Python is a versatile language that has gained immense popularity among developers and tech enthusiasts. One of its most powerful built-in functions is `range()`, which is essential for creating sequences of numbers and iterating through them. Whether you’re a beginner or an experienced Python programmer, understanding the `range()` function can enhance your coding efficiency and effectiveness. In this blog post, we will explore the basics of the `range()` function, its parameters, and various applications in Python programming. You will have a thorough grasp of how to use range()` to build code that is cleaner and more effective by the time you finish reading this article.
Introduction to Python’s Range Function
A built-in Python function that creates a sequence of numbers is called `range()`. It is frequently used in for loops to iterate over a sequence of numbers without explicitly creating a list. This function is a crucial tool for anyone looking to master Python programming.
In essence, `range()` allows you to create a sequence of numbers with a specified starting point, stopping point, and step (increment or decrement). This makes it extremely versatile and useful for a variety of programming tasks.
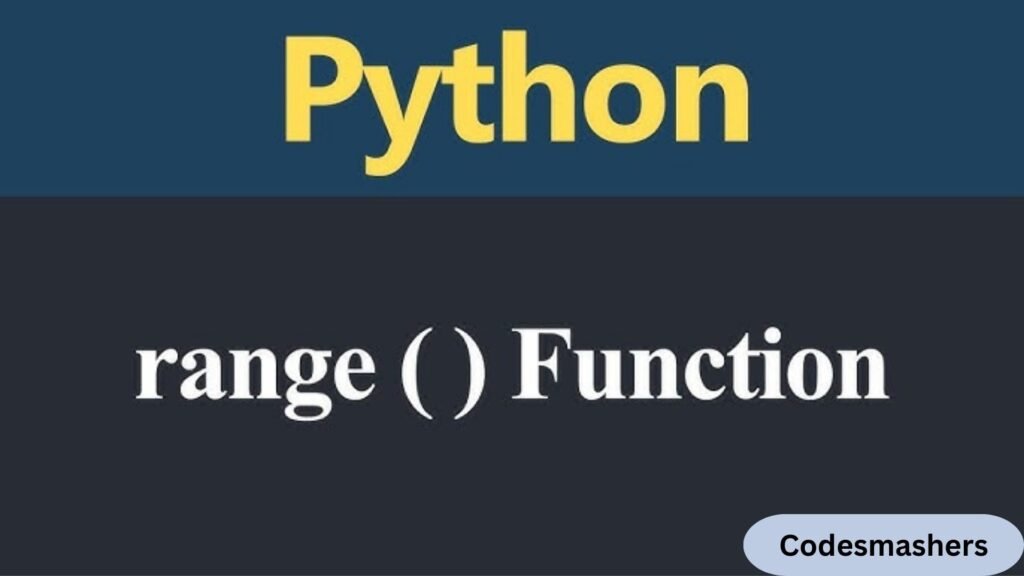
Understanding the Basics of Range Function
Before we dive into more complex applications of python, it’s important to understand the basic parameters of the `range()` function. The `range()` function consists of three parameters:
- Start: The starting value of the sequence. If omitted, the sequence starts at 0 by default.
- Stop: The end value of the sequence. The sequence will stop just before reaching this value.
- Step: The amount added to each consecutive number in the sequence. If omitted, it defaults to 1.
Examples of Basic Usage
To illustrate, let’s look at some simple examples:
- `range(5)` generates a sequence from 0 to 4 (start = 0, stop = 5, step = 1).
- `range(2, 10, 2)` generates a sequence from 2 to 8, stepping by 2 each time (start = 2, stop = 10, step = 2).
- `range(10, 2, -1)` generates a sequence from 10 to 3, stepping backward by 1 (start = 10, stop = 2, step = -1).
Understanding these parameters and their interactions is essential for effectively using the `range()` function to create and manipulate sequences.
Utilizing Range Function in For Loops
For loops are a fundamental aspect of Python, and the `range()` function is often used to define the iteration sequence. This is very helpful when you have a task that needs to be completed a certain number of times.
Example of Using Range in For Loops
Take a look at the sample that prints even numbers between 2 and 20:
for i in range(2, 21, 2):
print(I)
In this example, `range(2, 21, 2)` generates a sequence starting at 2, ending before 21, and incrementing by 2. The for loop outputs each integer each time this sequence is iterated over.
Another common use case is iterating over a sequence of indices to access elements in a list:
my_list = [‘apple’, ‘banana’, ‘cherry’]
for i in range(len(my_list)):
print(my_list[I])
Here, `range(len(my_list))` generates a sequence from 0 to the length of the list, allowing us to access each element by its index.
Leveraging Range Function with Iterators
An object that can be iterated over in Python is called an iterator (you may traverse over all the values). The `range()` function itself returns a range object, which is iterable.
Creating Custom Iterators with Range
You can use the `range()` function to create custom iterators. One may want to loop over a list in the opposite order, for example:
my_list = [1, 2, 3, 4, 5]
for i in range(len(my_list) – 1, -1, -1):
print(my_list[I])
In this case, `range(len(my_list) – 1, -1, -1)` creates a sequence starting from the last index and decrementing to 0, allowing you to iterate through the list in reverse.
Understanding how `range()` works with iterators can help you write more flexible and efficient code.
Performance Considerations and Best Practices
When working with large sequences, performance becomes a critical consideration. The `range()` function is memory-efficient, as it generates numbers on the fly rather than storing them in a list.
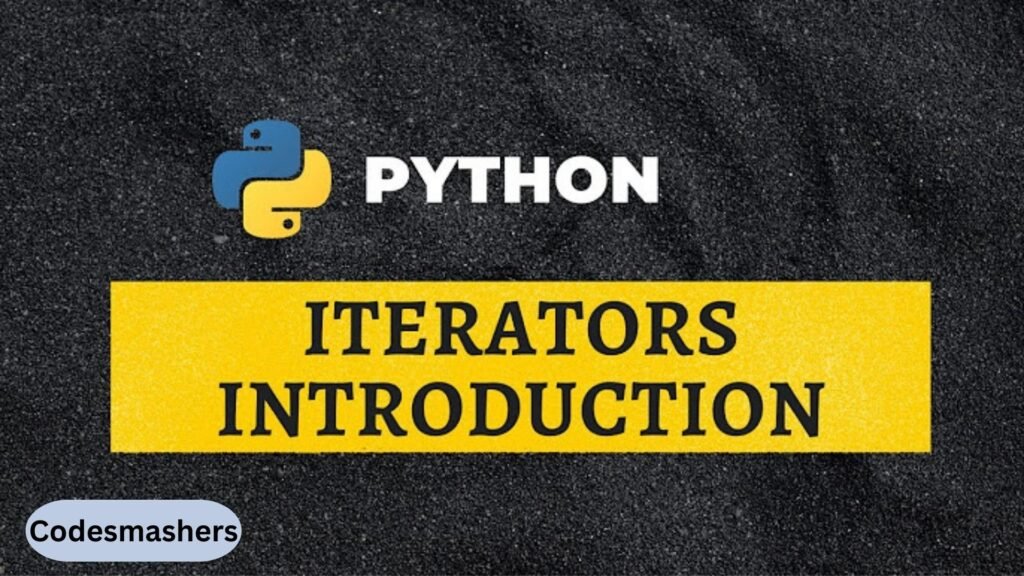
Memory Efficiency
Think about the distinction between making a list of numbers and using `range()}:
Using range()
for i in range(1000000):
pass
Using a list
for i in list(range(1000000)):
pass
The first example is more memory-efficient because `range()` generates the numbers on the fly, whereas the second example creates a list of one million numbers in memory.
Best Practices
- Use `range()` for Iteration: Always use `range()` instead of creating lists for iteration, especially when dealing with large sequences.
- Optimize Step Values: Choose appropriate step values to minimize the number of iterations and improve performance.
- Avoid Unnecessary Conversions: Avoid converting range objects to lists unless absolutely necessary to conserve memory.
By keeping these best practices in mind, you can use the `range()` function effectively and efficiently in your Python programs.
Real-world Use Cases and Examples
The `range()` function has numerous real-world applications. Here are some practical examples to illustrate its versatility:
Generating Sequences
You can use `range()` to generate sequences of numbers for various purposes, such as creating a series of timestamps or generating test data.
Counting Prime Numbers
Consider a program that counts prime numbers within a given range:
def is_prime(n):
If n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
If n% i == 0:
return False
return True
count = 0
for num in range(2, 100):
if is_prime(num):
count += 1
print(“Number of prime numbers:”, count)
In this example, `range(2, 100)` generates a sequence of numbers from 2 to 99, and the program counts the prime numbers within this range.
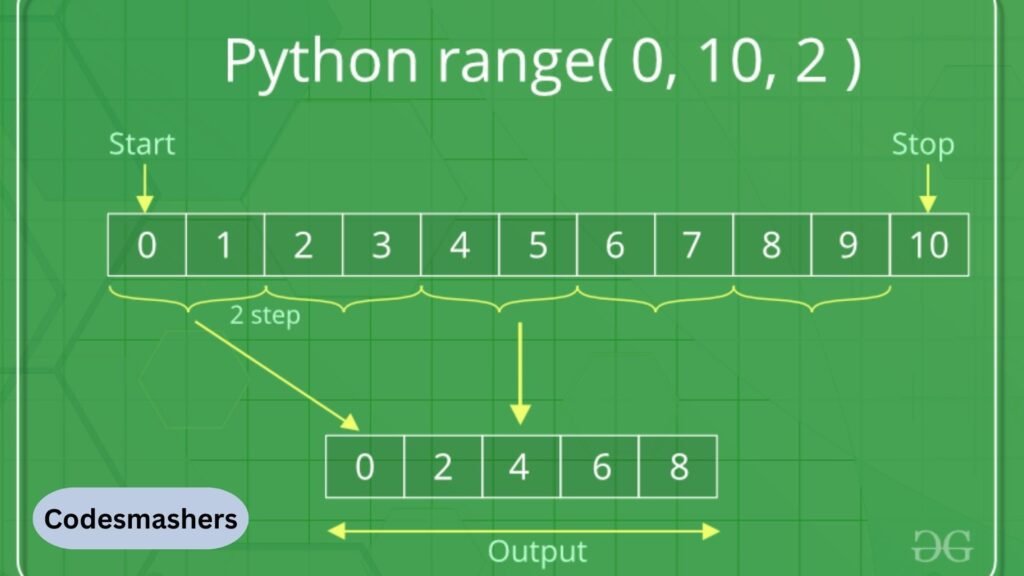
Iterating Over Collections
You can also use `range()` to iterate over collections, such as lists or dictionaries, to perform various operations:
students = {‘Alice’: 85, ‘Bob’: 90, ‘Charlie’: 78}
for i in range(len(students)):
print(list(students.keys())[i], list(students.values())[i])
This example demonstrates how to use `range()` to iterate through the keys and values of a dictionary.
Common Mistakes with the `range()` Function
When working with the `range()` function in Python, programmers may encounter a few common pitfalls that can lead to unexpected behavior or errors in their code. Being aware of these mistakes can help prevent issues and lead to more robust scripts.
Off-by-One Errors
One of the most frequent mistakes is the off-by-one error, where the end value of a range is mistakenly included. Since the `range()` function generates numbers up to but not including the stop value, forgetting this can cause logical errors. For instance, attempting to iterate through a sequence with `range(1, 10)` will result in a range from 1 to 9, not 1 to 10.
Incorrect Step Values
Setting incorrect step values can also lead to errors. This mistake typically occurs when the step value needed is negative for decrementing sequences and is either omitted or incorrectly specified. For example, using `range(10, 1)` will not result in a descending sequence; instead, the step should be explicitly set like `range(10, 1, -1)`.
Improper Use in Conditional Logic
Using `range()` directly within conditions can sometimes result in logical errors. When attempting to check membership or specific conditions, it’s essential to convert the `range` object into a list (if necessary) or use appropriate logic to ensure conditions are evaluated correctly.
Unnecessary Memory Usage
A common mistake is converting a `range` object into a list without necessity, leading to unnecessary memory allocation. Given that `range()` is inherently memory-efficient, converting it with `list()` should be avoided unless necessary.
By recognizing these typical errors, developers can make better use of the `range()` function and enhance the accuracy and performance of their programs.
Advanced Performance Tips
- Leverage Built-in Functions: Whenever possible, use Python’s built-in functions or libraries such as NumPy for numerical operations and data manipulation. These are typically optimized for performance, reducing the overhead of custom implementations.
- Avoid redundant calculations: compute values once and reuse them instead of recalculating them inside loops. For instance, cache the result of a frequently accessed computation outside a loop rather than recalculating it repeatedly.
- Effective Use of Generators: Python language Use generator expressions instead of list comprehensions when dealing with large data sets, as they provide a method to iterate through data without storing it all in memory at once.
- Profile Your Code: Utilize Python’s profiling tools, such as `cProfile`, to identify performance bottlenecks in your code. This analysis can guide the optimization of specific sections that are particularly resource-intensive.
- Optimize Data Structures: Choose the right data structures according to usage patterns. For instance, prefer sets for membership checks due to their O(1) time complexity, and lists are preferred for ordered sequences that will be frequently traversed.
Implementing these advanced techniques can aid in achieving optimal performance, especially in python programs that handle significant amounts of data or require efficient execution time.
Conclusion
Python’s `range()` function is an effective tool for developers to easily build and work with numerical sequences. Its applications and parameters will help you write code that is more organized and effective.
In this guide, we’ve explored the basics of the `range()` function, its use in for loops, its interaction with iterators, and best practices for performance. We’ve also provided practical examples to illustrate its real-world applications.
We encourage you to experiment with the `range()` function in your own projects and explore its full potential. If you have any questions or would like to share how you’ve used `range()` in your code, feel free to leave a comment or get in touch. Happy coding!