duplicate entries from a dataset. For example, if you have a list of email addresses with duplicates, converting it to a set will automatically remove the duplicates.
emails = [“test@example.com”, “user@example.com”, “test@example.com”]
unique_emails = set(emails)
This yields `{“test@example.com”, “user@example.com”}`.
Practical Applications of Tuples and Sets
Real-World Examples of Using Tuples and Sets in Python
Tuples and sets can be applied in various real-world scenarios. For instance, tuples are often used to store fixed sets of information, such as coordinates `(x, y, z)` or RGB color values `(255, 0, 0)`. Sets, on the other hand, are ideal for tasks that require unique elements, such as maintaining a collection of student IDs or processing large datasets to eliminate duplicates.
Advantages and Limitations of Using These Data Types
Tuples offer the advantage of immutability, making them perfect for read-only data. However, this also means they cannot be modified once created, which can be a limitation in some cases. Sets are highly efficient for membership tests and ensuring uniqueness, but their unordered nature means you can’t rely on element order.
By understanding the strengths and weaknesses of these data types, you can make informed decisions about when to use tuples and sets in your Python projects.
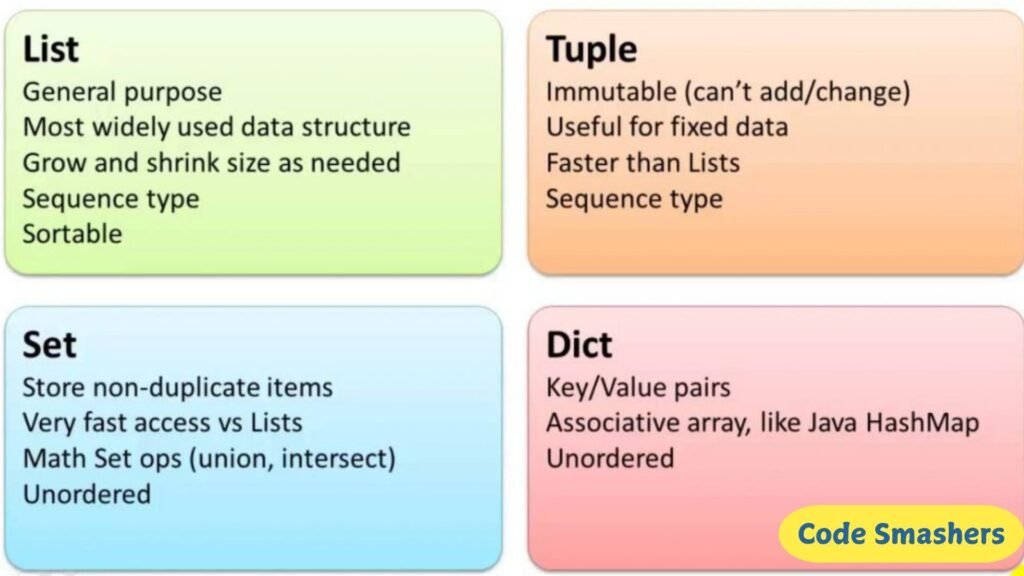
Best Practices and Tips for Working with Tuples and Sets
Guidelines for Optimizing Code Performance
When working with tuples and sets, there are several best practices to remember. For tuples, use them for fixed data sets to leverage their immutability and performance benefits. For sets, take advantage of their unique element property and use set operations to simplify your code.
Common Errors and How to Avoid Them
Common errors include attempting to modify a tuple or assuming sets maintain element order. To avoid these pitfalls, remember that tuples are immutable and sets are unordered. Additionally, ensure you understand the specific methods and operations available for each data type to avoid runtime errors.
Leveraging Tuples and Sets in Advanced Python Projects
For advanced Python projects, tuples can be used as keys in dictionaries due to their immutability, providing a powerful way to create complex data structures. Sets can be used alongside other data types to perform efficient membership tests and intersections, making them invaluable for tasks involving large datasets.
Conclusion
In summary, tuples and sets are powerful data types in Python with unique properties and versatile applications. Tuples offer immutability and efficiency, making them ideal for storing fixed data sets. Sets ensure element uniqueness and provide efficient membership tests and operations.
By understanding the characteristics, practical applications, and best practices for using tuples and sets, you can enhance your Python programming skills and optimize your code for better performance. Keep searching these data types and experimenting with different use cases to deepen your understanding.
If you found this blog post helpful, please share it with your network and continue learning about the fascinating world of Python programming. Happy coding!